To run a `.sh` file in Git Bash, simply navigate to the directory where the file is located and use the following command:
bash your_script.sh
Replace `your_script.sh` with the name of your shell script.
What is a .sh File?
A `.sh` file is a script written for the Bourne shell (or compatible shells) commonly used in Unix/Linux environments. These script files allow you to automate tasks by executing a series of commands line by line.
Common uses of `.sh` files include:
- Automation: Automate repetitive tasks such as backups or installations.
- Configuration: Set up environment variables and other settings for applications.
- Batch Processing: Execute multiple commands sequentially without user interaction.
While `.sh` files are popular, it’s essential to remember they are not the only script files available. For instance, Python scripts have the `.py` extension, and PowerShell scripts use `.ps1`.
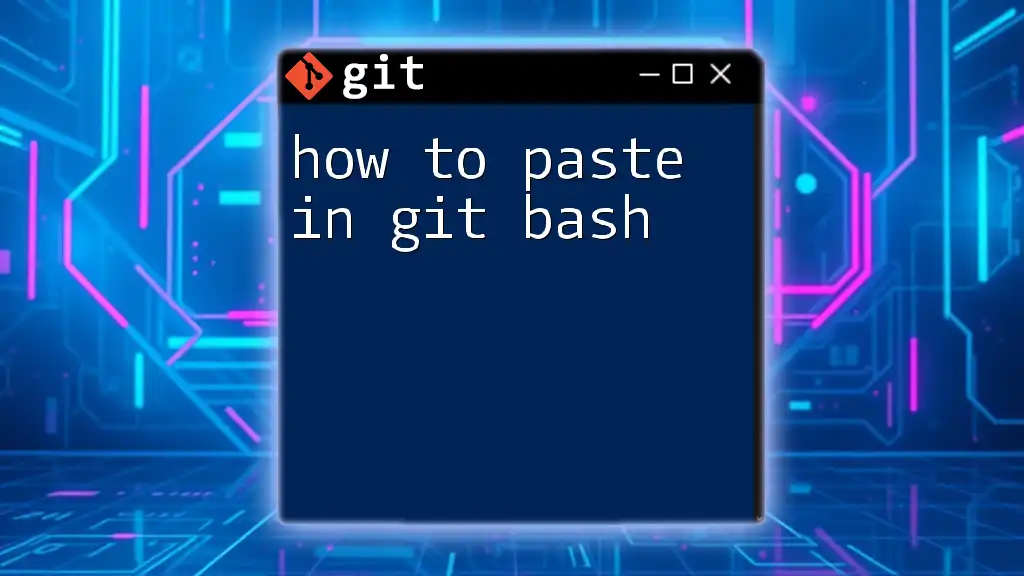
Setting Up Git Bash
Installation of Git Bash
If you haven’t yet installed Git Bash, follow these steps:
- For Windows: Download the installer from the official [Git website](https://git-scm.com/downloads) and follow on-screen instructions.
- For macOS: You may use Homebrew by running `brew install git`. Alternatively, download from the Git website.
- For Linux: Most distributions include Git. Use your package manager, e.g., `sudo apt install git` for Ubuntu.
Opening Git Bash
Once installed, open Git Bash from your application menu or by searching for it in the operating system’s search feature.
Confirming Installation
To ensure that Git Bash is installed correctly, run the following command in the terminal:
git --version
This command should output the installed version of Git, indicating that Git Bash is functioning properly.
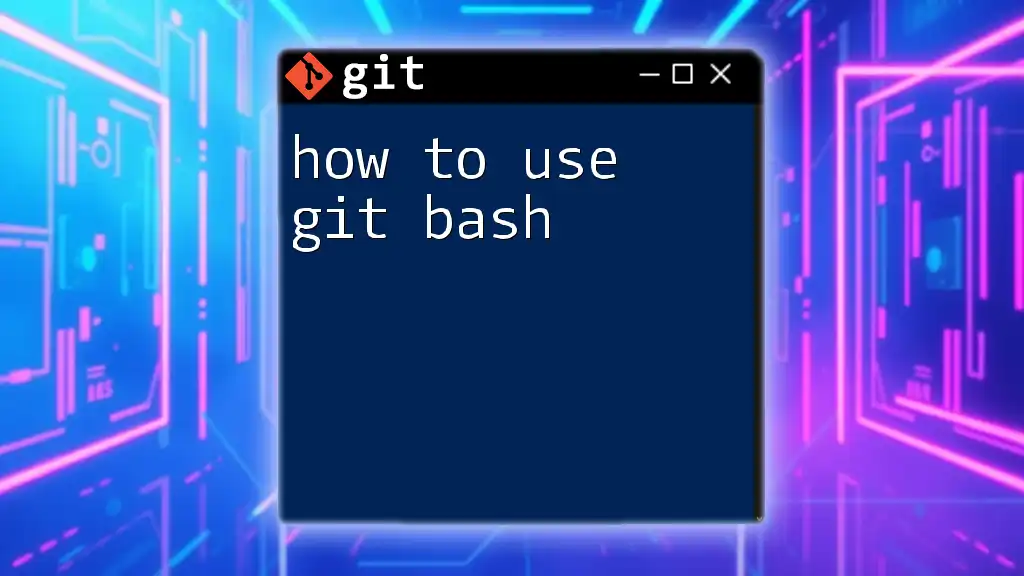
Understanding Basic Git Bash Commands
Navigating Directories
Understanding how to navigate through directories is crucial for working with `.sh` files. Here are the three primary commands:
- `cd`: Stands for “change directory.” Use this command to navigate between folders.
- `ls`: Lists files and directories in the current directory. This command helps you verify the existence of your `.sh` file.
- `pwd`: Displays the working directory, showing your current location in the file system.
Example Usage:
cd /path/to/your/directory
ls
pwd
Creating and Editing .sh Files
You can create and edit `.sh` files using text editors like `nano` or `vim`.
To create a new script file called `myscript.sh`, run:
nano myscript.sh
Inside the editor, you can write your shell script. For example, you might start with:
#!/bin/bash
echo "Hello, World!"
Once finished, save the file (in `nano`, use `CTRL + O` and then `CTRL + X` to exit).
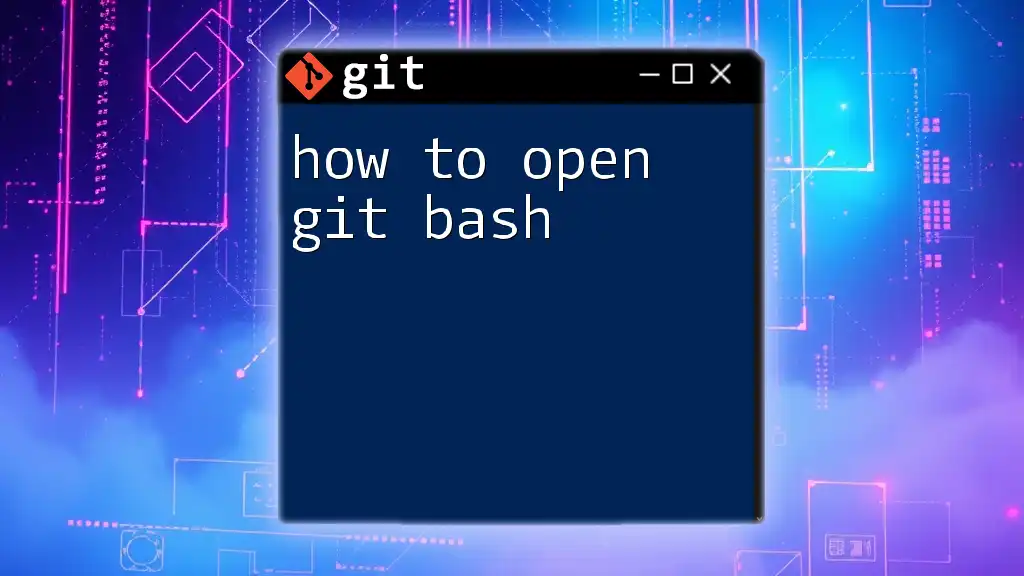
Preparing to Run Your .sh File
Setting Executable Permissions
For a `.sh` file to run, it needs executable permissions. This is set using the `chmod` command.
To grant executable permissions, use the following command:
chmod +x myscript.sh
This command effectively tells the system, "Allow this script to be run as an executable file."
Changing Directory to .sh File Location
Before executing your script, navigate to the directory where it resides using the `cd` command. Keeping your workspace organized is essential because running scripts from the wrong directory can lead to confusion and errors.
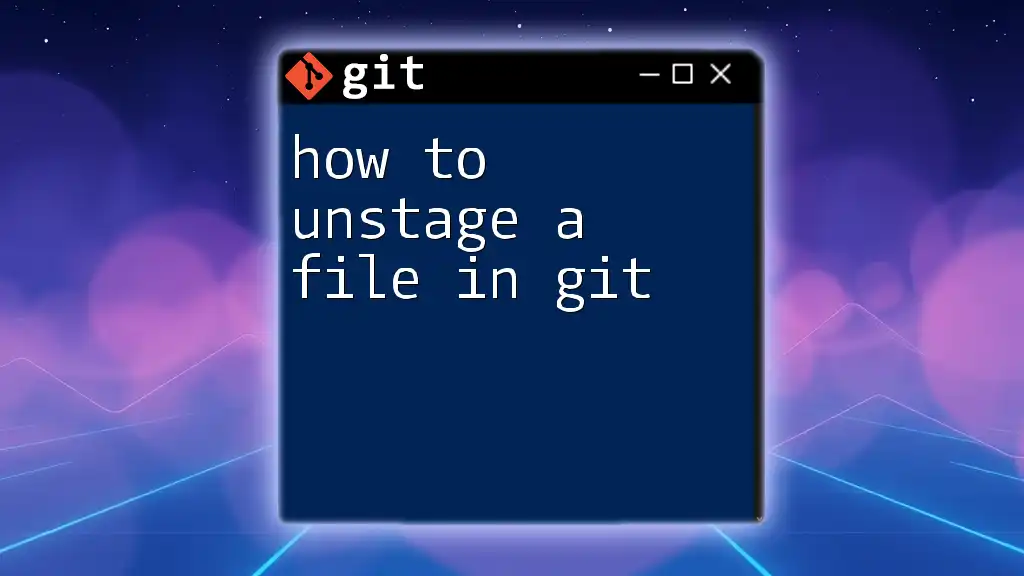
Running the .sh File in Git Bash
Executing the .sh File
Once you have set the necessary permissions and navigated to the correct directory, you are ready to run your `.sh` file. Execute it by prefixing the script name with `./` to indicate that it is located in the current directory:
./myscript.sh
Upon successful execution, you should see the output of your script. If it includes the line `echo "Hello, World!"`, you will see Hello, World! printed in the terminal.
Understanding Errors
When running scripts, you might encounter some common errors, such as:
- Command not found: Ensure you are in the correct directory and the file name matches precisely.
- Permission denied: This indicates that the file lacks executable permissions. Revisit your permission settings with `chmod +x`.
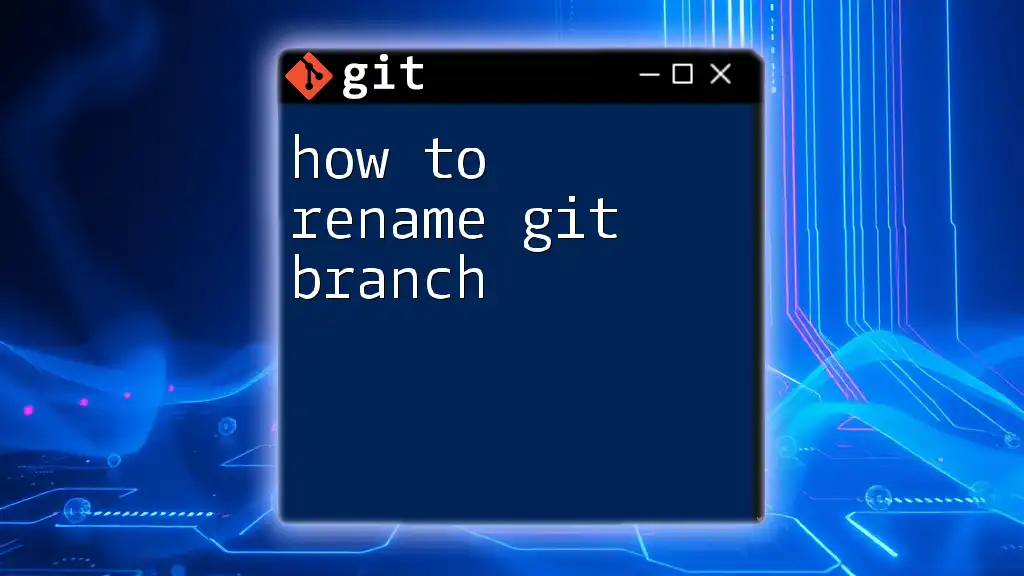
Advanced: Running .sh Files with Arguments
What are Arguments?
Arguments are inputs given to the script upon execution. They allow you to pass values or flags to modify the script's behavior.
Example of Running a Script with Arguments
To run `myscript.sh` with arguments, you might execute:
./myscript.sh arg1 arg2
In the above example, `arg1` and `arg2` are placeholders for any values you want the script to process.
Accessing Arguments within the Script
Within the script, you can access the passed arguments as follows:
- `$1` represents the first argument,
- `$2` represents the second argument, and so on.
For example, inside `myscript.sh`, you might have:
echo "The first argument is $1"
If you run `./myscript.sh Hello`, the output will be The first argument is Hello.
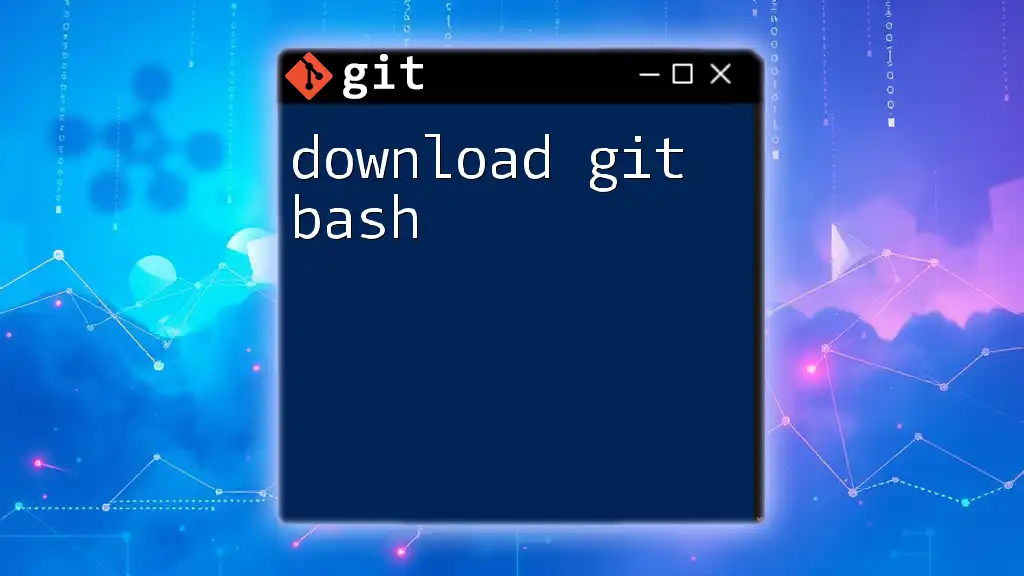
Best Practices for Writing .sh Files
Using Shebangs
At the top of your script, include a shebang line, which indicates what interpreter to use. For Bash scripts, use:
#!/bin/bash
This line should be the very first line in your script.
Commenting Your Code
Comments can greatly enhance the readability of your scripts. Use the `#` symbol to comment on your code. For instance:
# This script prints Hello, World!
echo "Hello, World!"
Organizing Script Structure
Maintain a clean and readable format by:
- Breaking your script into sections with meaningful comments.
- Using indentation and spacing for clarity.
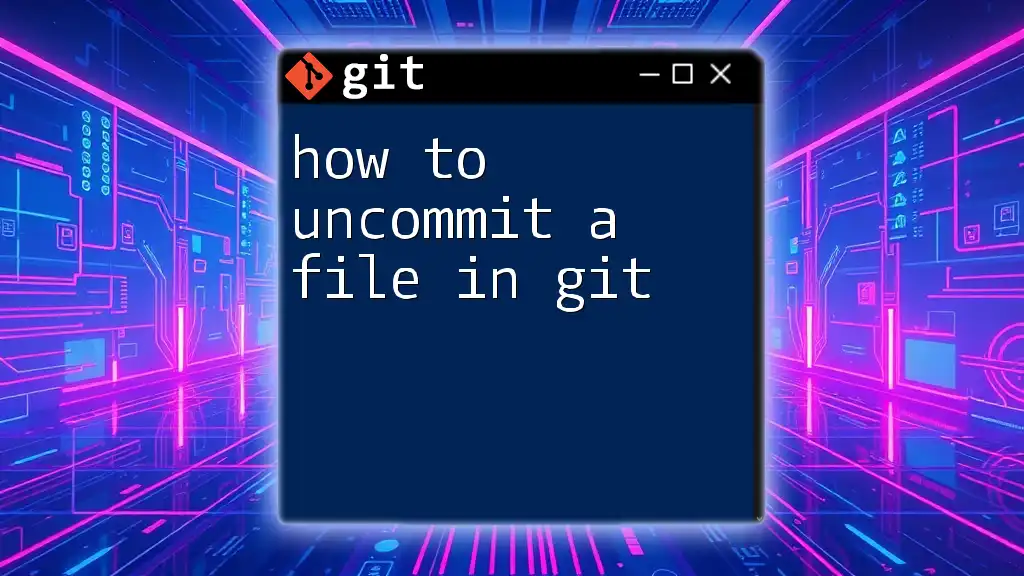
Troubleshooting Common Issues
File Not Found Errors
If Git Bash reports that it cannot find the specified script, ensure you have navigated to the appropriate directory and that the filename was entered correctly, considering capitalization.
Permission Denied Errors
Revisit your permission settings with the `chmod +x yourscript.sh` command to ensure your script is executable. If problems persist, check the directory permissions.
Syntax Errors in Scripts
If you encounter syntax errors, carefully read through your script. Bash is sensitive to minor mistakes, such as missing quotes or incorrect command syntax. Utilize debugging techniques, like adding `set -x` at the top of your script, which prints commands as they are executed.
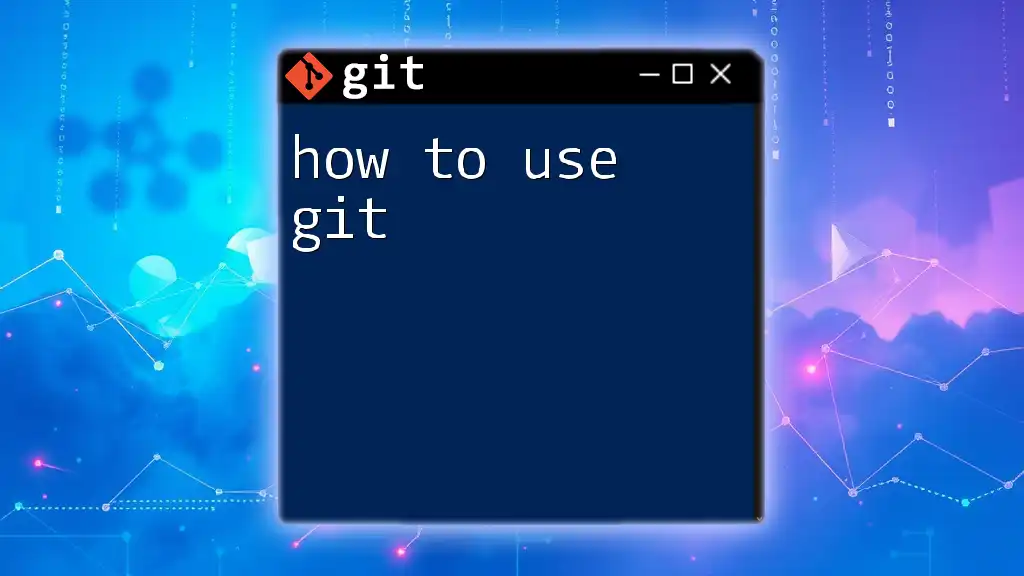
Conclusion
By following the steps outlined in this guide, you now have a solid understanding of how to run .sh files in Git Bash. Whether you’re automating tasks or configuring environments, proficiency with shell scripts enhances your programming capabilities.
Embrace the power of scripting, and don't hesitate to explore further Git commands and shell scripting enhancements to elevate your skills!
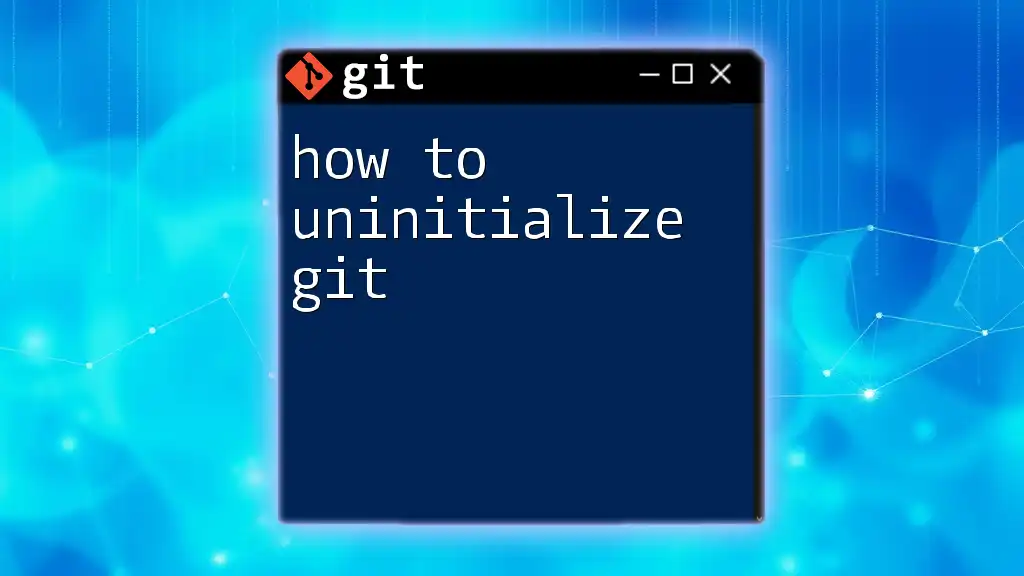
Additional Resources
- For in-depth details on Git functionalities, visit the official [Git documentation](https://git-scm.com/doc).
- Consider checking out interactive tutorials that delve into more advanced shell scripting techniques.
- Engage with community forums for additional insights and troubleshooting advice on Git and shell scripting.