To download a specific folder from a Git repository without cloning the entire repository, you can use the following command with `git sparse-checkout` feature after initializing a new repository.
git init
git remote add origin <repository-url>
git sparse-checkout init --cone
git sparse-checkout set <folder-name>
git pull origin <branch-name>
Replace `<repository-url>`, `<folder-name>`, and `<branch-name>` with the appropriate values for your repository.
Understanding Git Repositories
What is a Git Repository?
A Git repository is a structure that stores metadata for a set of files or directory structure, typically as part of a version control system. In Git, this repository can be local on your computer or hosted on platforms like GitHub, GitLab, or Bitbucket. Within a repository, folders help organize code, assets, and documentation into a coherent structure, making it easier to manage projects collaboratively.
Cloning vs. Downloading
When dealing with Git, it’s essential to differentiate between cloning a repository and downloading a specific folder. Cloning involves creating a local copy of the entire repository, which includes all the branches and commit history. This is accomplished using the `git clone` command.
On the other hand, downloading just a specific folder can be advantageous if the repository is large or if you only need a particular section of it. This guide focuses on how to download a folder from Git, minimizing the amount of data you need to handle.
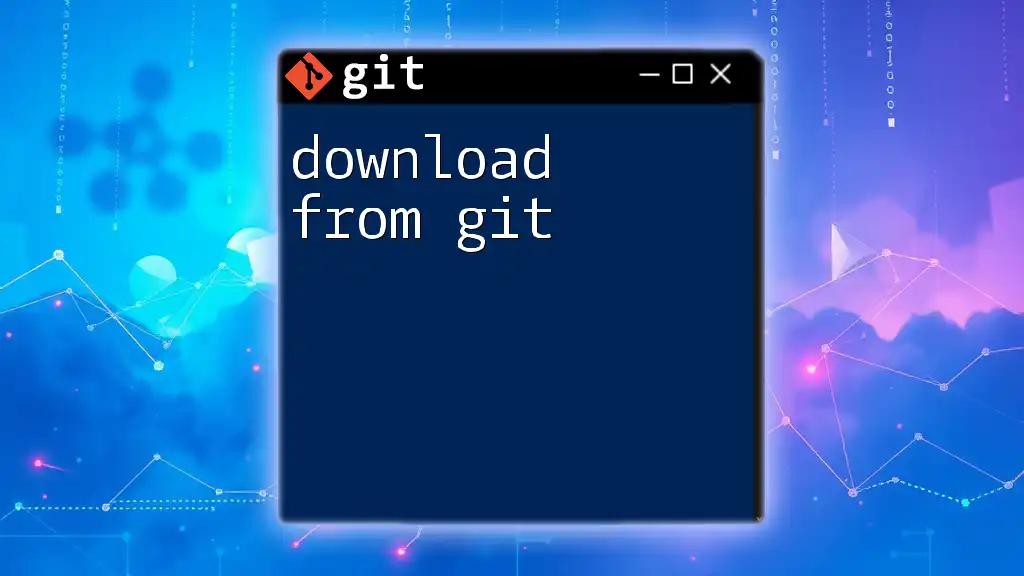
Methods to Download a Folder from Git
Using Git Commands
Prerequisites
Before proceeding, ensure you have Git installed on your machine. You can check this by running the following command in your terminal:
git --version
If Git isn’t installed, follow the official installation instructions for your operating system. Also, set your username and email if this is your first time using Git:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Downloading a Single Folder with Sparse Checkout
One effective method to download a folder from Git is by using sparse checkout. This method allows you to clone a repository without checking out all the files, which is particularly useful when dealing with large repositories.
-
Clone the Repository: Use the `--no-checkout` option to clone the repository without checking out any files:
git clone --no-checkout <repository-url>
-
Navigate to the Repository Directory:
cd <repository-name>
-
Enable Sparse Checkout: Initialize sparse checkout feature:
git sparse-checkout init --cone
-
Set the Desired Folder: Specify the folder you want to download:
git sparse-checkout set <path/to/folder>
-
Checkout the Main Branch: Finally, check out the desired branch (e.g., `main` or `master`):
git checkout main # or the appropriate branch
This method ensures that only the specified folder is downloaded, significantly reducing bandwidth and disk space usage.
Using GitHub or GitLab
Downloading Directly from the Web Interface
If you prefer not to use the command line, you can easily download a folder from Git directly through the web interface of repositories hosted on platforms like GitHub or GitLab.
- Navigate to the repository page on GitHub or GitLab.
- Locate the Desired Folder: Browse the repository content to find the folder you need.
- Access Folder Contents: Click on the folder to view its files.
- Download Option: If the platform provides a direct download option for folders, use it. If not, you may need to clone the entire repository and manually copy the folder you need.
For instance, on GitHub, while there isn’t a straightforward method to download just a folder via the web interface, you can use the "Download ZIP" option to pull down the entire repository and then extract only the needed folder from the downloaded files.
Using Third-Party Tools
GitZip for GitHub
GitZip is a convenient third-party tool that allows users to download specific folders from GitHub repositories without checking out the entire repository. It simplifies the process significantly, especially for users who may not be comfortable using command-line tools.
To use GitZip:
-
Navigate to the GitHub repository containing the folder you wish to download.
-
Use the GitZip service by entering the repository link in the format provided by GitZip. An example URL could look like this:
http://gitzip.github.io/
-
After you input the URL, select the specific folder you want to download, and GitZip will create a ZIP file for you.
This method is particularly useful when dealing with repositories that are unnecessarily large for your specific needs.
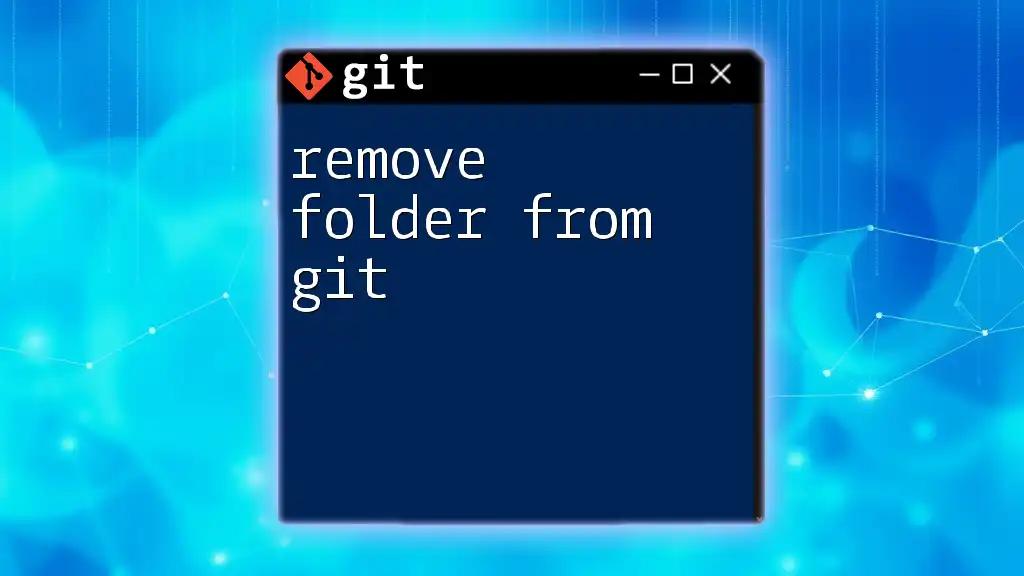
Common Issues and Troubleshooting
Permissions Issues
When trying to download a folder from Git, you may encounter issues related to permissions, especially if the repository is private. You need to ensure that you have the appropriate access rights.
If you face permission errors, verify that:
- You are logged into your Git account that has access to the repository.
- Your SSH keys or tokens are correctly set up if you are using Git over SSH.
Large Folders
Downloading large folders may lead to slow performance or timeouts, depending on your internet connection. If faced with these challenges, consider the following approaches:
- Use Git sparse checkout as described above to limit the amount of data downloaded.
- If the folder structure is particularly large, reevaluate whether you need all contents within. Sometimes, focusing on specific files can also be beneficial.
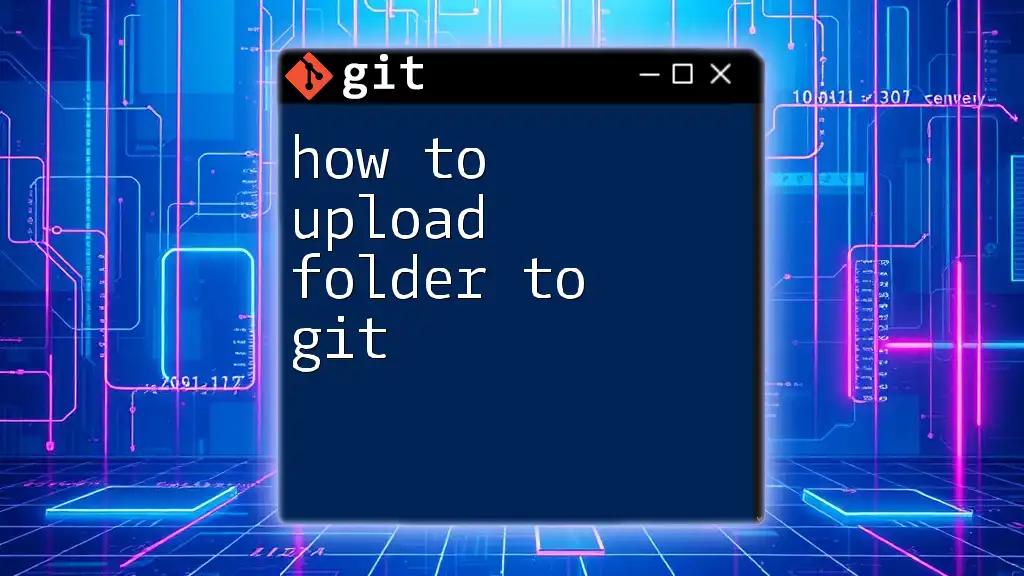
Conclusion
In conclusion, knowing how to effectively download a folder from Git is a valuable skill that can save you time and resources. Whether you choose to use Git commands, web interfaces, or third-party tools, each method offers unique advantages. By mastering these techniques, you streamline your workflow and enhance your productivity in handling Git repositories.
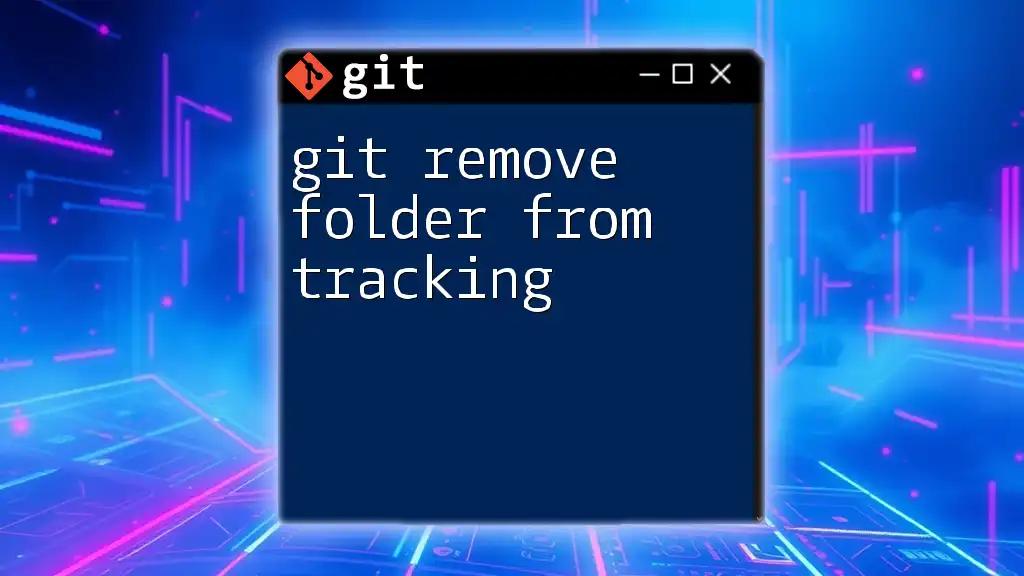
Call to Action
For more insightful Git tutorials and tricks, subscribe to our updates! Feel free to share your own experiences or questions about downloading folders from Git in the comments below.
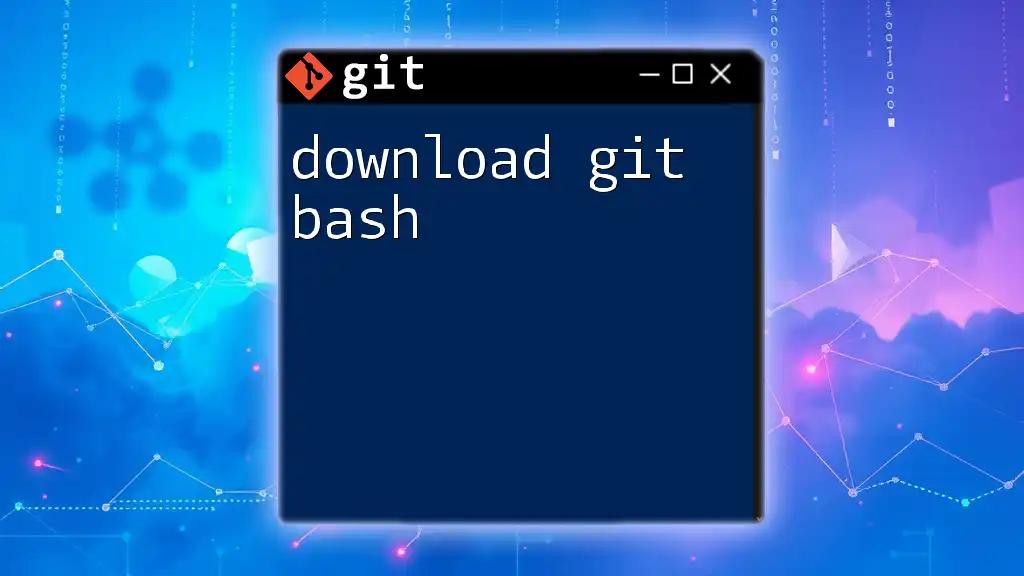
Additional Resources
For further reading, check out the official Git documentation and explore recommended tools that can enhance your Git experience. Whether you're a beginner or a seasoned developer, understanding these concepts is crucial for effective version control.