To use Git on Windows, first install Git for Windows, then open Git Bash, and you can start with basic commands like initializing a repository or checking the status. Here's a simple code snippet to initialize a new Git repository:
git init
Setting Up Git on Windows
Downloading Git
To begin learning how to use Git on Windows, you'll first need to download it. Head over to the official Git website at [git-scm.com/download/win](https://git-scm.com/download/win) to find the latest installer for Windows. This is a straightforward first step that ensures you have the right software to manage your version control tasks effectively.
Installing Git
After downloading the installer, double-click the file to launch the installation process. You will be guided through a series of steps. Pay attention to the following important options:
-
Default Editor: During installation, you'll be prompted to select a default text editor. Git will use this editor for commit messages. You might choose well-known options like *Notepad++, VS Code, or the default Vim.
-
Adjusting the PATH Environment: You will also see an option to adjust the PATH environment. Select the option that allows you to use Git from the command line and other tools.
Verifying Installation
Once Git is installed, you should verify that the installation was successful. Open your command prompt and type:
git --version
If the installation was successful, you'll see the version of Git that you installed. This confirms that Git is ready to be used on your Windows machine.
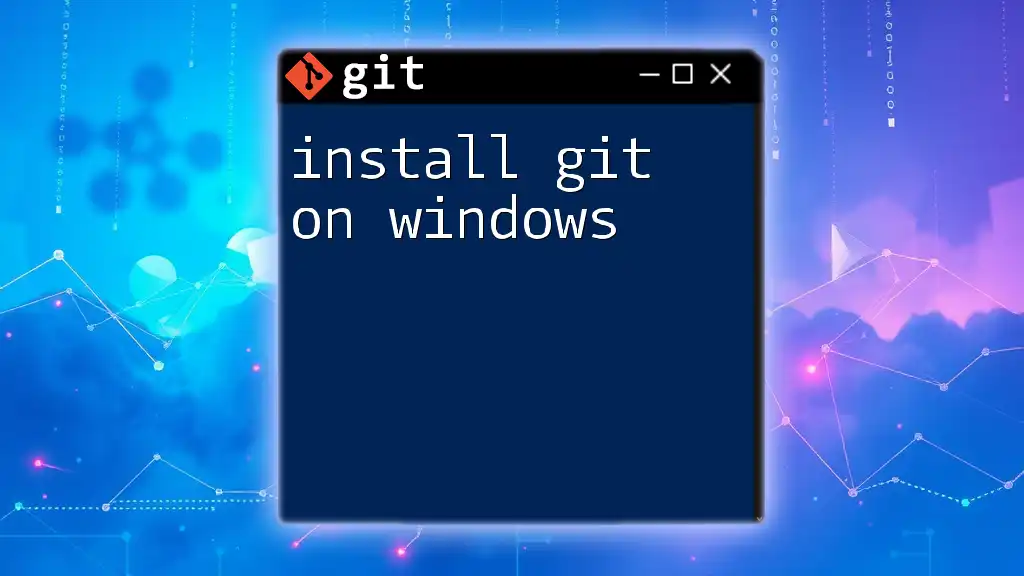
Configuring Git
Setting Up Global Configurations
After verifying the installation, it's crucial to configure Git with your personal information. This information will be associated with your commits, making it easier to track contributions. Use the following commands to set up your global configurations:
git config --global user.name "Your Name"
git config --global user.email "your_email@example.com"
Replace `"Your Name"` and `"your_email@example.com"` with your actual name and email address. This step ensures that your identity is clear in the project history.
Checking Current Configuration
To view the settings you've just configured, you can use the command:
git config --list
This command will display all of your current Git configurations, allowing you to confirm that your information is correctly set up.
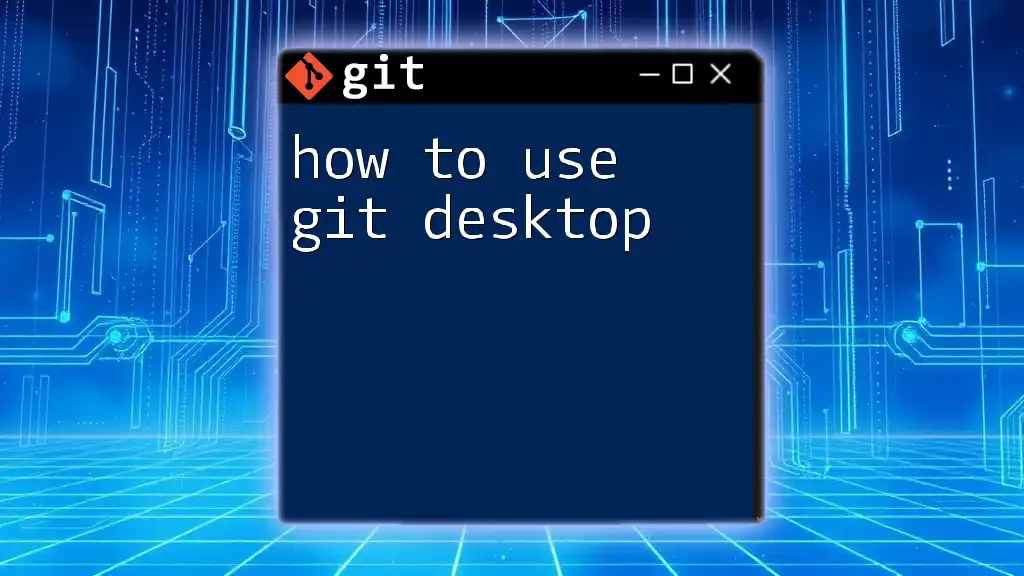
Basic Git Commands on Windows
Initializing a Repository
One of the first tasks you'll perform when learning how to use Git on Windows is to initialize a new repository. To do this, create a new directory for your project and navigate into it:
mkdir project-name
cd project-name
git init
The command `git init` creates a new Git repository in your project's directory. This step transforms the ordinary directory into one that Git can track changes within.
Cloning a Repository
If you want to get started with an existing project, you can clone a repository instead. Use the following command:
git clone https://github.com/username/repository.git
Replace the URL with the actual link to the repository you wish to clone. Cloning allows you to create a local copy of the project on your machine, enabling you to contribute to it.
Tracking Changes
Staging Changes
After making modifications to files, you'll want to stage these changes for committing. Use:
git add filename
To stage a specific file, replace `filename` with the actual file name. To stage all changes in your directory at once, use:
git add .
This command adds every modified file to the staging area, ready to be committed.
Committing Changes
Once you've staged your changes, you should commit them with a descriptive message. This is crucial for tracking the history of your project:
git commit -m "Your commit message"
Importance of Meaningful Commit Messages
Always write clear and descriptive commit messages. For example, instead of writing "fixed stuff", you could articulate "Fixed bug in user login module". This practice helps you and your collaborators understand the history and context of the project more clearly.
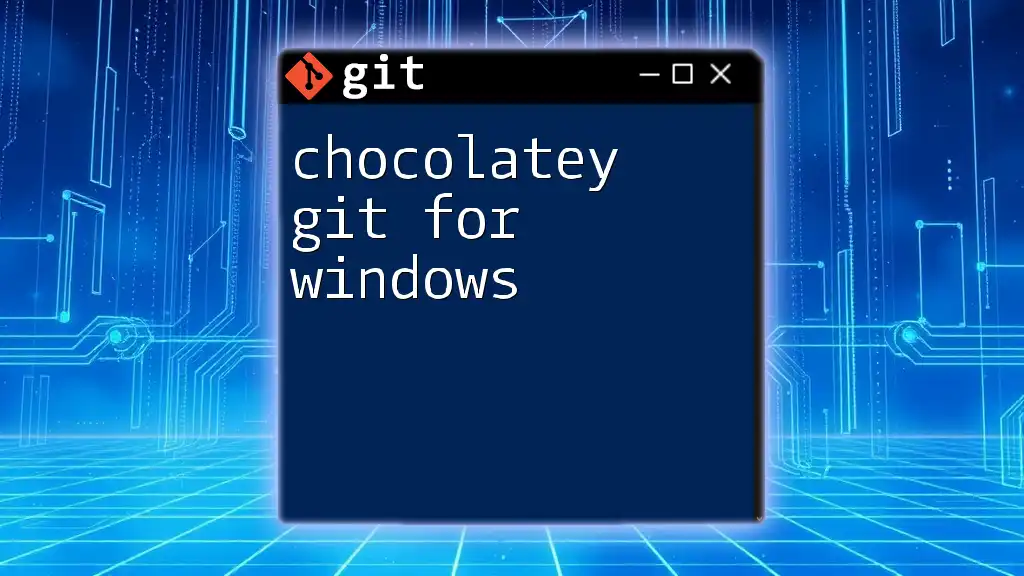
Branching and Merging
Understanding Branches
Branches in Git allow you to develop features or fix bugs in isolation from the main codebase. This is especially useful when working in teams, as multiple people can work on different branches without interfering with each other's work.
Creating a Branch
To create a new branch, use the command:
git branch new-branch-name
Replace `new-branch-name` with an appropriate name for your branch. This separates your work from the main branch, allowing for safe experimentation.
Switching Between Branches
When you want to switch to the newly created branch or any other branch, use:
git checkout new-branch-name
This command moves you to the specified branch, allowing you to begin working there.
Merging Branches
Once you've completed your changes on a branch, you will likely want to merge these changes back into the main branch. First, switch to the main branch:
git checkout main
Then run the merge command:
git merge new-branch-name
This merges the changes from `new-branch-name` into the `main` branch. Keep an eye out for merge conflicts, which can occur if changes were made to the same lines of a file on both branches.
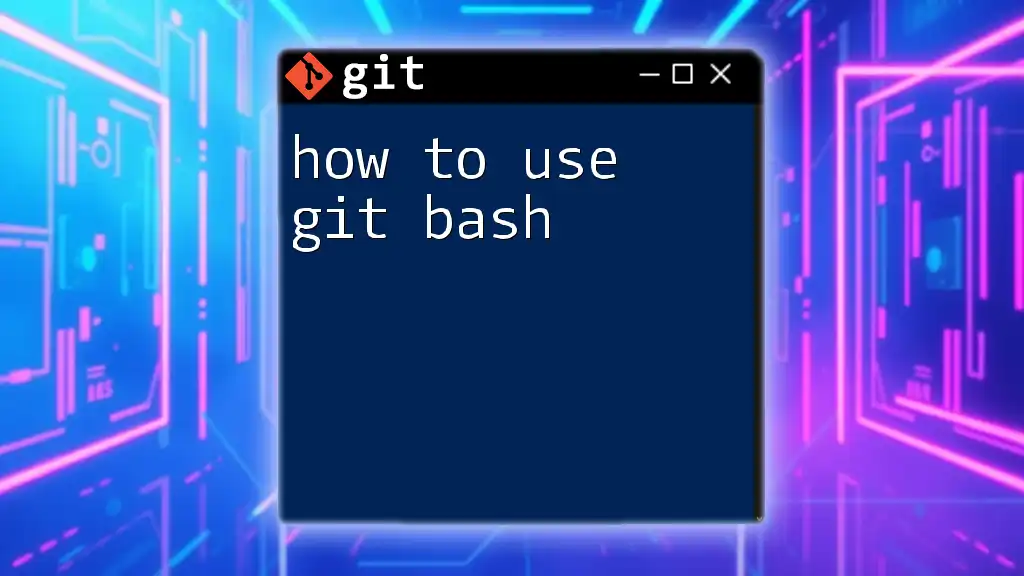
Working with Remotes
Understanding Remote Repositories
Remote repositories allow multiple collaborators to contribute to a project from different locations. This makes it possible to share your work easily with a team.
Adding a Remote
To associate your local repository with a remote repository on a platform like GitHub, run:
git remote add origin https://github.com/username/repository.git
This command creates an alias called `origin`, which points to the specified URL.
Pushing Changes
When you're ready to share your local commits, push them to the remote repository:
git push origin main
If you're on a different branch, substitute `main` with your current branch name. This action updates the remote repository to reflect your changes.
Pulling Changes
To keep your local copy up to date with changes made by others, use:
git pull origin main
This command fetches and merges changes from the remote repository into your local branch.
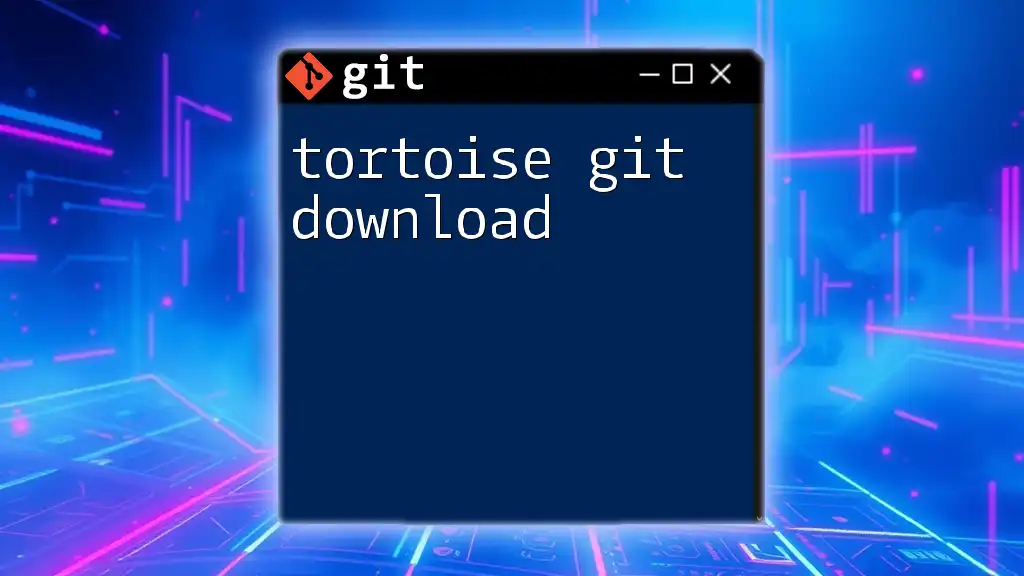
Handling Merge Conflicts
What is a Merge Conflict?
A merge conflict occurs when different changes are made to the same line of a file in two branches. Git needs your help to resolve these conflicts.
How to Resolve Merge Conflicts
When a conflict arises, Git will mark the conflicted files. You must:
- Open the conflicting files in your text editor.
- Locate the sections marked by `<<<<<<<`, `=======`, and `>>>>>>>`.
- Determine which changes to keep, edit the content as necessary, and remove the conflict markers.
Committing Resolved Conflicts
After resolving the conflicts, stage the resolved files:
git add resolved-file
Then commit your changes:
git commit -m "Resolved merge conflict"
This finalizes the merge after all conflicts have been addressed.
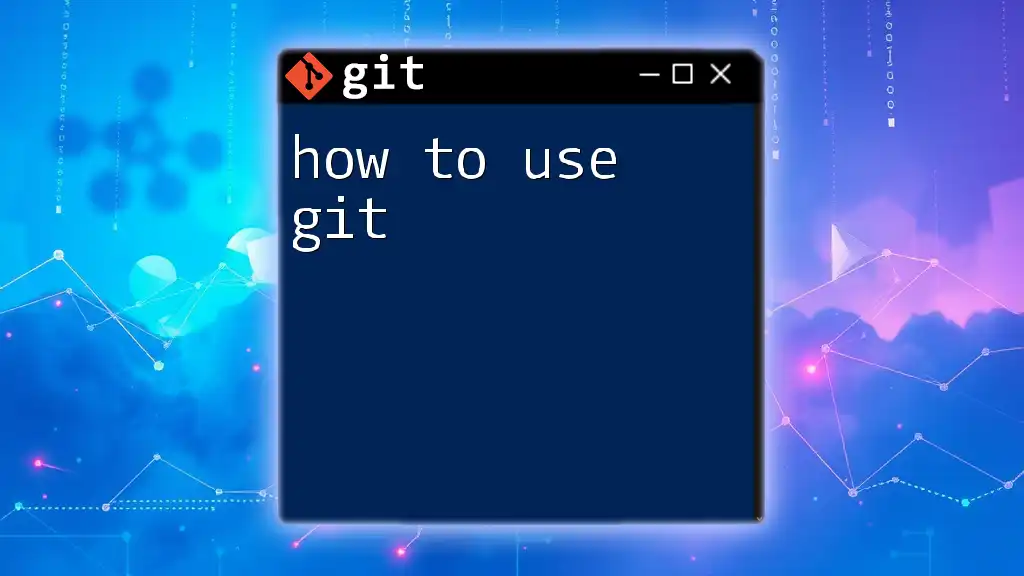
Conclusion
Understanding how to use Git on Windows equips you with essential tools for project management and collaboration. From setting up your Git environment to pushing and pulling changes, mastering these commands enhances your ability to manage code effectively.
As you grow more comfortable with Git, incorporate best practices like meaningful commit messages and conflict resolution strategies. Such practices will benefit both you and your future collaborators, paving the way for successful team projects.
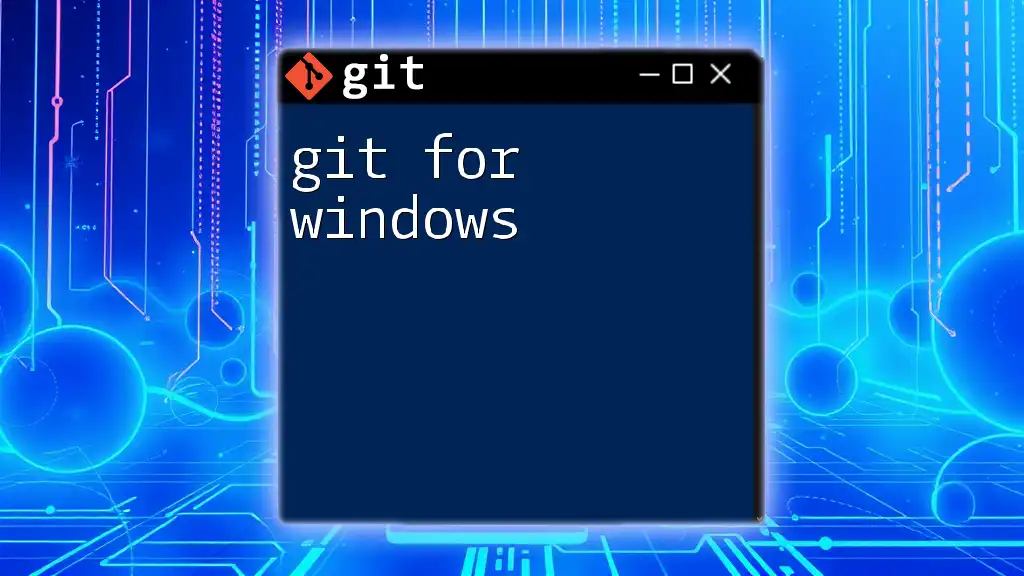
Resources & Further Reading
For additional insights and tutorials, consult the official Git documentation and various online courses. There are also many excellent Git GUI clients available that can make using Git even more user-friendly on Windows.
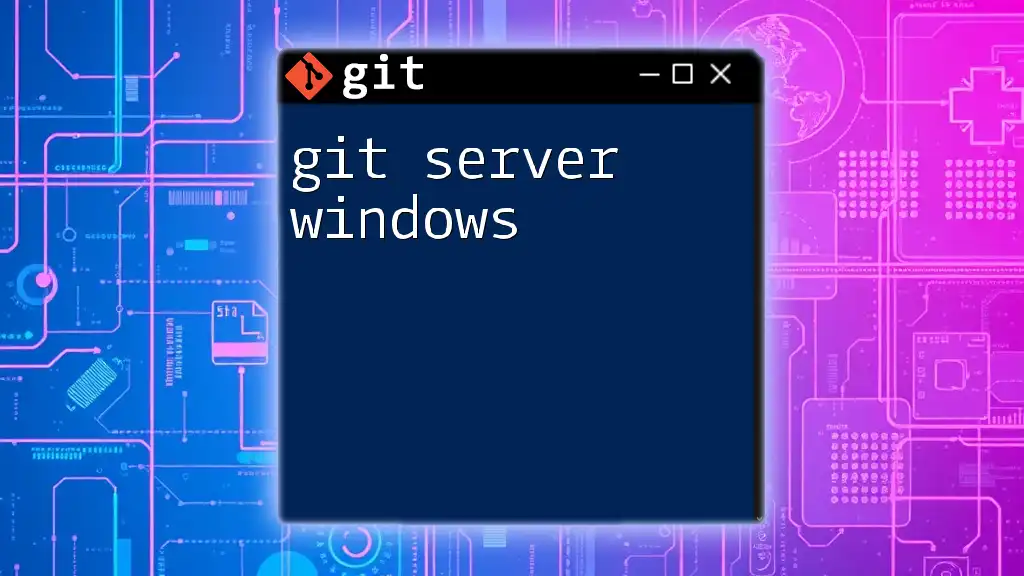
Call to Action
If you found this guide helpful, consider subscribing for more tutorials on Git and version control. My hope is to empower you with the skills needed to excel in collaborative coding environments. Share your experiences and questions in the comments section; your feedback is invaluable!