To upload a folder to a remote Git repository, you first initialize a local repository, add the desired folder, commit the changes, and then push them to the remote repository using the following commands:
git init
git add <folder_name>
git commit -m "Add folder_name"
git remote add origin <remote_repository_URL>
git push -u origin master
Understanding Git Basics
What is Git?
Git is a powerful version control system that allows developers to track changes in their code, collaborate with others, and manage project versions efficiently. Unlike centralized version control systems, Git tracks changes locally and enables smooth operation even when offline.
Key Concepts in Git
To effectively utilize Git and successfully upload a folder using Git commands, it is essential to understand a few key concepts:
Commits are snapshots of your project that record changes over time. Each commit is like a historical marker allowing you to revert to previous states if necessary. The ability to manage commits properly is crucial for any successful workflow.
Branches enable multiple lines of development. They allow you to work on new features or fixes without affecting the main codebase. This helps facilitate collaboration among multiple developers.
Remote Repositories are hosted versions of your local repository. They give you a shared space to push your updates and pull changes made by others. Some popular platforms for hosting Git repositories include GitHub, GitLab, and Bitbucket.
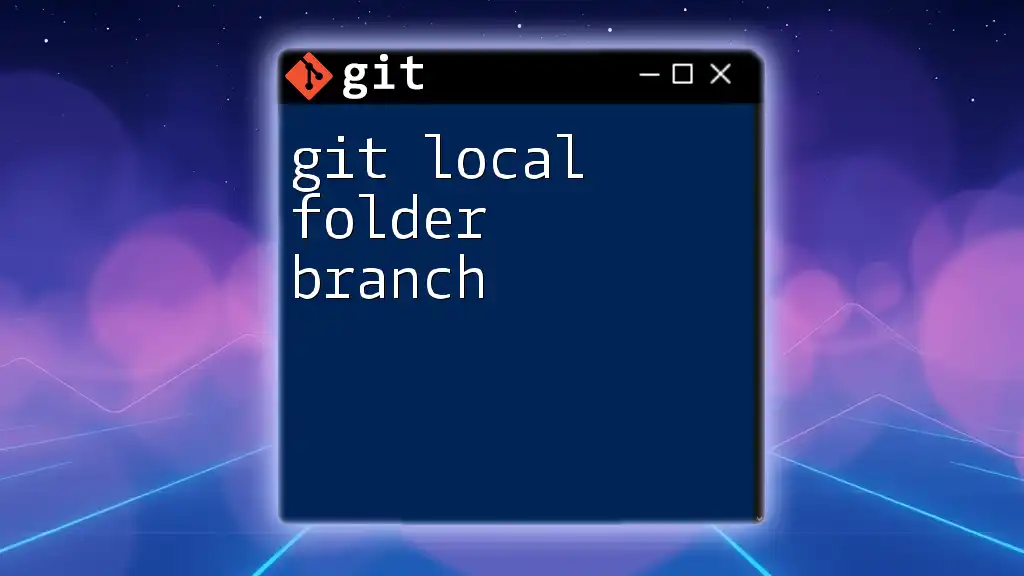
Preparing Your Environment
Setting Up Git
Before you can upload a folder, you need to set up Git on your computer. Follow these steps based on your operating system:
- Windows: Download the Git installer from the official Git website, run the installer, and follow the setup instructions.
- macOS: Use Homebrew and run:
brew install git
- Linux: Use your package manager, for example:
sudo apt-get install git # For Debian/Ubuntu based distributions
Once installed, verify that Git is working by running:
git --version
This command displays the installed version of Git, indicating the setup was successful.
Creating a New Repository
After installing Git, you can create your new repository. To initialize a Git repository, open your terminal and run:
git init my-repo
cd my-repo
This command creates a new directory named `my-repo` and initializes a new Git repository inside it.
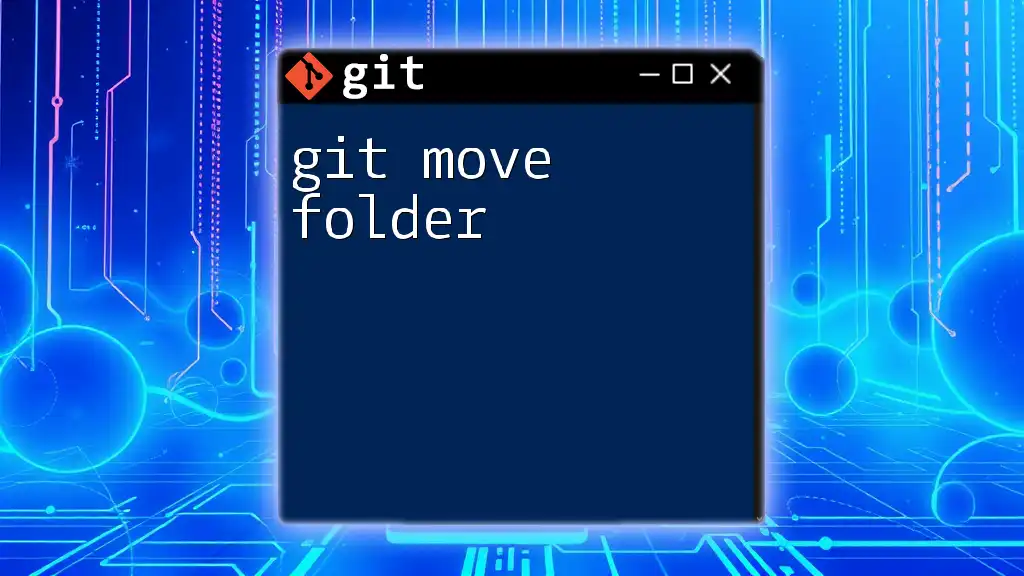
Uploading a Folder to Git
Adding Files to the Repository
Git tracks changes to files in your repository, and before uploading anything, you need to tell Git which files to include.
You can use the `git add` command to stage changes. To add specific files:
git add <file-path>
If you want to add all changes in the current directory, simply use:
git add .
This command stages all new, modified, or deleted files, making it easier to prepare everything for your next commit.
Committing Changes
Once you've staged your desired files, it’s time to create a commit. A commit serves as a checkpoint in your project history. It’s essential to write clear, descriptive commit messages. For example:
git commit -m "Initial commit with project folder"
A solid commit message provides context and makes it easier to understand the project’s history.
Pushing to a Remote Repository
Setting Up a Remote Repository
To upload your folder to a remote repository, first, you need to create a new repository on a hosting platform such as GitHub. After creating it, you'll receive a remote repository URL.
Adding a Remote URL
To link your local repository to the remote repository, you must add the remote URL using:
git remote add origin <remote-repo-URL>
This command creates a reference named `origin` that points to your newly created remote repository.
The Push Process
Now that you've set up the remote repository and associated it with your local repository, you're ready to upload your changes.
To push your changes to the remote repository, use:
git push -u origin master
This command pushes your committed changes to the `master` branch of the remote repository. The `-u` flag sets the upstream tracking, making future push and pull commands easier.
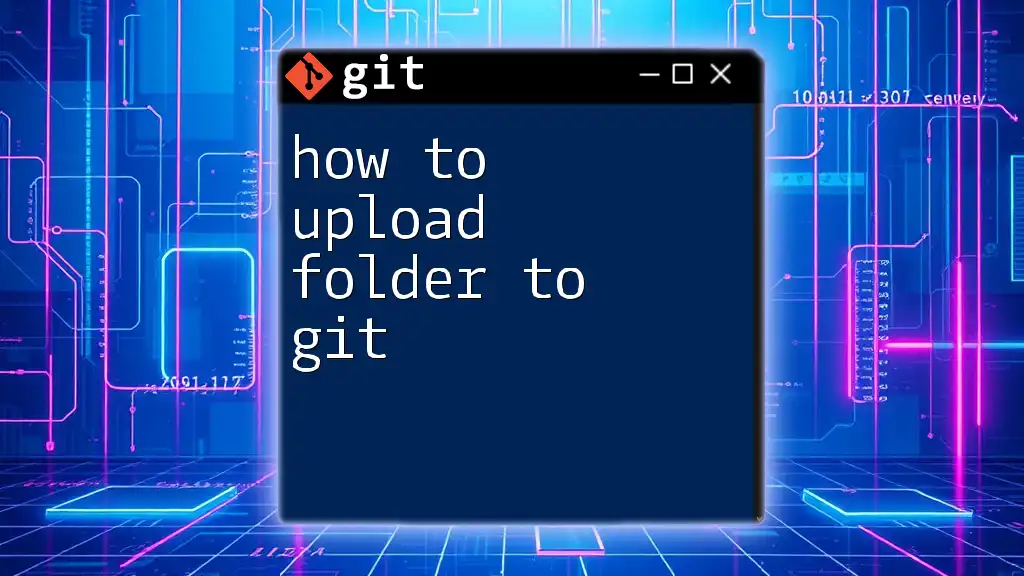
Best Practices for Organizing Your Uploads
Structuring Your Project
To enhance collaboration and maintainability, it’s essential to organize your project structure logically. A well-structured project typically includes:
- A `README.md` file to help others understand your project.
- A `src` folder for source code.
- A `docs` folder for documentation.
Using .gitignore
The .gitignore file tells Git which files or directories to ignore when staging and committing changes. This is especially useful for excluding unnecessary files such as build artifacts, log files, or dependency caches.
For instance, a typical `.gitignore` file might look like this:
*.log
node_modules/
dist/
By creating a `.gitignore` file for your project, you prevent these files from being tracked in your Git history.
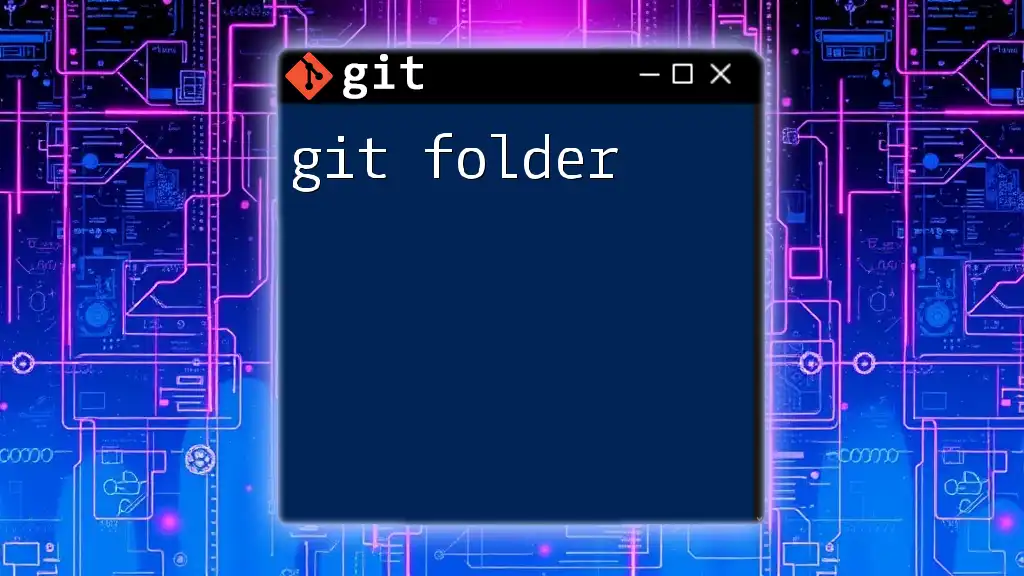
Troubleshooting Common Issues
Authentication Errors
Sometimes you may encounter authentication errors while pushing to a remote repository. Ensure that your Git credentials are correctly configured. If necessary, run:
git config --global credential.helper cache
This command will cache your credentials for a specified time, allowing easier pushes without repeated logins.
Merge Conflicts
Merge conflicts occur when multiple people make changes to the same lines of code. To better avoid conflicts, regularly pull the latest changes from the remote repository before starting new work.
Large Files and Git LFS
When dealing with large files, traditional Git may not perform optimally. Git Large File Storage (LFS) is a solution for managing large files. You can install Git LFS and track specific file types using commands like:
git lfs install
git lfs track "*.psd"
In this case, all Photoshop files (`*.psd`) would be managed by Git LFS.
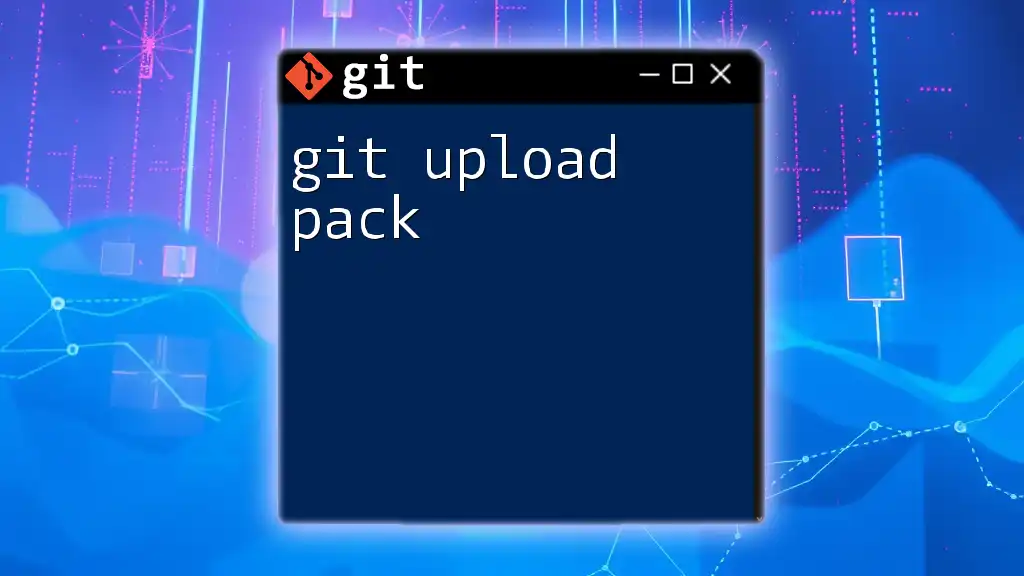
Conclusion
Uploading a folder to a Git repository is a straightforward process once you grasp the fundamental concepts of Git. Following the outlined steps from setting up your environment to carefully managing your commits can enhance both individual and team productivity. By adhering to best practices in project organization and using tools like `.gitignore` and Git LFS, you can significantly streamline your workflow.
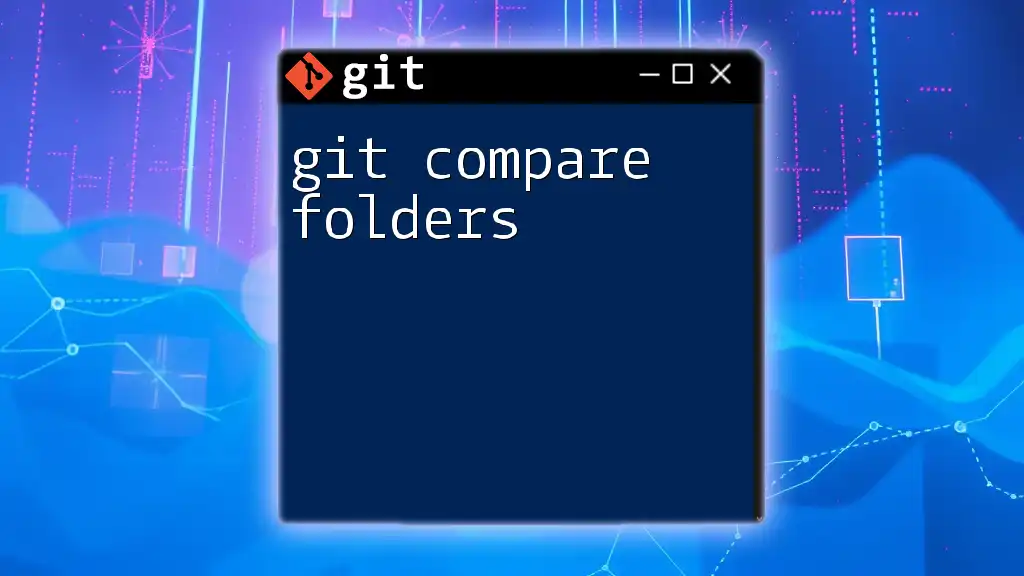
Additional Resources
To further refine your Git knowledge, consider exploring the official Git documentation and robust online tutorials focusing on advanced Git features. This will enable you to utilize Git’s full potential and improve your project management skills dramatically.