To create a folder in a Git repository, you need to create the directory in your local file system and then stage it for commit using the following command:
git add folder_name/
Understanding Git Folders
What is a Folder in Git?
In the context of a Git repository, a folder (or directory) serves as a container for organizing files. Folders help in maintaining the structure of a project, categorizing files based on their functionality or purpose. It is essential to recognize that the concept of folders in a filesystem may differ from how Git interacts with them, especially considering that Git tracks changes to files rather than directories themselves.
Why Create Folders in Git?
Creating folders in Git provides several advantages:
- Enhanced Organization: Properly structured directories make it easier to locate and manage files, especially as projects grow in complexity.
- Improved Collaboration: By maintaining a clear folder structure, team members can quickly navigate the repository, find relevant files, and understand the overall organization of the project.
- Version Control Clarity: A well-organized repository allows for clearer insights into changes, making it easier to track the evolution of specific features or components.
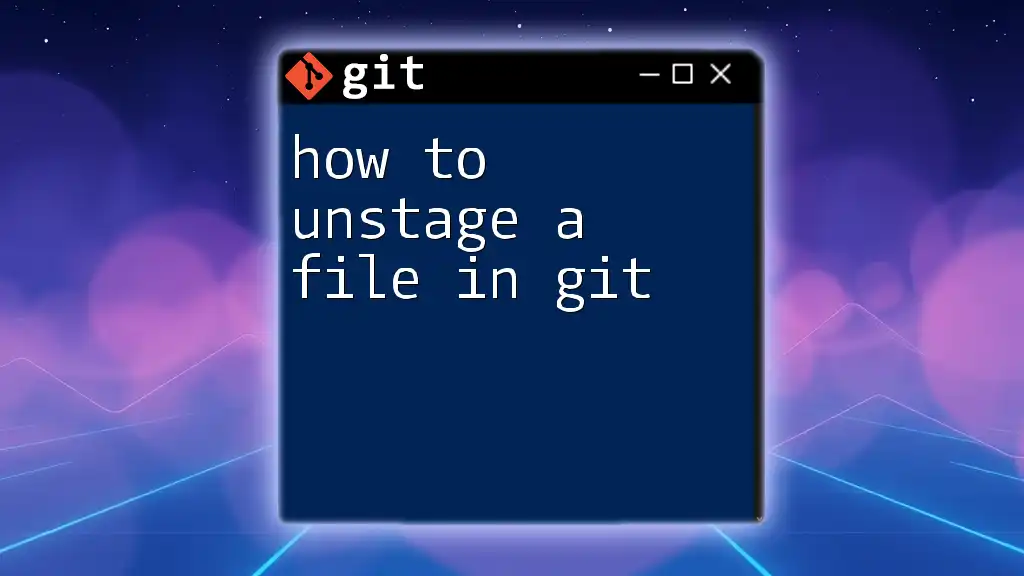
Requirements
Prerequisites
Before diving into how to create a folder in Git, ensure the following:
- You have Git installed on your local machine.
- You possess a basic understanding of Git concepts such as repositories, commits, and staging.
Command Line vs. GUI
While this article primarily focuses on command-line usage, it’s essential to recognize that Git can also be managed through various GUI tools such as GitHub Desktop, Sourcetree, or GitKraken. Each option has its pros and cons; the command line provides more control and flexibility, while GUI tools can simplify the process with visual cues.
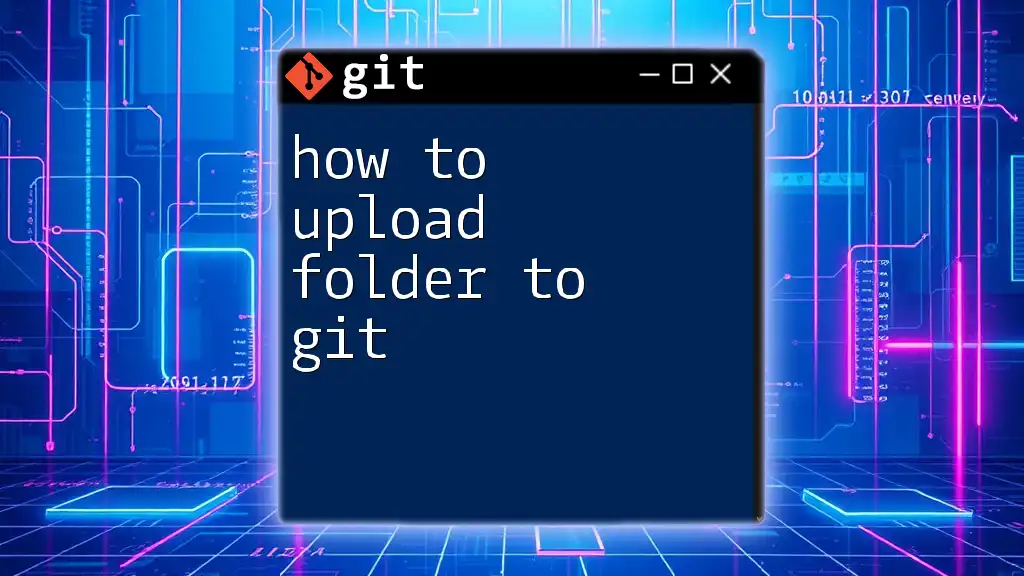
Creating a Folder in a Local Git Repository
Step-by-Step Instructions
Navigating to the Repository
First, you need to navigate to your Git repository using your terminal or command prompt. This is where you will create the new folder.
cd path/to/your/repository
Replace `path/to/your/repository` with the actual path to your Git repository.
Creating the Folder
To create a new folder, you can use the `mkdir` command. This command stands for "make directory."
mkdir new-folder-name
In this example, `new-folder-name` is the name you wish to give your new folder. This command creates the folder in your current directory.
Verifying Folder Creation
To ensure that your folder has been successfully created, list the contents of the directory using the following command:
ls
This command will display all files and folders in the current directory, allowing you to confirm the presence of `new-folder-name`.
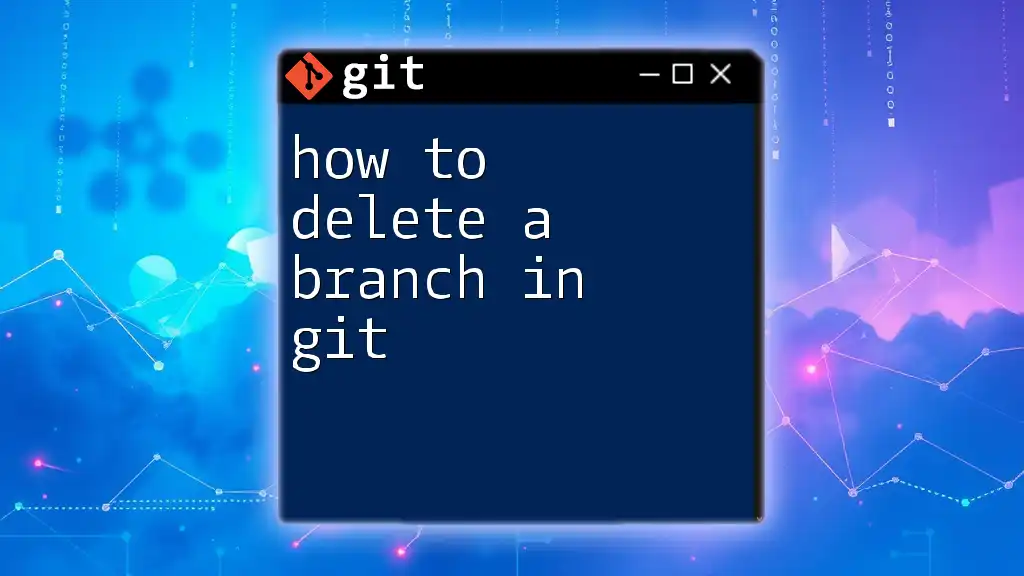
Adding the Folder to Git
Staging the New Folder
Once your folder is created, you need to stage it before you can commit the changes to your repository. Staging tells Git which changes you want to include in your next commit.
git add new-folder-name
Note that Git does not track empty folders; it only tracks the files within them. Therefore, staging an empty folder alone will not suffice.
Committing the Changes
After staging your new folder, it’s crucial to commit the change to your repository. Committing is a way to save your changes to the version history.
git commit -m "Added a new folder: new-folder-name"
The `-m` flag allows you to provide a concise message describing the changes made. This helps maintain clear project documentation.
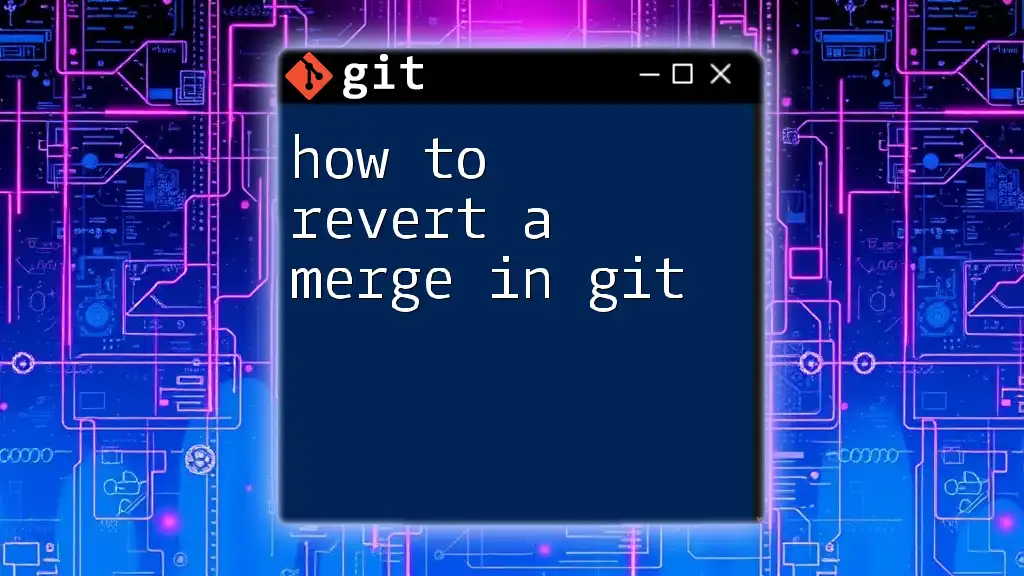
Creating Folders with Files
Adding Files Inside the New Folder
You often want to create new files inside the folder you've just created. You can do this with the `touch` command, which allows you to create a new empty file.
touch new-folder-name/example-file.txt
In this example, `example-file.txt` is the name of your new file that resides within the `new-folder-name`.
Staging and Committing the File
Just as you staged the folder, you'll want to stage this new file and then commit it.
git add new-folder-name/example-file.txt
git commit -m "Added example file in new-folder-name"
By committing the file, you ensure that both the folder and its contents are recorded in the version history, which is vital for tracking changes over time.
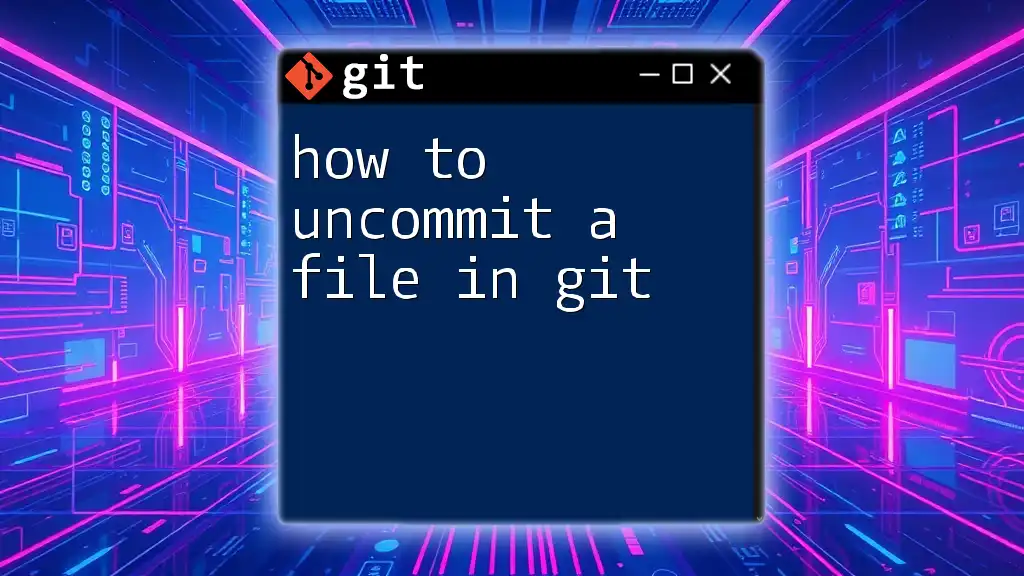
Best Practices for Organizing Folders in Git
Naming Conventions
When creating folders, it’s essential to follow naming conventions to maintain clarity and consistency. Good practices include using:
- Lowercase letters: This avoids confusion on case-sensitive systems.
- Dashes or underscores: They help separate words (e.g., `new-folder`, `new_folder`).
Avoid using spaces or special characters, which can lead to complications when referencing folder names in commands.
Structuring Projects
Consider various strategies for structuring your projects. Here are common ways to organize directories:
- By Feature: Group files together based on their functionality. For example, a `login` folder could contain all files related to user authentication.
- By Type: Separate files into types, such as `src`, `tests`, and `docs`.
- By Module: For more extensive projects, create modules that encapsulate specific parts of your application.
This clarity will enhance both individual workflow and collaborative efforts.
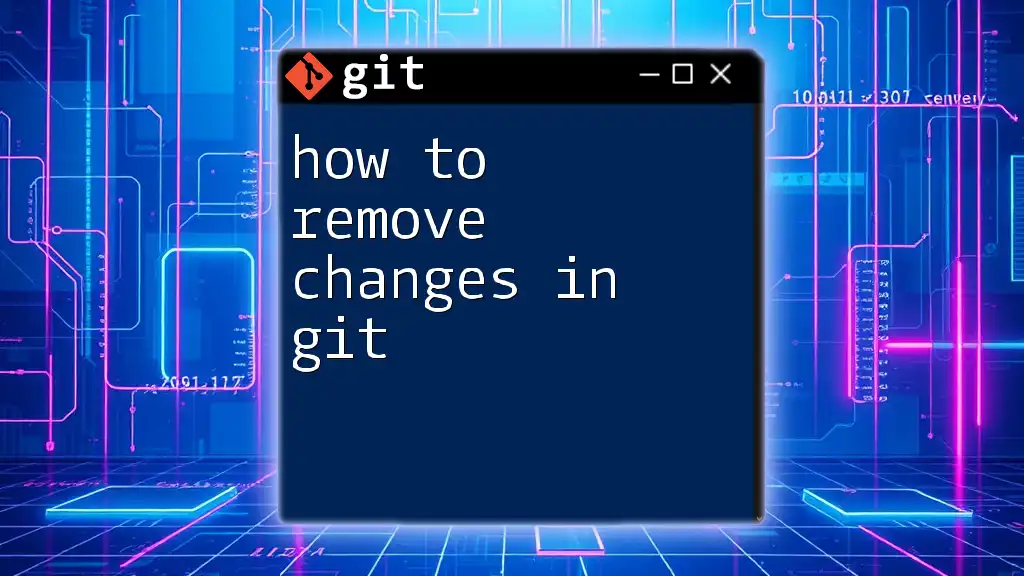
Troubleshooting Common Issues
Folder Not Showing Up in Git
If the folder you created is not showing up in Git, check the following:
- Staging: Ensure you have staged the folder with `git add new-folder-name`.
- Committing: Confirm that you have committed the change.
- Viewing Changes: Use `git status` to see if your folder is recognized by Git.
Dealing with Git Ignoring Folders
Be aware that if your `.gitignore` file has entries excluding specific folders, Git will not track them. If you find your new folder missing, check the `.gitignore` file and edit it accordingly:
/new-folder-name/
Removing or commenting out this line will allow Git to track changes to this folder.
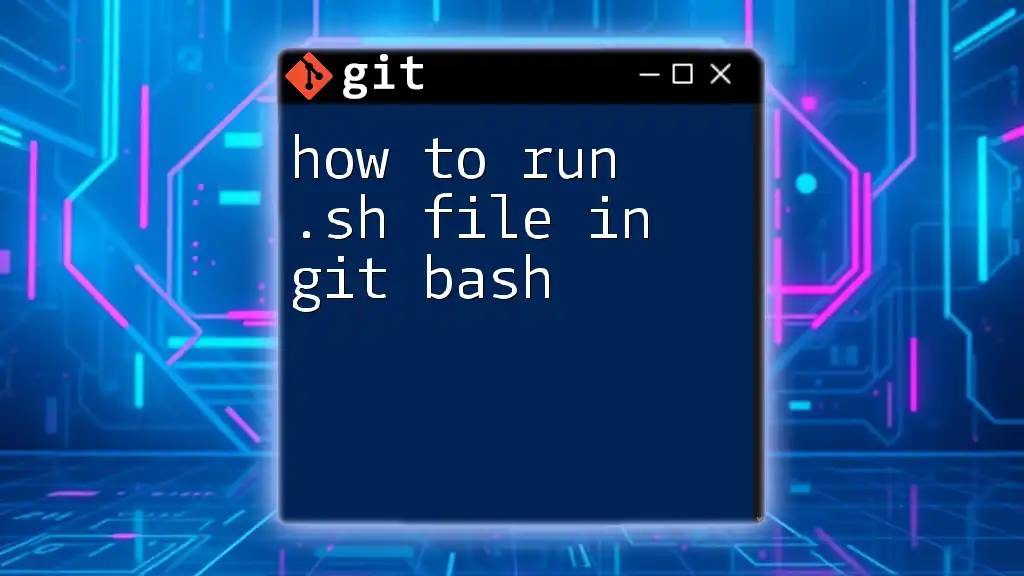
Conclusion
Creating a folder in Git is a straightforward process that significantly enhances project organization. By understanding the steps and best practices associated with folder creation, you can maintain a clean, efficient repository that benefits both individual developers and collaborative teams.
By implementing effective folder structures and adhering to naming conventions, you facilitate easier project navigation and foster better collaboration among team members. Remember to continually refine your Git skills, explore more advanced topics, and consider joining workshops or tutorials for a deeper dive into mastering Git commands.
Engage with the community, explore resources, and enhance your understanding as you embark on this exciting journey with Git!