To delete a file from a Git repository, use the `git rm` command followed by the file name, and then commit the changes to reflect the deletion in the repository.
Here’s the command in markdown format:
git rm <file-name>
git commit -m "Remove <file-name>"
Understanding Git and Its File Management
What is a Git Repository?
A Git repository is essentially a directory that contains your project files, along with a hidden `.git` folder that holds all the metadata, history, and version control information. This structure allows you to keep track of changes made to your files over time, making it easier to collaborate and manage your project's evolution efficiently.
The Nature of Files in Git
In Git, files can exist in three primary states: modified, staged, and committed. Understanding these states is crucial for file management, including the process of deletion. When you make changes to a file, it is marked as modified. Once you stage these changes using `git add`, the file is prepared for committing. Finally, a committed file is safe and recorded in your repository’s history.
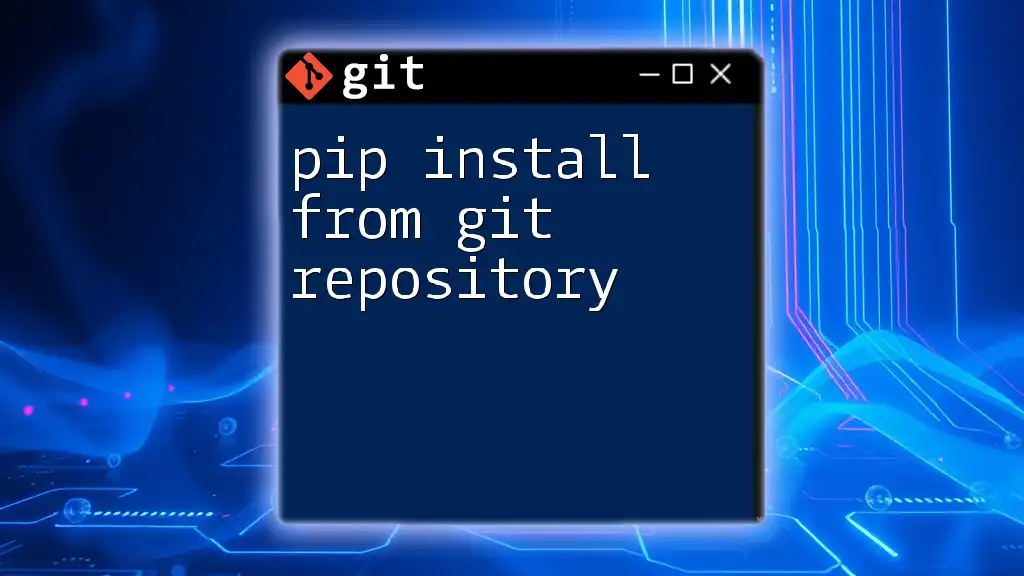
Types of File Deletion in Git
Working Directory vs. Staging Area
Before we dive into the deletion commands, it's important to recognize the difference between the working directory and the staging area. The working directory contains files and folders that you are actively working on, whereas the staging area holds files that are ready to be committed. Understanding these distinctions will help you manage file deletions effectively and anticipate the implications of your actions.
Permanent Deletion vs. Logical Deletion
When you delete a file in Git, you can either permanently remove it from the repository's history or just stop tracking it (logical deletion). Permanent deletion implies that the file will be removed from the repository, and it won’t be accessible unless you use commands to recover it. In contrast, logical deletion allows the file to remain in your local directory while no longer being tracked by Git.
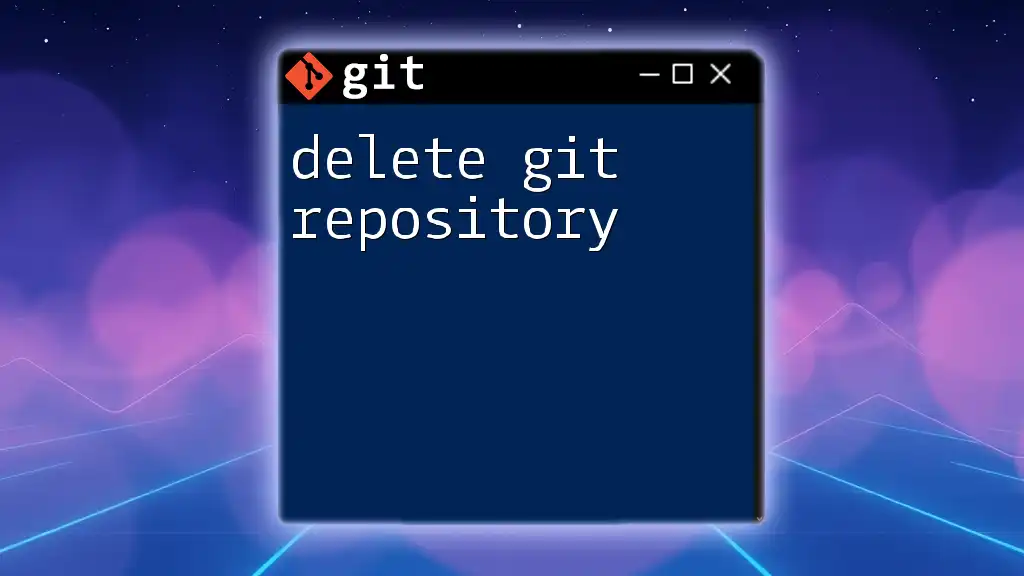
How to Delete a File from a Git Repository
Deleting a File from the Working Directory
To remove a file entirely from your repository, you can use the `git rm` command. This command will delete the file from both your working directory and the staging area, preparing the deletion for the next commit.
git rm <filename>
For example, if you need to delete a file named `old_version.txt`, run the following command:
git rm old_version.txt
After this command, the file is gone from your working directory, and Git has staged this deletion for the next commit.
Deleting a File While Keeping It Locally
If you want to untrack a file but keep it on your local machine, you can use the `--cached` option with `git rm`. This removes the file from the staging area without deleting it from your filesystem.
git rm --cached <filename>
Suppose you have a sensitive configuration file, such as `config.json`, that you do not want to be tracked by Git but need for local development. You would execute:
git rm --cached config.json
Deleting Files from the Staging Area Only
If you accidentally staged a file for deletion and want to restore it back to the staging area, you can use the `git restore` command.
git restore --staged <filename>
For instance, if you mistakenly staged `old_version.txt` for deletion, run:
git restore --staged old_version.txt
This command will revert the staged deletion, allowing you to continue working on the file as usual.
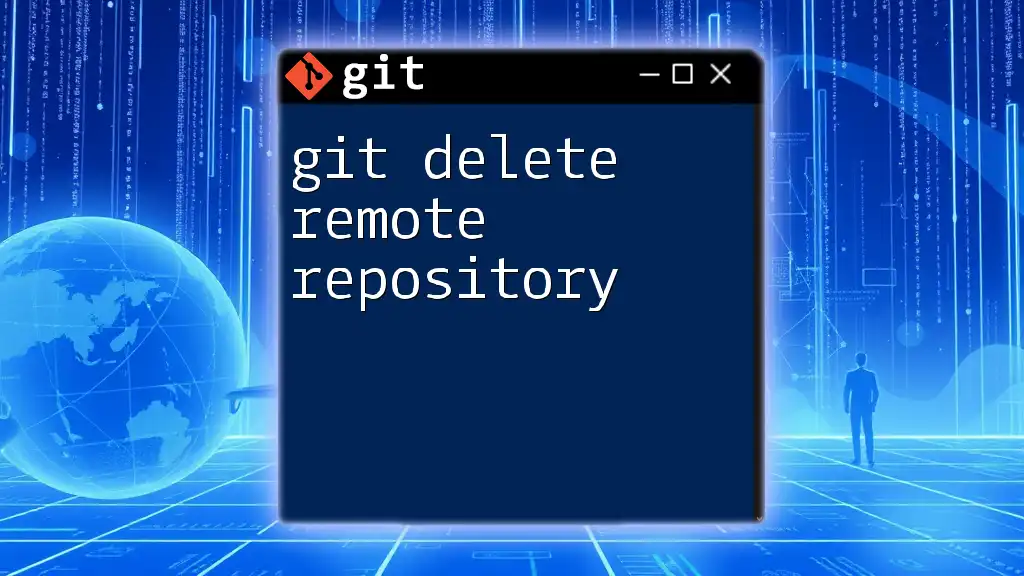
Committing Changes After Deletion
Once you've deleted your files using one of the methods above, it's essential to commit those changes to finalize the deletion process. You can do this with the `git commit` command.
git commit -m "Deleted file <filename>"
Following the deletion of `old_version.txt`, you would run:
git commit -m "Deleted file old_version.txt"
This step records the deletion in your Git history, ensuring that your project remains clean and organized.
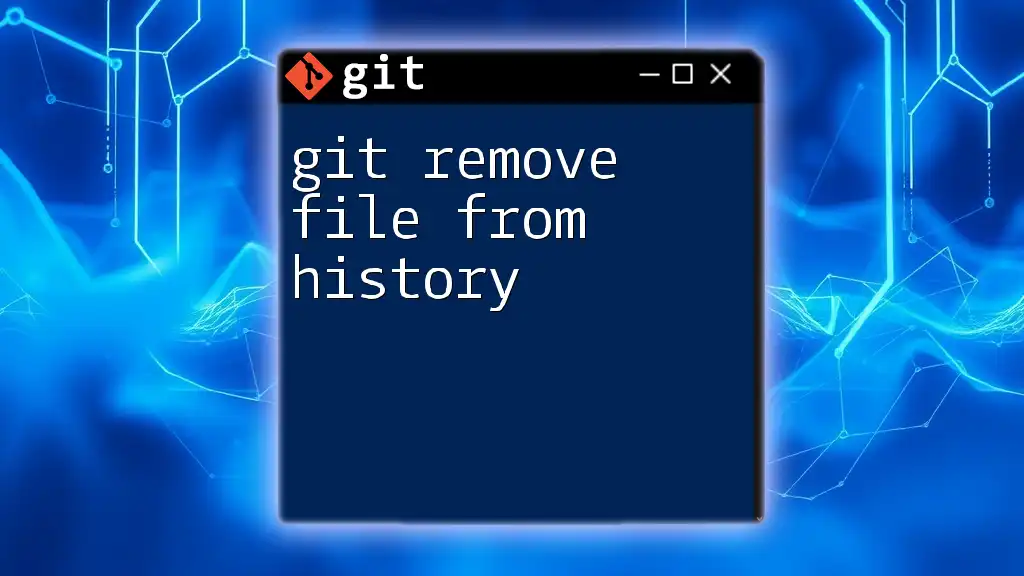
Handling Deleted Files in Git History
Recovering Deleted Files
If you need to recover a deleted file, don't worry! Git allows you to restore files from previous commits. You can use the `git checkout` command for this purpose.
git checkout <commit-hash> -- <filename>
To find the specific commit hash, you can run `git log` to view your history. Once you've noted the correct commit hash, you can replace `<commit-hash>` in the above command with it.
Best Practices in File Deletion
When deleting files from your Git repository, it's best to be cautious. Always double-check which files you are removing and consider the implications for your team and project. Maintain clear and descriptive commit messages, as these will help keep your project's history clean and easy to understand.
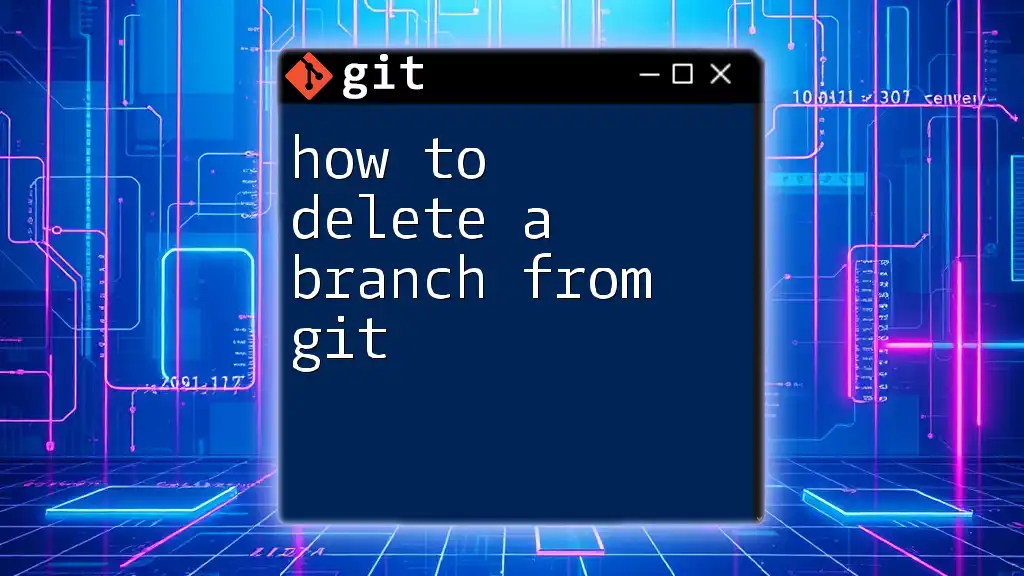
Troubleshooting Common Issues
File Not Found Errors
If you encounter file not found errors when attempting to delete or restore files, ensure that you are in the correct branch and that the file name is spelled correctly, including its case. Git is case-sensitive, so inadvertent capitalization differences can lead to confusion.
Untracked Files
Sometimes, you may face issues with untracked files. These are files that Git is aware of but are not being tracked. You can check the status of your files using:
git status
This command highlights which files are staged, modified, and untracked, enabling you to manage your deletions accurately.
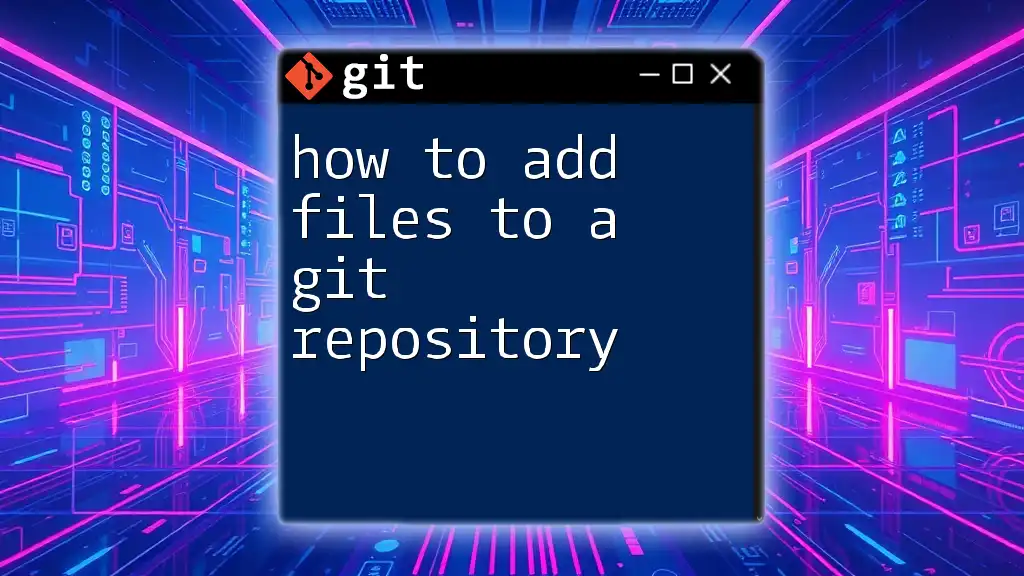
Conclusion
In summary, mastering how to delete a file from a Git repository involves understanding the methods available for file management. By utilizing commands like `git rm`, `git rm --cached`, and `git restore`, you can effectively manage your project's files. Remember to commit your changes afterward to maintain a clean history. Practice these commands in your own Git projects to become proficient, ensuring a seamless workflow as you navigate your version-controlled projects.
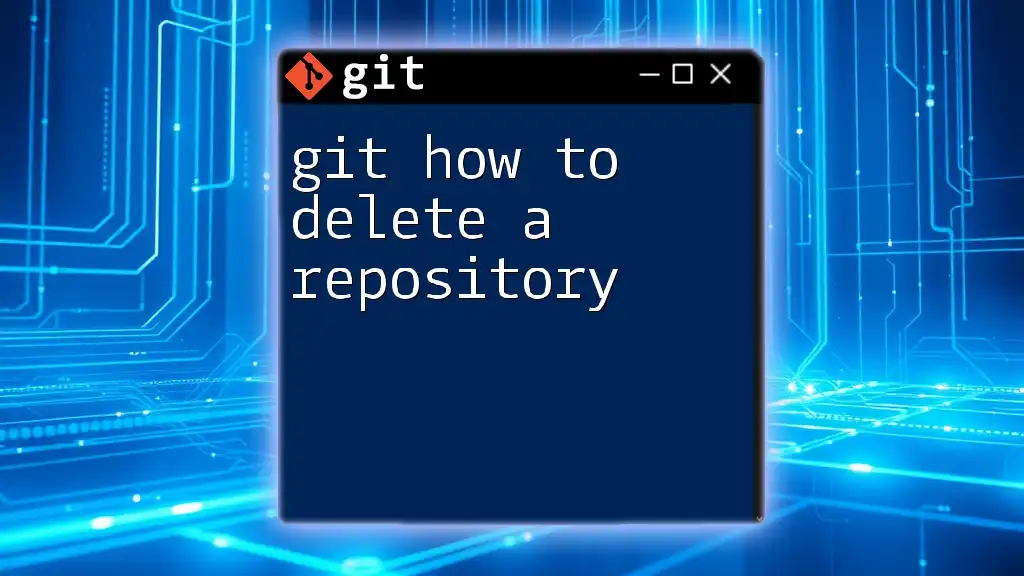
Additional Resources
For further insights into Git, you can explore the official Git documentation and additional tutorials that delve deeper into version control strategies. Engaging with these resources can enhance your skills, enabling you to become a more competent Git user.