To remove a file from a pull request while retaining it in your local repository, use the `git reset` command followed by the file name and then commit the change.
git reset HEAD^ -- <file_name>
git commit -m "Remove <file_name> from pull request"
Understanding Pull Requests
What is a Pull Request?
A pull request (PR) is a feature offered by platforms like GitHub, GitLab, and Bitbucket that allows developers to propose changes to a repository. It serves as a request for these changes to be reviewed by other contributors before merging them into the main codebase. PRs facilitate collaboration, enabling code review, discussions, and suggestions, making them essential in team projects.
Why You Might Need to Remove a File
There are several situations where removing a file from a PR becomes necessary. You might have mistakenly staged a file that should not be part of your changes, or perhaps you've included a file that holds sensitive data, such as API keys or passwords. Keeping unwanted files in a PR can clutter the review process, cause confusion, or expose vulnerabilities if merged. Thus, it's critical to address these issues promptly.
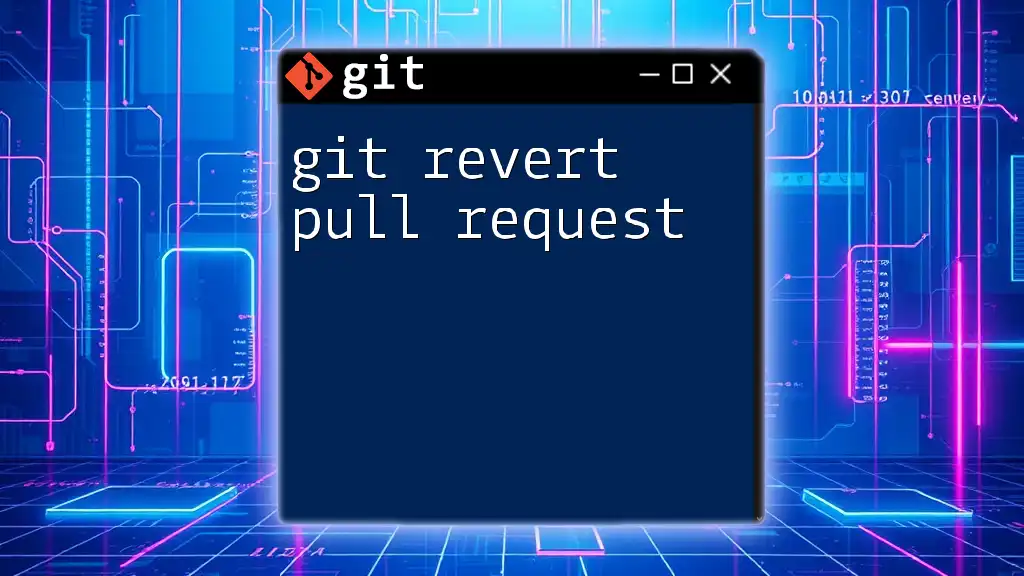
Preparing the Environment
Confirming Your Repository
Before making any changes, confirm that you're in the correct directory of your Git repository. This can be easily done using:
cd /path/to/your/repository
Once you're in the right directory, check your current branch status with:
git status
This command informs you of any changes, uncommitted files, and your current branch, ensuring that you're ready to work on the right context.
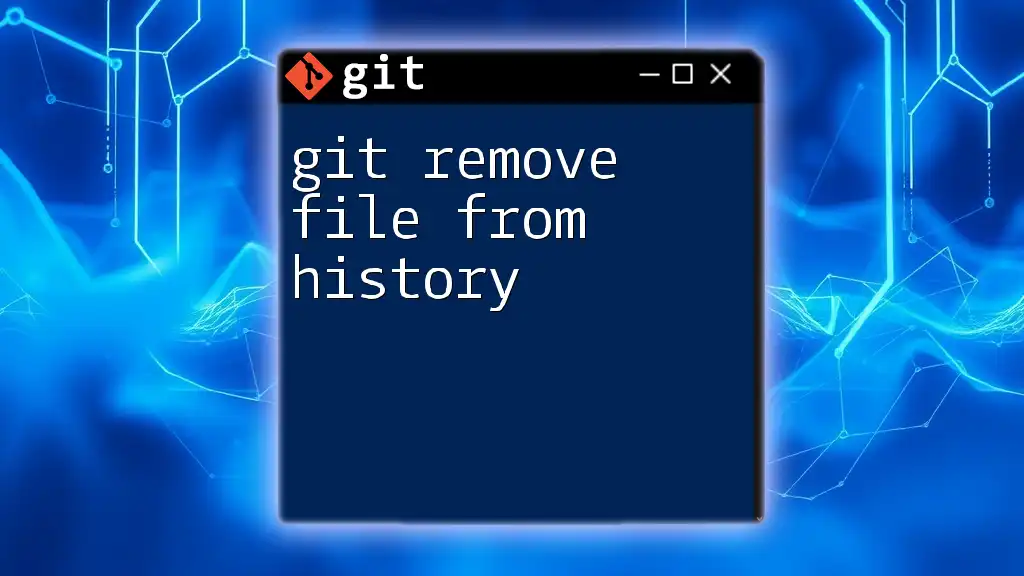
Methods to Remove a File from a Pull Request
Method 1: Using git reset
What is git reset?
The `git reset` command is used to remove files from the staging area without deleting them from your working directory. This is particularly useful if you want to unstage a file before committing your changes.
Step-by-Step Guide to Remove a File
-
Identify the file you want to remove: Locate the specific file you wish to exclude from the PR in your working directory.
-
Execute the reset command: Run the following command to unstage the file.
git reset HEAD path/to/file
This command resets the file's state in the staging area, making it unstaged while leaving it intact in your working directory.
-
Check the status: Use the command below to confirm that the file has been successfully unstaged:
git status
You should see your file now marked as "Changes not staged for commit," confirming that it is no longer included in your upcoming commit.
Method 2: Using git rm
Overview of git rm
The `git rm` command is used for both removing files from the working directory and staging the removal. This command permanently deletes the file from your local repository but allows you to commit this removal, effectively removing the file from the PR.
Steps to Use git rm
-
Remove the file from the repository: If you are certain that the file should be deleted, execute the following command:
git rm path/to/file
This command will simultaneously remove the file from both the working directory and the staging area.
-
Confirm removal: After running `git rm`, check your repository's status again using:
git status
The output should confirm that the file has been deleted and is staged for commit.
-
Commit the changes: Finally, commit your changes with a descriptive message to clarify what you modified:
git commit -m "Remove unwanted file from pull request"
This step finalizes the removal in your local history.
Method 3: Interactive Rebase (Advanced)
Overview
Interactive rebase is a powerful Git feature that allows you to edit previous commits in your branch history. It can be used to remove files from a PR if the changes have already been committed and you wish to alter earlier commits to exclude specific files.
How to Remove a File during Rebase
-
Start interactive rebase: Initiate the interactive rebase process by running:
git rebase -i HEAD~n
In this command, replace `n` with the number of commits you need to review. This action opens an editor displaying the most recent commits.
-
Choose the commit to modify: Look for the commit that added the file you want to remove. Change the word `pick` to `edit` next to this commit. Save and close the editor.
-
Edit the commit file to remove the specific file: Git will stop at the commit you wish to modify, allowing you to make changes. To remove the file, run:
git rm path/to/file
-
Continue the rebase: After removing the file, finalize the changes by continuing the rebase:
git rebase --continue
This command will carry on with the rebase process, applying the remaining commits.
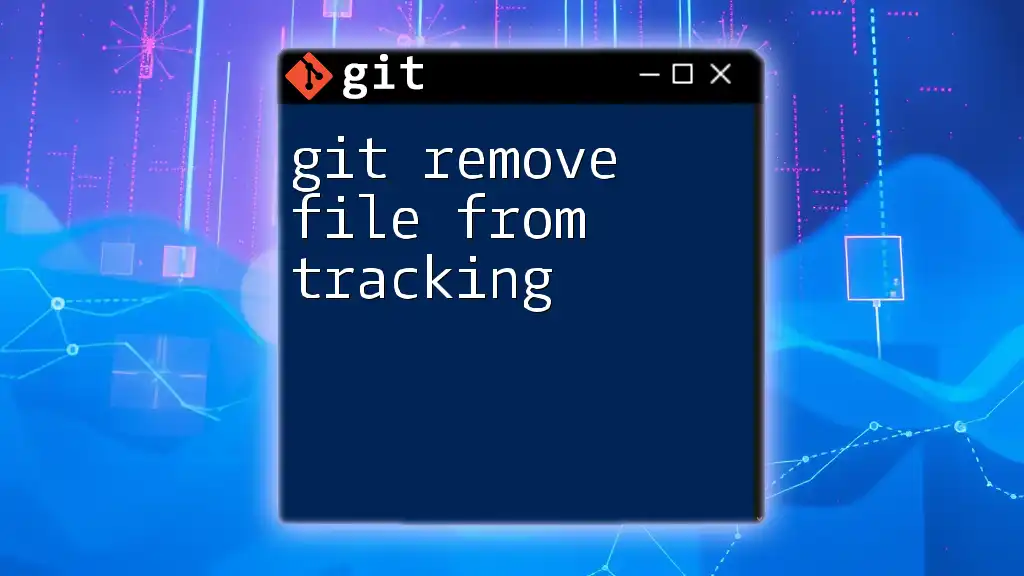
Verifying Changes in the Pull Request
How to Check Your Pull Request
Once you have removed the file, verify that the changes are reflected correctly in the pull request. Navigate to your pull request on GitHub or whichever platform you are using. Review the files changed section to ensure the unwanted file no longer appears.
Confirming the Pull Request Status
Additionally, you can use the command line to confirm that your changes are reflected correctly in your branch by executing:
git show [your-branch-name]
This will display the latest commits and changes in the specified branch, helping you double-check that everything is in order before merging your PR.
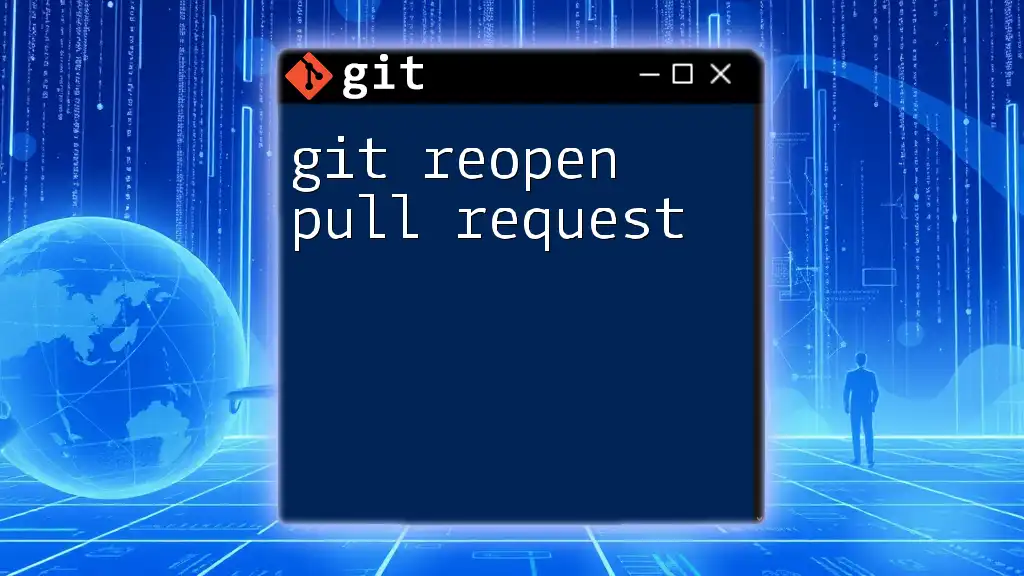
Best Practices When Working with Pull Requests
Keeping Your Pull Requests Clean
One of the best practices when working with PRs is maintaining a clean and focused set of changes. Here are a few tips:
- Commit Often: Frequent commits with clear messages help maintain clarity in your changes.
- Review Your Changes: Always review your staged changes before committing to catch any files you may have unintentionally included.
Common Pitfalls to Avoid
Be cautious of common mistakes such as:
- Forgetting to check the status of your PR before merging.
- Accidentally removing important files or irreversible actions during the removal process. Always back up or review your changes.
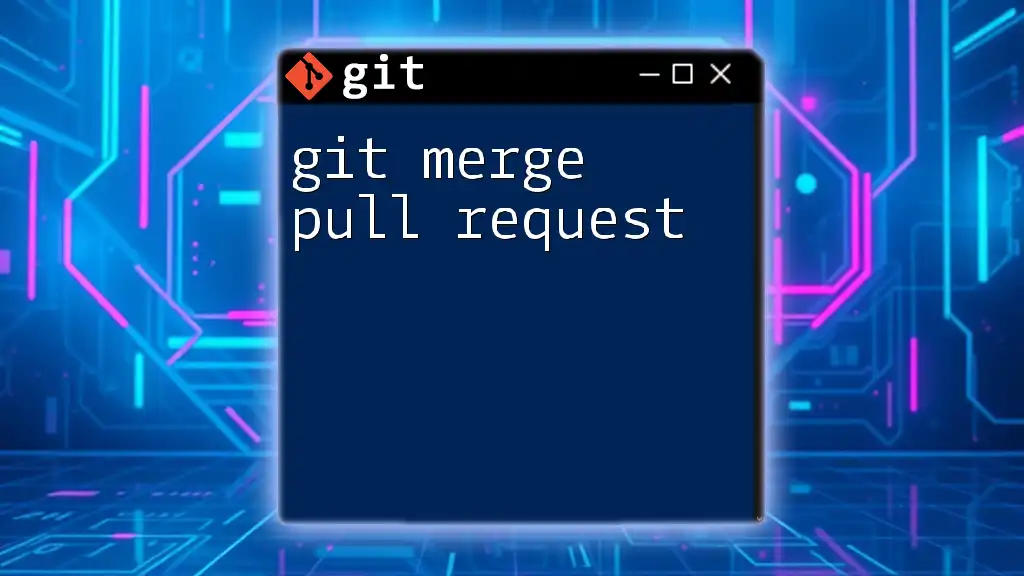
Conclusion
Removing a file from a pull request is a straightforward process that can be accomplished using various methods depending on your needs. By keeping your pull requests clear and free of unnecessary files, you'll streamline the review process and maintain a clean project history. Mastering these simple Git commands not only enhances your workflow but also contributes to the overall efficiency of your development team.
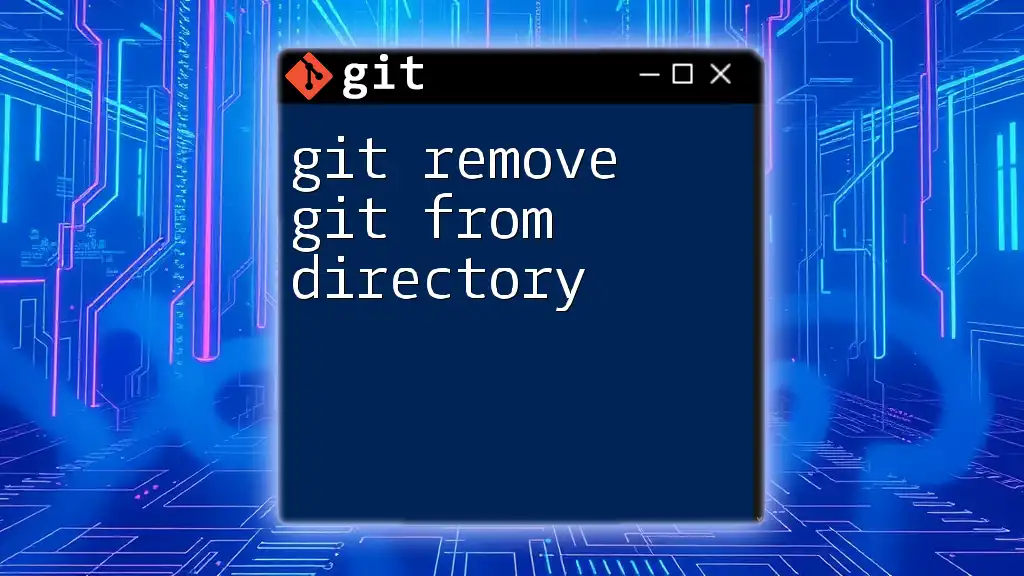
Additional Resources
For those eager to learn more, consider checking out the official Git documentation related to pull requests, `git reset`, `git rm`, and interactive rebase. Engaging with tutorials and courses can further solidify your understanding and proficiency with Git commands.
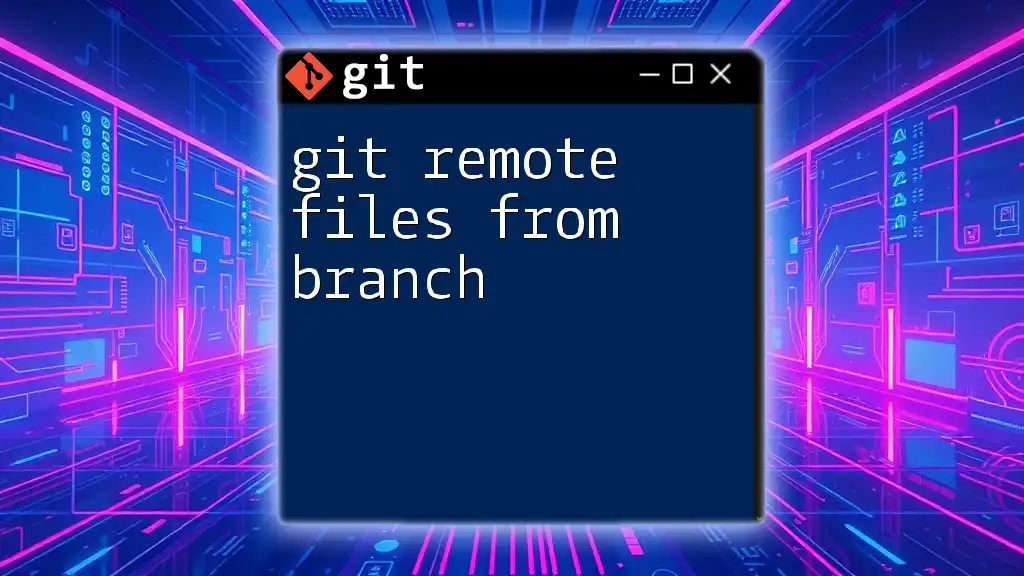
Call to Action
Have you encountered issues while using Git to manage your pull requests? Share your experiences or questions in the comments below, and don't forget to subscribe for more concise Git-related tips and tutorials!