When git remote files are not showing up locally, it often indicates that changes haven't been fetched or the branch hasn't been checked out correctly; you can resolve this by running the following command to fetch and update your local repository from the remote:
git fetch origin
git checkout main # replace 'main' with your branch name
Understanding the Basics of Git Remote Repositories
What is a Git Remote?
A remote in Git refers to a version of your repository hosted on the internet or an intranet. Remotes allow multiple users to collaborate on projects by sharing code changes, maintaining a central source of truth. When you clone a repository, Git automatically sets up a remote named `origin` that points to the cloned repository's URL.
Common Operations with Remotes
To effectively manage remote repositories, it’s essential to understand common Git operations such as:
- Cloning a Repository: Using the `git clone` command, you create a local copy of a remote repository.
- Fetching Changes: The `git fetch` command retrieves updates from the remote but does not merge them into your current branch.
- Pulling Changes: The `git pull` command combines fetching and merging in one step, updating your local branch with the latest changes from the remote.
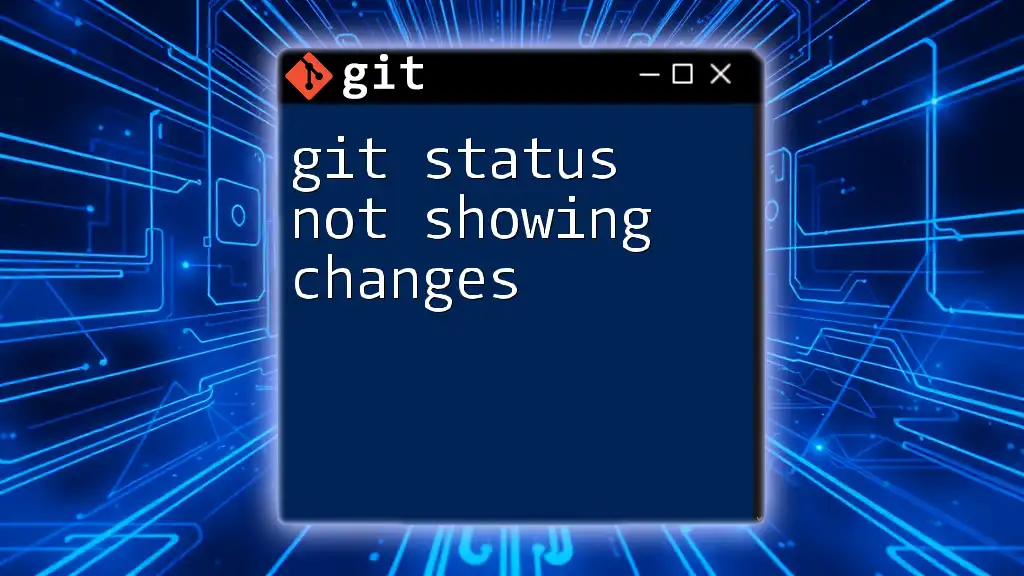
Diagnosing the Issue of Missing Local Files
Identifying the Problem
The first step to addressing the issue of git remote files not showing up local is to ascertain the symptoms. If after pulling updates you still do not see newly created files from the remote, this could be due to several factors, such as:
- Local changes not being committed or merged
- Being on the wrong branch
- Improper remote configuration
Confirming Remote Configuration
To verify your current remote configuration, run the following command:
git remote -v
This command shows the list of remotes and their URLs. Ensure the `origin` is correctly pointing to the desired remote repository.
Checking for Local Changes
Before you proceed, check for uncommitted local changes that may interfere with the incoming updates. Execute:
git status
If there are changes listed, assess whether you want to commit, stash, or discard them before continuing.
Ensuring Proper Branch Tracking
Tracking branches is critical for successfully pulling changes. To check which remote branch your local branch is tracking, run:
git branch -vv
This will show you the upstream branch for your current branch. If it doesn’t point to the correct remote branch, you won’t receive updates as expected.
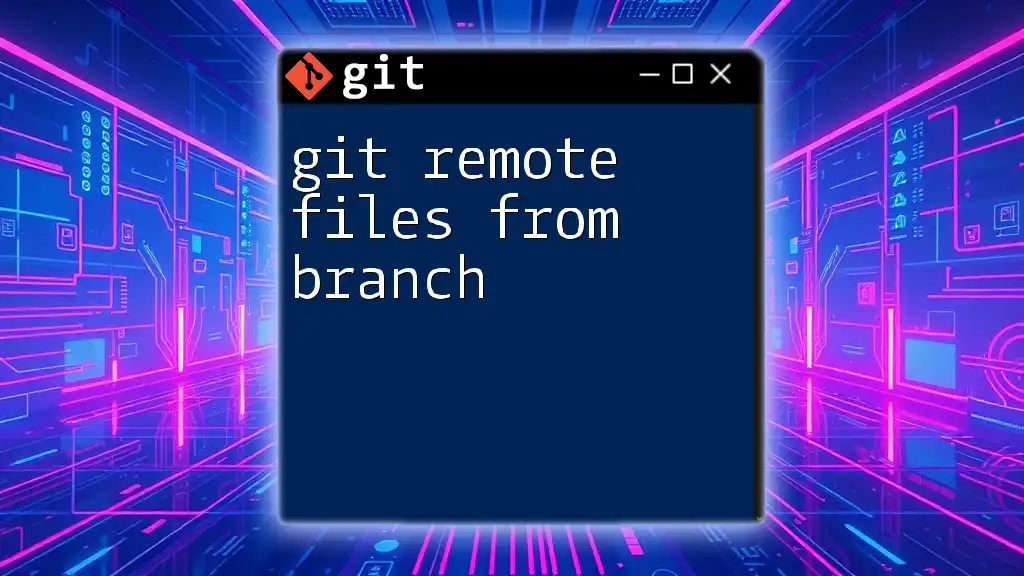
Solutions to Fix the Issue
Fetching Remote Changes
One of the first remedies for git remote files not showing up local is to fetch changes from the remote. This action retrieves new commits without merging them into your working directory. Execute the command:
git fetch origin
After fetching, check if the files now appear by inspecting the branch or using `git status`.
Pulling Latest Changes from Remote
If changes are still not visible, you may need to pull the latest updates. This command not only fetches but tries to merge the changes into your current branch:
git pull origin main
Replace `main` with the name of your branch if you're working in a different one.
Checking for Detached HEAD State
If you encounter issues even after executing the above commands, ensure you aren’t in a detached HEAD state. Being in this state means you're not on any branch, which can lead to missing remote files. Check your current branch status with:
git branch
If you see `HEAD detached`, switch back to your intended branch using:
git checkout main
Ensuring You Are on the Correct Branch
To resolve git remote files not showing up local, always ensure you are on the correct branch. If you need to switch, the following command will help you do that:
git checkout <branch-name>
Replace `<branch-name>` with the name of the branch you intend to synchronize with.
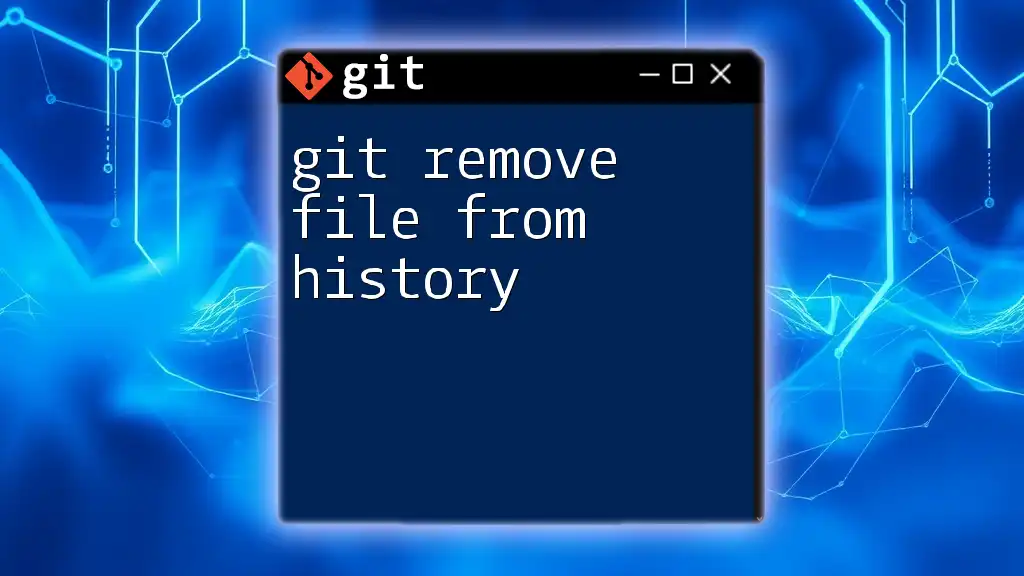
Advanced Troubleshooting Tips
Dealing with Merge Conflicts
Sometimes, when you pull changes, you might encounter merge conflicts, especially if changes were made to the same line of code. When this happens, Git will notify you, and you'll have to resolve conflicts manually. Look for files with conflict markers, edit them, and then mark them as resolved using:
git add <file>
After resolving conflicts, commit the changes:
git commit -m "Resolved merge conflicts"
Investigating Untracked Files
Another possible reason files may not appear is due to untracked files. You can view any untracked files using:
git ls-files --others --exclude-standard
If important files are untracked, you may want to add them to the index:
git add <file>
Utilizing the Git Log
To further investigate if remote updates are present, examine the commit history. The `git log` command can provide insight into any recent changes on the remote that haven’t synced yet:
git log --oneline --graph --decorate --all
This command displays a visual representation of the commit history across all branches.
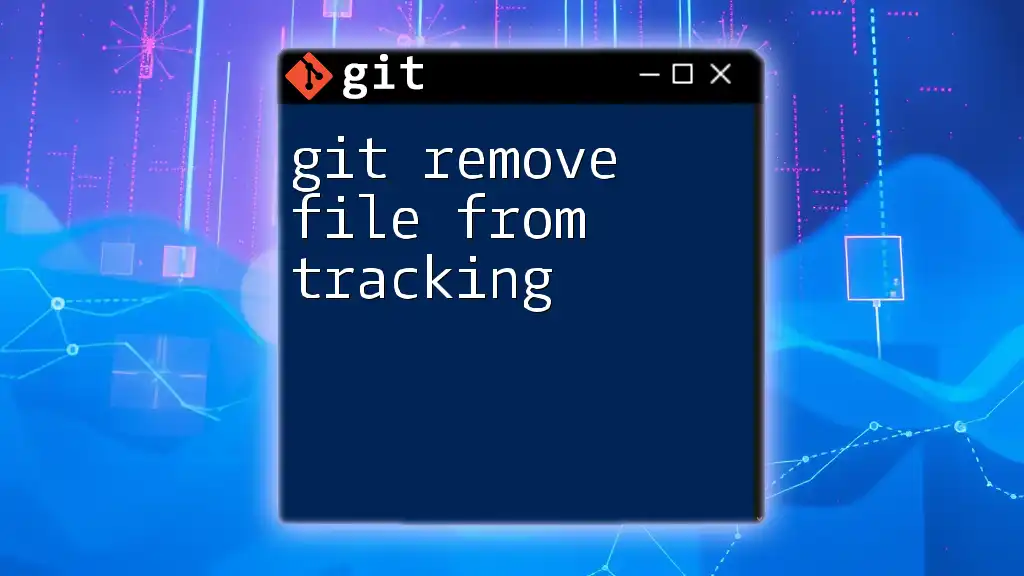
Conclusion
Addressing the issue of git remote files not showing up local requires an understanding of Git fundamentals and various troubleshooting techniques. By following the outlined steps, including fetching and pulling changes, ensuring correct branch tracking, and resolving merge conflicts, you can efficiently synchronize your local repository with the remote.
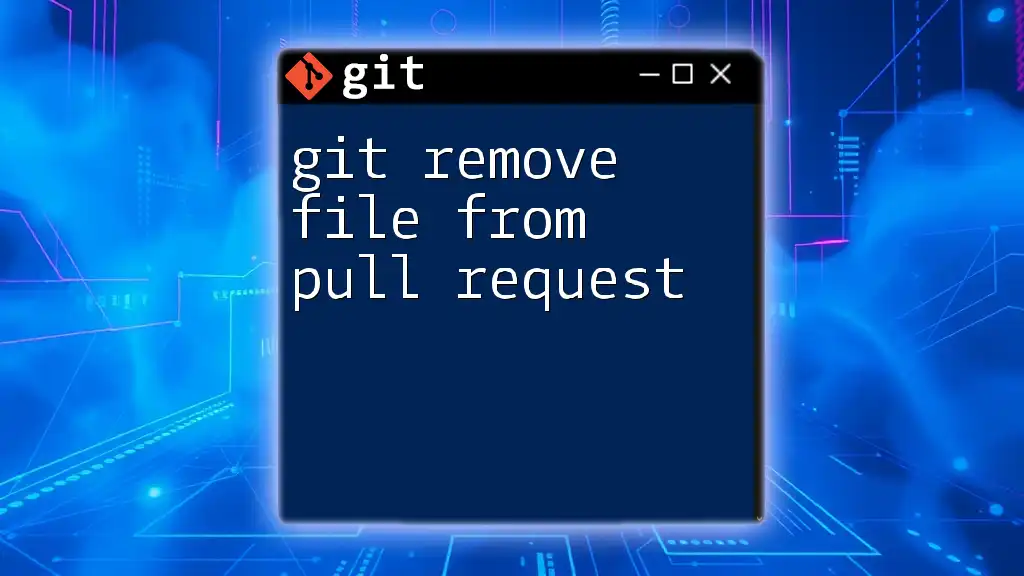
Call to Action
If you find these troubleshooting steps helpful, consider signing up for our Git courses for a deeper understanding of version control. Don't forget to share this article with others who might be facing similar challenges!
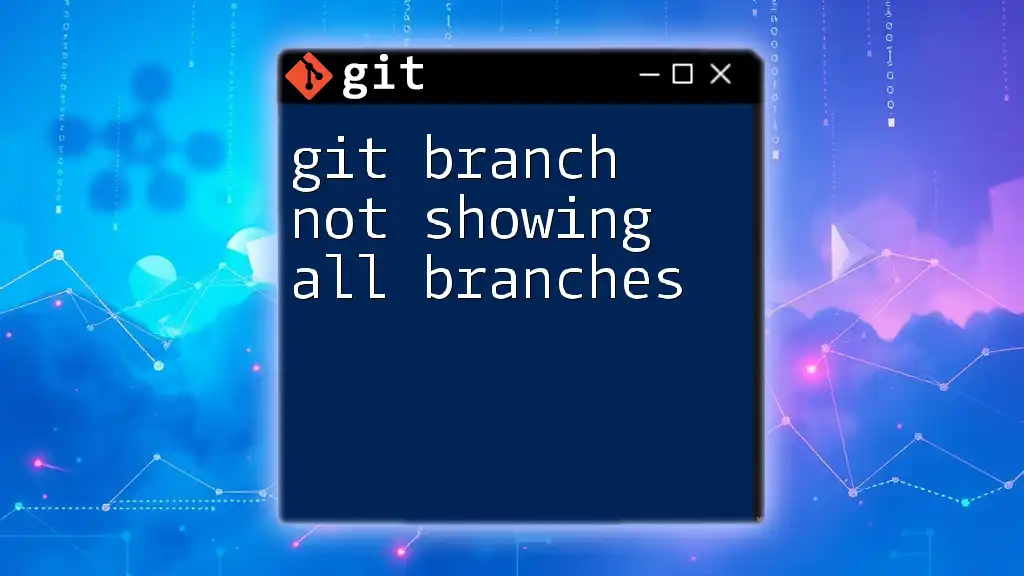
Additional Resources
Recommended Git Commands Cheat Sheet
Download our comprehensive Git commands cheat sheet to bolster your Git knowledge and workflow efficiency.
Further Reading
Explore additional articles and documentation on Git to further your learning journey and enhance your skills in version control!