Git is a distributed version control system that allows multiple developers to work on a project simultaneously while tracking changes, managing versions, and coordinating collaboration.
Here's an example of a basic Git command to initialize a new repository:
git init
What is Git?
Git is a distributed version control system designed to manage the development of source code across multiple files and collaborators. Unlike centralized version control systems (like SVN), Git allows each user to have a complete copy of the repository, including its history, which facilitates better collaboration and independence.
Key Features of Git
-
Distributed Architecture: Every user working with Git has a full copy of the repository on their local machine. This means that operations such as committing changes, viewing history, and creating branches can be done without needing access to a central server.
-
Efficiency: Git is built for speed. Most operations are performed locally, which makes them extraordinarily fast. For instance, committing changes and branching operations are nearly instantaneous regardless of the project's size.
-
Data Integrity: Git uses checksums (SHA-1 hashing) to ensure the integrity of data. Every change is tracked and identified by a unique hash, which helps in maintaining the history of the project and preventing data corruption.
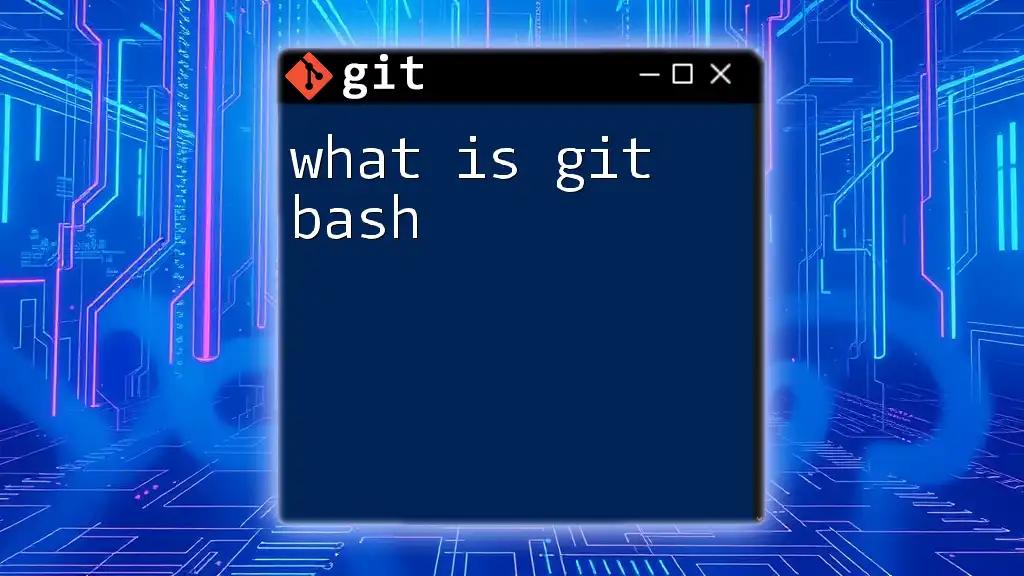
Why Use Git?
Collaboration Made Easy
One of the primary advantages of Git is its ability to facilitate collaboration. Multiple team members can contribute to the same project without conflicts by working on different branches and later merging their changes. This approach encourages experimentation while keeping the main codebase stable.
Example: Imagine a team of developers working on a feature. Each team member can create their branch to develop different aspects of the feature independently. Later, they can merge their branches back into the main branch.
Version Tracking
Git excels at keeping track of changes made to files over time. It allows you to revert to a previous state, view the history of a project, and even branch off from specific points in history.
Code Snippet: You can see the complete commit history of your project by running:
git log
This command provides a chronological list of all commits, showing the author, date, and commit message.
Branching and Merging
Branching is one of the most powerful features of Git. It allows developers to create isolated environments for new features or experiments without impacting the main codebase.
Code Snippet: You can create a new branch with:
git branch new-feature
Switching to this new branch is done with:
git checkout new-feature
Merging these changes back into the main branch can be as simple as:
git merge new-feature
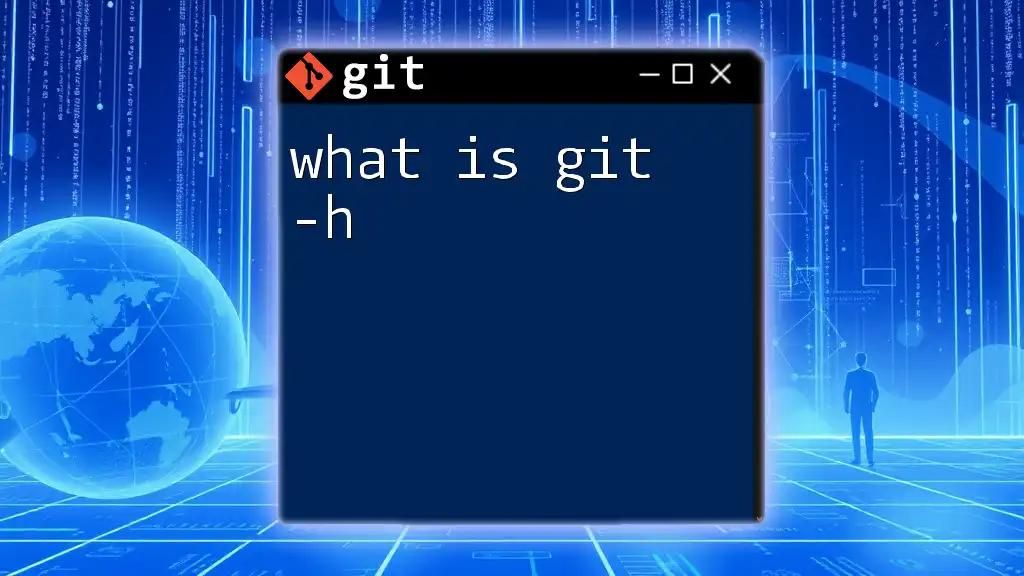
Basic Git Commands
Setting Up Git
Installation
To get started with Git, you first need to install it on your machine. Git can be installed on various platforms:
- Windows: Download the Git installer from the official Git website and run it.
- Mac: You can install Git using Homebrew by executing:
brew install git
- Linux: Use your package manager to install Git. For example, on Ubuntu, you can run:
sudo apt-get install git
Configuration
After installing Git, it's important to configure it by setting your username and email address. This information will be attached to your commits, helping you and others understand who made changes.
Code Snippet: Set your username and email with these commands:
git config --global user.name "Your Name"
git config --global user.email "your_email@example.com"
Essential Commands
Cloning a Repository
To start working on an existing project, use the `git clone` command to make a copy of the repository on your machine.
Code Snippet: You can clone a repository with:
git clone https://github.com/username/repository.git
This command creates a local copy of the repository, allowing you to work on it without needing a constant internet connection.
Checking Status
The `git status` command provides insights into the state of your working directory and staging area. It shows you which files are staged for the next commit, which are modified, and which files aren't being tracked.
Code Snippet: To check the status, simply run:
git status
Adding Changes
To prepare your changes for committing, you must stage them using the `git add` command. This means you're telling Git which changes you want to include in your next commit.
Code Snippet: You can stage all changes with:
git add .
This command adds all modified and new files in the current directory to the staging area.
Committing Changes
Once your changes are staged, you can commit them to your local repository. A commit records changes with a message describing what was done, which is essential for understanding a project's history.
Code Snippet: To commit your changes, run:
git commit -m "Your commit message"
Make sure your commit messages are descriptive for better traceability.
Pushing Changes
After committing your changes locally, you often want to share them with others by pushing them to a remote repository. The `git push` command sends your committed changes to the specified remote branch.
Code Snippet: To push changes to the main branch, use:
git push origin main
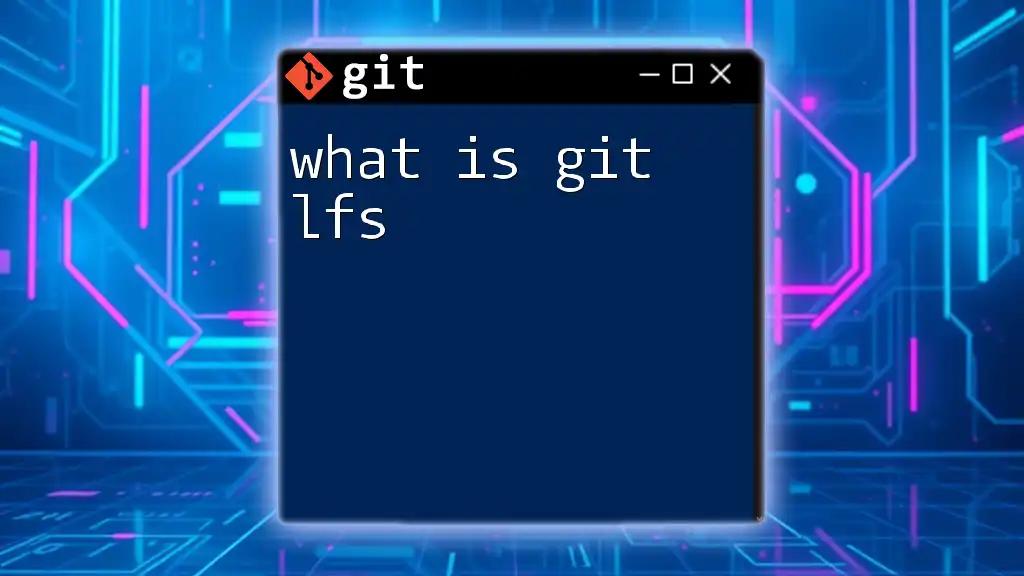
Advanced Features of Git
Branching
Creating branches in Git allows developers to work on new features, fixes, or experiments without affecting the stable code. Branches can be created, switched, and deleted easily.
Code Snippet: To create a branch:
git branch feature-x
You can then switch to your new branch with:
git checkout feature-x
Merging and Rebasing
Merging combines the changes from one branch into another, while rebasing moves or "replays" changes from one branch onto another.
Code Snippet: To merge a branch into your current branch:
git merge feature-x
Rebasing can be done with:
git rebase feature-x
Conflict Resolution
Merge conflicts can arise when changes in both branches collide. Git will notify you of the conflict, and you can manually resolve these in your code editor. Once resolved, you can continue the merge or rebase process.
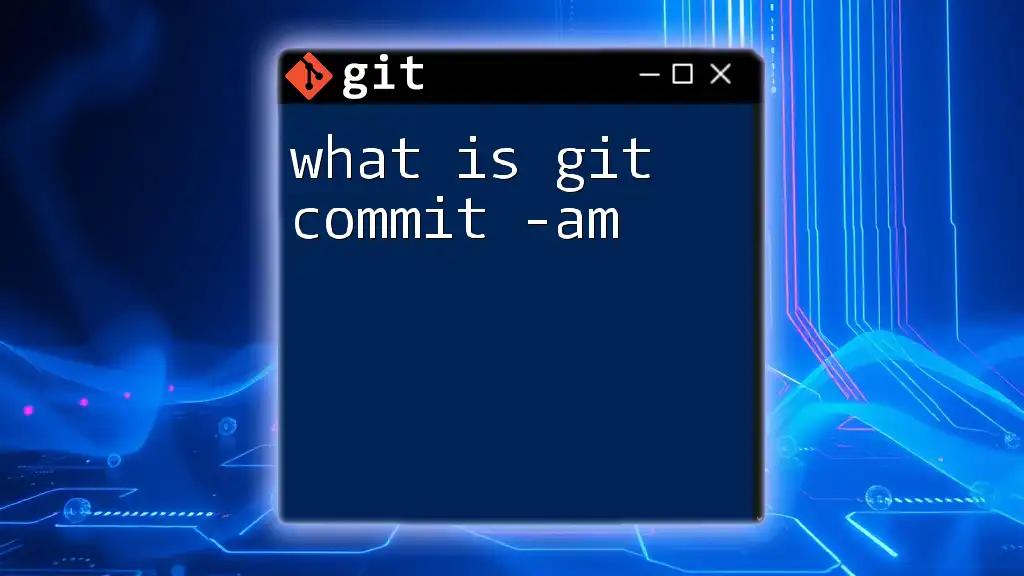
Common Use Cases of Git
Open Source Collaboration
Git popularized collaboration in open-source projects. It allows anyone to contribute by forking repositories, making changes, and submitting pull requests to share their contributions.
Continuous Integration and Deployment
Git integrates seamlessly with CI/CD tools. These tools automate testing and deployment workflows, ensuring that only tested code reaches production and enabling rapid development cycles.
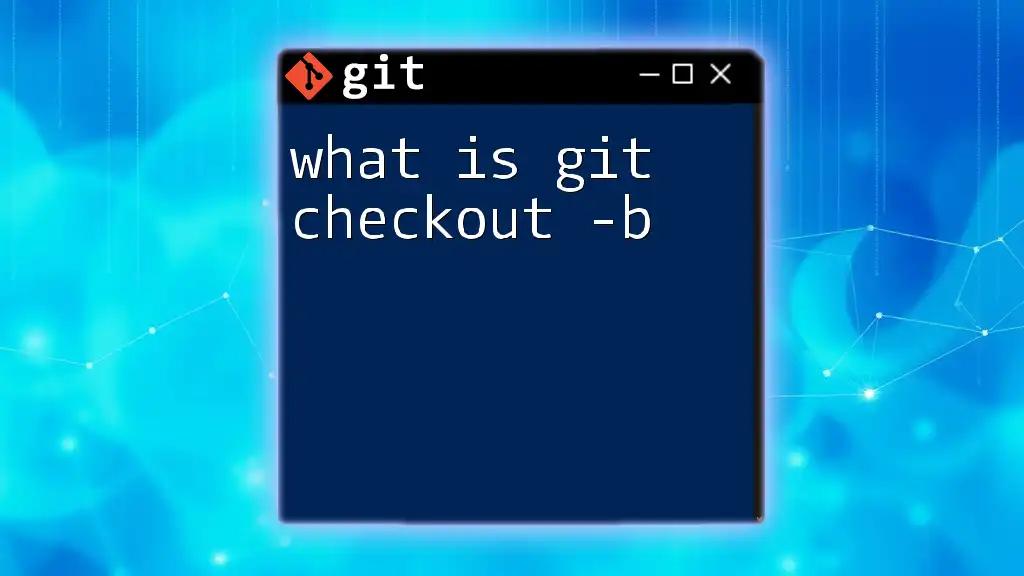
Conclusion
In summary, understanding what Git is and how it works provides a vital skill for modern software development. With its robust version control features, collaboration capabilities, and speed, Git is an essential tool for both individuals and teams. As you become more familiar with basic commands, you'll find that delving into more advanced features of Git opens even greater possibilities for collaboration and project management.
By practicing these commands and exploring Git's full range of capabilities, you can enhance your development workflow and ensure that your projects remain organized and collaborative. Explore our additional resources to deepen your knowledge and mastering Git.