To remove a file from the Git history, you can use the `git filter-branch` command to rewrite the history and remove the file from all commits.
git filter-branch --force --index-filter "git rm --cached --ignore-unmatch path/to/your/file" --prune-empty --tag-name-filter cat -- --all
Understanding Git History
What is Git History?
Git history is a record of all the changes made in a Git repository. Each change is associated with a commit, which acts as a snapshot of your project at a specific point in time. This history is crucial for tracking progress, identifying when changes were made, and collaborating with others.
How Git Stores Data
Git does not save data as a series of differences between versions; it maintains snapshots of your entire file system each time you make a commit. This means that if a file changes, Git records the new version as a new snapshot instead of just noting what has changed. Each commit includes a reference to the previous commit, forming a chain of history.
The Implications of Altering History
Altering Git history is a significant operation; it can disrupt collaborative efforts if not handled with care. When history is rewritten, collaborators will have to reconcile their local histories with the new one, potentially leading to confusion and lost work. Always proceed with caution when planning to `git remove file from history`.
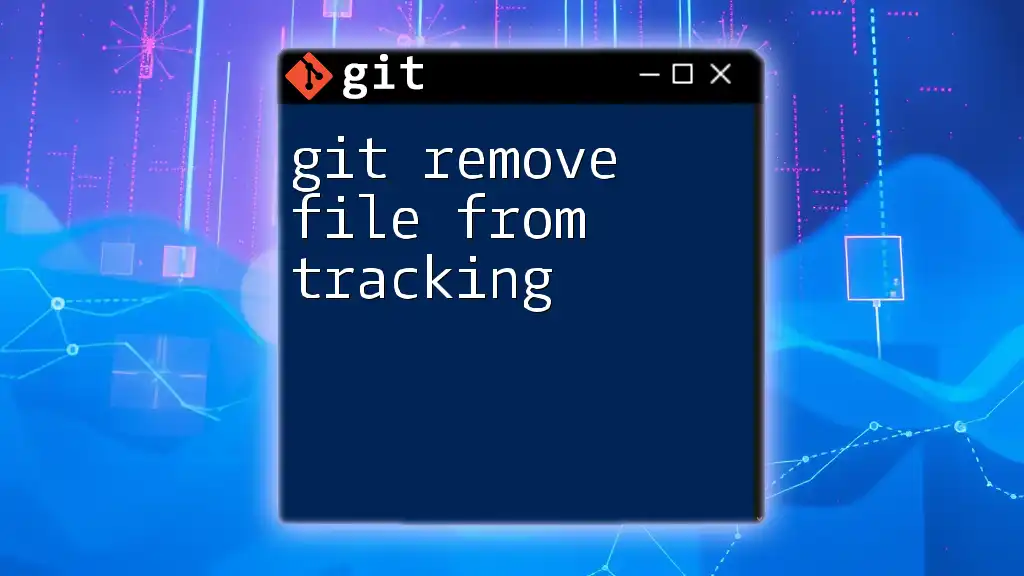
Methods to Remove a File from Git History
Using `git filter-branch`
What is `git filter-branch`?
`git filter-branch` is a powerful command that allows you to rewrite Git history. You can use it to make extensive changes across a series of commits, including removing files from earlier commits.
Removing a File from All Commits
To remove a file from all commits, you can use the following command:
git filter-branch --index-filter 'git rm --cached --ignore-unmatch <file>' HEAD
In this command:
- `--index-filter` allows you to specify how the index (staging area) should be modified for each commit.
- `git rm --cached --ignore-unmatch <file>` tells Git to remove the specified file from the index (i.e., from the repository) without deleting it from your working directory.
Force Pushing Changes
After executing the filter-branch command, the branch will have a new history. You must push these changes to the remote repository using:
git push origin --force
Important: Using `--force` can disrupt the history for anyone else working on the repository. Ensure you communicate with your team before proceeding.
Caveats and Best Practices
- Always back up your data before using `git filter-branch`.
- Consider tagging important commits for future reference.
- Be ready to assist collaborators in reconciling their local repositories.
Using `git rebase`
What is `git rebase`?
`git rebase` is another way to rewrite Git history. It allows you to modify a series of commits, including rearranging, editing, or removing them.
Interacting with Commits
With `git rebase`, you can enter an interactive mode that allows you to remove a file from specific commits.
Step-by-Step Example
To start an interactive rebase of the last `n` commits, use:
git rebase -i HEAD~n
Replace `n` with the number of commits you're targeting. This command opens a text editor where you can choose commits to modify.
For each commit, change `pick` to `edit` for those commits from which you want to remove the file. For example:
edit <commit_hash> old commit message
pick <commit_hash> next commit message
After editing, Git will pause the rebase process at each specified commit, allowing you to remove the file:
git rm --cached <file>
git commit --amend
After you've repeated this for all relevant commits, use:
git rebase --continue
to complete the process.
Finalizing Changes
Once you finish the rebase, push your changes with:
git push --force
Always communicate with your team, as they will also need to sync their repositories due to rewritten history.
Using BFG Repo-Cleaner
What is BFG Repo-Cleaner?
BFG Repo-Cleaner is a user-friendly and faster alternative to `git filter-branch`, specifically designed for bulk removal tasks in Git repositories.
Installation and Setup
First, download the BFG Repo-Cleaner from its [official site](https://rtyley.github.io/bfg-repo-cleaner/). Following the instructions for your operating system will set you up.
Example Command to Remove a File
To remove a specific file from your Git history, execute:
bfg --delete-files <file>
This command scans through the repository and removes all instances of the specified file from history, significantly speeding up the process compared to traditional commands.
Post-Cleaning Steps
After running BFG, it's essential to clean your repository with:
git reflog expire --expire=now --all
git gc --prune=now --aggressive
These commands help ensure that all references to the file have been removed, allowing for a clean slate in your repository.
Manual Removal of Sensitive Files (If Applicable)
When Manual Cleanup May Be Best
In certain scenarios, automated tools may not suit your needs, particularly if you have complex or sensitive data. Manual cleanup allows for tailored solutions based on your specific situation.
Steps for Manual Removal
- Identify Files and Commits: Use `git log` to find commits affecting the file.
- Crafting Commits: For each commit containing the file, you may want to cherry-pick or create new commits that do not include the sensitive data.
- Pushing Updates: Finally, ensure to `push --force` for these changes.
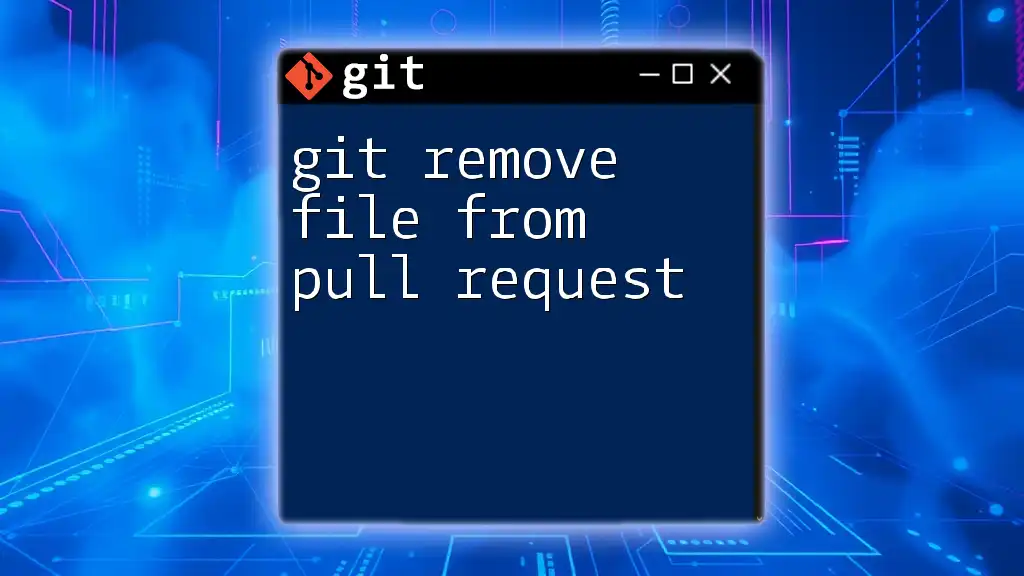
Verifying Removal
How to Check History
To verify that a file has been removed completely from the history, you can use:
git log -- <file>
If the file appears in the history, it hasn't been fully removed. You can also use `git diff` to compare commits before and after the operation.
Confirming Success
After verification, ensure you check with your collaborators that they are aware of the changes and how to adjust their local repositories accordingly.
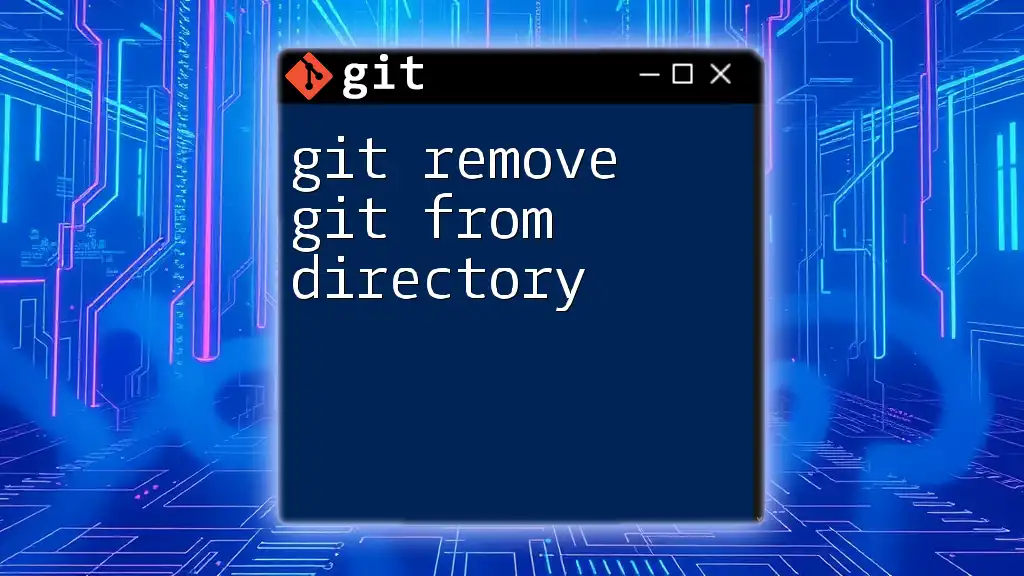
Additional Considerations and Best Practices
Backup Before Changing History
Always create a backup branch before making significant changes. You can do this by:
git checkout -b backup-branch
This safety net allows you to revert to the original state if anything goes wrong.
Communication with Collaborators
Open communication is paramount when modifying history. Inform your team about the changes, and explain how they need to adjust their local repositories to align with the new history.
Using Tags for Important Releases
Tags can help maintain crucial checkpoints in your project history. If you anticipate frequent changes or have essential releases, consider tagging them to simplify rollback processes.
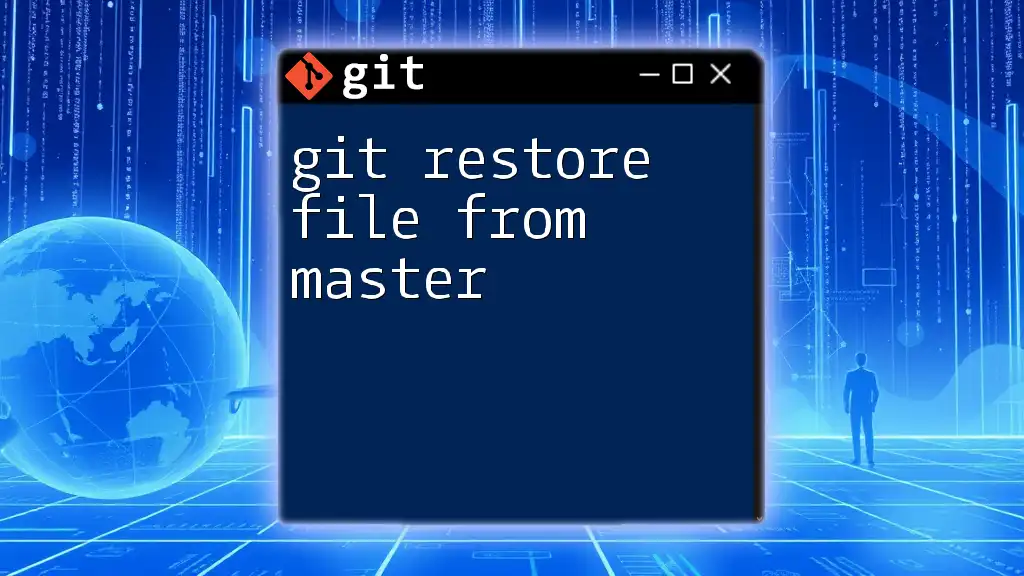
Conclusion
Removing a file from Git history is a nuanced task that requires consideration and care. Understanding the implications of altering commit histories is vital for maintaining collaboration and data integrity. Whether you opt for `git filter-branch`, `git rebase`, or BFG Repo-Cleaner, always ensure you back up your repository and communicate with your team. By following these best practices, you can manage sensitive data and streamline your Git workflow effectively.
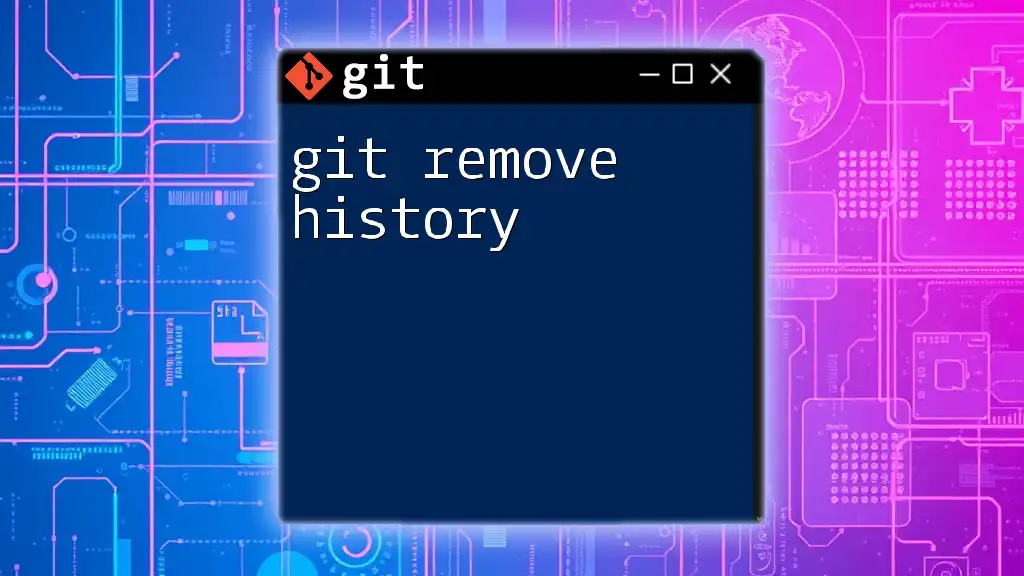
Additional Resources
For further exploration of Git commands and practices, consider consulting the official Git documentation, community forums, and reputable books focused on version control. These resources provide in-depth knowledge, helping you continue your journey in mastering Git.