To unstage a file in Git, you can use the `git reset` command followed by the file name you wish to unstage.
git reset <file-name>
Understanding the Staging Area
What is the Staging Area?
The staging area—often referred to as the "index"—is a critical component of the Git version control system. It acts as a bridge between your working directory (the current files you're editing) and the repository (the stored versions of your project). Understanding the staging area is essential when learning how to unstage a file in git, as it helps clarify where files stand in the versioning process.
In a typical workflow, you edit files in your working directory, stage them (a way of telling Git you want to include changes in the next commit), and then commit them to your repository.
Why You Might Need to Unstage Files
There are several scenarios where you might find yourself needing to unstage files:
- Committed the Wrong File: You staged a file that you later realize isn't ready for inclusion, perhaps because it contains unfinished work.
- Need to Make Additional Changes: You’ve discovered additional modifications are necessary before committing.
- Mistakenly Added Unwanted Files: Sometimes, you accidentally stage files that should remain outside the commit.
Knowing how to effectively unstage files is vital for maintaining a clean and functional commit history.

How to Unstage a File
Using the `git reset` Command
Overview of `git reset`
The `git reset` command is a powerful tool for untracking changes from the staging area. It's important to understand its implications, as it can also alter the state of your working directory, depending on how you use it.
Command to Unstage a File
To unstage a file, the command you will use is:
git reset <file>
Example: Imagine you've added a file called `unwanted_file.txt` that you now need to unstage. The command will look like this:
git add unwanted_file.txt
git reset unwanted_file.txt
After executing this command, `unwanted_file.txt` will be removed from the staging area and returned to the working directory without changing the file itself.
Using a More Refined Method with Git GUI Tools
Introduction to Git GUI Tools
While command-line usage is powerful, many users may prefer a graphical interface for managing Git operations. GUI tools like GitKraken, SourceTree, or GitHub Desktop simplify the process of unstaging files through visual interactions.
Steps to Unstage Using a GUI Tool
If you are using GitKraken, for example, follow these steps:
- Open GitKraken and navigate to your repository.
- Under the Staged Files section, right-click on the file you wish to unstage.
- Select the Unstage option from the context menu.
This approach provides a more visual method of managing staged and unstaged files and can be helpful for users who are less comfortable with command-line syntax.

Examples of Unstaging Files
Single File Example
Unstaging a single file is straightforward. For instance, if you want to unstage a file named `example.txt`, you would:
git add example.txt
git reset example.txt
Here, `example.txt` moves back to the working directory, allowing you to make further changes before potentially adding it again.
Multiple Files Example
To unstage multiple files simultaneously, you can specify each file in the `git reset` command:
git add file1.txt file2.txt
git reset file1.txt file2.txt
This command illustrates how easy it is to manage several files with a single operation. It's efficient, especially when working on larger projects with numerous changes.
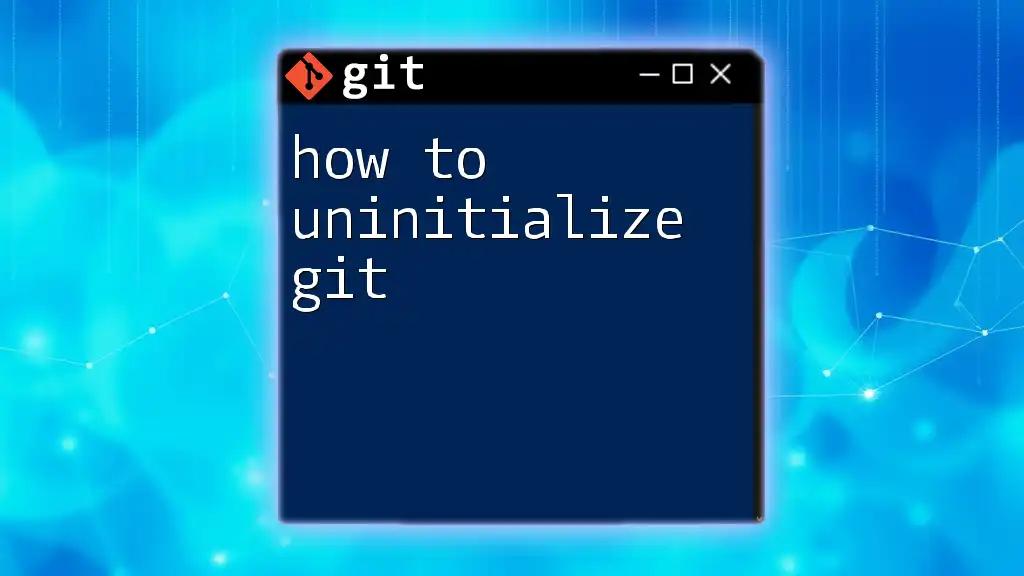
Verifying Your Changes
Using `git status`
After you have unstaged files, it's essential to verify your changes. This can be done with the following command:
git status
The output will display a list of staged files and files that are no longer staged, clearly showing where each file stands in the process. This verification step is crucial for ensuring that your staging area reflects your intentions.
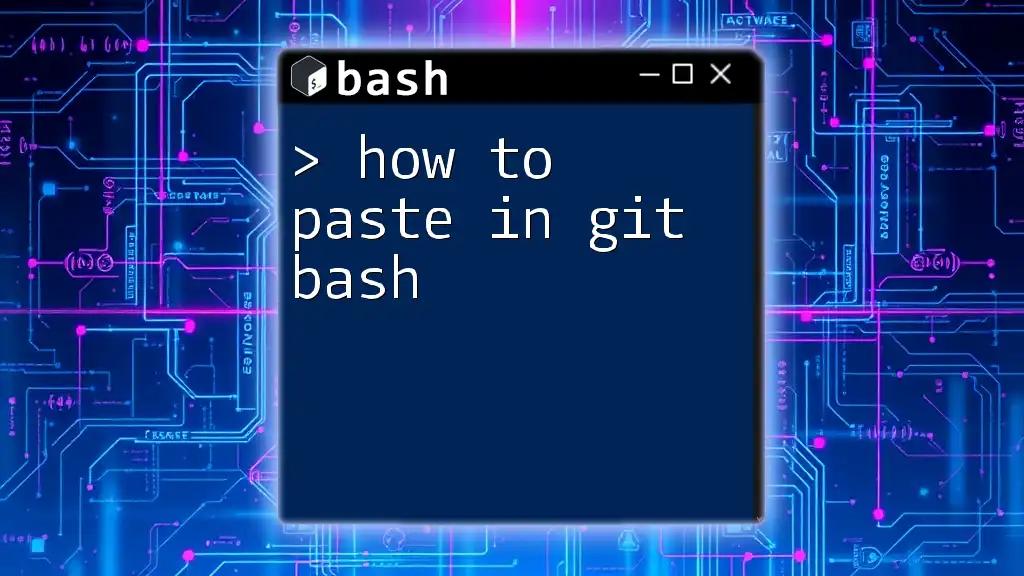
Common Issues and Solutions
Forgetting to Add Files
One common pitfall is forgetting to stage a file before committing. If this happens, you can simply stage your files and make a new commit that includes all the changes.
Unintended Resets
Accidentally unstaging the wrong file is also a possibility. If this occurs, don't worry—you can quickly re-add the file with:
git add <file>
Understanding the implications of `git reset` and how it alters the staging area will minimize frustrations.
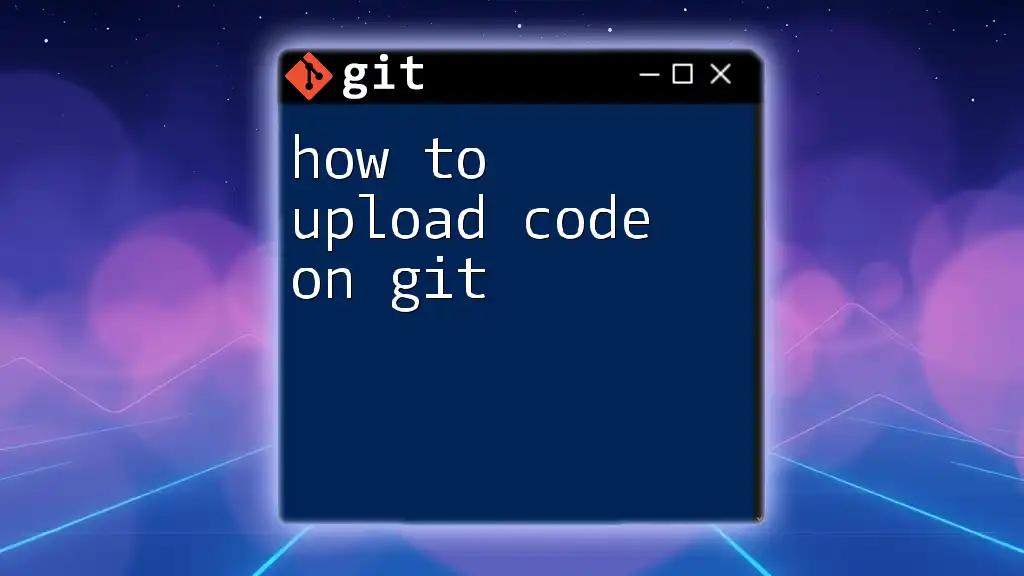
Conclusion
Knowing how to unstage a file in git is a vital skill for managing your project's version control effectively. By understanding the staging area and familiarizing yourself with `git reset`, you can maintain a cleaner commit history and avoid common pitfalls.
Practice these unstaging commands and methods frequently to reinforce your learning and improve your comfort level with Git control.
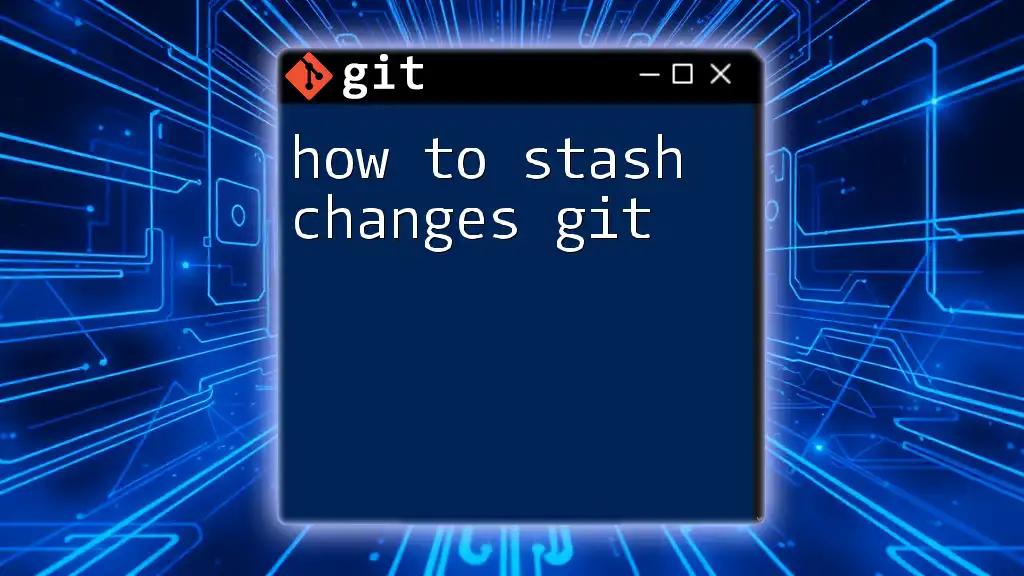
Additional Resources
For further exploration of Git features and commands, consult the [official Git documentation](https://git-scm.com/doc). Additionally, various books and tutorials delve into deeper Git functionalities that can enhance your version control skills.

FAQs
What is the difference between `git reset` and `git checkout` in this context?
While both commands manipulate the state of files, `git reset` is used specifically for altering the staging area, while `git checkout` can change the current branch or switch files in the working directory to previous states.
Can I unstage all files?
Yes, to unstage all files, you can simply execute:
git reset
This command removes all files from the staging area.
Is there a way to undo the unstage?
If you've mistakenly unstaged a file and need to re-add it, just use:
git add <file>
This command allows you to bring whatever file you need back into the staging area.
By mastering these concepts, you're not only enhancing your Git proficiency but also ensuring a smoother development process in your projects.