A Git API pull request is a request to merge code changes from one branch into another, typically used in collaborative projects to facilitate code review and discussion before integrating new features or fixes.
git pull origin feature-branch
What is a Pull Request?
A pull request (PR) is a fundamental concept in collaborative software development, particularly in Git-based workflows. It serves as a mechanism for contributors to propose changes to a project hosted in a version control repository, allowing team members to collaborate efficiently. When a developer completes work on a feature or bug fix, they can create a pull request to signal that their changes are ready for review and potential merging into another branch, usually the main or development branch.
Why Use the Git API for Pull Requests?
Utilizing the Git API for managing pull requests offers numerous advantages:
- Automation: Automate the creation and management of pull requests, streamlining workflows and reducing manual effort.
- Integration: Allows integration with CI/CD tools, enabling smoother deployment processes directly from pull request events.
- Customization: Customize and build tools tailored to specific needs without having to rely solely on the web interface.

Understanding Git Pull Requests
What Happens During a Pull Request?
The pull request lifecycle typically involves the following stages:
- Creation: The contributor submits a pull request to propose changes.
- Review: Team members review the proposed changes, suggest improvements, and provide feedback.
- Merge: After addressing any concerns raised during the review, the pull request can be merged into the target branch.
Each pull request contains key information, such as the title and description that explain the changes, the branches involved, designated reviewers, and any necessary status checks.
The Role of Branches in Pull Requests
Branches are essential in the context of pull requests. Typically, developers create individual feature branches for new work. This approach helps maintain a clean and stable main branch, preventing incomplete features from impacting production.
To create a new branch for a feature, you can use the following Git command:
git checkout -b feature/my-new-feature
This command creates a new branch named `feature/my-new-feature` and switches to it, allowing you to work on your changes in isolation.

Using the Git API to Create Pull Requests
Overview of the Git API
The Git API is a set of RESTful APIs provided by Git hosting platforms, such as GitHub and GitLab, enabling developers to interact programmatically with Git repositories. It covers a myriad of functionalities, including creating and managing pull requests.
Authentication with the Git API
Before you can make requests to the Git API, authentication is crucial. You can use methods such as OAuth or Personal Access Tokens (PATs) to authenticate your API requests. Using a PAT is straightforward and can be done like this:
curl -H "Authorization: token YOUR_PERSONAL_ACCESS_TOKEN" https://api.github.com/user
In this command, replace `YOUR_PERSONAL_ACCESS_TOKEN` with your actual token obtained from your Git provider.
Making a Pull Request via Git API
To create a pull request using the Git API, follow these steps:
-
Prepare the Branch: First, ensure you have committed and pushed your changes to a remote branch.
-
Using the API Endpoint to Create a Pull Request: You will use the endpoint:
`POST /repos/:owner/:repo/pulls`
Required fields typically include:
- Title: A brief title for your pull request.
- Base: The branch you want to merge into (usually the main branch).
- Head: The branch you are merging from.
- Body: An optional description to provide context for reviewers.
Here’s an example of how to use cURL to create a pull request:
curl -X POST -H "Authorization: token YOUR_PERSONAL_ACCESS_TOKEN" \
-d '{
"title": "My New Feature",
"body": "This pull request adds a new feature",
"head": "feature/my-new-feature",
"base": "main"
}' \
https://api.github.com/repos/OWNER/REPOSITORY/pulls
Remember to replace `OWNER` and `REPOSITORY` with the relevant values for your project.
Handling Pull Request Reviews
Once a pull request is created, it enters the review phase. This phase is vital for maintaining code quality and ensuring that multiple minds check the code before merging. As part of the review process, you can request specific team members to review your pull request. Engaging with feedback is equally important; it demonstrates your willingness to collaborate and improve.
To respond to reviewer comments, one common approach is to update the pull request. This can be done by amending your commits:
git commit --amend
git push --force
The `--force` option is necessary when updating branches that have already been pushed.
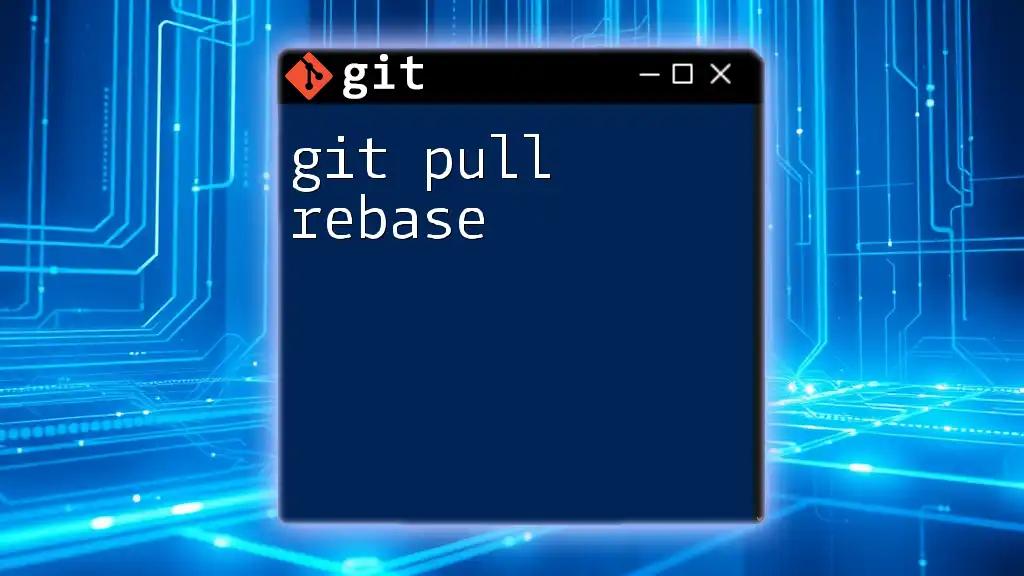
Advanced Pull Request Management Using Git API
Listing Pull Requests
To keep track of open pull requests in your repository, you can retrieve them using the API. Here’s how to get a list of all open pull requests:
curl -H "Authorization: token YOUR_PERSONAL_ACCESS_TOKEN" \
https://api.github.com/repos/OWNER/REPOSITORY/pulls
This command provides a JSON response containing details of all open pull requests, enabling you to monitor progress and status.
Merging Pull Requests
When a pull request is approved, merging can be performed via the API. You can merge a pull request with the following command:
curl -X PUT -H "Authorization: token YOUR_PERSONAL_ACCESS_TOKEN" \
-d '{"commit_title": "Merging my feature", "commit_message": "Merging my new feature into main", "merge_method": "merge"}' \
https://api.github.com/repos/OWNER/REPOSITORY/pulls/PULL_NUMBER/merge
Replace `PULL_NUMBER` with the appropriate number from the pull request you wish to merge.
Deleting Merged Branches
After merging, consider deleting the corresponding feature branch to keep the repository tidy. You can do this easily with the following command:
git push origin --delete feature/my-new-feature
Cleaning up these branches helps manage clutter and keeps the focus on active development lines.
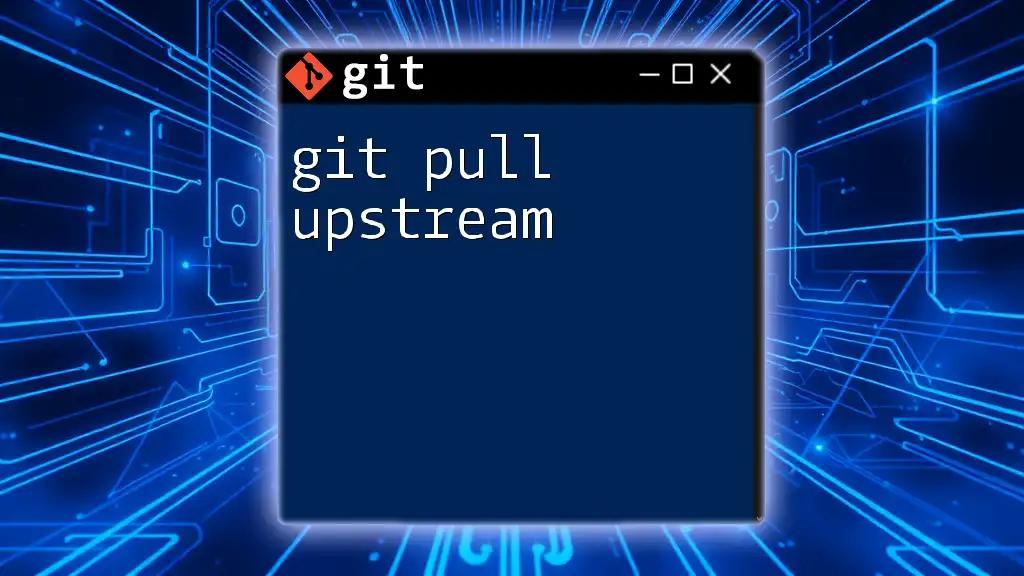
Common Issues with Pull Requests
Troubleshooting Pull Request Failures
While working with pull requests, you may encounter various issues, such as merge conflicts between branches or errors in commit history. Understanding the common pitfalls can save time:
- Merge Conflicts: These happen when two branches have changes to the same line of a file. Resolve conflicts by manually merging or using Git's conflict resolution tools.
- Failed Status Checks: Automated tests may fail, blocking the merge. Ensure your pull request passes all checks before proceeding.
Tips for Successful Pull Requests
Best practices enhance the success rate of pull requests:
- Keep PRs small and focused: Each pull request should address a single issue or feature to simplify reviews.
- Include detailed descriptions: Providing context helps reviewers understand the intention behind changes.
- Engage with reviewers actively: Be responsive to feedback and demonstrate a collaborative mindset.
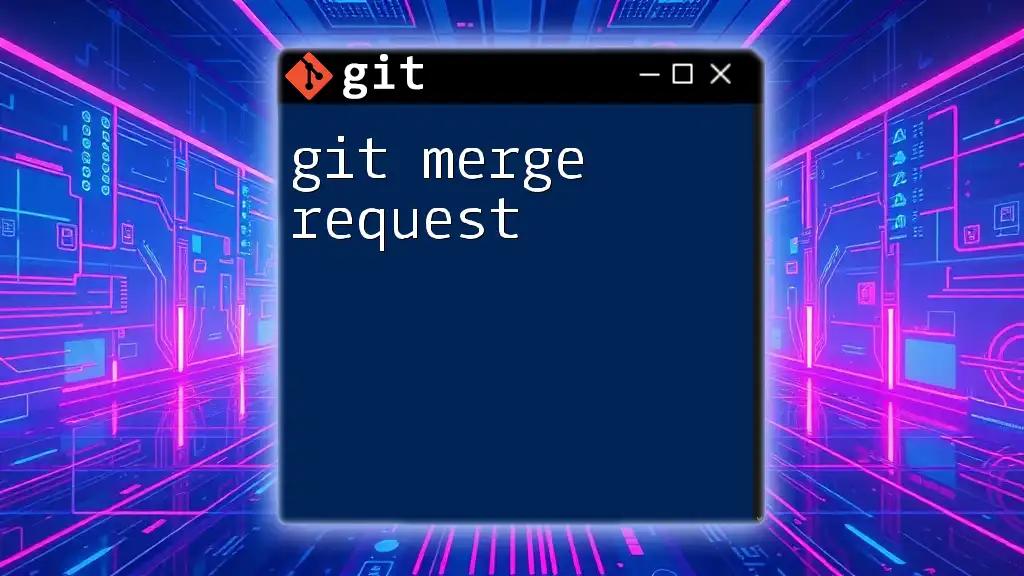
Conclusion
Understanding how to create and manage a git api pull request is crucial for modern developers. The ability to automate and integrate pull request workflows through the Git API not only enhances individual productivity but also fosters vibrant team collaboration. Start practicing these concepts and watch your development process become more efficient!
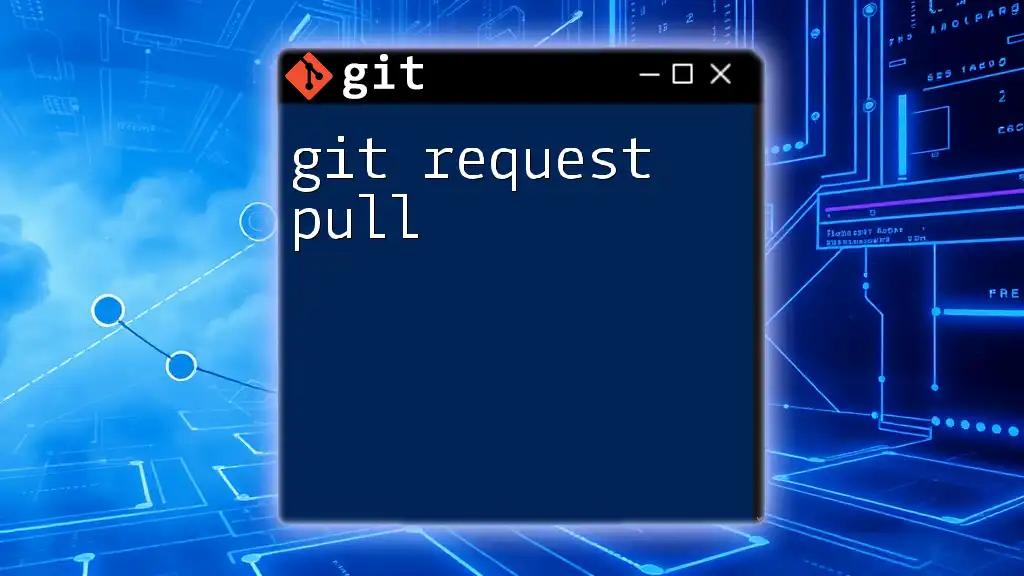
Additional Resources
For further learning, consider exploring the following resources:
- Official Git documentation for a deeper dive into Git concepts.
- Git API tutorials and guides to build on this foundational knowledge.
- Recommended tools that can help streamline your Git workflows.