To upload a folder to Git, first initialize a Git repository in the folder, add the files, and then commit and push them to the remote repository using the following commands:
cd your-folder
git init
git add .
git commit -m "Initial commit"
git remote add origin your-remote-repo-url
git push -u origin master
Understanding Git Basics
What is Git?
Git is a distributed version control system designed to manage and track changes in source code during software development. Its main features allow individuals and teams to collaborate efficiently by providing tools for version tracking, branching, and merging. By using Git, developers can maintain a history of their work, enabling easier tracking of modifications and collaboration among team members.
Git Repositories
Understanding the concept of a Git repository is crucial. A repository can be categorized as either local or remote. A local repository exists on your own machine, while a remote repository is typically hosted on platforms like GitHub or GitLab.
Within these repositories lies the critical `.git` directory, which contains all the metadata for the repository, including commit history and configuration settings. Without this directory, Git wouldn't be able to function effectively.
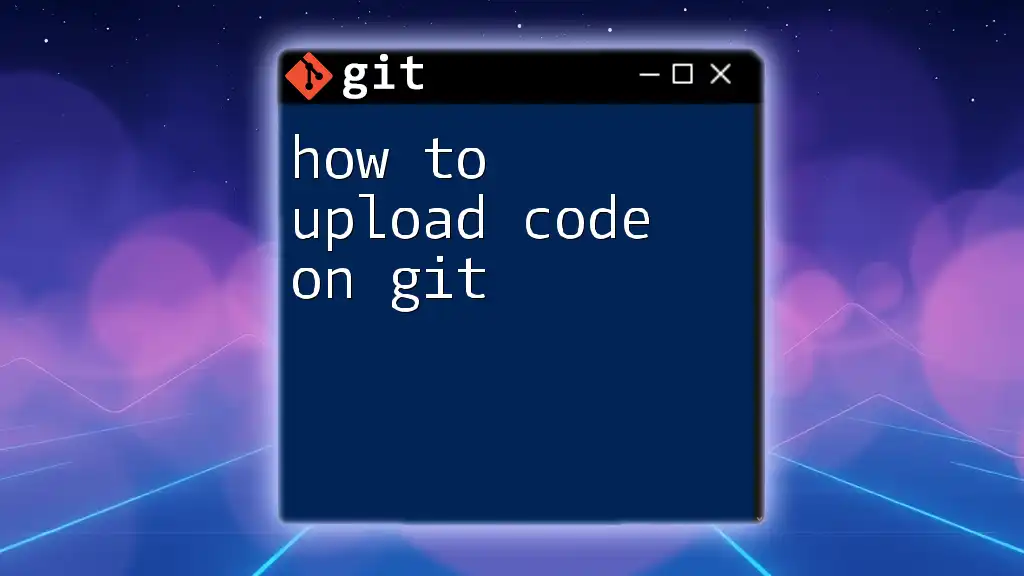
Preparing to Upload a Folder
Setting Up Git
Before uploading a folder to Git, you'll need to install it on your machine. Here’s a quick guide for various platforms:
- Windows: Download the installer from the [official Git website](https://git-scm.com/download/win) and follow the prompts.
- macOS: Use Homebrew (`brew install git`) or download the installer from the Git website.
- Linux: Use your distribution’s package manager (e.g., `sudo apt-get install git` for Ubuntu).
Once Git is installed, it's crucial to configure your environment. Set your username and email address, which are attached to your commits:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Initializing a Git Repository
After setting up Git, navigate to the folder you want to upload. If you haven't already created a repository there, you'll need to initialize one. Here’s how:
mkdir my_project
cd my_project
git init
Running `git init` creates a new directory named `.git`, which contains all the necessary files for version control.
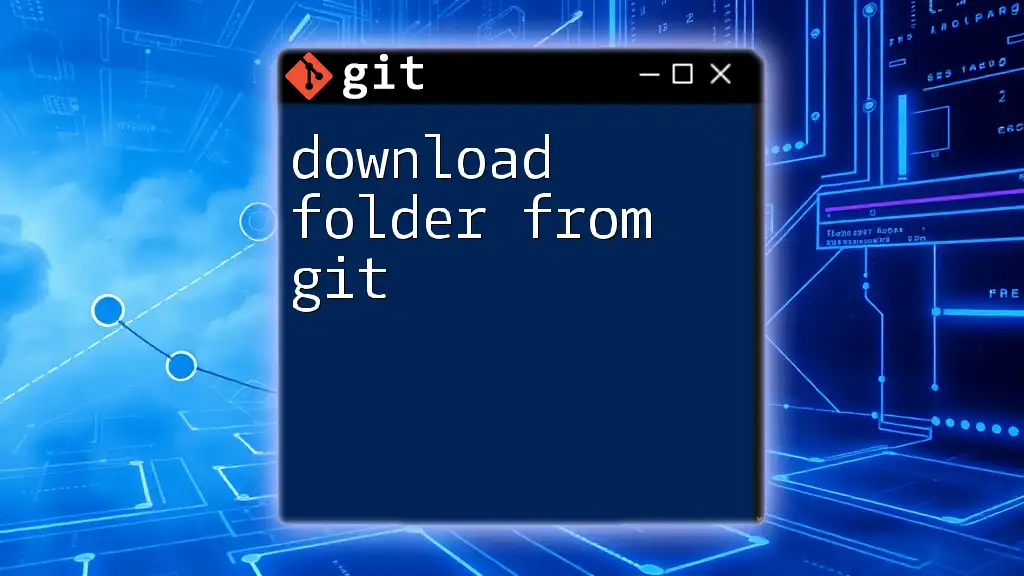
Uploading a Folder to Git
Step 1: Navigate to Your Project Folder
First, you need to change your directory to the folder you intend to upload. This is done using the `cd` command:
cd path/to/your/folder
Step 2: Initialize Git (if not already initialized)
If you haven’t yet done so, run the `git init` command in this folder. You should see a new `.git` directory, confirming that Git is tracking this folder.
Step 3: Adding Files to the Staging Area
Before you can commit your files, you need to stage them. The staging area allows you to prepare files for the next commit. You can stage all files in your folder by using:
git add .
Here, the dot (`.`) signifies that all files in the current directory should be added. It’s essential to understand what goes into this staging area, as it determines what will be included in your next commit.
Step 4: Committing Changes
Now that your files are staged, it’s time to commit your changes. This action finalizes the changes in the repository and saves a history of your project. Always try to include a meaningful commit message to describe the changes made:
git commit -m "Initial commit of my folder"
This command records your changes and makes them part of the project’s history.
Step 5: Linking to a Remote Repository
For broader collaboration, you’ll likely want to push your changes to a remote repository. This allows others to access and contribute to your project. To set this up, first, create a repository on a platform like GitHub or GitLab, then link your local repository to it:
git remote add origin https://github.com/username/repo.git
Replace `https://github.com/username/repo.git` with your actual repository URL.
Step 6: Pushing Changes to the Remote Repository
Finally, to upload your commits to the remote repository, use the `push` command:
git push -u origin master
The `-u` (or `--set-upstream`) flag informs Git to remember the upstream repository for the current branch, making it easier to push and pull changes in the future.
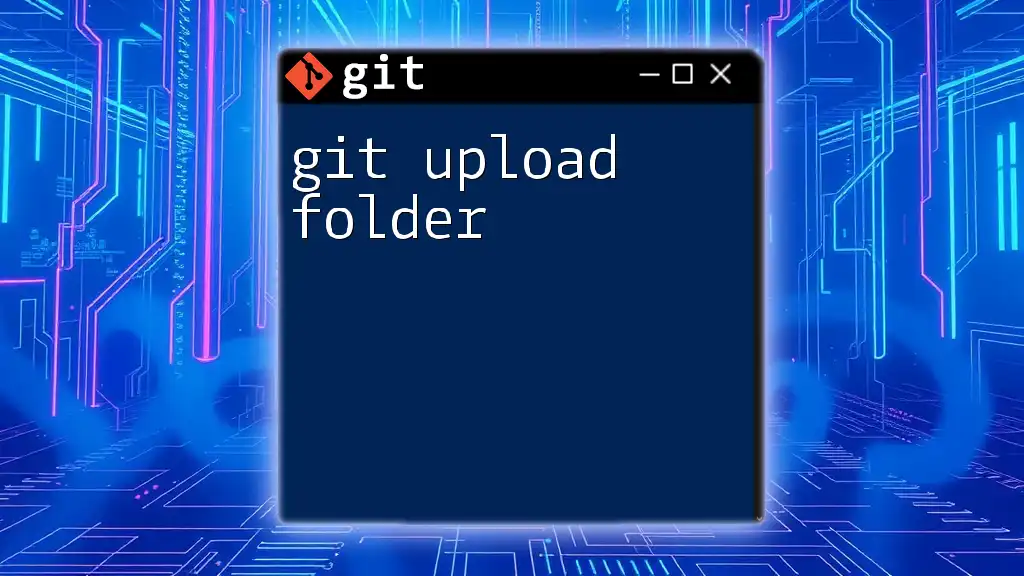
Common Issues and Troubleshooting
Authentication Problems
When pushing to a remote repository, issues may arise due to authentication failures. To resolve this, consider setting up SSH keys for secure access or using HTTPS protocols instead. For detailed setup guides, consult the documentation for your chosen Git hosting platform.
Handling Merge Conflicts
Sometimes, you might encounter merge conflicts when collaborating with others. Merge conflicts occur when different changes to the same part of a file are attempted at the same time. To resolve these conflicts, Git provides helpful tools to manually select the correct changes. Learning how to resolve conflicts can significantly enhance your collaboration effectiveness.
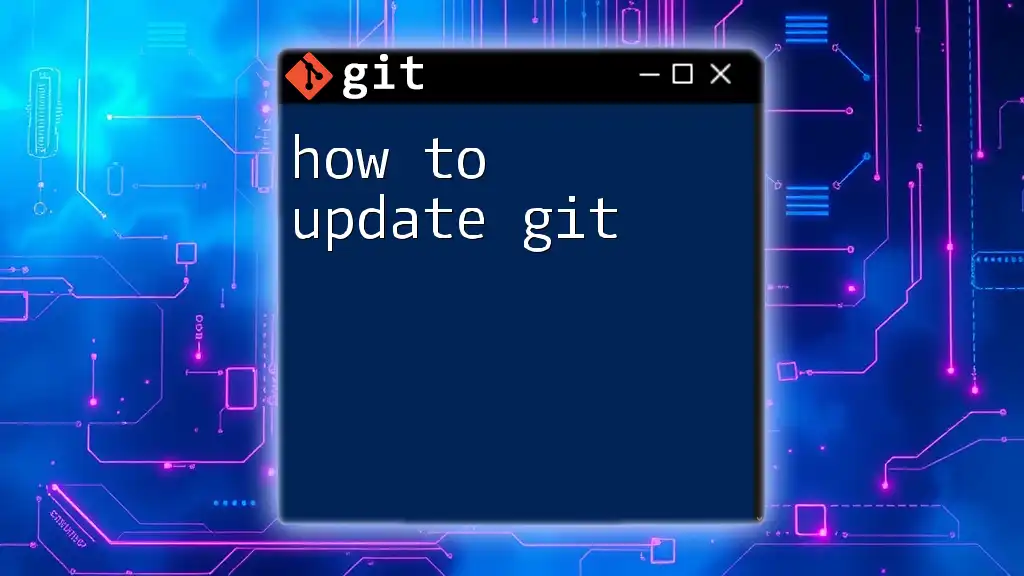
Best Practices for Using Git
Following certain best practices can help maintain a more efficient and organized project:
- Regular Commits: Make small, frequent commits, each with meaningful messages to describe the purpose of the changes.
- Clean and Organized Repository: Regularly review your project structure to keep it tidy and manageable.
- Leverage `.gitignore`: Use a `.gitignore` file to specify files and directories that Git should ignore, such as temporary files or sensitive information. Here’s an example `.gitignore` file:
node_modules/
.env
*.log
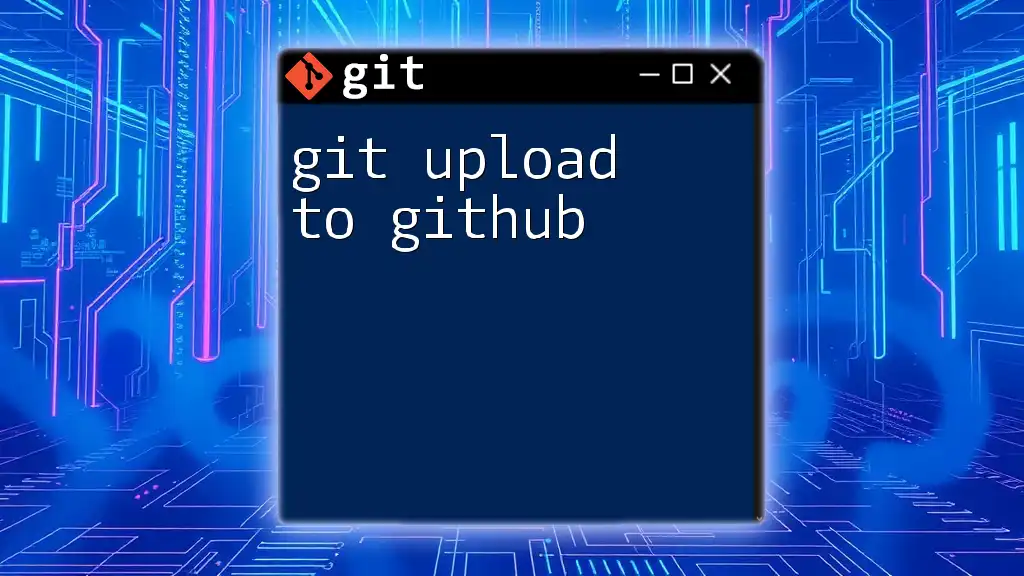
Conclusion
Uploading a folder to Git is a straightforward process that equips you with valuable tools for version control and collaboration. By following the steps outlined in this guide, you can easily manage your projects and work more effectively with others.
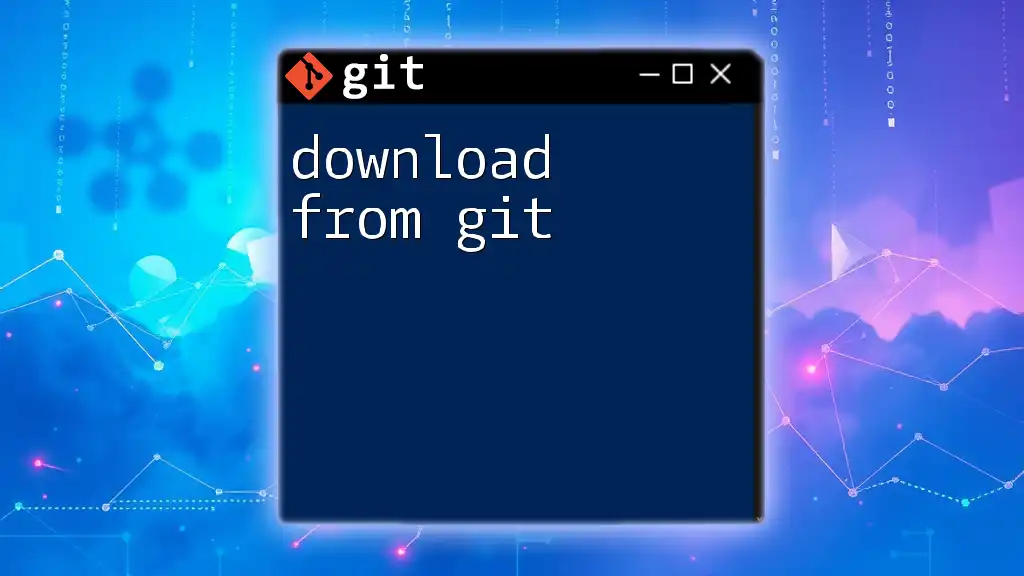
Additional Resources
To deepen your understanding of Git, you can explore the official [Git documentation](https://git-scm.com/doc), enroll in online tutorials, and engage with community forums filled with experienced Git users ready to offer insights and assistance.