To temporarily store your uncommitted changes in Git without losing them, you can use the `git stash` command. Here's how you can do it:
git stash
What is Git Stash?
Git stash is a powerful feature in Git that allows you to temporarily save changes in your working directory without committing them. This is particularly useful when you want to switch contexts, such as changing branches or pulling updates from a remote repository, but you’re not quite ready to commit your changes. By stashing your changes, you can quickly restore your work later without cluttering your commit history.
Scenarios for Using Git Stash
There are several scenarios in which using git stash proves beneficial:
-
Switching Branches: If you’re in the middle of a feature and need to switch to another branch to address an urgent issue, stashing allows you to save your current work without committing.
-
Pulling Updates: When collaborating with others, you might need to pull the latest changes from the main branch. If you have local changes that are not ready to be committed, stashing them can prevent conflicts.
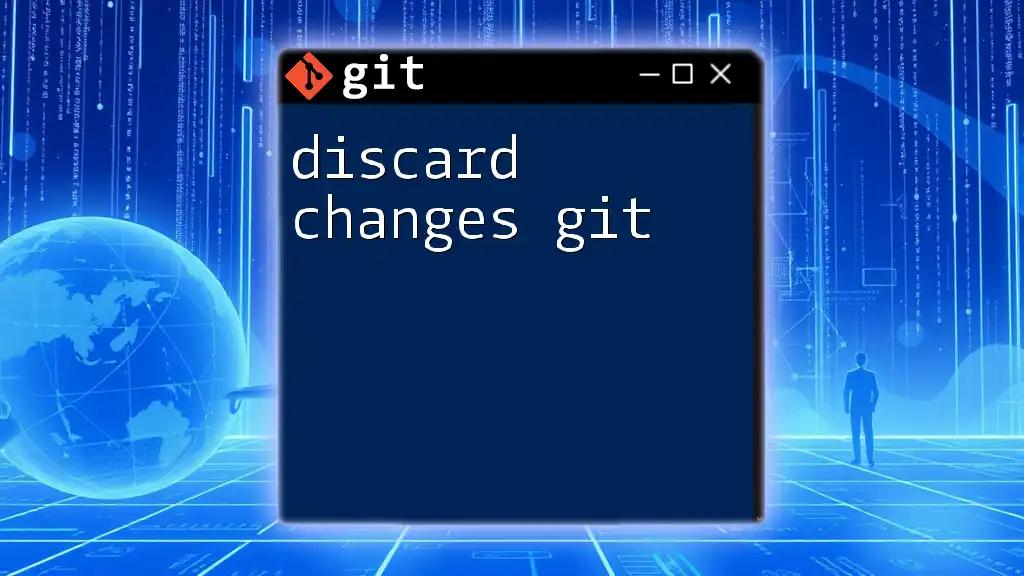
How to Stash Changes in Git
The Basic Stash Command
The most straightforward way to stash your changes is to use the command:
git stash
Running this command will save your current changes and revert your working directory to the state of the last commit. This means that you return to a clean working directory, ready for whatever action you need to take next. However, remember that any untracked files will not be stashed unless you specify otherwise.
Stashing Untracked Files
When you want to stash only the changes to tracked files and leave untracked files, you would use:
git stash push
In this case, Git saves only the changes made to files already being tracked. If you’ve also made changes to new files that you haven’t staged yet, those will remain in your working directory.
Including Untracked Files
To include untracked files in your stash, you can use the `-u` option:
git stash push -u
This command tells Git to stash both tracked modifications and any untracked files, effectively capturing all your work.
Including Ignored Files
If you need to stash everything, including files that are ignored in your `.gitignore` file, you can use:
git stash push -a
The `-a` or `--all` option allows you to stash all types of files, giving you the complete flexibility to manage your workspace as you see fit.

Viewing Stashed Changes
Listing Stashes
To see a list of all stashed changes, you can run:
git stash list
This command displays a summary of all stashed items, including their index and a brief message. The list helps you identify which stash you might want to restore or drop later.
Viewing Stashed Changes
If you want to check the details of your most recent stash, use:
git stash show
This command provides a summary of what changes are included in the stash. For a more detailed view of these changes, you can add the `-p` flag:
git stash show -p
Here, `-p` (or `--patch`) will show the full diff of the stashed changes, allowing you to review exactly what you saved.
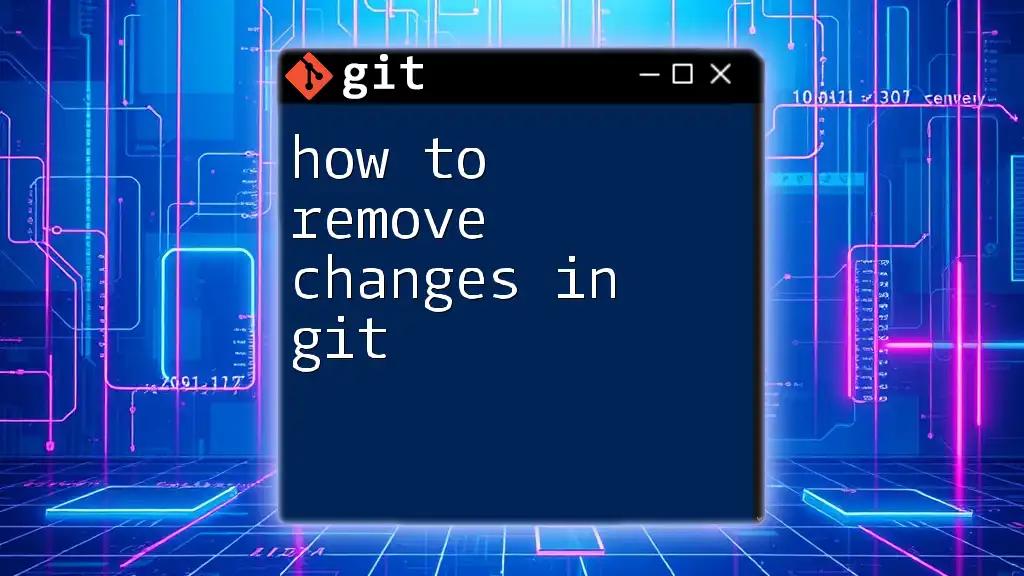
Applying Stashed Changes
Applying the Most Recent Stash
To apply the changes from the most recent stash, simply use:
git stash apply
This command restores the files that were stashed and keeps the stash entry in your stash list. Use it when you’re not ready to remove the stash yet.
Applying a Specific Stash
If you need to apply a specific stash, you can refer to it by its index:
git stash apply stash@{1}
This flexibility is crucial when managing multiple stashes, allowing you to retrieve exactly what you need.
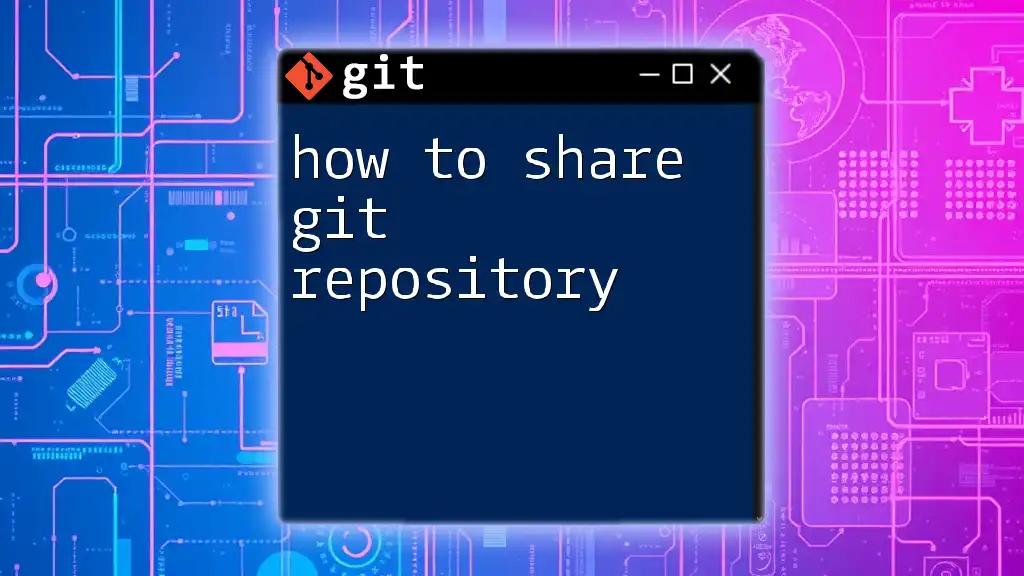
Removing Stashed Changes
Dropping a Stash
If you want to permanently remove a specific stash from the list, you can use:
git stash drop stash@{0}
This command deletes the specified stash, freeing up space and reducing clutter in your stash list.
Clearing All Stashes
To remove all stashes at once, you can run:
git stash clear
Be cautious with this command; it will delete all your stashed changes irreversibly. It’s important to ensure that you don’t need any of those stashes before executing it.
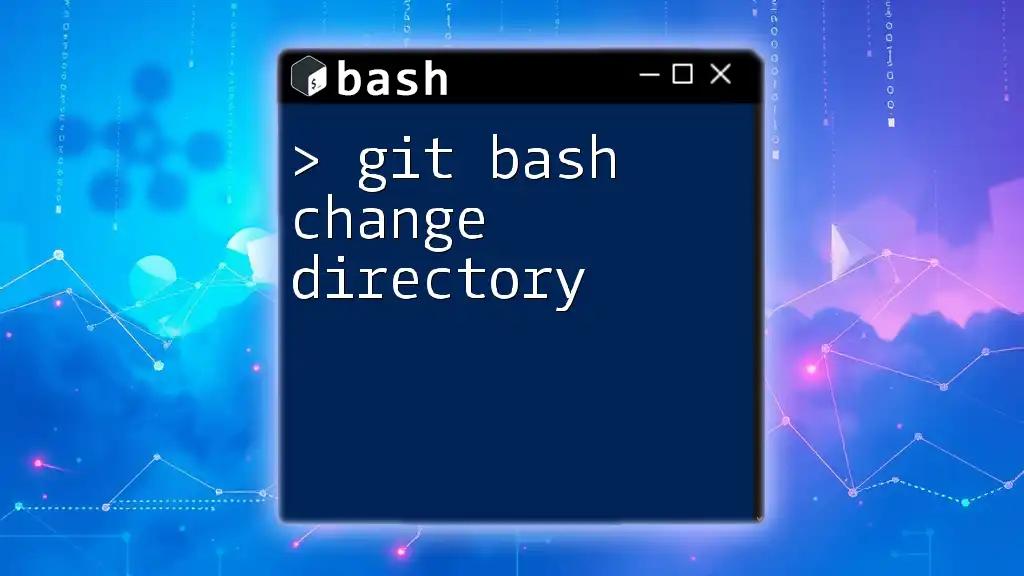
Conclusion
Understanding how to stash changes in Git is essential for effective version control and streamlining your workflow. Whether switching branches or pulling updates, the stash feature enables you to manage your code efficiently without cluttering your commit history. Practicing these stashing techniques will certainly improve your working experience with Git, allowing you to focus on developing rather than managing interruptions.
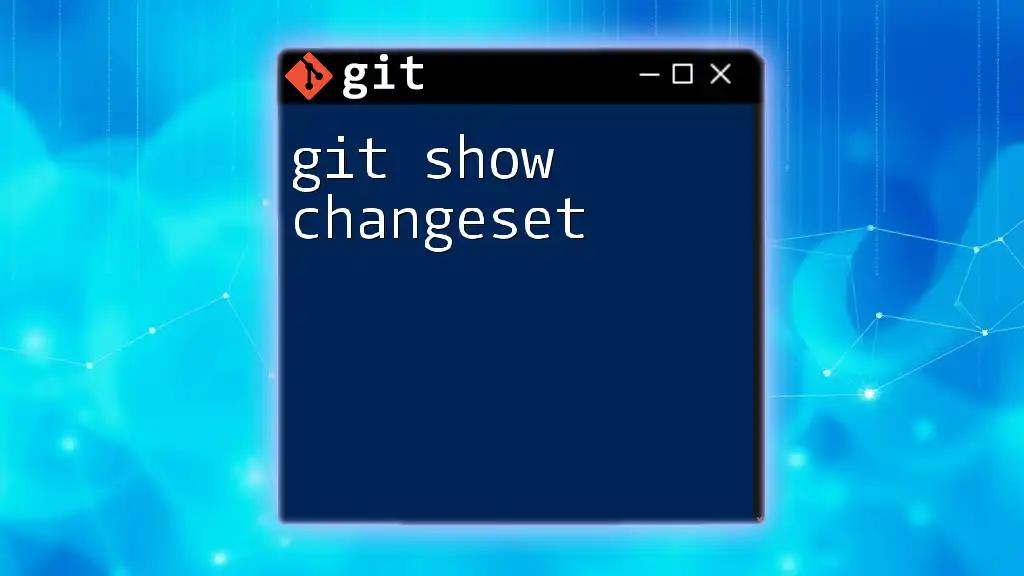
Additional Resources
For more advanced techniques and in-depth learning, consider referring to the official Git documentation and participating in community forums. These resources provide additional insights and help foster greater mastery over Git and its features.