If Git is not showing all remote branches, you may need to fetch the latest branches from the remote repository by using the following command:
git fetch --all
Understanding Remote Branches in Git
What are Remote Branches?
Remote branches play a crucial role in Git's ability to support collaborative programming. Essentially, a remote branch represents a branch hosted on a remote repository. Each time you push changes, you're interacting with these branches, which allows your teammates to access your updates and vice versa.
In contrast to local branches, which exist only in your local repository, remote branches are tracked and synchronized with a shared repository. This design ensures that multiple team members can contribute to the same source code without stepping on each other's toes.
Understanding the distinction between local and remote branches is vital—local branches can diverge from their remote counterparts, which sometimes leads to the issue of Git not showing all remote branches.
Common Scenarios for Missing Remote Branches
Several situations can cause your Git client to overlook certain remote branches:
- Working with multiple remotes: If you're collaborating with various repositories, you might miss branches in another remote.
- Recent pushes by team members: Changes made by your teammates may not yet be reflected in your local repository if you haven’t fetched the latest updates.
- Remote branches not yet fetched: The branches you expect to see haven’t been retrieved yet.
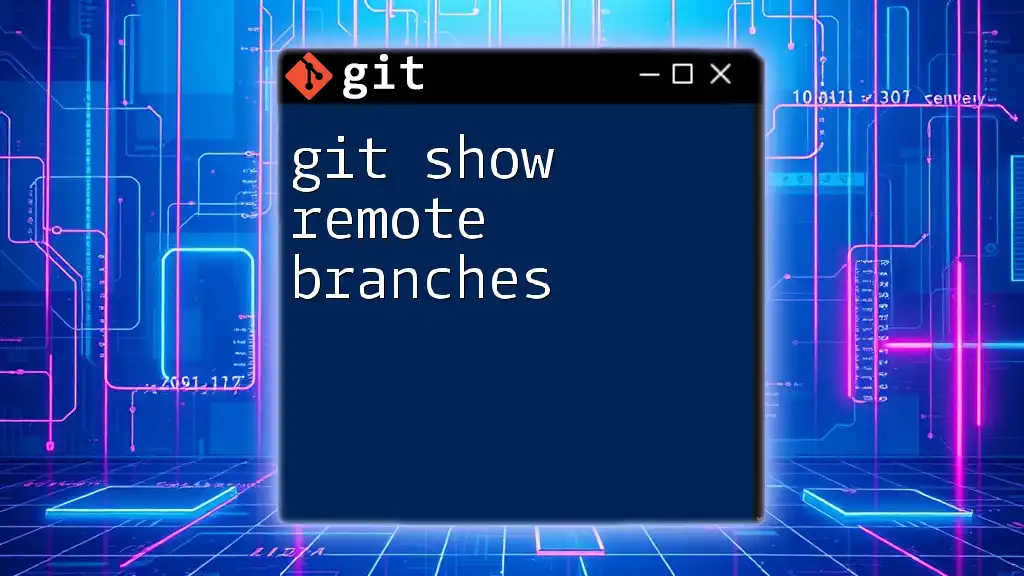
Initial Checks to See Remote Branches
Using `git branch -r`
The first command to check remote branches is `git branch -r`, which lists all the branches available on the remote repository. This simple command can clarify whether you’re truly missing branches or just overlooking them.
Example usage:
git branch -r
Output: You will see a list of remote branches like:
origin/feature-1
origin/feature-2
origin/master
If you notice that a branch you expect isn’t listed, it confirms that the problem lies elsewhere.
Accessing All Branches with `git fetch`
To ensure your local repository has all the updates from the remote, use the `git fetch` command. Unlike `git pull`, which also merges changes into your working directory, `fetch` merely updates your remote tracking branches.
To fetch all branches, you can run:
git fetch --all
After this command, check your remote branches again with `git branch -r`. This simple step often resolves issues where branches seem to have disappeared.
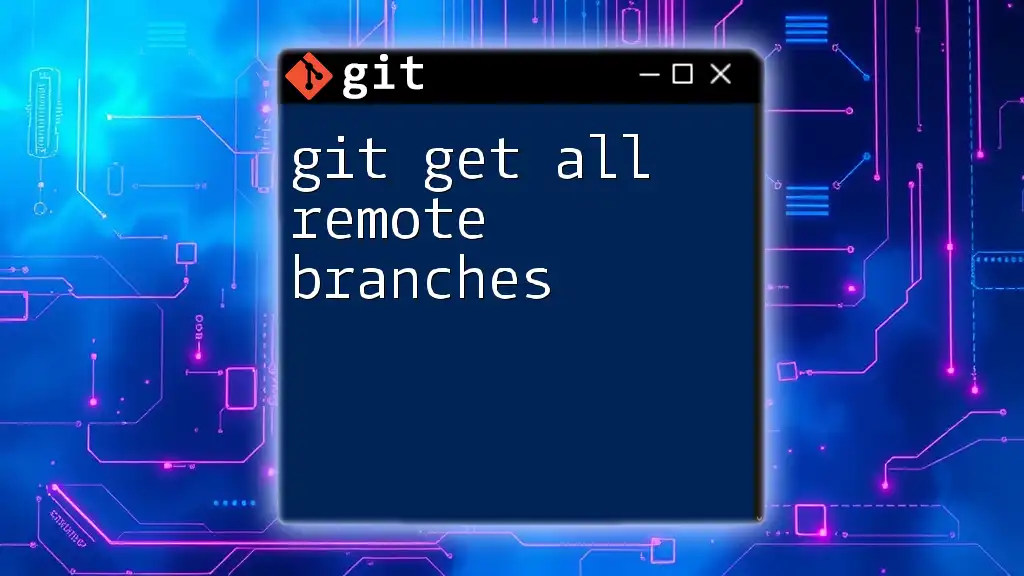
Troubleshooting Steps for Missing Remote Branches
Verify Remote Configuration
Checking the Remote URL
Sometimes the URL that points to your remote repository might be incorrect. By running the command:
git remote -v
You can check the URL associated with each remote.
Example output:
origin https://github.com/user/repo.git (fetch)
origin https://github.com/user/repo.git (push)
If the URL is incorrect, you'll need to set the correct remote URL using:
git remote set-url origin <new-url>
Listing All Remotes
To delve deeper into your repository’s configuration, you might want to run:
git remote show origin
This command provides comprehensive details about your remote, including all remote branches and their tracking status. Analyzing this information can often highlight misconfigurations that cause branches not to appear.
Checking Permissions and Access Rights
In some cases, access rights might prevent you from seeing all remote branches. If you don’t have adequate permissions for the repository, certain branches may be inaccessible. Ensure your SSH keys or HTTPS authentication credentials are correctly configured.
It’s important to check with your repository administrator if you suspect this is the case.
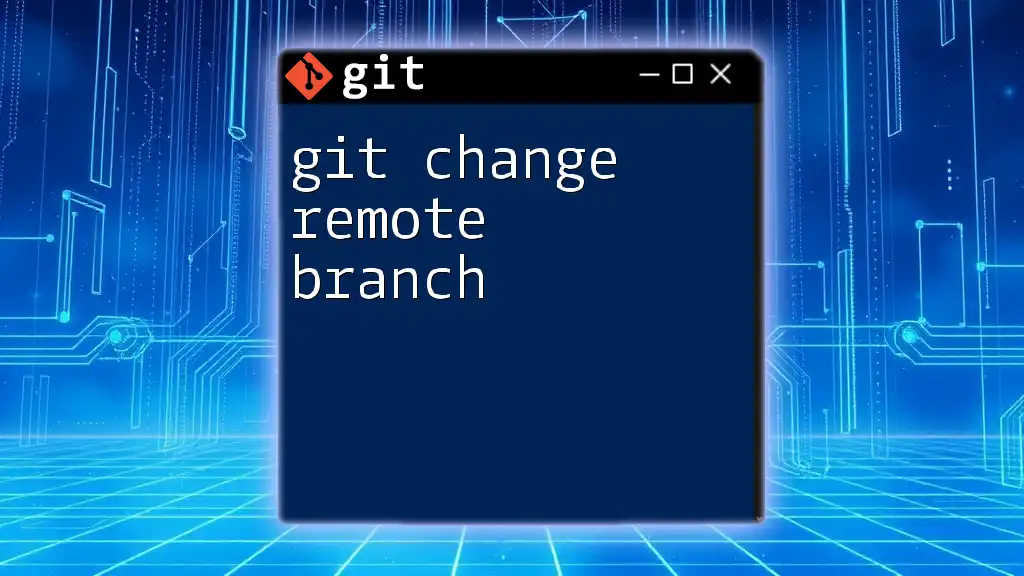
Home on the Range: Exploring Remote Branch Tracking
Understanding the Tracking Branches
When working with remote branches, it’s essential to grasp the concept of tracking branches. A tracking branch is a local branch that has a direct relationship with a remote branch, tracking its changes and updates.
To create a tracking branch, you can run:
git branch --track new-branch origin/new-branch
This command ensures that your local branch updates according to changes in the specified remote branch.
Ensuring Remote Branches Are Fully Tracked
Properly tracking remote branches is crucial to avoid the issue of Git not showing all remote branches. To keep your remote branches synchronized with local ones, using `git pull` versus `git fetch` can make a significant difference.
Remember, `git pull` performs two actions: it fetches changes from the remote and merges them into the current branch. Meanwhile, `git fetch` only updates your remote tracking branches.
For instance, you might run:
git pull origin master
This fetches updates for your local `master` branch from the `origin`, ensuring it's current.
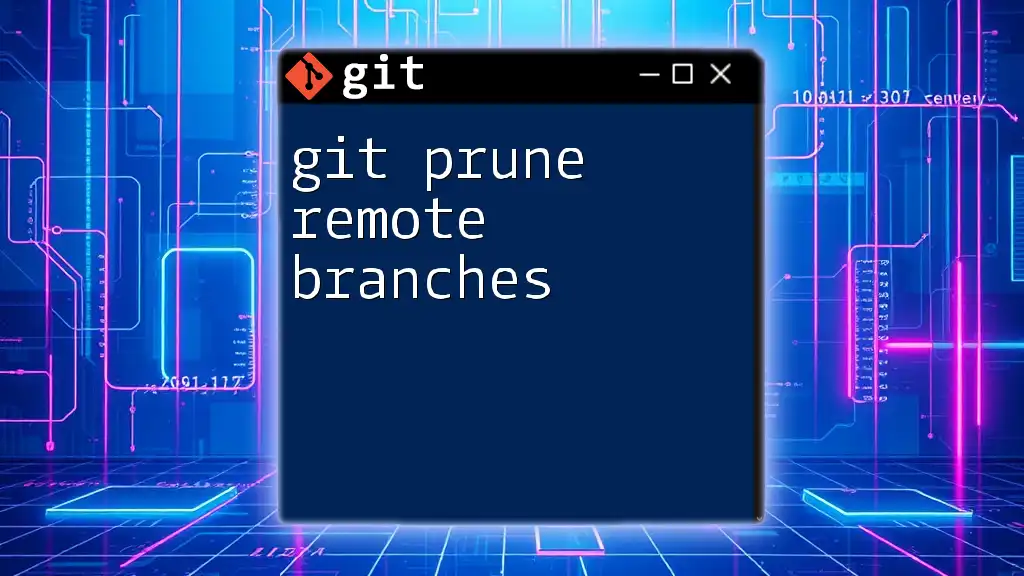
When Branches Still Don’t Appear: Advanced Troubleshooting
Dealing with Stale References
Sometimes, the presence of stale references in your Git configuration can undermine visibility of remote branches. These references often stem from branches that have been deleted on the remote but remain on your local tracking.
To clean up these stale references, execute:
git remote prune origin
This action will remove references to branches that no longer exist on the remote repository.
Checking Your Git Configurations
Misconfigurations in your Git settings could also lead to missing remote branches. Run the following command to list your configurations:
git config --list | grep remote
Carefully review the output for any settings that might be affecting your remote branch visibility, like `remote.origin.fetch` configurations.
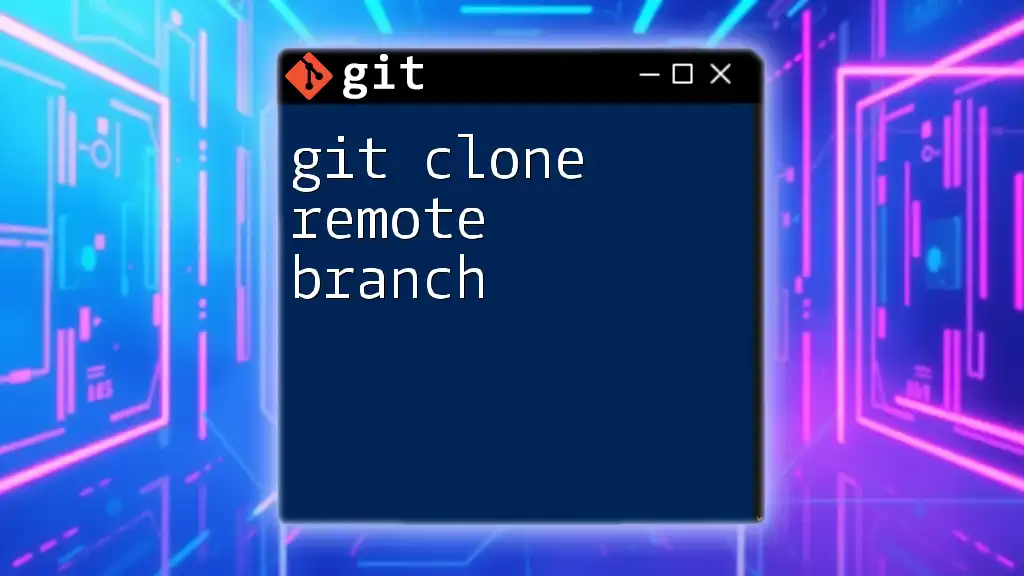
Conclusion
Understanding how to manage and troubleshoot remote branches is vital for any Git user. By following the steps outlined above, you can effectively address the issue of Git not showing all remote branches. Encouraging hands-on practice with these commands will equip you to handle similar situations in the future with ease.
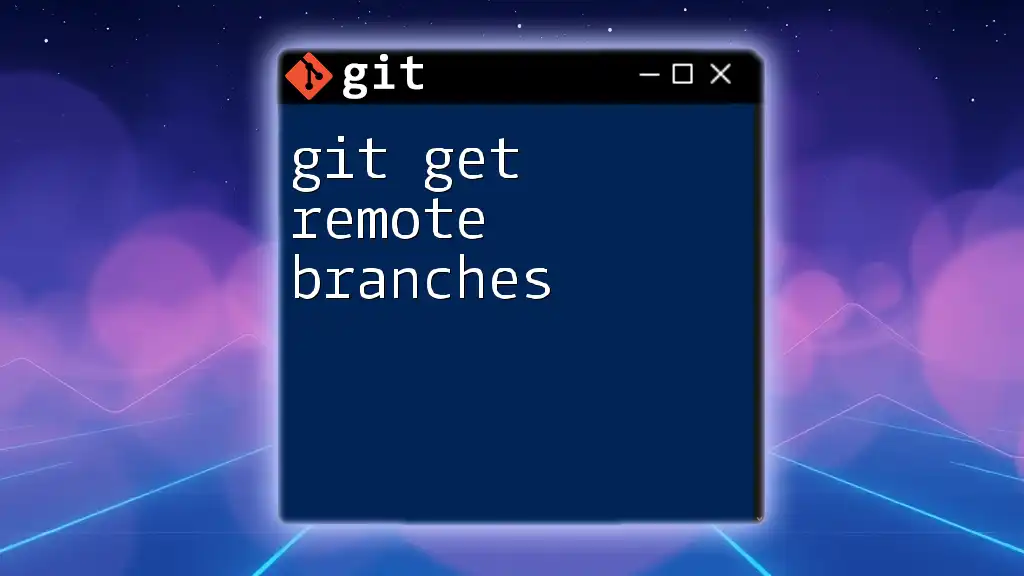
Additional Resources
For further reading, check out the official Git documentation, and don't forget to join community forums for support. If you want to enhance your skills, consider exploring video tutorials or Git-focused books as valuable resources.
By mastering these techniques, you will not only enhance your Git skills but also streamline your collaboration with team members, ensuring a smoother workflow!