To use Git, you can initialize a repository, make changes, and commit them with the following commands:
git init
git add <file-name>
git commit -m "Your commit message"
What is Git?
Definition of Git
Git is a distributed version control system that allows multiple people to work on a project simultaneously while keeping a detailed history of changes. Unlike centralized version control systems, Git enables local repositories on every developer’s machine, facilitating efficient collaboration and tracking of file modifications.
Key Benefits of Using Git
- Collaboration Across Teams: Git makes it easy for teams to collaborate on projects from different locations, allowing multiple developers to work on the same codebase without overwriting each other's changes.
- Tracking Changes and History: Each commit in Git records a snapshot of the project, enabling users to revert to previous states easily and understand how a project has evolved over time.
- Handling Multiple Versions: You can work on different features or experiment with new ideas without disrupting the main codebase by using branches.
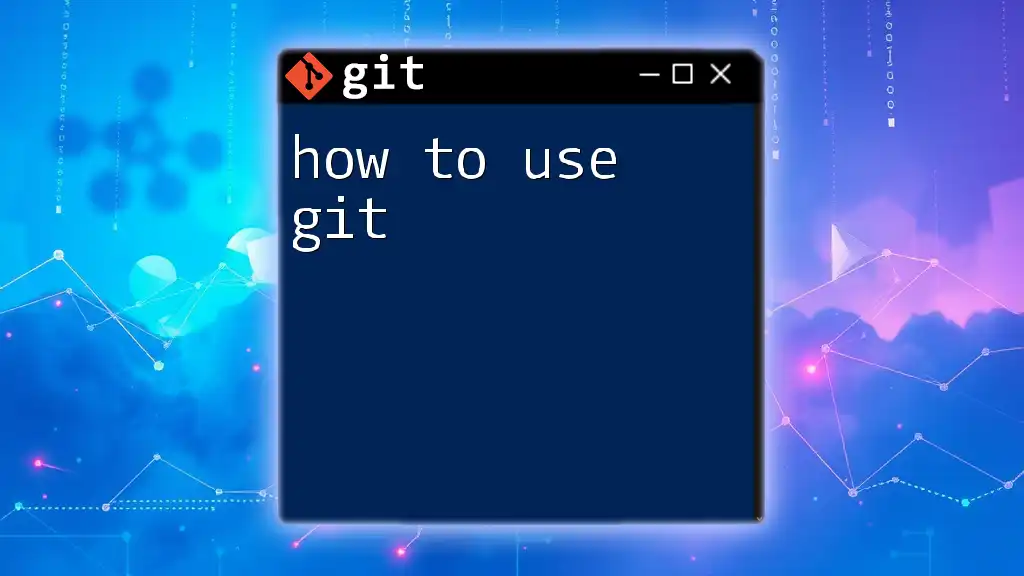
Getting Started with Git
Installing Git
Installing Git is the first step towards leveraging its capabilities.
For Windows: Download the official installer from [git-scm.com](https://git-scm.com). Run the installer and follow the prompts.
For macOS: You can install Git using Homebrew by running:
brew install git
For Linux: Use your distribution’s package manager. For example, on Ubuntu, you can install Git by running:
sudo apt-get install git
Configuring Git
Once installed, you need to set up your global username and email, as these will be associated with your commits. You can do this as follows:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
To verify your configuration, use the command:
git config --list
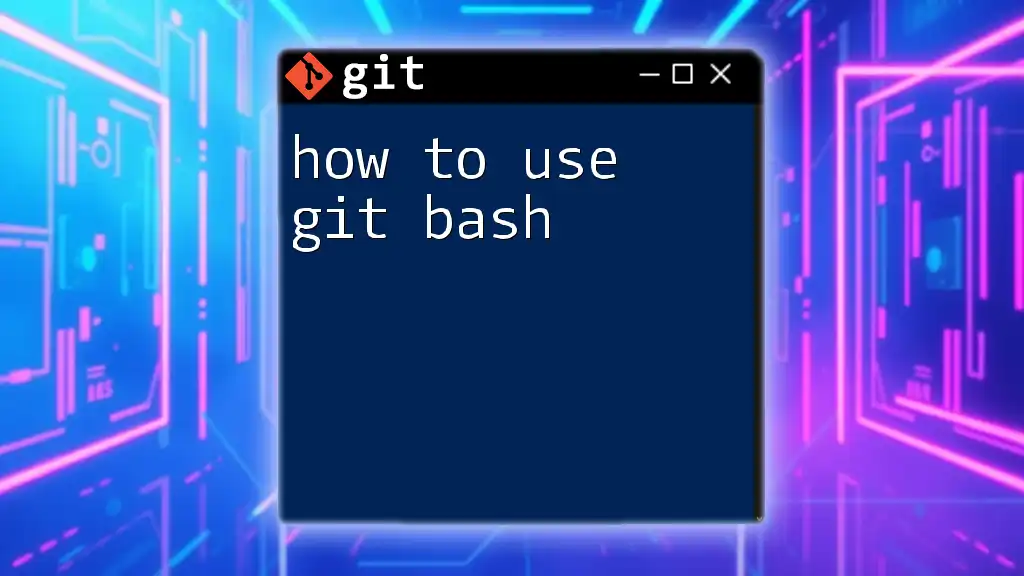
Basic Git Commands
Initializing a Repository
A new project begins with either initializing a local repository or cloning an existing one.
Creating a new repository can be done using:
git init my-repo
This command creates a new directory named `my-repo` with an empty Git repository.
Cloning an existing repository is straightforward:
git clone https://github.com/username/repo.git
This command copies a complete version of the repository from the specified URL to your local machine, including all commit history.
Making Changes
As you modify files in your Git repository, you will need to stage and commit them.
Adding Files
To stage changes and prepare them for a commit, use:
git add filename.txt
Or to stage all modified files, simply run:
git add .
Committing Changes
Once your changes are staged, create a commit by running:
git commit -m "Your commit message"
This command saves the changes you made along with a descriptive message, which is essential for tracking your project's history. It’s best practice to keep commit messages clear and concise.
Viewing Changes
To check the status of your repository and see which files are modified or staged, use:
git status
To view the entire commit history, including details about each commit:
git log
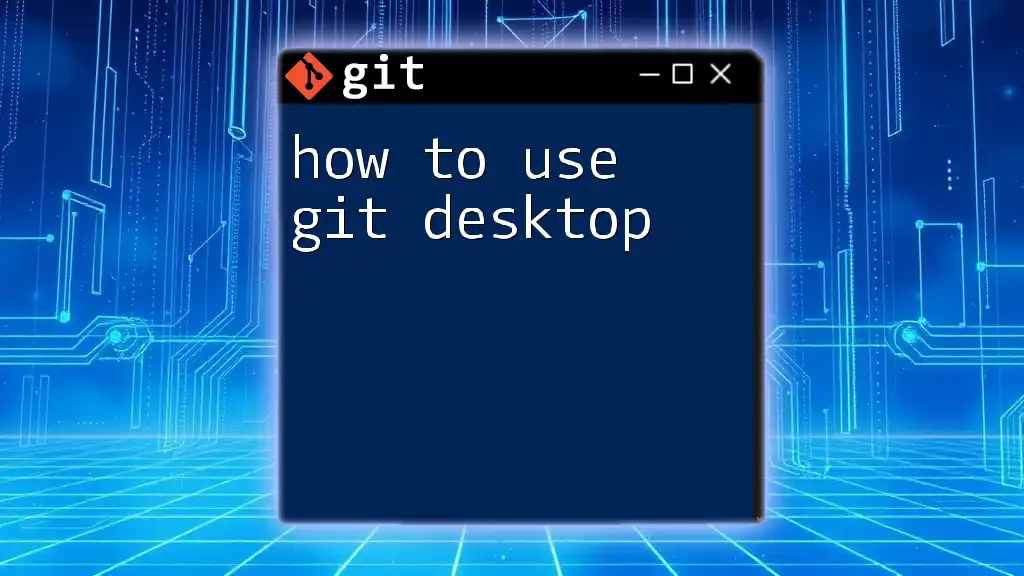
Branching and Merging
Understanding Branches
A branch is a separate line of development in Git that allows you to work on features or experiments independently without affecting the main codebase (often referred to as the main or master branch).
Creating and Switching Branches
To create a new branch, use:
git branch new-feature
To switch to this new branch, run:
git checkout new-feature
For newer versions of Git, you can use:
git switch new-feature
Merging Branches
To integrate changes from one branch into another, first, switch to the branch you want to merge into (e.g., `main`):
git checkout main
Then, run the merge command:
git merge new-feature
If there are conflicts due to changes made in both branches, Git will notify you, allowing you to resolve those conflicts before completing the merge.
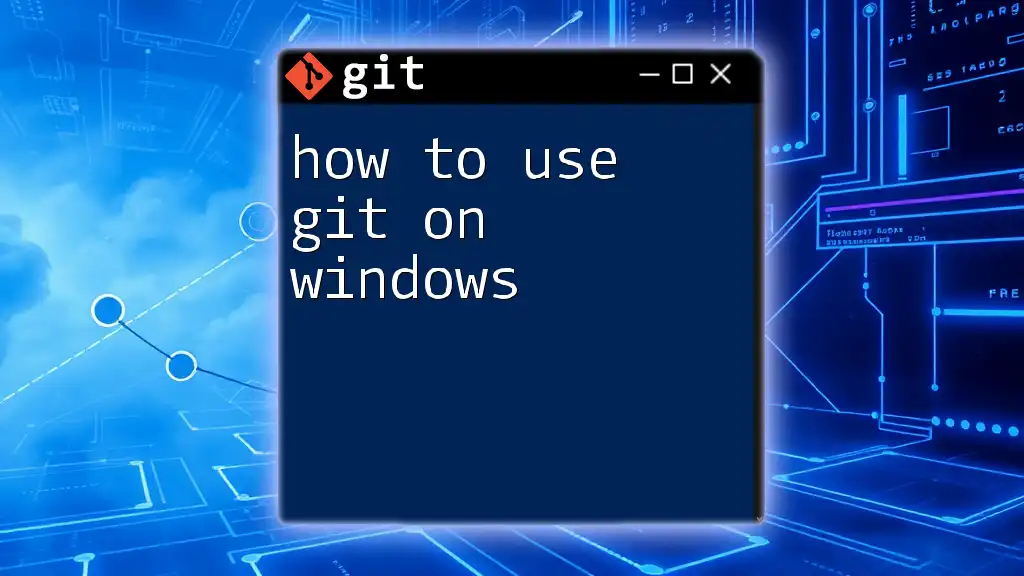
Remote Repositories
Working with Remote Repositories
Remote repositories allow you to collaborate with others and share your work. Understanding how to manage these interactions is crucial.
Adding Remote Repositories
To link your local repository with a remote one, use:
git remote add origin https://github.com/username/repo.git
This command sets the remote repository where your changes can be pushed.
Pushing Changes
To send your commits to the remote repository, run:
git push origin main
This command updates the remote branch with your local changes.
Pulling Changes
To fetch and merge changes from the remote repository into your current branch, use:
git pull origin main
This command helps keep your local repository synchronized with colleagues' changes.
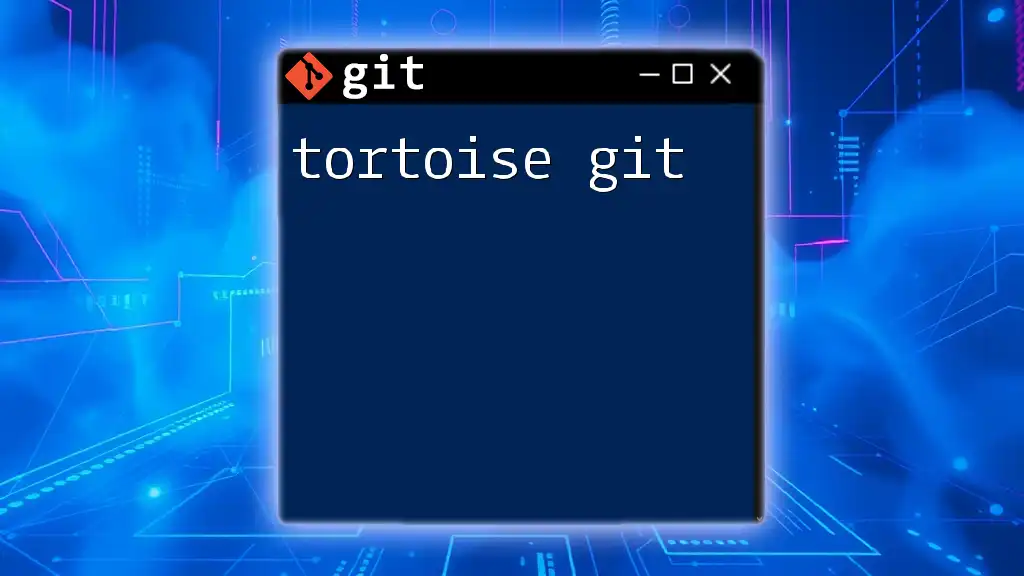
Advanced Git Features
Stashing Changes
When you want to save your changes temporarily without committing, you can stash them. This is useful when you need to switch branches but are not ready to commit your work:
git stash save "description of stashed changes"
To apply the stashed changes later, use:
git stash pop
Tags
Tags are useful for marking specific points in your project’s history, such as release versions. To create a tag, run:
git tag -a v1.0 -m "Version 1.0"
You can list all tags with:
git tag
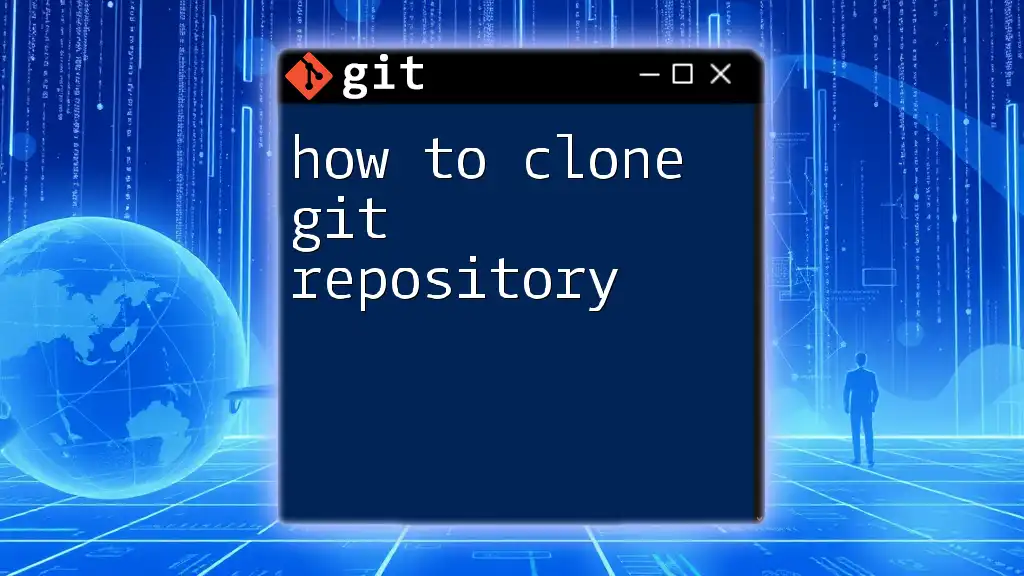
Best Practices for Using Git
- Regular Committing and Pushing: Commit frequently to track small changes and push often to back up your work.
- Writing Meaningful Commit Messages: Use clear, descriptive commit messages to make the history understandable at a glance.
- Keeping Your Repository Organized: Maintain a logical structure, use branches appropriately, and delete stale branches as needed.
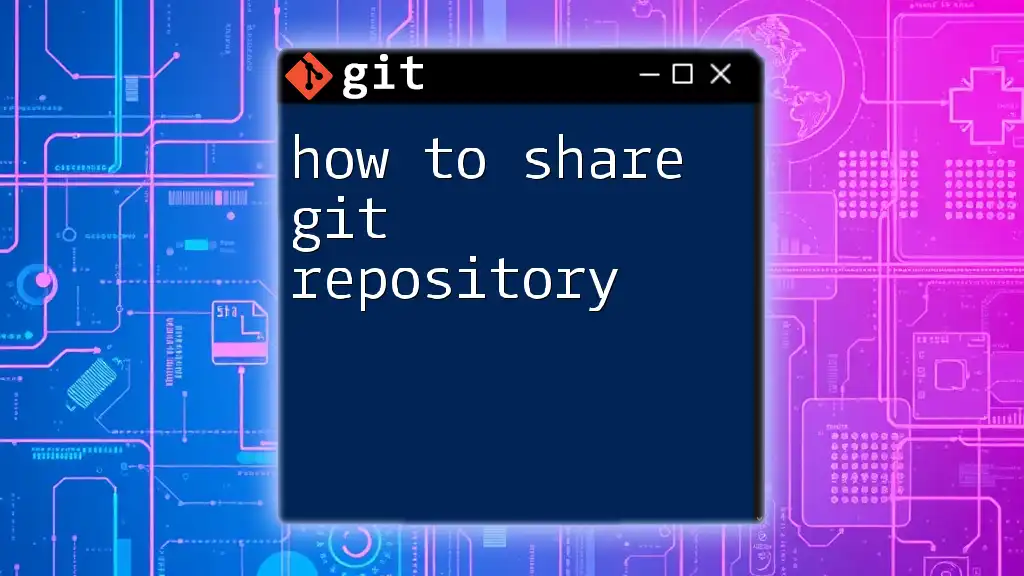
Conclusion
Mastering Git can significantly enhance your development workflow by promoting efficient collaboration, traceability, and management of code changes. By understanding and utilizing Git commands, you can work more effectively on projects, whether solo or as part of a team.
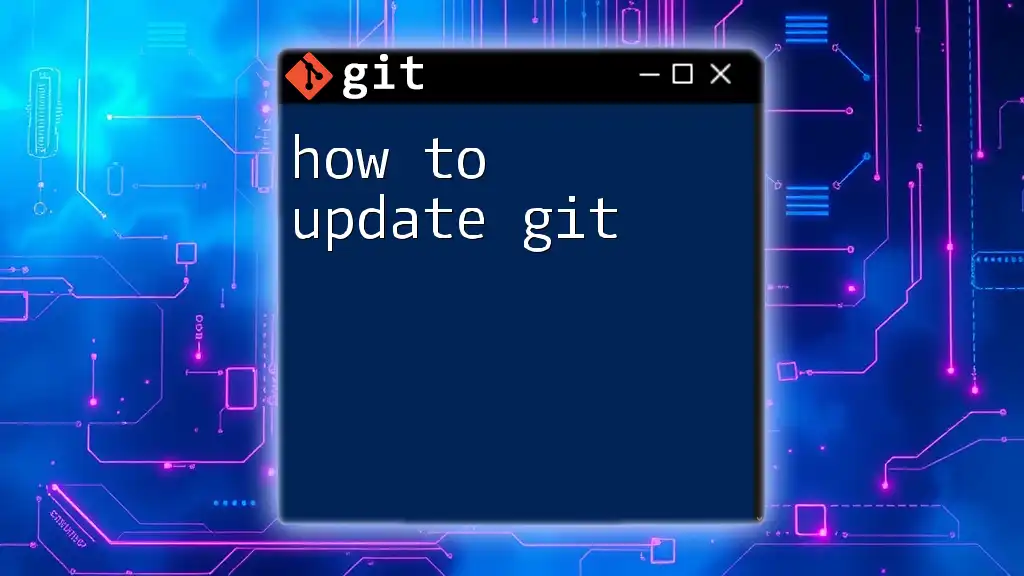
Additional Resources
Explore the [official Git documentation](https://git-scm.com/doc) for further reading. Check resources like GitHub and Stack Overflow for community support, tutorials, and examples of Git in use. Consider starting small projects to apply what you've learned and build confidence in using Git.
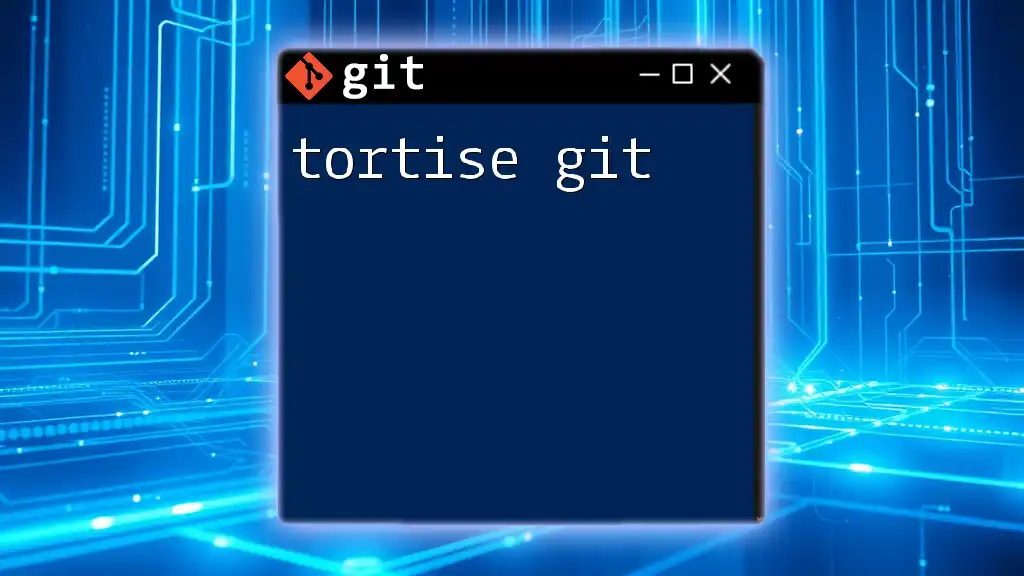
Call to Action
Now that you've learned how to use Git, share your experiences! Have questions about using specific commands or workflows? Feel free to reach out for more guidance or to discuss your Git journey!