To remove a file from Git tracking while keeping it in your working directory, use the following command:
git rm --cached <filename>
Understanding Git Tracking
What is Git Tracking?
In Git, tracking refers to keeping track of changes made to files in a repository. When you add a file to your Git project, it can be categorized as either tracked or untracked. Tracked files are those that Git is aware of and monitors for changes, whereas untracked files are those that have not yet been added to Git’s index.
Why You Might Want to Remove a File from Tracking
There are various scenarios in which you might find it necessary to untrack a file. For example:
- Temporary Files: Files generated during the development process, such as compilation logs or caches, that don’t need to clutter the repository.
- Sensitive Information: Configuration files containing passwords or API keys should not be tracked to prevent them from being pushed to shared repositories.
Understanding these scenarios can help maintain a clean and efficient Git repository, ultimately facilitating better collaboration with your team.
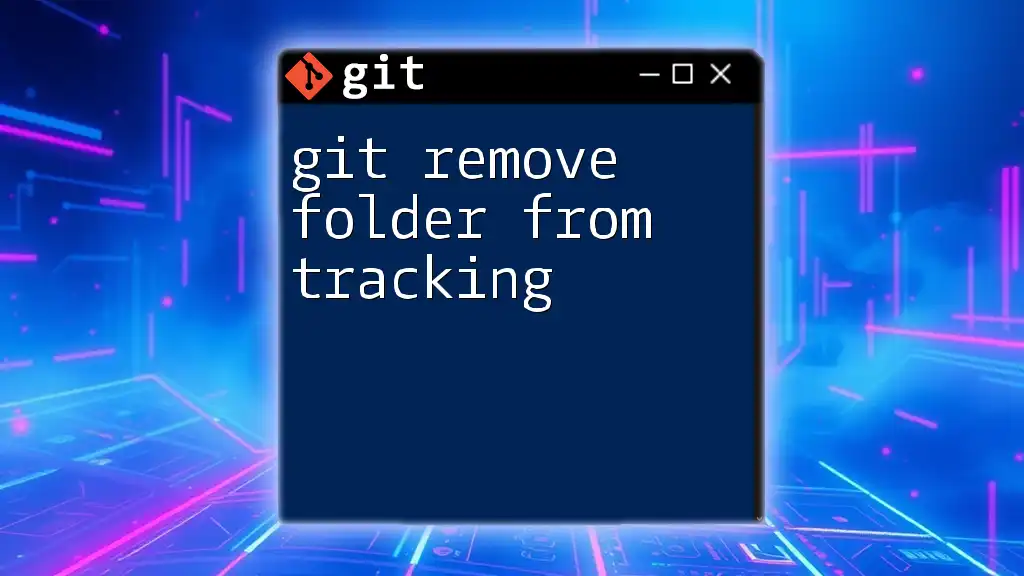
How to Remove a File from Tracking
The Basic Command: `git rm --cached`
To remove a file from tracking in Git, you can use the command:
git rm --cached <file>
The `--cached` option tells Git to remove the file from tracking but keeps it in your working directory. This is crucial because you might still need the file locally.
Examples of Using `git rm --cached`
Example 1: Untracking a Single File
Suppose you realize that a log file, such as `debug.log`, is being tracked and it should not be. To untrack this file, execute the following command:
git rm --cached debug.log
After running this command, check the status of your files using:
git status
You should see that `debug.log` is no longer tracked, yet it remains in your project directory.
Example 2: Untracking Multiple Files
When you have several files you wish to untrack, you can specify them all in one command. For instance, if you want to untrack both `file1.txt` and `file2.tmp`, you would run:
git rm --cached file1.txt file2.tmp
After executing this command, use `git status` again to confirm the changes.
Removing All Tracked Files of a Specific Type
Explanation of Using Wildcards
Git allows the use of wildcards for efficiently untracking multiple files of the same type.
Example: Untracking All `.log` Files
If there are multiple log files you wish to untrack, you might prefer using a wildcard. The command would look like this:
git rm --cached *.log
This command removes all files that have the `.log` extension from Git’s tracking. Again, remember to check the status with `git status` to confirm the untracking has taken place.
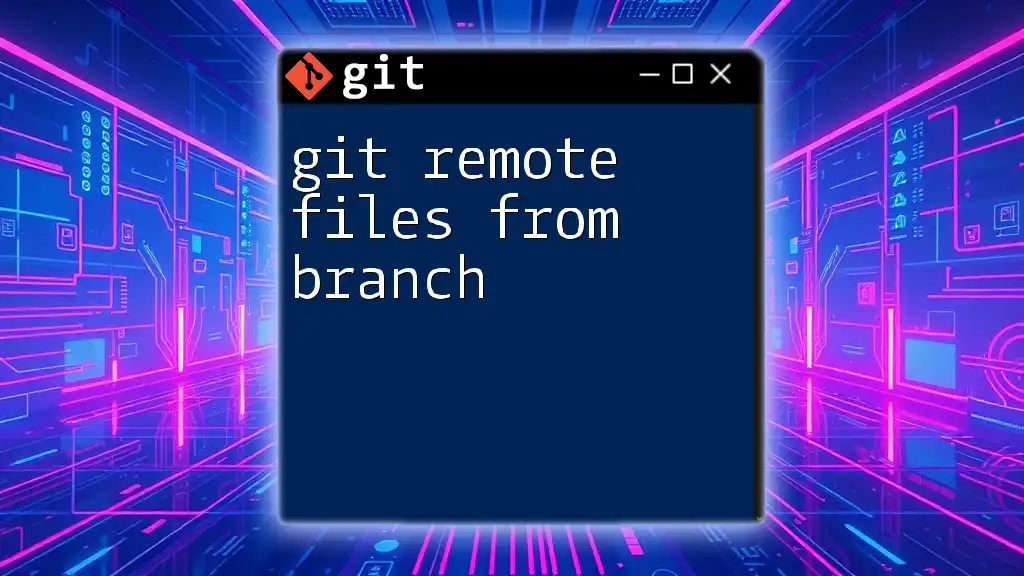
Making Changes Permanent
Committing the Change
After you've removed files from tracking, it’s vital to commit these changes. This ensures that any collaborators will also see that these files are no longer tracked. To commit your changes, use:
git commit -m "Removed files from tracking"
This action documents your decision to untrack the files and keeps your project's history clean.
Verifying the Changes
To ensure everything is correct, it’s essential to verify your changes using:
git status
This command will show you any tracked or untracked files, allowing you to confirm that your intended files are indeed untracked.
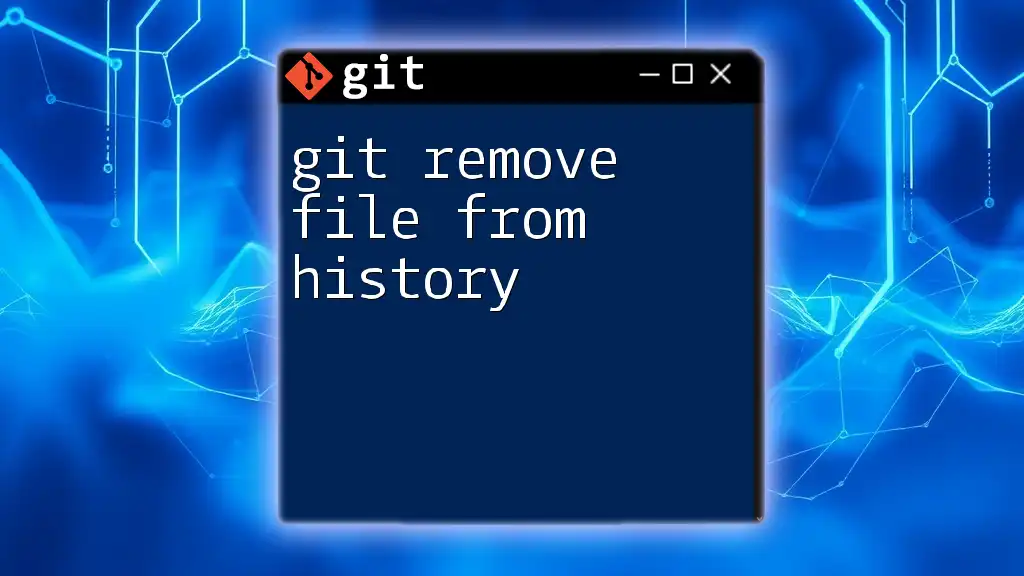
Ignoring Files with `.gitignore`
What is `.gitignore`?
The `.gitignore` file is utilized to specify files and directories that should be ignored by Git. By adding files to this list, you prevent them from being tracked in the future.
How to Add Files to `.gitignore` After Untracking
Once you've untracked a file, you can add it to the `.gitignore` file to ensure that it remains untracked in subsequent commits. Simply open (or create) a `.gitignore` file and add your patterns, such as:
*.log
This regex pattern will ignore all log files going forward, keeping your repository clean.
The Importance of `.gitignore`
Utilizing the `.gitignore` file is crucial for managing project cleanliness and ensuring that unnecessary or sensitive files aren't shared with collaborators, protecting both your project and your data.
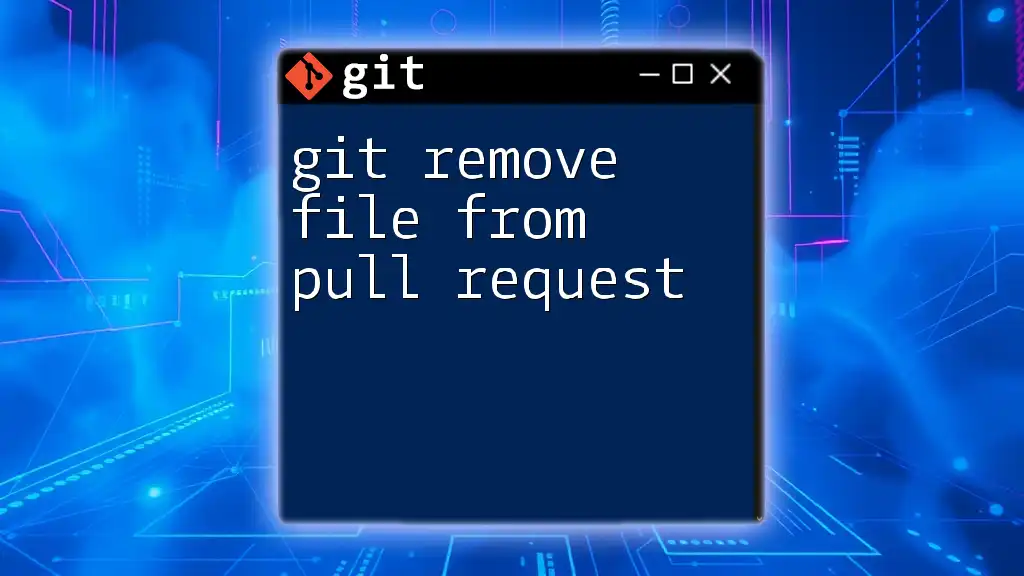
Common Pitfalls to Avoid
Forgetting to Commit
A frequent mistake when removing files from tracking is neglecting to commit the changes. If you do not commit, the changes will not be recorded, and others may still see the files as tracked.
Untracking Files Prematurely
It’s important to double-check your intentions before untracking a file. Removing crucial files from tracking can create challenges during development and collaboration, particularly if the file is essential for the build or deployment process.
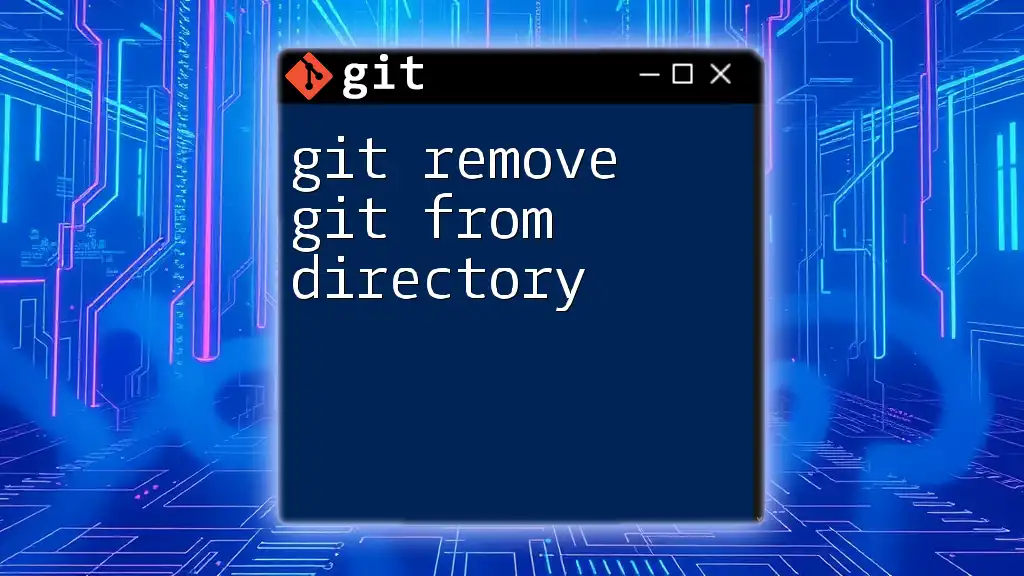
Conclusion
Understanding how to remove files from tracking in Git is a vital skill for keeping your repository organized and efficient. By utilizing commands like `git rm --cached` and the `.gitignore` file effectively, you can ensure that your version control process is streamlined and free of unnecessary clutter.
As you explore Git further, embracing these tools will aid both your personal projects and collaborative efforts with your team. Don't hesitate to experiment with Git commands in a safe environment to deepen your understanding and proficiency.
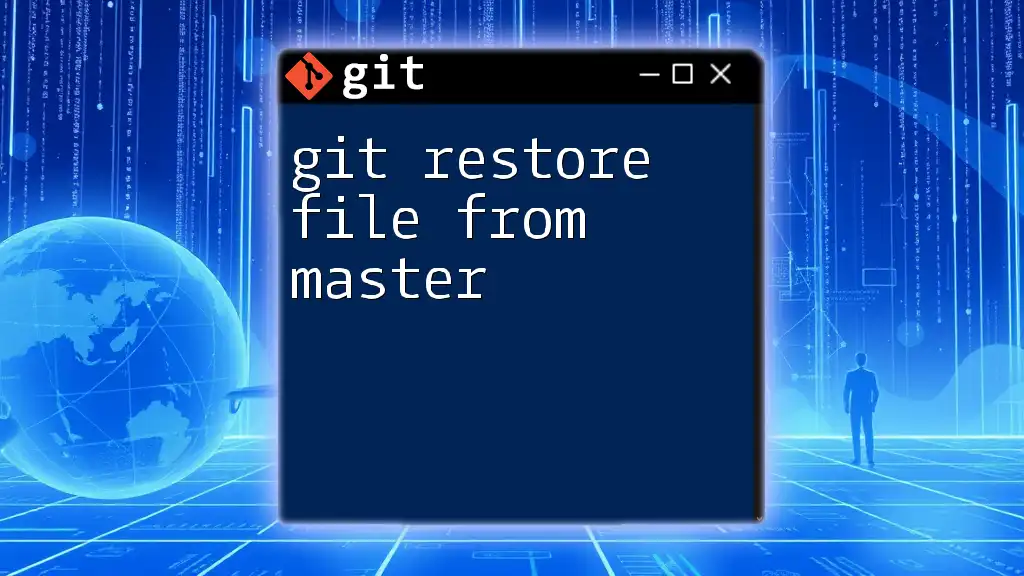
Additional Resources
For those interested in learning more about Git, consider visiting the official Git documentation or engaging with community forums and support platforms. These resources can provide valuable insights and guidance as you navigate the vast potential of version control systems.