The `git commit` command is used to save changes made to the files in your local repository, creating a snapshot of the project at that moment.
Here’s an example of how to use it:
git commit -m "Add feature X implementation"
What is a Git Commit?
A commit in Git is a snapshot of your files at a particular point in time. It encapsulates the changes you have made since the last commit, serving as a building block in the history of your project. Each commit contributes to an ongoing, chronological record of your development work.
The importance of commits cannot be overstated. They allow you to return to a specific state of your code, making it easier to identify when a bug was introduced or to revert changes that don’t work as intended. Essentially, every time you make a commit, you're saving an incremental progress check for your project.
The Role of a Commit in Git Workflow
Commits play a crucial role in the Git workflow. When developing software, you often work on multiple changes at once. Before you can commit, you must first stage your changes. Staging allows you to select which changes you want to include in the next commit, giving you fine control over your project’s history.
The process typically involves making modifications to your files, staging these changes, and subsequently committing them. The basic flow looks like this:
- Modify files in your project directory.
- Stage the desired changes using the `git add` command.
- Create a commit with the `git commit` command.
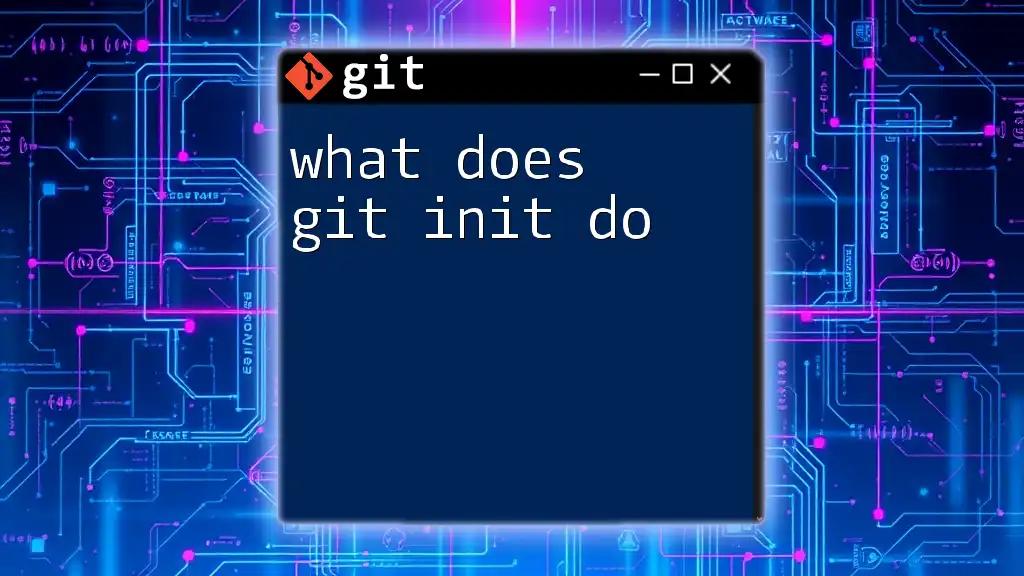
How to Use `git commit`
Using the `git commit` command is straightforward once you understand its syntax. The general command structure looks like this:
git commit -m "Your commit message"
Committing Changes
To effectively commit changes in your repository, follow these steps.
Imagine you have a file named `index.html` that you have just edited. To commit your changes, you would:
-
Edit your file: Make your desired changes in `index.html`.
-
Check status: Use `git status` to see which files have changed. This will help you confirm that your modifications are ready to be staged.
-
Stage your changes: Stage the edited file using the following command:
git add index.html
-
Commit the changes: Finally, commit the staged changes with a meaningful message:
git commit -m "Updated content in index.html"
By following these steps, you effectively save the state of your `index.html` file in your project’s history.
Understanding Commit Messages
A crucial aspect of using `git commit` is writing clear and descriptive commit messages. A well-crafted commit message serves as documentation for what specific changes were made and why they were necessary.
A good commit message typically has a subject line and a body, if needed. The subject line should be concise, summarizing the changes in less than 50 characters. The body can elaborate on the details of the changes if necessary. Here’s a breakdown:
-
Good Commit Message:
Add navigation menu to index.html - Implemented the new navigation menu with dropdown features. - Ensured responsiveness on mobile.
-
Bad Commit Message:
Fixed stuff
Writing meaningful messages not only aids your future self but also helps other team members understand your contributions when reviewing changes.
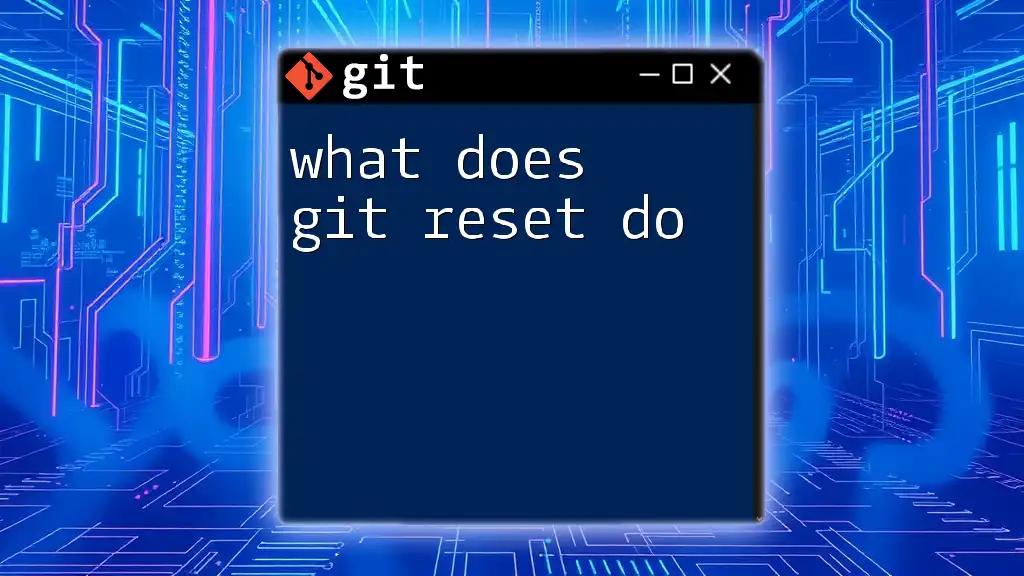
The Flags and Options for `git commit`
Commonly Used Flags
Git offers several flags that enhance the functionality of the `git commit` command.
-
`-m`: This flag is used to add a commit message inline.
-
`-a`: This flag allows you to stage files that have been modified and deleted without needing to run `git add` separately.
Using the `-a` flag can save time in busy workflows. For instance, if you’ve modified several files and want to commit all changes at once, you can use:
git commit -a -m "Updated all modified files"
Interactive Commit
For more control over what gets committed, Git allows you to interactively choose changes to commit using the `--interactive` option. Here’s how to use it:
git commit --interactive
This command lets you interactively select hunks from your modified files, which can be useful when you want to commit only specific changes rather than all modifications.
Amend Last Commit
At times, you might realize you forgot to include some changes in your last commit or need to update the commit message. In such cases, you can use the `--amend` flag to modify the last commit. Here’s how:
git commit --amend -m "Updated commit message"
This command allows you to adjust your most recent commit, whether it's amending the message or adding new changes that were overlooked.
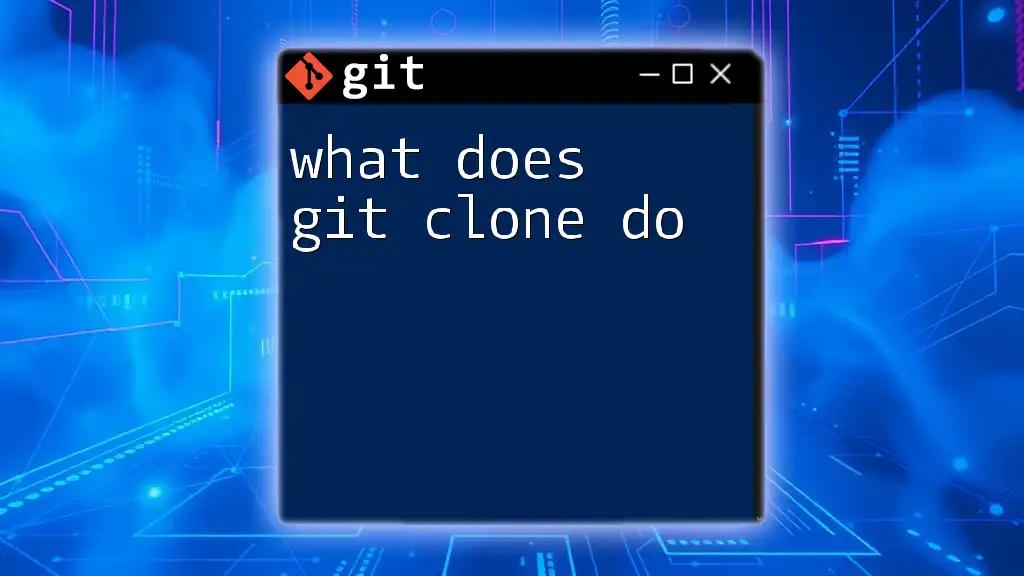
The Commit History
Viewing Commit History
To view your commit history, you can use the command:
git log
This displays a log of all commits made in your repository, including details such as the commit hash, author, date, and message. Understanding the output of `git log` is essential for navigating your project’s history effectively. You can customize the log format using flags like `--oneline` for a concise output or `--graph` for a visual representation of the commit tree.
Reverting Commits
If you need to undo a change, `git revert` allows you to create a new commit that undoes the changes made by a previous commit. The command looks like this:
git revert <commit-hash>
You can easily find the `<commit-hash>` by using `git log`. This approach is preferable for maintaining a clear history, as it doesn’t erase past commits but simply adds a new commit that counteracts previous changes.
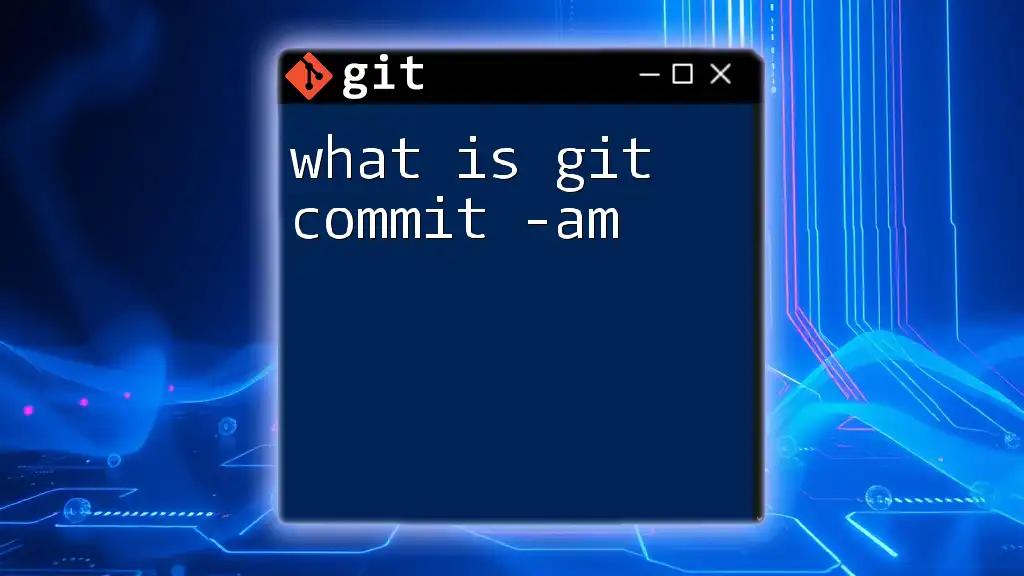
Best Practices for Committing
To maintain good version control practices, consider the following tips:
-
Commit Frequently: It's advantageous to make smaller, more frequent commits. This not only keeps your commit history manageable but also makes it easier to identify where bugs may have been introduced.
-
Meaningful Messages: Always strive for clarity and precision in your commit messages. This habit fosters better collaboration and understanding within your team.
-
Atomic Commits: Try to group related changes together in a single commit. This way, each commit represents a single, coherent change, making it easier to review and understand.
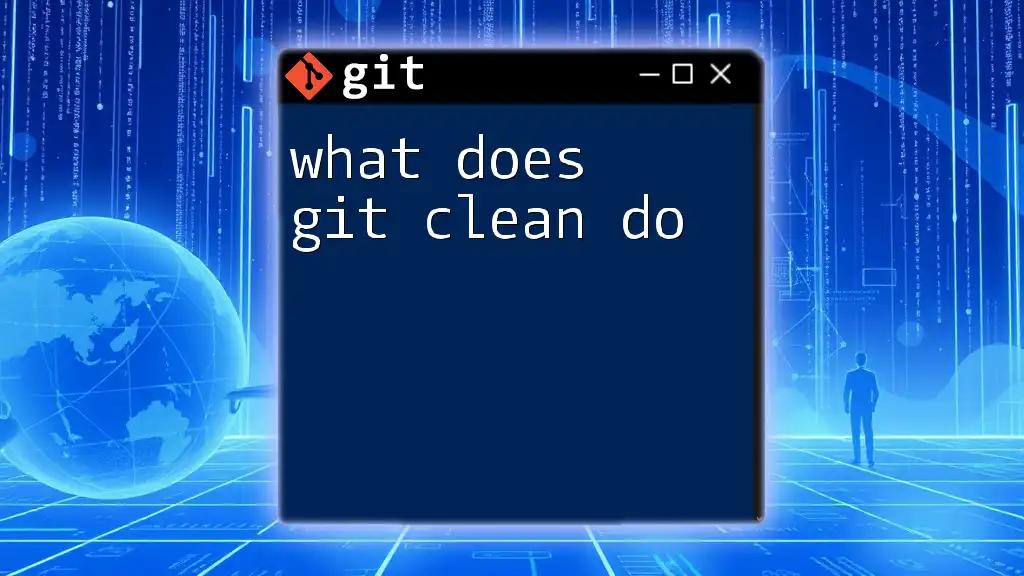
Conclusion
In summary, understanding what `git commit` does is fundamental for anyone working with version control. By utilising this command effectively, along with best practices for clear commit messages and consistent workflows, you can enhance both your individual and team development processes. Practice these tips to master Git, and you'll find yourself more comfortable and efficient in tracking your project’s progress.
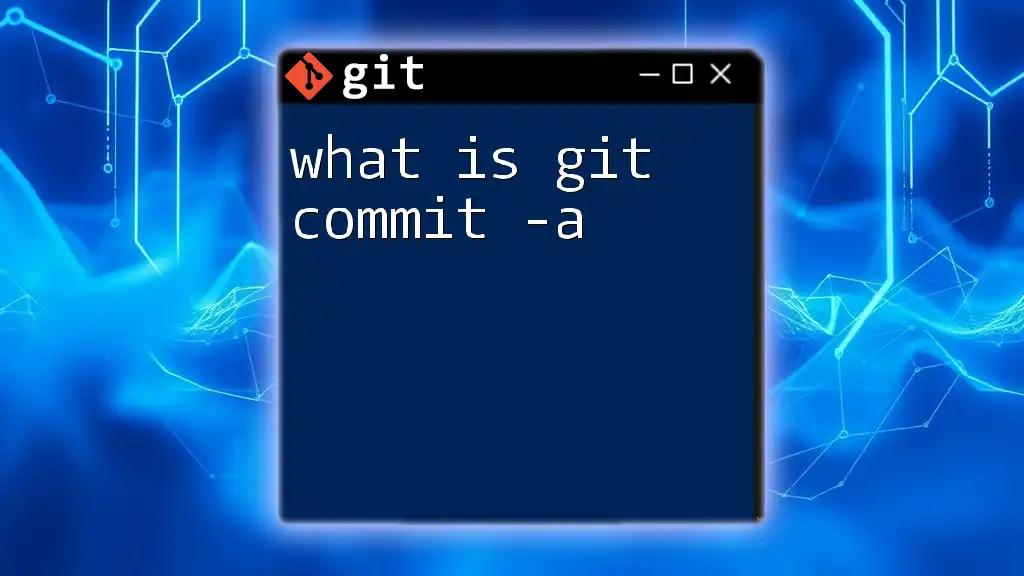
Additional Resources
To further expand your knowledge, consider checking out established Git tutorials, community forums, and official documentation. Engaging with these resources will reinforce your understanding of Git commands and best practices in version control.