To use Git effectively, simply familiarize yourself with essential commands that help you manage versions of your code, such as initializing a repository and committing changes. Here's a quick example of initializing a new Git repository and making your first commit:
git init # Initialize a new Git repository
git add . # Stage all changes for commit
git commit -m "Initial commit" # Commit the staged changes with a message
What is Git?
Git is a powerful distributed version control system designed to handle everything from small to very large projects with speed and efficiency. Unlike traditional version control systems, which may maintain a central repository, Git enables every developer to have a full copy of the project history on their local machine. This allows for offline access, improved collaboration, and more robust data integrity.
Key features of Git include:
- Snapshot-based architecture: Git tracks changes as snapshots of your files rather than as a set of file changes, making it faster and more efficient at storing and retrieving historical versions.
- Branching and Merging: Git facilitates the creation of branches to allow for parallel development, enhancing collaboration among multiple team members. Merging enables seamless integration of changes made in different branches.
By grasping the fundamentals of how to use Git, you can effectively manage project versions and collaborate with others.
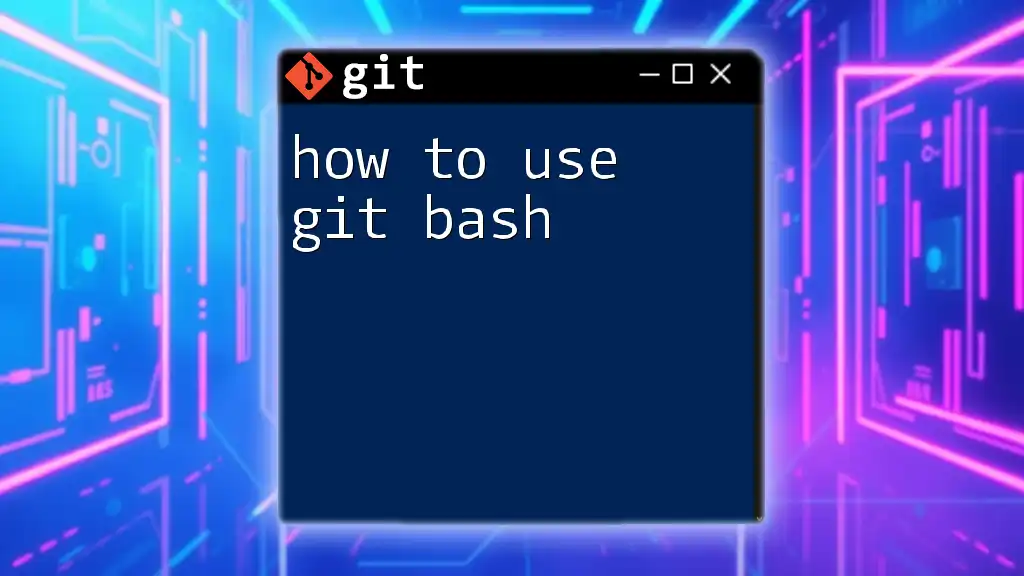
Getting Started with Git
Installing Git
Before you can learn how to use Git, you need to install it.
-
For Windows: Download the Git installer from the official Git website. Follow the setup wizard, and make sure to select the options that suit your needs, particularly ones related to your command line preferences.
-
For macOS: Open your Terminal and type:
brew install git
(This requires Homebrew to be installed).
-
For Linux: Use your distribution's package manager. For instance:
sudo apt-get install git
Configuring Git
Once Git is installed, you’ll need to configure it to identify yourself. This can be done using the following commands in your terminal:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
After configuring, you can check your settings using:
git config --list
This command provides a list of all the configuration settings you've applied.
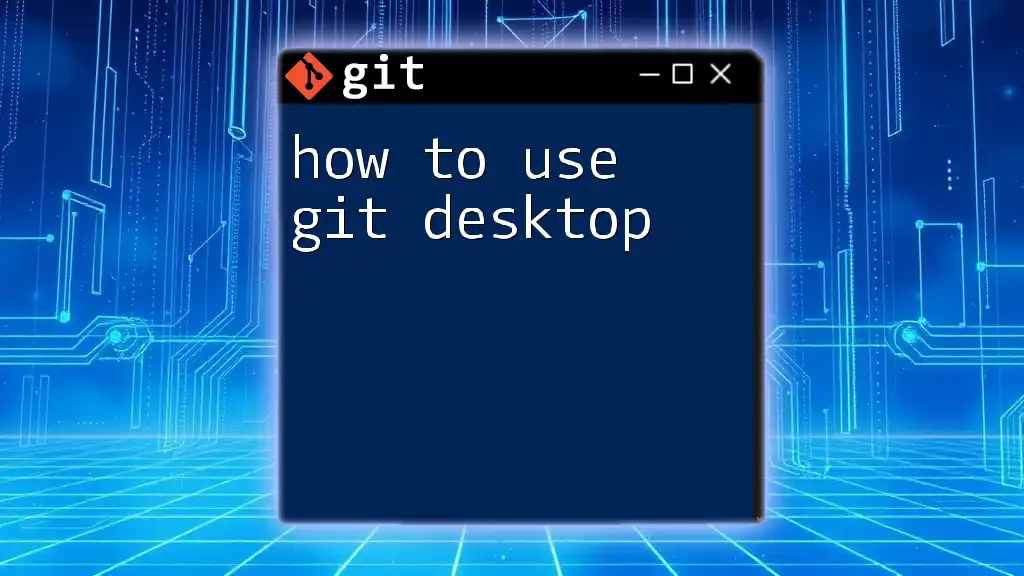
Basic Git Commands
Creating a Repository
To start using Git, you'll need a repository:
-
Initializing a new Git repository: Navigate to your project folder and type:
git init
This command creates a new `.git` directory that contains all the necessary metadata for your repo.
-
Cloning an existing repository: If you want to copy an existing Git repository, use this command:
git clone [repository-url]
For example, to clone a public GitHub repository, use:
git clone https://github.com/user/repo.git
Tracking Changes
After your repository is set up, you can start tracking changes.
-
Checking the status of your repository:
git status
The output will show the current state of your working directory, including which files have been modified or staged.
-
Staging changes for commit: If you've modified files and are ready to include those changes in your next commit, use:
git add [file-name]
To stage all changes at once:
git add .
-
Committing changes: This step records the staged changes to your repository history. Use:
git commit -m "Meaningful commit message"
Commit messages should be specific and concise about the changes made.
Viewing the History
Understanding the project history is crucial.
-
Examining commit history: To view all your commits, run:
git log
This will display a log of recent commits including commit IDs, authors, and messages.
-
Viewing changes made between commits: To see the differences between the last two commits, use:
git diff HEAD~1 HEAD
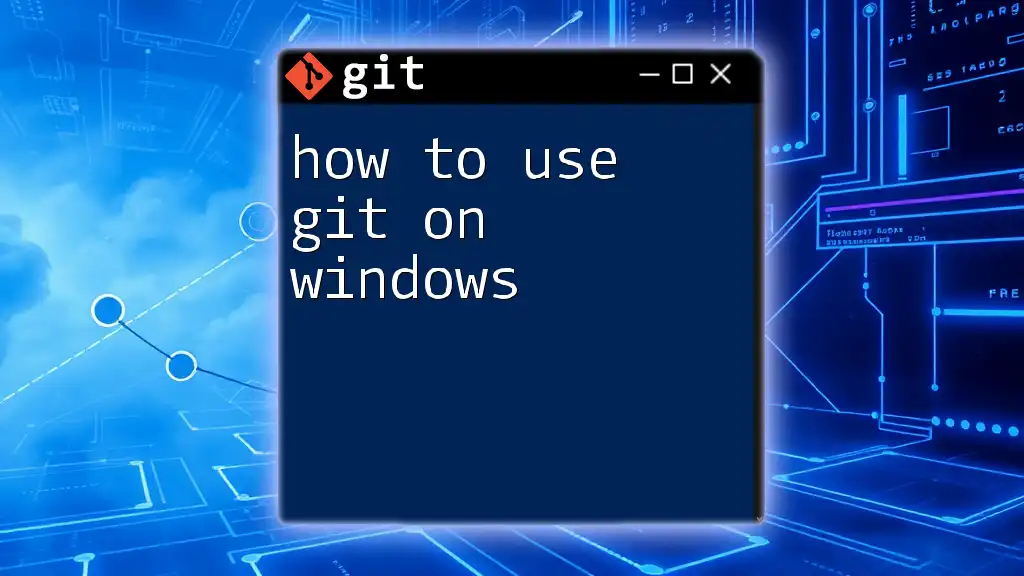
Branching and Merging
Understanding Branches
Branches are essential to doing effective work in Git, enabling you to work on different features or bugs simultaneously without affecting the main codebase.
- Creating a new branch:
To create a new branch, type:
This does not switch to the new branch immediately; you’ll need to check it out next.git branch [branch-name]
Switching Branches
- Changing branches:
Use the following command to switch to your newly created branch or any existing branch:
This enables you to work on the code associated with that branch.git checkout [branch-name]
Merging Branches
When you've completed work on a feature branch, it's time to merge it back into the main branch.
-
Merging changes: First, switch to the branch you want to merge into (usually `main`), then use:
git merge [branch-name]
-
Handling merge conflicts: Occasionally, changes on different branches conflict with one another. Git will prompt you to resolve these before finalizing the merge. You will need to manually edit the files to resolve the conflicts and then use:
git add [resolved-file] git commit
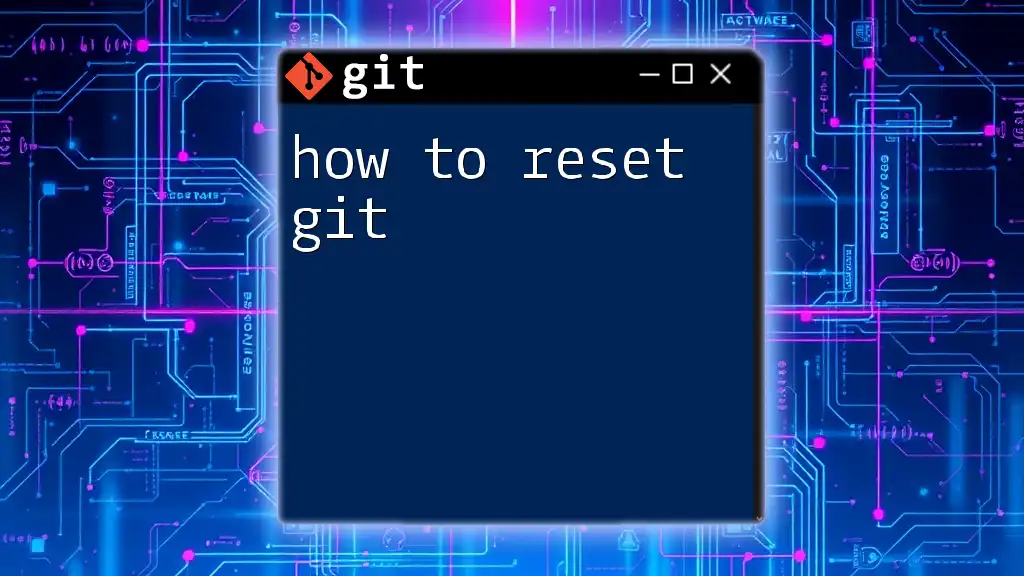
Remote Repositories
Working with Remotes
Remote repositories are crucial for collaboration. They serve as central points where developers can push their changes or pull updates from others.
- Adding a remote:
To link your local repository with a remote one, use:
git remote add origin [repository-url]
Fetching and Pulling Changes
-
Fetching changes: Use this command to download changes from the remote without merging:
git fetch
-
Pulling changes: To download and merge changes in one command, use:
git pull
Pushing Changes
To upload your local commits to the remote repository, use:
git push origin [branch-name]
If this is your first push on a new branch, you might need to set the upstream branch:
git push --set-upstream origin [branch-name]
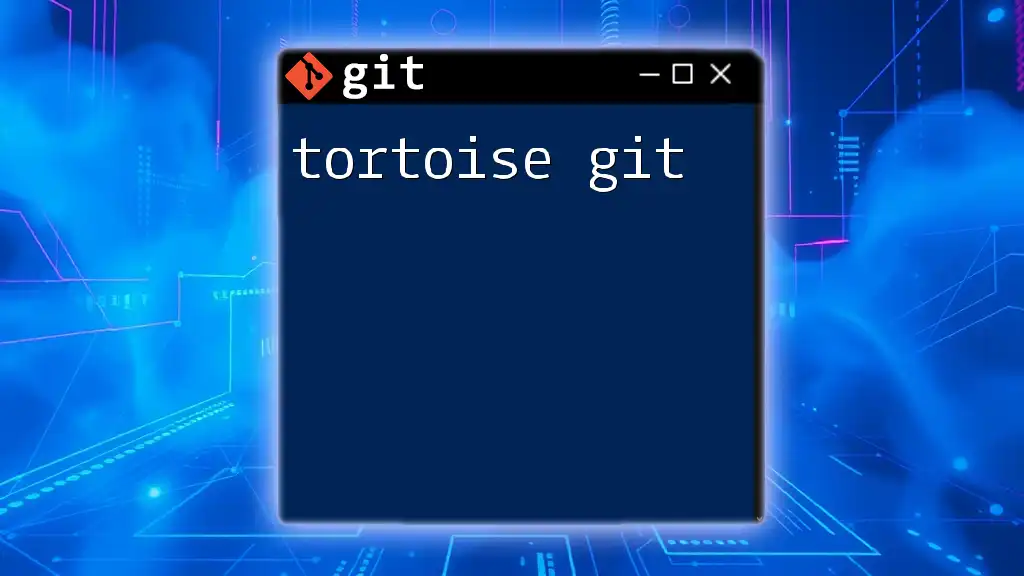
Common Git Workflows
Feature Branch Workflow
This workflow allows developers to create a new branch for each feature or bug fix. It promotes collaboration and reduces the risk of conflicts by isolating changes until they are ready for production.
- Create a new branch for the feature.
- Work on the feature and commit changes.
- Merge the feature branch back into the main branch when complete.
Git Flow
The Git Flow methodology outlines several branches that correspond to project stages, such as:
- `master` for production-ready code
- `develop` for the latest delivered development changes
- `feature` for new features
This structured approach ensures clarity and smooth integration of concurrent developments.
Forking Workflow
In collaborative environments, the forking workflow allows multiple contributors to work on independent copies of the project. Changes are made in forks, and then the original repository is updated via pull requests, making it easy to review changes before integrating them.
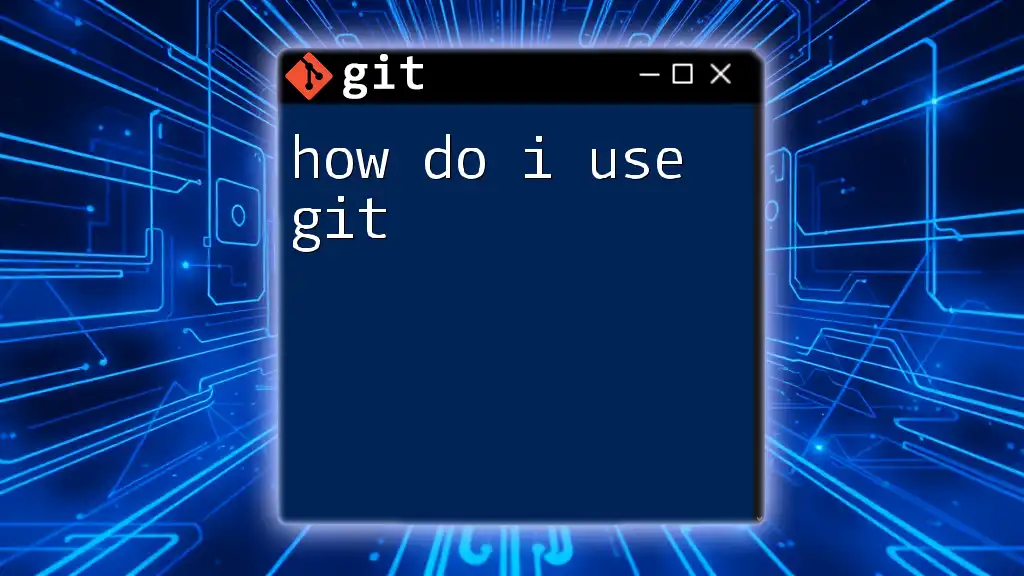
Best Practices
To effectively learn how to use Git, you should adhere to best practices:
-
Write meaningful commit messages that clearly explain what changes have been made and why.
-
Keep commits focused: Each commit should represent a single, logical change to the project, making it easier for teammates to understand your progress.
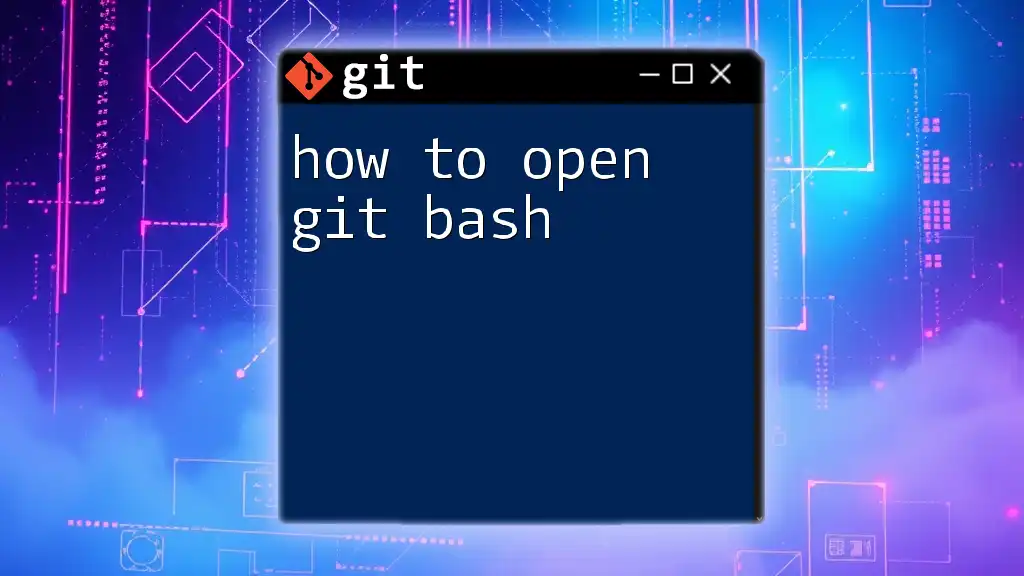
Conclusion
By applying the above concepts and commands, you can confidently manage your projects using Git. This robust version control system empowers you to track changes, collaborate effectively, and maintain the integrity of your code. Don’t hesitate to dive deeper into each command and explore more advanced features as you become comfortable with the basics of how to use Git.