Git rebase is a command that allows you to integrate changes from one branch into another, effectively placing your commits on top of another branch's history for a cleaner and more linear project history.
git rebase main
What is Git Rebase?
Git rebase is a powerful command in Git that enables you to incorporate changes from one branch into another. Unlike merging, which creates a new commit that combines the histories of both branches, rebasing alters the commit history by reapplying commits on top of another base commit. This results in a cleaner, linear project history.
Rebasing is especially important in collaborative environments where multiple contributors may be working on varying branches. Understanding rebasing helps teams maintain a clear history of changes, making it easier to track progress and resolve issues.
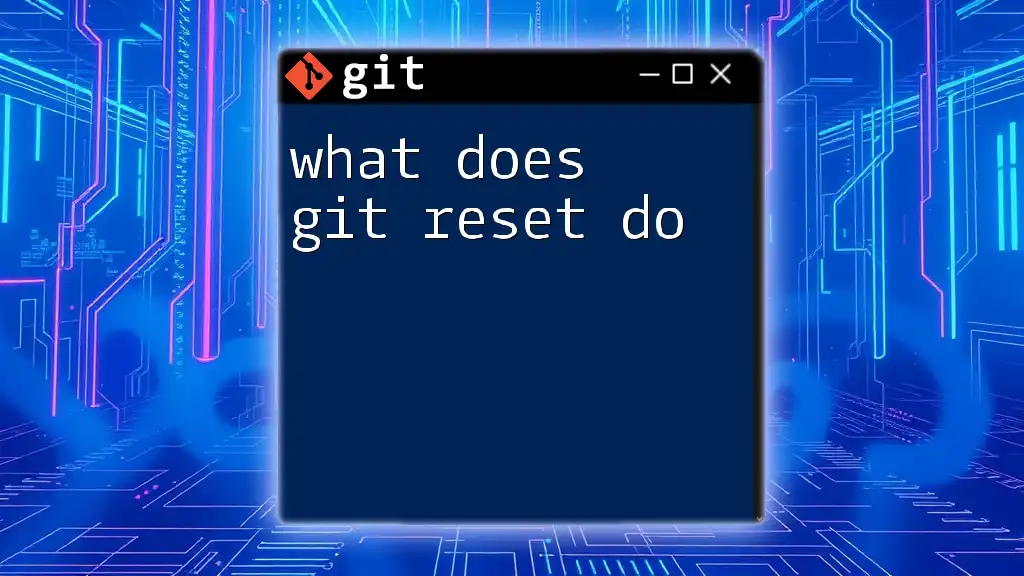
How Does Git Rebase Work?
The Basic Concept
When you run the rebase command, Git takes the commits from the current branch and replays them onto the specified base branch. This essentially means that the changes introduced in the current branch are applied against the state of the base branch at that moment.
The fundamental command for performing a rebase is:
git rebase <base-branch>
This operation changes the commit history, and here’s what happens under the hood:
- Git identifies the common ancestor between the two branches.
- It temporarily saves the changes introduced by the commits in the current branch.
- The base branch is updated to the target commit.
- Finally, Git reapplies the saved commits one-by-one on top of the new base.
The Rebase Process
To illustrate a typical rebase operation, let’s use a practical example:
You have a `feature-branch` that you want to rebase onto the `main` branch. Here’s how you would proceed:
git checkout feature-branch
git rebase main
During this operation, if there are any conflicts between the changes made in `feature-branch` and those in `main`, Git will pause the rebase process, allowing you to resolve those conflicts. Once you've fixed the conflicts, continue the rebase with:
git rebase --continue
This process will repeat until all commits have been successfully reapplied.
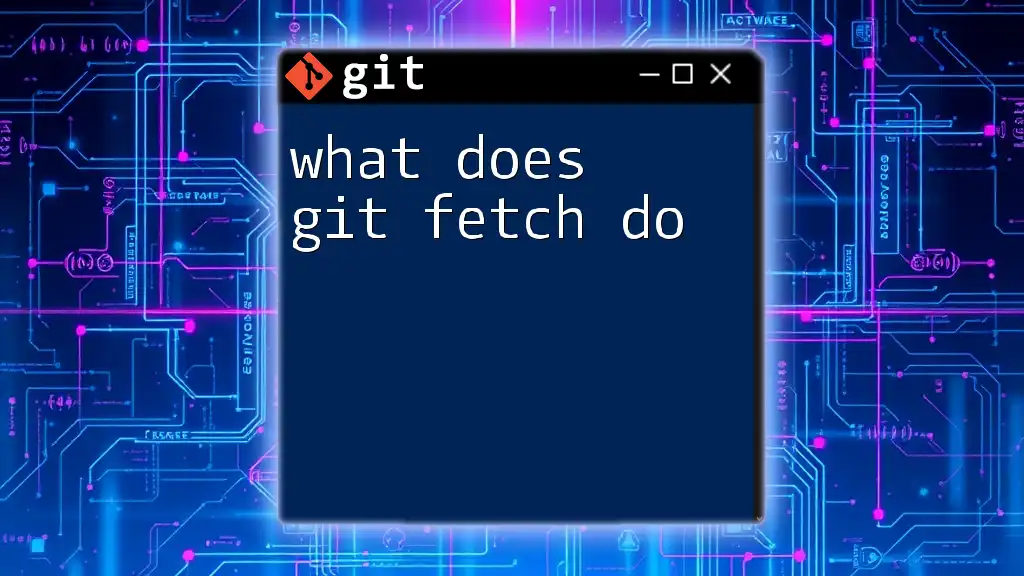
Types of Git Rebase
Interactive Rebase
Interactive rebase is an essential tool within Git that allows you to edit commits during the rebase process. By running an interactive rebase, you can modify commit messages, squash multiple commits into a single one, or even drop commits entirely.
You initiate an interactive rebase with the following command, which lets you go back three commits:
git rebase -i HEAD~3
When you execute this command, Git opens an editor displaying the last three commits. In this interface, you can manage each commit through a set of commands (pick, squash, edit, drop). This level of control provides significant flexibility in managing your commit history and is invaluable for cleaning up messy commit logs before merging to the main branch.
Automatic Rebase
In some cases, Git may perform a basic form of rebase automatically when you use commands like `git pull --rebase`. This command fetches changes from the remote and attempts to reapply your local commits on top of the new changes from the remote repository. While automatic rebase saves you the trouble of manual rebasing, it’s crucial to understand how it works to avoid potential issues down the line.
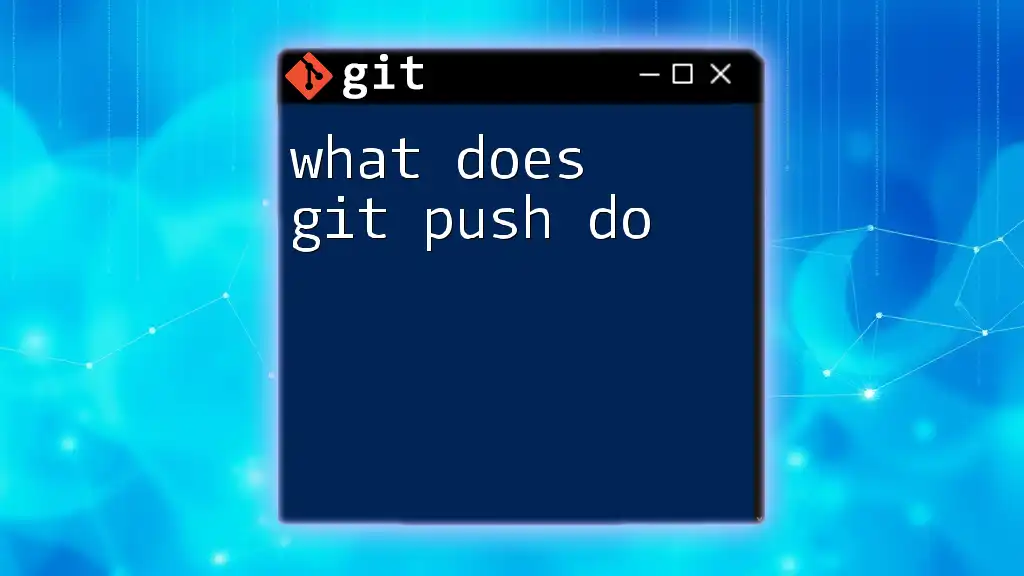
Key Advantages of Using Git Rebase
Cleaner History
One of the primary advantages of using rebase is the ability to maintain a cleaner commit history. When multiple developers are contributing changes, using rebase can help create a linear history that’s easier to understand at a glance. A linear commit log simplifies tracking the project’s evolution, as it eliminates the "merge bubbles" created by frequent merges.
Easier Conflict Resolution
Rebasing can simplify conflict resolution compared to merging because you deal with conflicts on a commit-by-commit basis, rather than having to resolve all conflicts at once. This can make it easier to identify which specific changes are problematic.
For example, consider this rebase command when you want to rebase a `feature-branch` onto `main`:
git checkout feature-branch
git rebase main
If conflicts arise, Git will stop the rebase process at the conflicting commit, allowing you to address the conflict immediately before proceeding to the next commit.
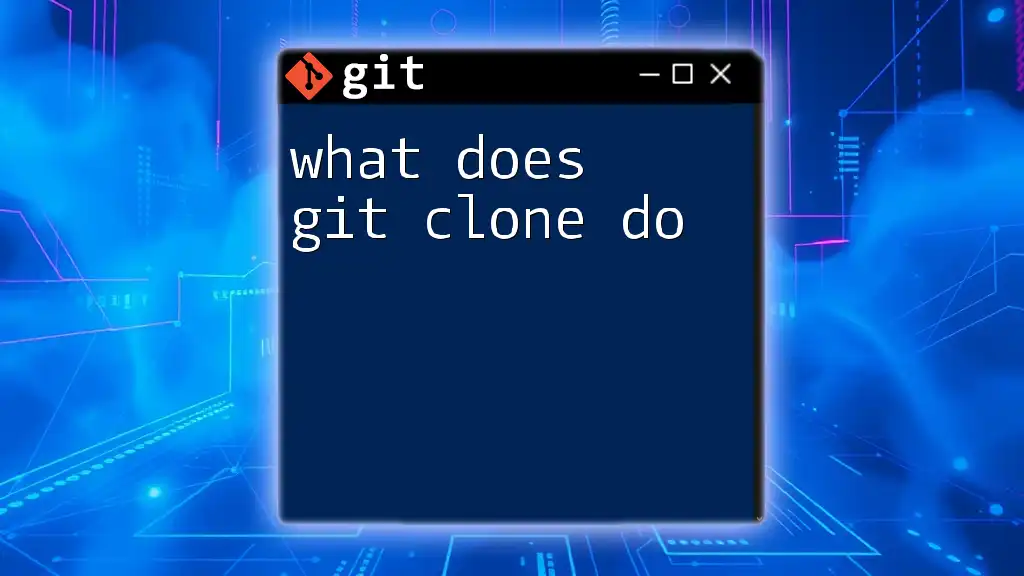
Potential Risks and Downsides of Git Rebase
Loss of Context
While rebasing has many advantages, one significant risk is the loss of context that can occur when altering commit history. Rewriting history may obscure the original sequence of changes, as rebasing can modify commit timestamps and authorship. This loss of context can lead to confusion when tracking down bugs or understanding the rationale behind specific changes.
Conflicts and Complexity
Another potential disadvantage of rebasing is that it can lead to more complicated conflicts, especially in large teams where many developers are making changes simultaneously. It's essential to be cautious and ensure that you fully understand the implications of rebasing before proceeding, particularly on shared branches.
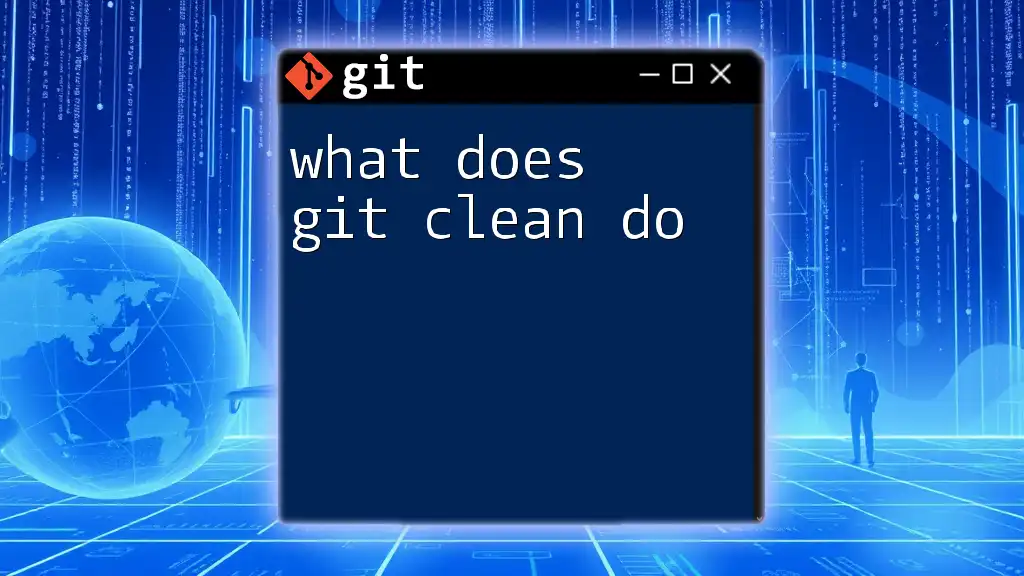
Best Practices for Using Git Rebase
When to Use Rebase
Rebasing is advantageous when you want a clean, linear project history—especially before merging a feature branch back into the main branch. It's ideal for organizing your commits to reflect logical changes without the noise that can come from merging. However, it's advisable to avoid rebasing commits that have already been pushed to a shared remote repository, as this can disrupt the work of others.
How to Safely Rebase
To successfully perform a rebase, follow these best practices:
-
Make sure you have backups: Always create a backup branch before starting a rebase. You can do this with:
git branch backup-feature-branch
-
Use `git reflog`: If something goes wrong during the rebase, you can use `git reflog` to find a previous commit and return to that state.
-
Perform rebases carefully: Always review the commits being rebased, and ensure you understand any changes before proceeding.
Concluding Thoughts on Rebase
In conclusion, understanding what Git rebase does is crucial for managing your Git workflows effectively. While rebasing can significantly enhance the cleanliness and clarity of your project history, it's essential to weigh the advantages against potential risks. Practicing rebase operations in a controlled environment will build your confidence and proficiency with this powerful tool.
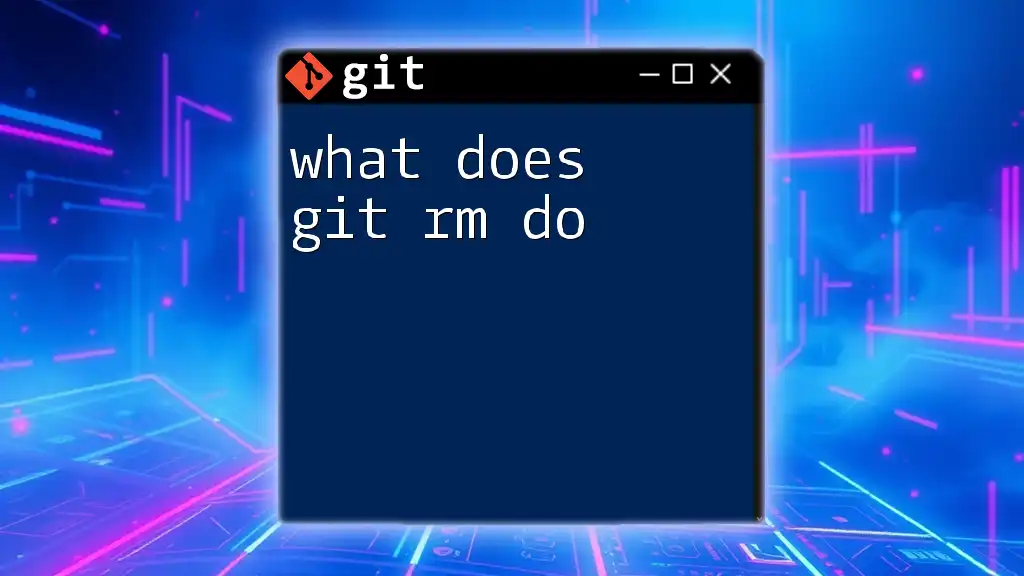
Conclusion
Recapping the key discussions surrounding Git rebase can forge a deeper understanding for developers looking to streamline their workflows. Experimenting with the various facets of rebasing and its impacts will empower programmers to make informed decisions in their collaborative environments.
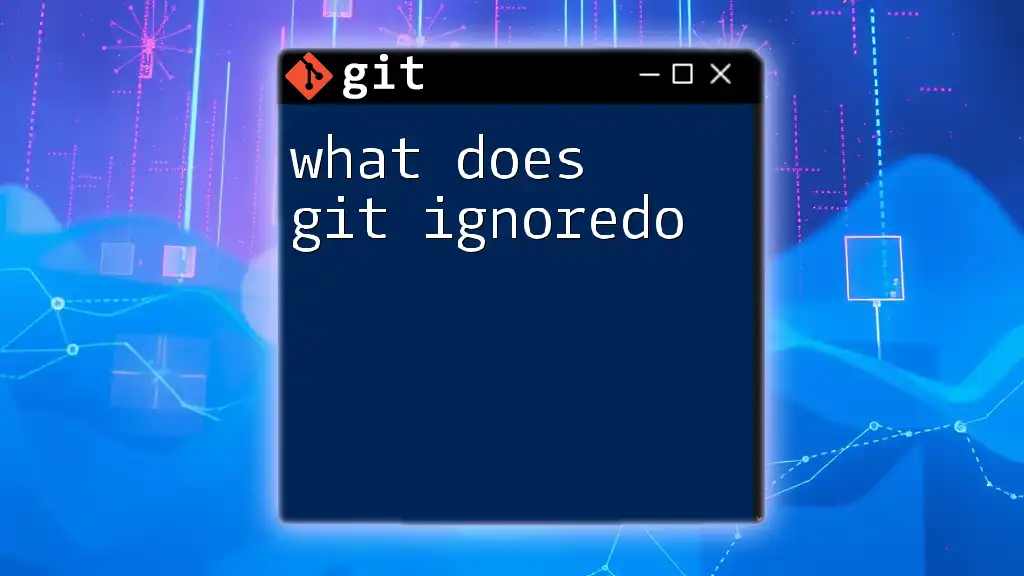
Additional Resources
For further reading and resources on Git rebase, consider checking the official Git documentation and reputable tutorials that provide exercises and explanations on various commands. These resources will enrich your understanding and mastery of Git, positioning you well for collaborative software development.