The `git pull` command fetches changes from a remote repository and merges them into your current branch, ensuring your local repository is up to date with the latest changes made by others.
git pull origin main
Understanding Version Control Systems
What is Version Control?
Version control systems (VCS) are tools that help developers manage changes to source code over time. They enable multiple contributors to work on a project simultaneously while keeping track of every modification. In an environment where collaboration is essential, understanding version control is foundational.
Differences Between Local and Remote Repositories
In Git, a local repository resides on your own machine, while a remote repository is hosted on the internet or a server. Commands like `git pull` facilitate synchronization between these two types of repositories, allowing developers to share their code changes seamlessly.
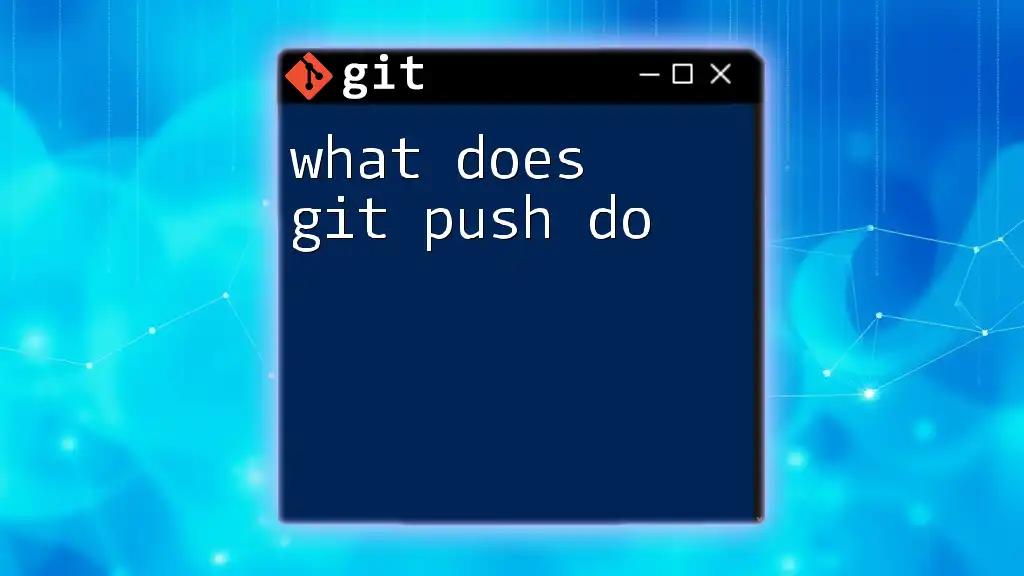
Introduction to the `git pull` Command
Defining `git pull`
The command `git pull` is essential in Git’s collaborative ecosystem. It combines two powerful commands, `git fetch` and `git merge`, to update your local repository with changes from a remote source. When you execute `git pull`, you’re essentially saying, “I’d like to retrieve new changes from the remote repository and integrate them into my current branch.”
Basic Syntax
The basic syntax for `git pull` is as follows:
git pull [options] [<repository> [<refspec>...]]
- `[options]`: Optional flags that modify the command behavior.
- `[<repository>]`: The name of the remote repository (usually `origin`).
- `[<refspec>]`: The specific branch or tag you wish to pull.
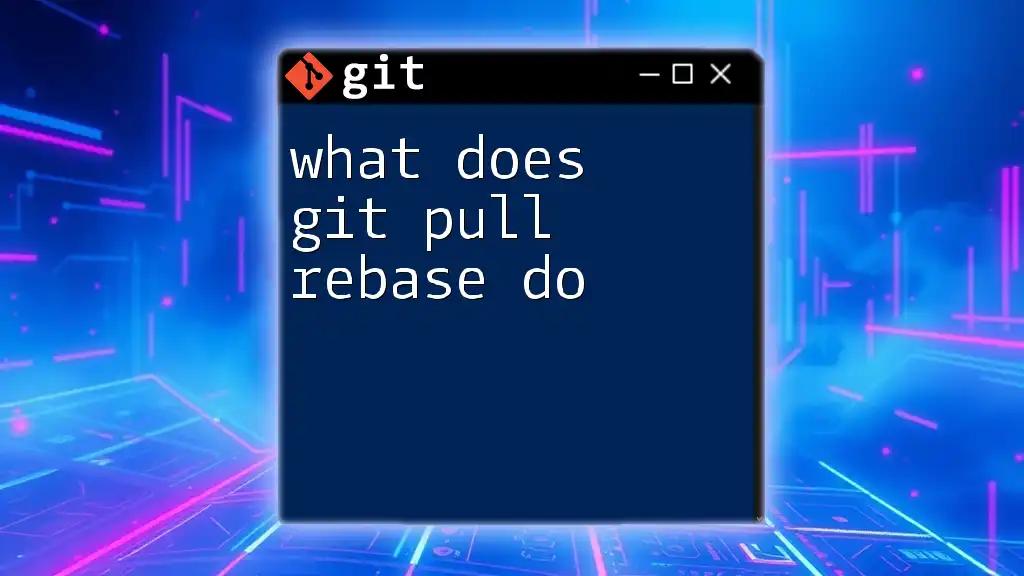
How `git pull` Works
Fetching Changes
When you run `git pull`, the first operation it performs is to fetch updates from the remote repository. This action downloads the data but doesn’t change your local files just yet.
To illustrate fetching changes explicitly, you might run the following command:
git fetch origin
This command retrieves updates from the `origin` remote but keeps your working directory unchanged until you decide to apply those changes.
Merging Changes
After fetching the updates, `git pull` automatically tries to merge these changes into your current working branch. This merging process means integrating any new commits from the remote repository with your local changes.
The actual merge happens when you run:
git merge origin/main
This command merges changes from the `main` branch of your remote repository into your current branch, allowing you to review and incorporate those updates.
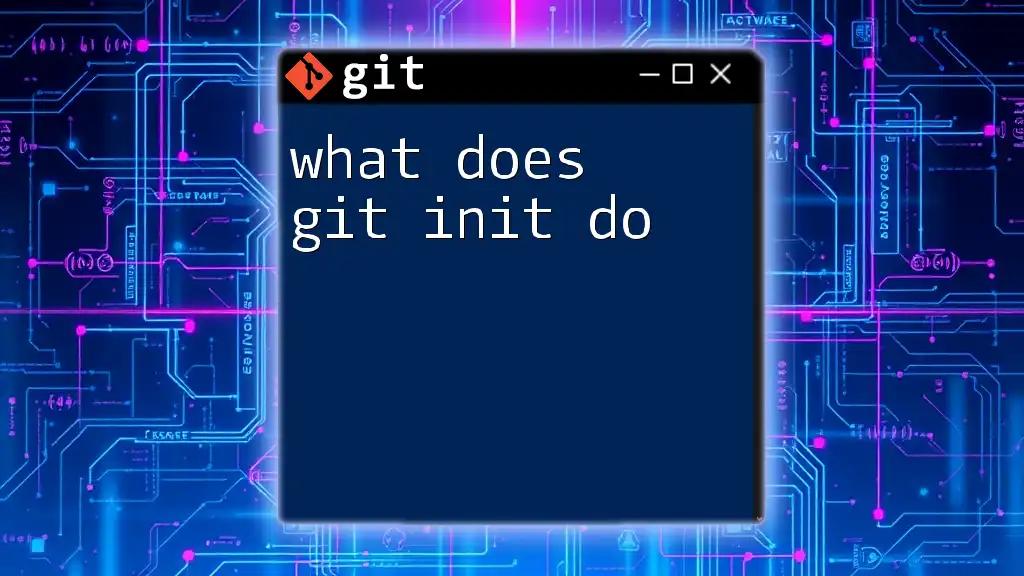
Practical Examples of Using `git pull`
Example 1: Basic Pull Command
To perform a straightforward update of your local branch based on its corresponding branch in the remote repository, you can invoke:
git pull
This command fetches and merges updates from the `origin` remote by default, making it a quick and efficient way to stay up-to-date.
Example 2: Pulling from a Specific Branch
In cases where you need to bring changes from a specific branch not tracked by your current working branch, you can specify that branch:
git pull origin feature-branch
This command pulls the changes from the `feature-branch` on `origin` and merges them into your current branch.
Example 3: Handling Merge Conflicts
Sometimes, conflicts may arise when merging changes, especially if both you and another contributor have modified the same lines of code. If this happens, Git won't be able to perform the merge automatically.
To resolve merge conflicts, you'll need to manually edit the conflicting files. After making adjustments, use:
git mergetool
This command helps you leverage graphical tools to resolve conflicts more efficiently.
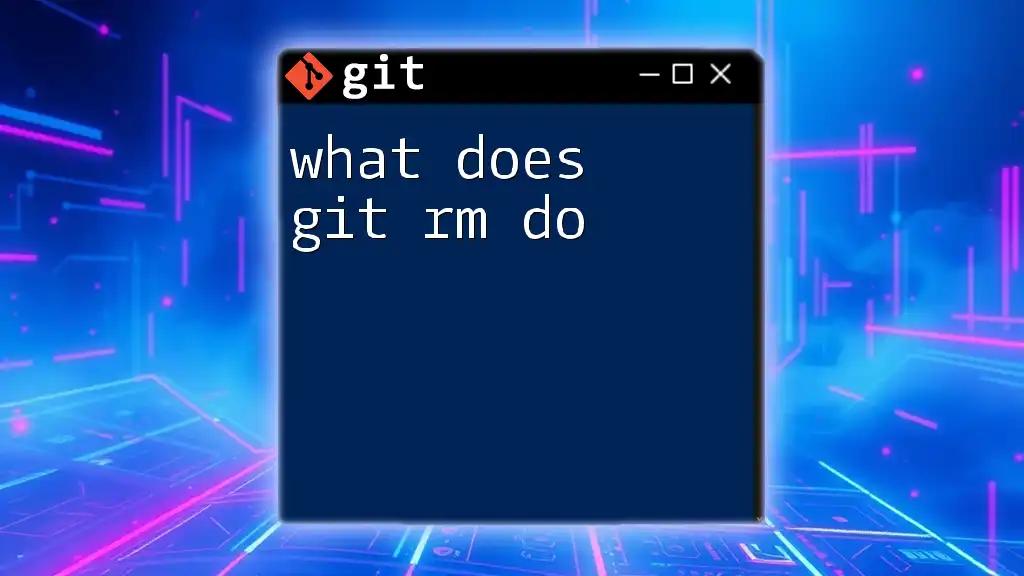
Advanced Tips for Using `git pull`
Using Pull with Rebase
Rebasing is a technique that allows you to integrate changes from one branch into another more smoothly. If you wish to pull changes while keeping a cleaner commit history, you can use the rebase option:
git pull --rebase
This approach avoids unnecessary merge commits by applying your local commits on top of the newly fetched commits.
Using Pull Options
Various options enhance the functionality of `git pull`. For example:
- `--ff` (fast-forward): This option allows Git to fast-forward to the latest commit if there are no conflicting changes in your local branch.
- `--no-commit`: This option prevents Git from creating an automatic commit after the merge, allowing you to make further adjustments before committing.
Here’s how you might use one of these options:
git pull --ff
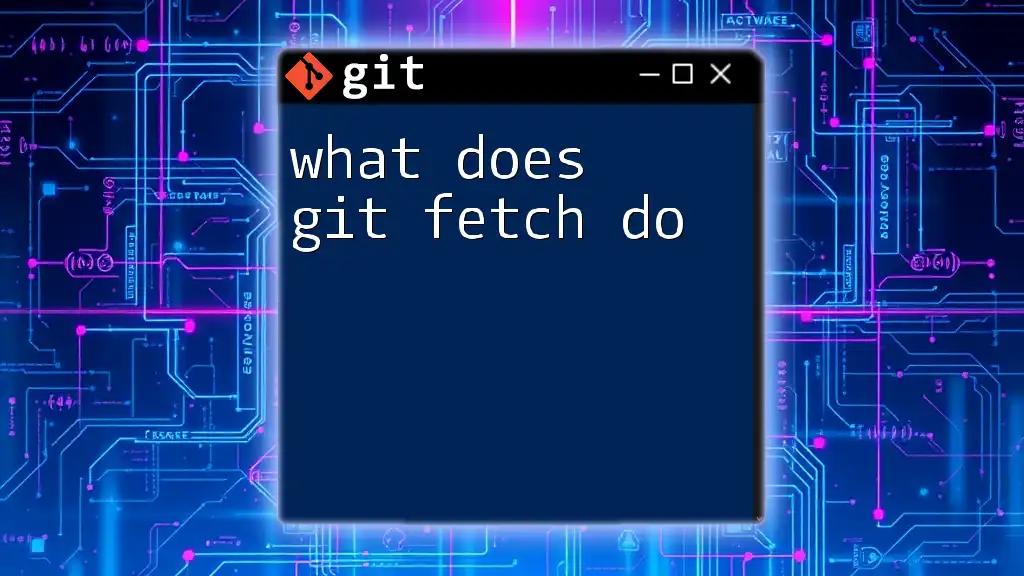
Best Practices for Using `git pull`
When to Use `git pull`
Using `git pull` is ideal when you’re ready to synchronize your local branch with the latest changes from your team. It’s essential to do this regularly, especially before starting new features or bug fixes to ensure you’re working with the most current codebase.
What to Avoid
Before executing `git pull`, ensure that you’ve committed or stashed your own changes. Pulling without confirming your local status can lead to potential conflicts or unintended overwrites of your work. Always be cautious and check your branch’s state with:
git status
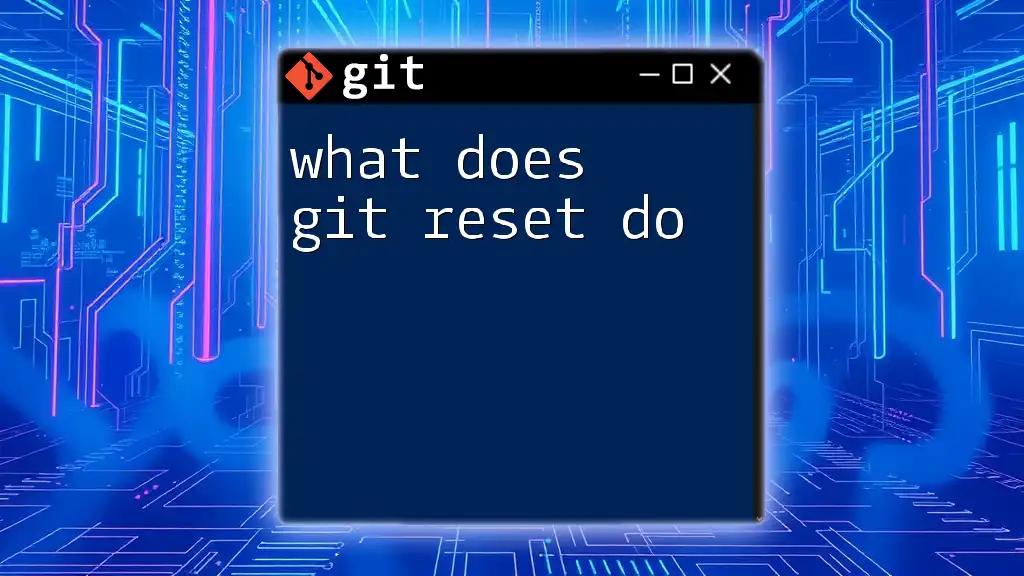
Conclusion
In summary, understanding what `git pull` does is crucial for effective collaboration and code management in Git. By mastering this command, you empower yourself to maintain synchronization with remote repositories, resolve conflicts, and integrate changes seamlessly. Regular practice and adherence to best practices will help you leverage `git pull` confidently in collaborative projects.
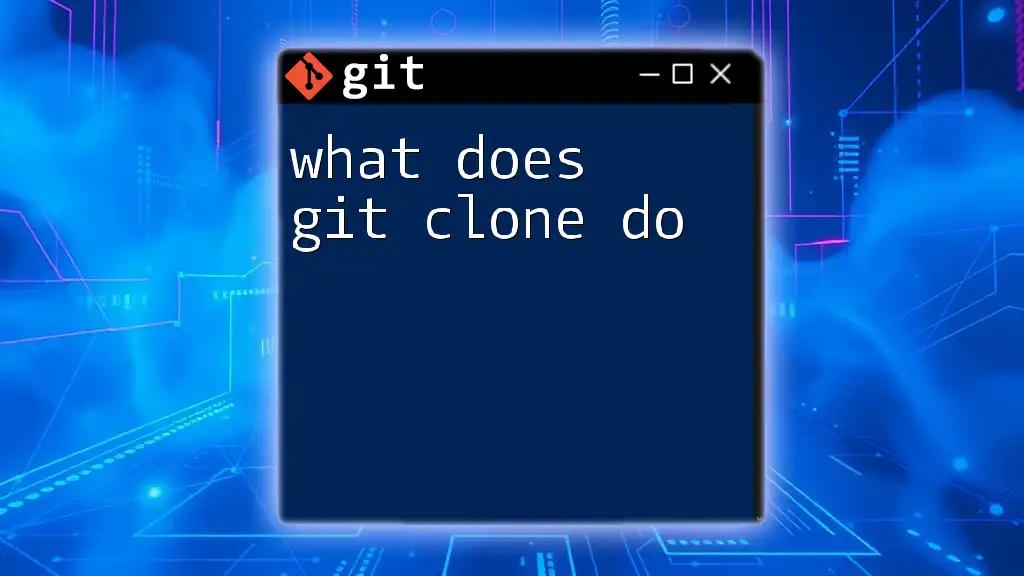
Additional Resources
For further reading, consider referring to the official Git documentation and exploring dedicated tutorials available online. These resources can greatly enhance your understanding and efficiency with Git.
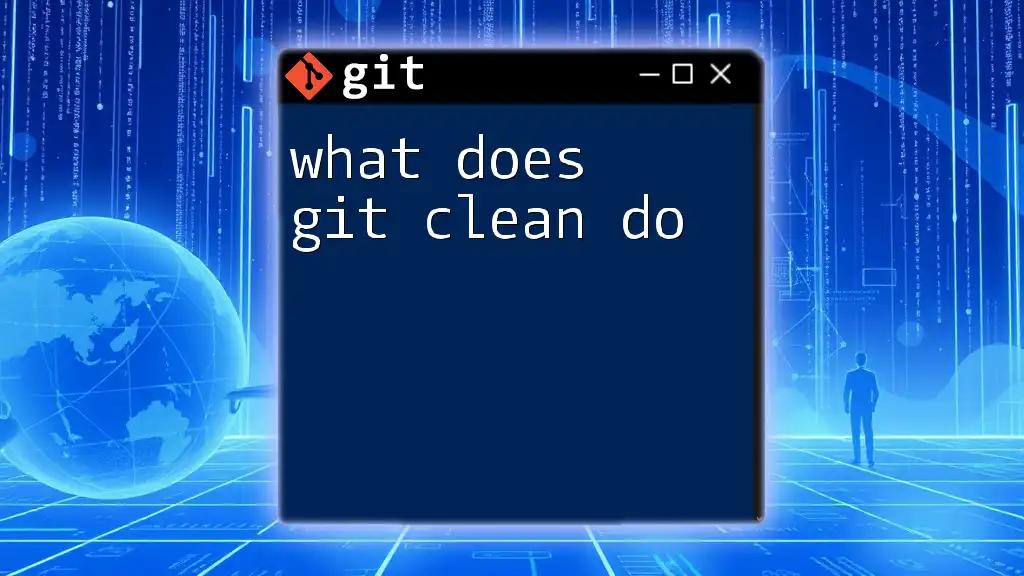
Call to Action
Feel free to leave any questions or comments below and share this article. Don’t forget to subscribe for more concise Git tips and tricks to help you on your coding journey!