Git Bash is a command-line interface that allows users to interact with Git repositories using Unix-like commands on Windows.
git clone https://github.com/username/repository.git
What is Git Bash?
Definition
Git Bash is a command line interface that provides a powerful way to interact with Git, a version control system commonly used in software development. It combines the functionality of a Bash shell with Git, allowing users to execute a wide range of Git commands directly from the command line.
Key Features
Git Bash offers several advantages, including:
- Bash command line interface: This familiar environment allows users to run Linux commands and scripts on Windows, making it easier for developers who are accustomed to working in UNIX-like systems.
- Integration with Git: Git Bash seamlessly integrates with Git, enabling users to manage their code repositories efficiently.
- Cross-platform compatibility: While primarily used on Windows, Git Bash can be run on any platform that supports Git, providing a versatile tool for developers working in various environments.
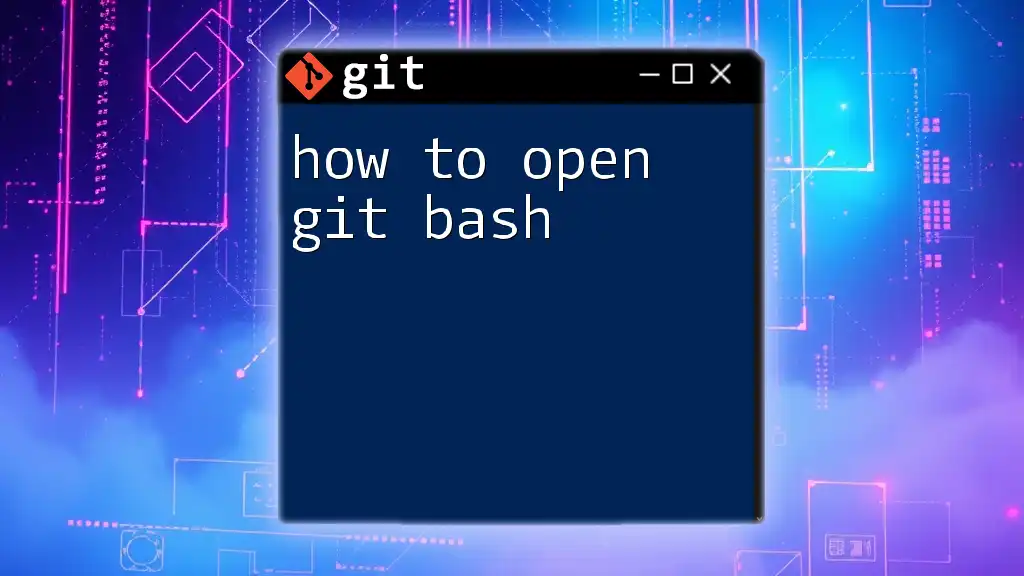
Setting Up Git Bash
Installing Git Bash
Setting up Git Bash is straightforward, and it can be installed on various operating systems.
To install Git Bash on Windows:
- Download the installer from the official Git website.
- Run the installation wizard and follow the prompts. It's recommended to accept the default settings.
- Once installed, you can launch Git Bash from the Start menu.
To install on macOS or Linux, the command can be run through the terminal using package managers like Homebrew or the system's package manager.
Initial Configuration
After installing Git Bash, it's essential to configure your user identity:
- Start by setting your user name and email:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
- You can verify that your configuration is correct by running:
git config --list
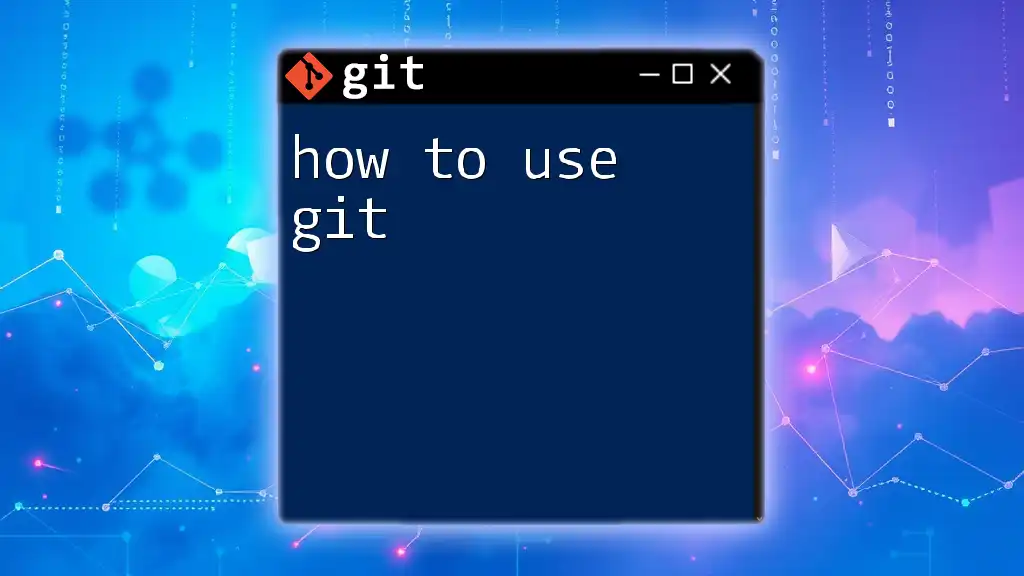
Navigating Git Bash
Basic Command Line Navigation
Navigating through directories and files is crucial when using Git Bash.
- Use the following commands:
- `pwd` to print the working directory, showing the current path.
- `ls` to list directory contents.
- `cd` to change directories to navigate where necessary.
For instance, to navigate to a specific directory, you can use:
cd /path/to/directory
Creating and Managing Directories
You can quickly create new directories and manage existing ones with these commands:
To create a new directory:
mkdir new-project
To remove a directory:
rmdir old-project
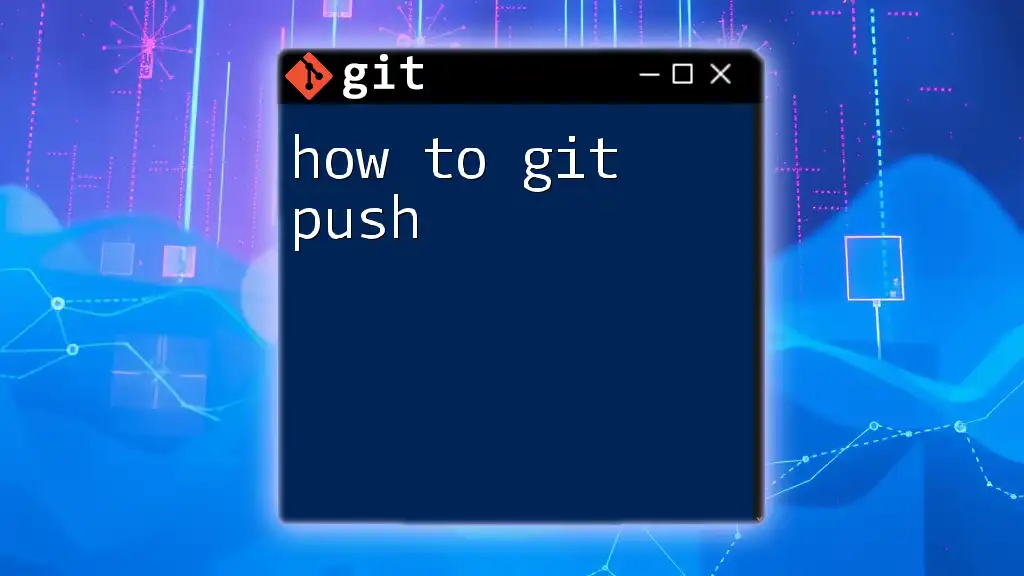
Basic Git Commands
Initializing a Git Repository
Starting a new project in Git Bash begins with creating a Git repository. You can initialize a repository by using:
git init project-name
This command sets up a new Git repository in a folder named `project-name`.
Adding Files
Once your repository is set up, you need to stage your files before committing them. Use `git add` to stage changes.
For example, to stage a single file:
git add filename.txt
To stage all changes in the current directory:
git add .
Committing Changes
After staging your files, it’s time to commit those changes. A commit is a snapshot of your project at a particular time. Execute:
git commit -m "Initial commit"
Make sure to use a meaningful commit message that describes the changes made.
Viewing Changes
To check the status of your repository, use:
git status
This command shows which files are staged, unstaged, or untracked. If you want to see the exact differences made to files between the last commit and your current changes, use:
git diff
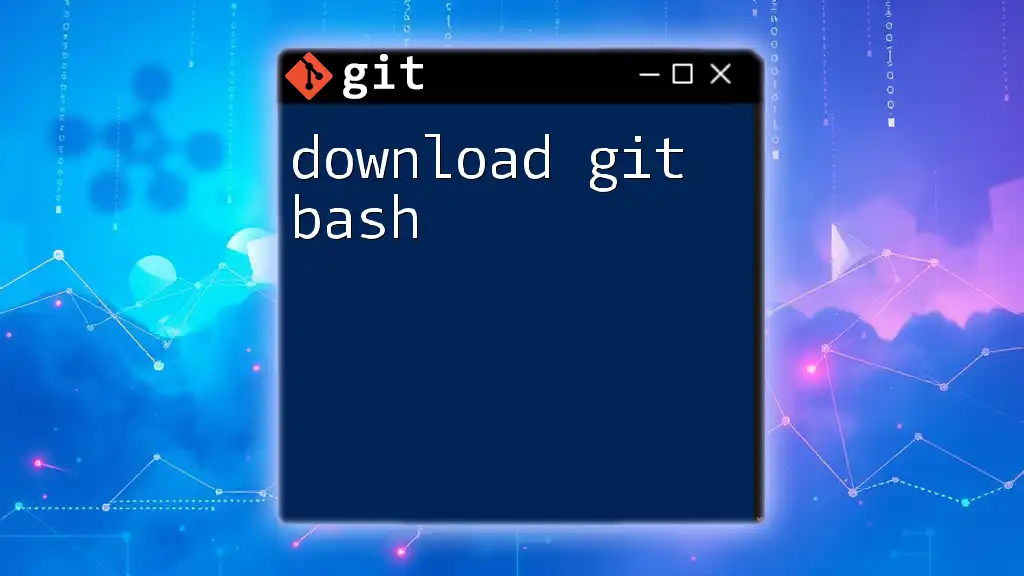
Working with Branches
Creating and Switching Branches
Branches are essential for managing different lines of development. To create a new branch and switch to it:
git branch new-feature
git checkout new-feature
Alternatively, you can combine the commands:
git checkout -b new-feature
Merging Branches
Once you are ready to merge branches, switch to your main branch and execute:
git merge new-feature
When merging, if there are conflicting changes, Git will highlight those, allowing you to resolve them manually.
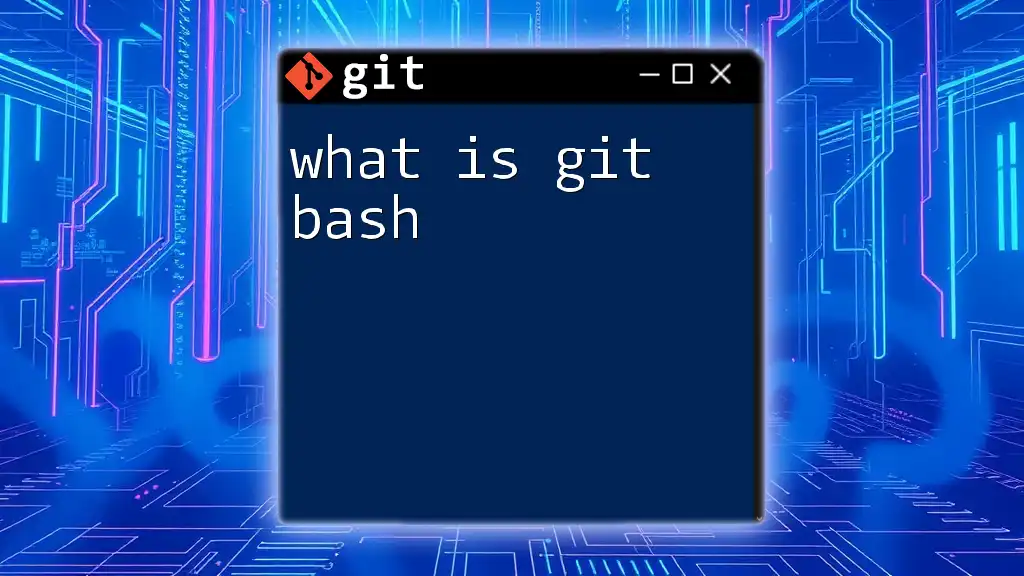
Remote Repositories
Adding Remote Repositories
To collaborate on projects, you often need to work with remote repositories. You can add a remote repository with:
git remote add origin https://github.com/user/repo.git
This command links your local repository to the specified remote repository.
Pushing and Pulling Changes
To send your local commits to the remote repository, use:
git push origin main
Conversely, to update your local repository with changes from the remote repository, run:
git pull origin main
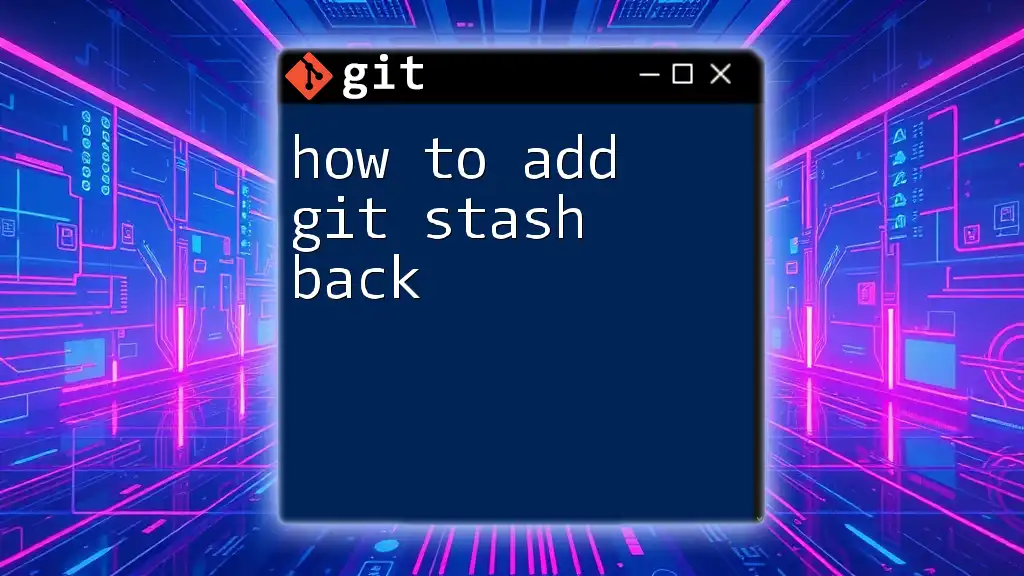
Troubleshooting Common Git Bash Issues
Common Errors and Fixes
While using Git Bash, you might encounter various error messages. Common issues include merge conflicts or errors related to uncommitted changes. When faced with a merge conflict, Git will indicate the conflicting files, allowing you to manually edit them and then commit the resolved changes.
Best Practices
To maintain an efficient workflow:
- Keep Git Bash updated to leverage the latest features.
- Commit changes regularly to avoid losing progress and to keep a clear project history.
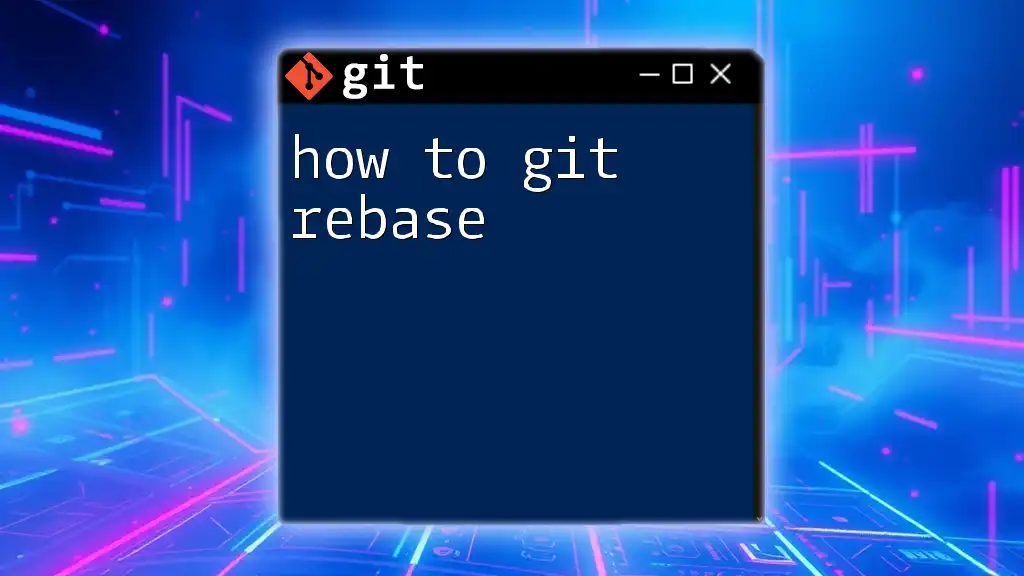
Conclusion
Mastering how to use Git Bash equips you with essential skills for efficient version control. As you practice these commands and develop your workflow, you'll find that using Git Bash enhances your productivity as a developer. Remember to explore additional resources for further learning and engagement with your Git skills. Happy coding!
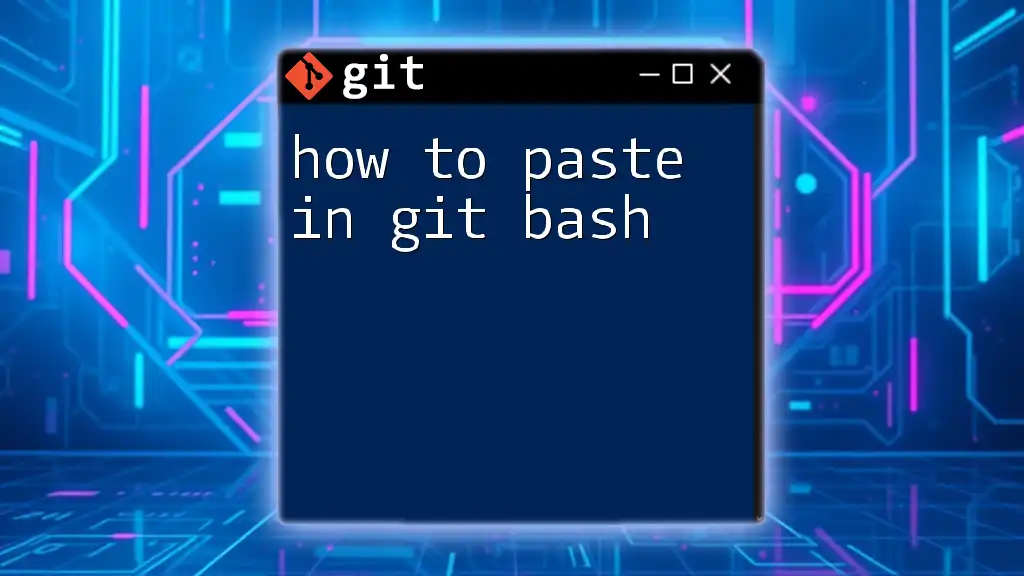
Additional Resources
For more in-depth understanding and practical exercises on Git and Git Bash, consider delving into tutorials, online courses, and official documentation.