To undo a git commit, you can use the command `git reset --soft HEAD1` to remove the last commit while keeping your changes staged, or `git reset --hard HEAD1` to discard them completely.
Here's the command syntax:
git reset --soft HEAD~1 # Keep changes staged
or
git reset --hard HEAD~1 # Discard changes completely
Understanding Git Commits
What is a Git Commit?
A Git commit encapsulates a snapshot of your project at a specific moment in time. Every time you commit, Git saves your changes and creates a unique identifier (hash) that allows you to track, navigate, and reference those changes later. Commits play a crucial role in version control, enabling collaboration, historical analysis of the project, and recovery from errors.
Why You Might Need to Undo a Commit
There are several situations in which you might find yourself needing to undo a commit. These include:
- Mistakes in code: If you realize that a recent commit introduced bugs or broke functionality.
- Incorrect commit messages: If you mistyped or misrepresented the changes in the commit message.
- Accidental commits: When you accidentally commit changes you didn't intend to include.
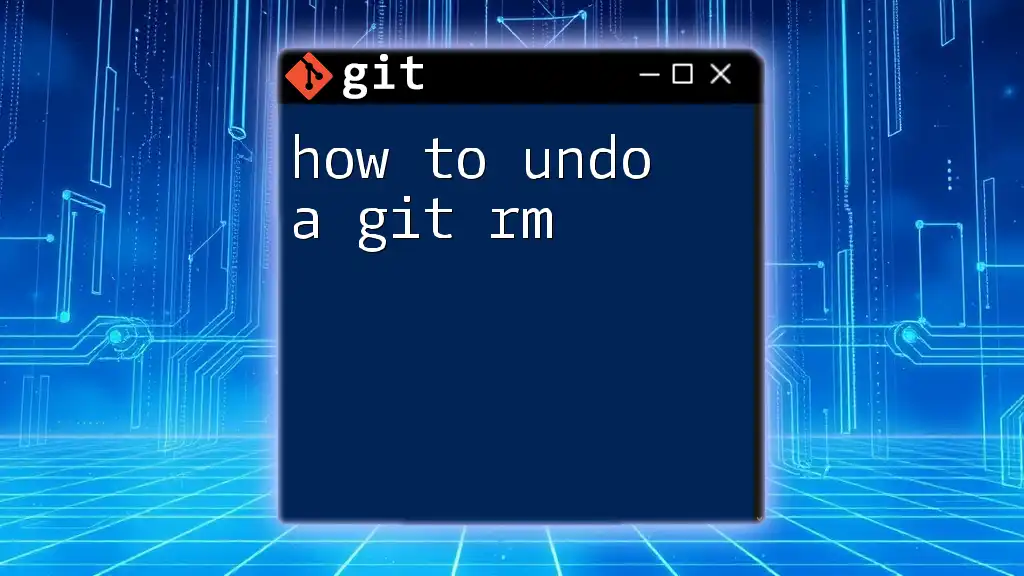
Basic Commands for Undoing Commits
Using `git reset`
What is `git reset`?
The `git reset` command alters your repository history by moving the current branch pointer backward. It can affect your working directory and staging area, depending on the reset mode you choose.
Different Modes of `git reset`
-
Soft Reset
A soft reset allows you to undo a commit, keeping the changes in your staging area. This is particularly useful if you need to amend a commit or make additional adjustments before recommitting.Example:
git reset --soft HEAD~1
This command moves the HEAD pointer back by one commit, retaining your changes staged for the next commit.
-
Mixed Reset
The mixed reset is the default mode for the `git reset` command, and it undoes the commit while un-staging the changes. This gives you a chance to correct your changes before re-staging and committing again.Example:
git reset HEAD~1
This command will move the HEAD back by one commit, and any changes made will remain in your working directory but not staged.
-
Hard Reset
A hard reset is a drastic approach that not only undoes commits but also discards all changes in your working directory. Be wary, as this can lead to permanent loss of uncommitted work.Example:
git reset --hard HEAD~1
This command will revert the branch to its state before the last commit, including all changes in the working directory.
Using `git revert`
What is `git revert`?
Unlike `git reset`, which modifies your project history, `git revert` is a safer way to "undo" a commit. It creates a new commit that negates the changes made by previous commits, ensuring that the history remains intact.
How to Revert a Commit
To revert a commit, use the commit hash of the commit you wish to undo.
Example:
git revert <commit_hash>
This creates a new commit that effectively undoes the specified commit's changes. This method is particularly useful when working on shared branches, as it maintains the integrity of the project history.
When to Use `git revert` Over `git reset`
Opt for `git revert` if you are on a public/shared branch or if you want to keep the commit history clear and traceable. Using `git reset` on public branches can lead to confusion and conflicts among team members.
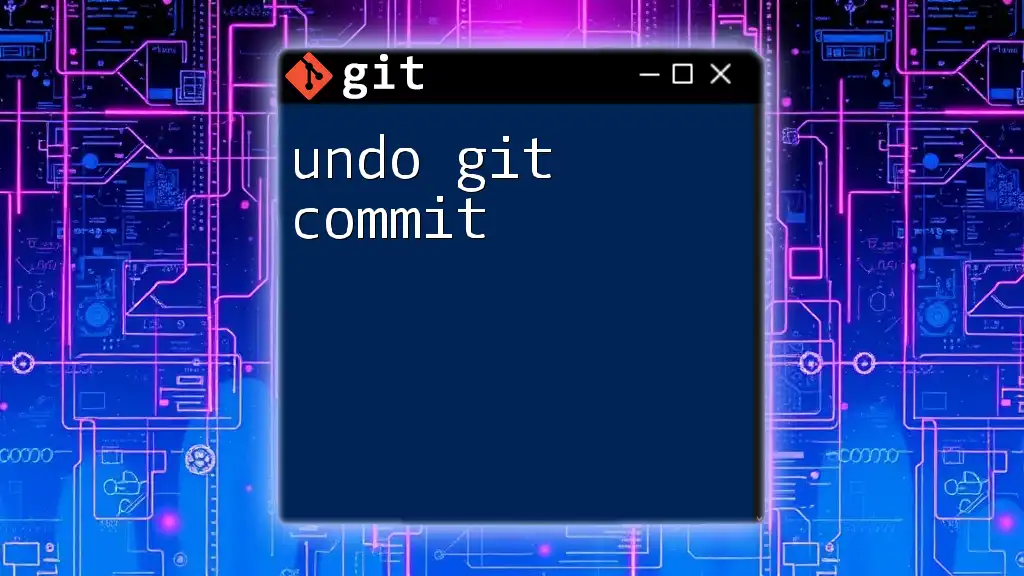
Advanced Techniques for Undoing Commits
Undoing Multiple Commits
Using a Range of Commits
If you need to revert several commits at once, you can specify a range of commits. This is particularly useful when you realize a series of changes need to be backed out.
Example:
git revert HEAD~3..HEAD
This command will create individual revert commits for the last three commits. It’s a clean approach to un-doing multiple changes while keeping history intact.
Configuring Git to Avoid Common Mistakes
Setting Up Aliases
Creating custom command aliases can streamline your workflow in Git, especially for frequent operations like undoing commits.
Example:
git config --global alias.undo 'reset --soft HEAD~1'
With this alias, you can simply run `git undo`, which will execute the soft reset command, making it easier and quicker to undo recent commits.
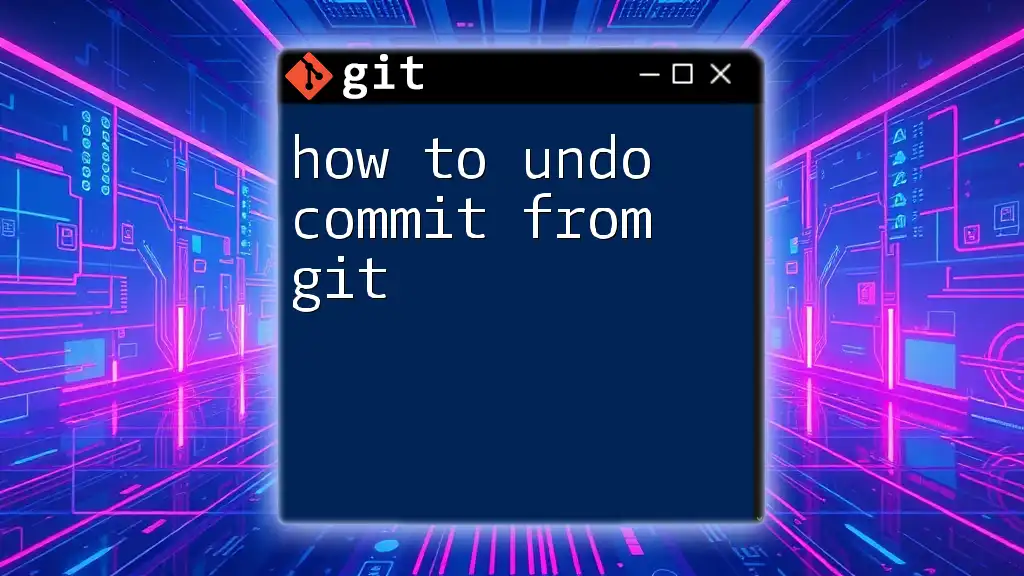
Best Practices for Undoing Commits
Establishing a Commit Policy
Developing a solid commitment policy is key to reducing the frequency of needing to undo commits. This involves crafting clear, meaningful commit messages and breaking large changes into smaller, manageable commits. By adhering to these practices, you not only minimize errors but also enhance collaboration.
Backing Up Changes Before Undoing
Before executing any command that could potentially result in data loss—especially `git reset --hard` or a bulk revert—it's wise to back up your changes. You can do this by creating a temporary branch:
git checkout -b backup-branch
This way, your current state is preserved, providing a safety net even if something goes wrong.
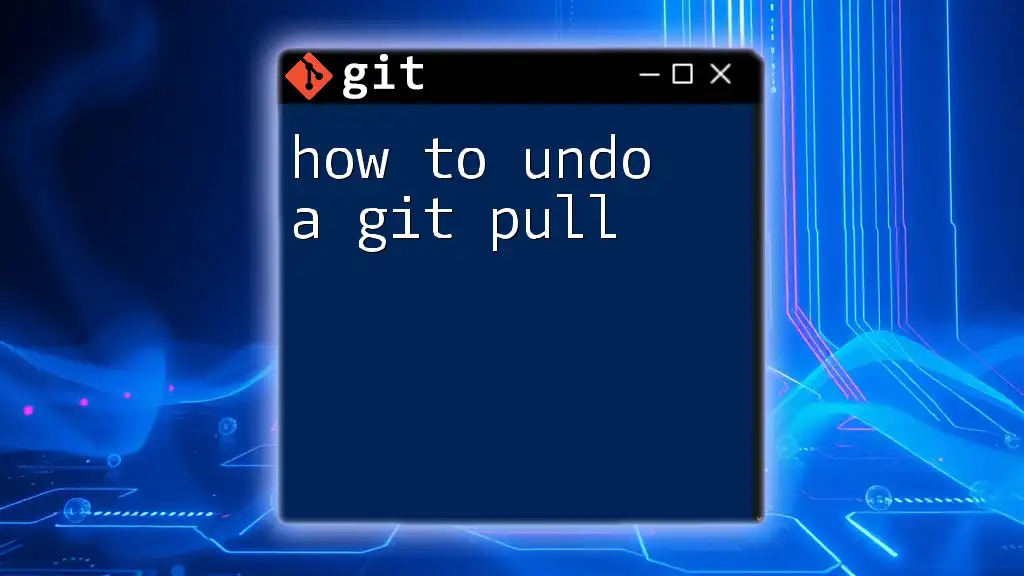
Conclusion
Understanding how to undo a git commit is an essential skill for any developer using Git for version control. Whether it's correcting mistakes, improving commit messages, or managing project history, knowing the right commands and when to use them will greatly enhance your efficiency and confidence in handling your code repositories. Practice these commands in safe environments to gain proficiency and avoid potential pitfalls on future projects.
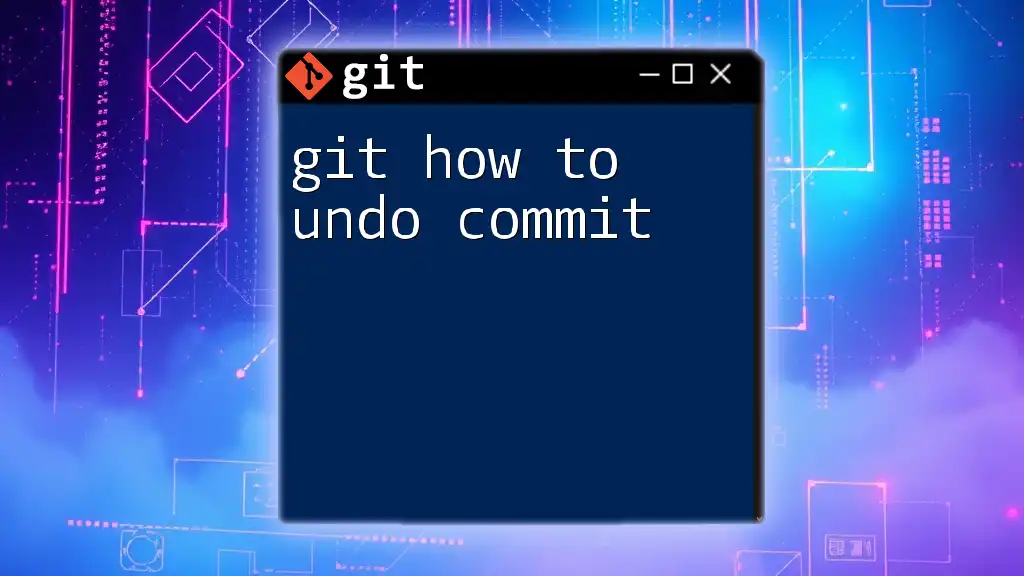
Additional Resources
For further learning, consult the official Git documentation and consider recommended books and online courses dedicated to mastering Git and version control. This continuous learning will empower you to utilize Git effectively and troubleshoot issues with ease.