To restore a specific file from the `master` branch to your current working directory, you can use the following command:
git checkout master -- path/to/your/file
Understanding Git Branches
What are Git Branches?
In Git, branches act as independent lines of development within a project. They allow developers to work on features, fixes, or experiments without interfering with the main codebase. When you create a branch, you essentially create a snapshot of your code at that point in time, enabling parallel development without compromising the main project's stability.
What is the 'master' Branch?
Historically, the master branch has been the default branch in Git repositories, serving as the central hub for production-ready code. It's where completed features are merged and deployed. Note that due to a shift towards inclusivity, many projects are transitioning to naming this default branch main. Regardless of its name, this branch typically contains the most stable version of your code. Understanding how to access files from the master branch is crucial for efficient development.
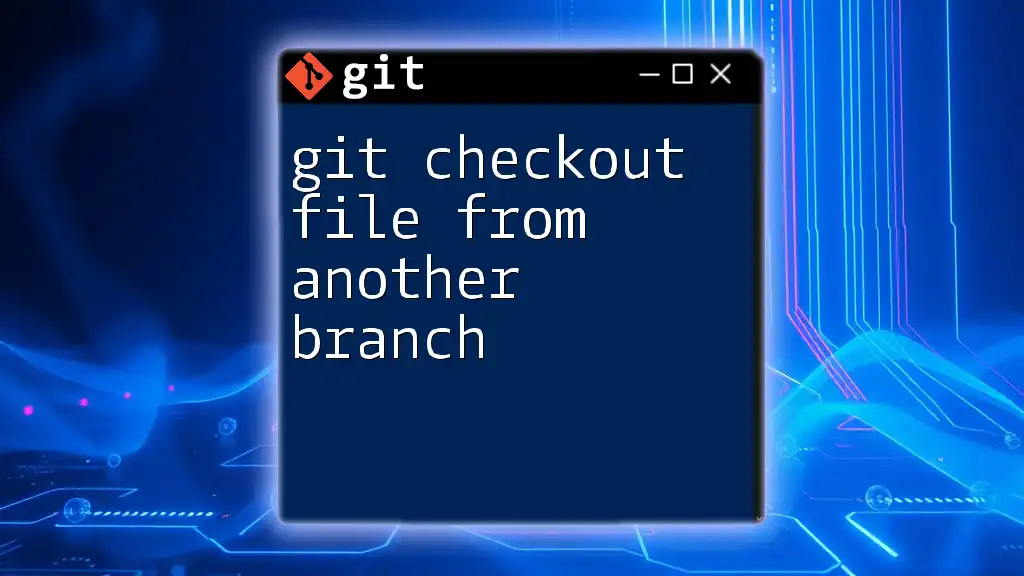
The Need to Checkout a File from Master
Why Checkout a File?
There are several scenarios where you may need to check out a file from the master branch:
- Fixing Merge Conflicts: If a file has changed in both your current branch and the master, you may want to revert to the version in the master to resolve conflicts.
- Reverting Changes: Perhaps you've made alterations to a file that you later determine are unnecessary or problematic. Checking out the file from master allows you to discard your changes quickly.
- Updating Files: Sometimes, you may need to fetch the latest changes from the master branch to ensure that your current work is based on the most recent code.
It's important to understand that checking out a file can overwrite your local changes, so proceed with caution.
Risks Involved
Before executing a checkout command, be aware of the potential risks. If you've made significant modifications to a file and attempt to check out the version from master without properly staging or committing your changes, you risk losing all your uncommitted work. Always ensure you understand the state of your working directory prior to executing the command.
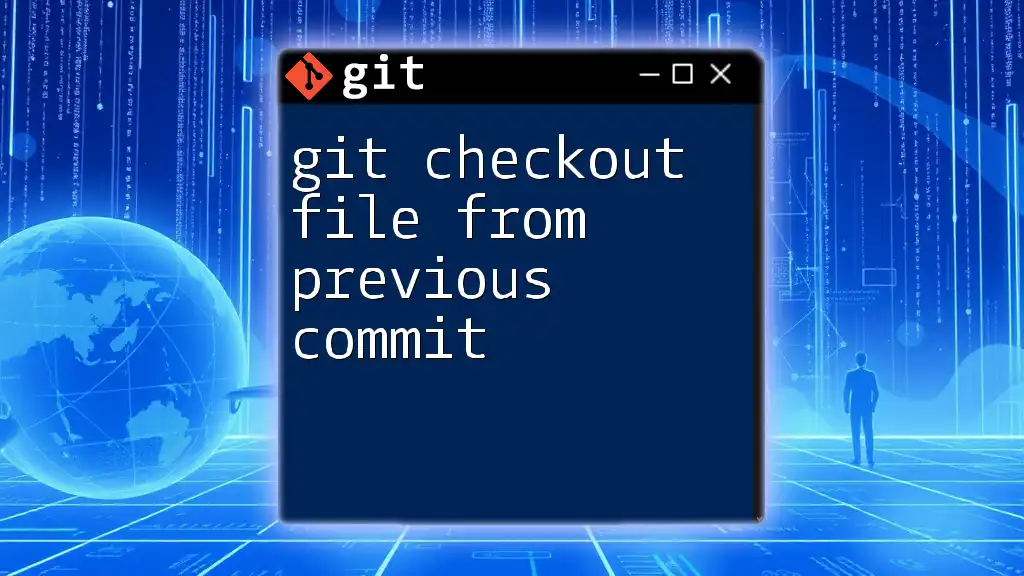
How to Checkout a File from Master
Pre-requisites
Before diving into the command, ensure your repository is set up correctly. You should have Git installed on your machine, and you need to be within a Git repository when executing commands. Familiarity with basic commands like `git status` and `git branch` will also be beneficial.
The Basic Command
The command to check out a specific file from the master branch follows this syntax:
git checkout master -- path/to/file.txt
In this command:
- `git checkout` is the command for switching branches or restoring working tree files.
- `master` specifies the branch from which you want to check out a file.
- `--` separates the branch name from the file path, indicating that you are checking out a file rather than switching branches.
- `path/to/file.txt` is the relative path to the file you wish to restore.
Step-by-Step Guide
Step 1: Check the Current Branch
Before you proceed with checking out a file, it’s wise to verify your current branch. Use the following command to see which branch you’re on:
git branch
This will list all branches, highlighting which one you’re currently working in. Knowing your current branch is crucial to avoid unintentional overwrites.
Step 2: Understand the File Path
To ensure that you are checking out the correct file, confirm the file path you want to restore from the master branch. The path must be accurate, as any errors will result in an unsuccessful command.
Step 3: Execute the Checkout Command
Once you’re aware of your current branch and the correct file path, you can proceed to execute the checkout command. Here’s an example scenario:
git checkout master -- src/components/myComponent.js
Executing this command will fetch the latest version of `myComponent.js` from the master branch and replace the version in your current working directory. After running the command, you'll see a message indicating the file has been successfully checked out.
Example Scenario
Case Study: Fixing a Bug in a File
Imagine you’re working on a feature branch called `new-feature`, and you notice a bug in `myComponent.js` that you know has been fixed in the master branch. Instead of merging all changes from master into your current branch (which could introduce additional complications), you can simply check out `myComponent.js` from master:
git checkout master -- src/components/myComponent.js
This allows you to restore the bug-free version of the file and address your bug without affecting any of your feature developments.
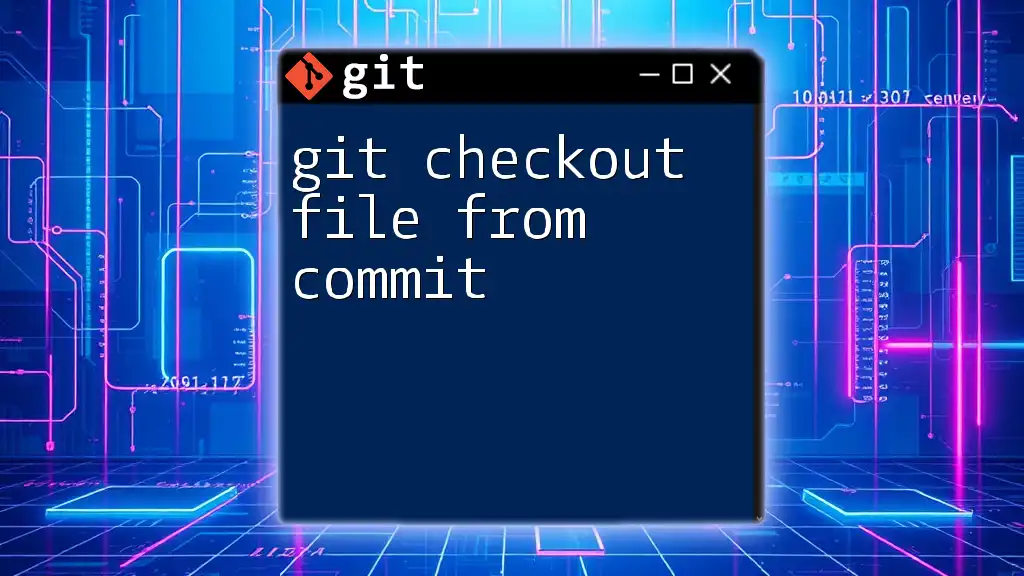
Confirming the Changes
Checking the Status of the Workspace
After checking out the file, it’s wise to verify that the changes have taken place effectively without any unexpected issues. You can use the following command:
git status
This command will show you any modified files in your working directory, confirming that `myComponent.js` has been updated.
Viewing the File Changes
To examine the differences between your current file and the one you just checked out from the master branch, use:
git diff HEAD path/to/file.txt
This command shows what has changed in the file since the last commit. Understanding these changes will help you ensure everything is as expected.
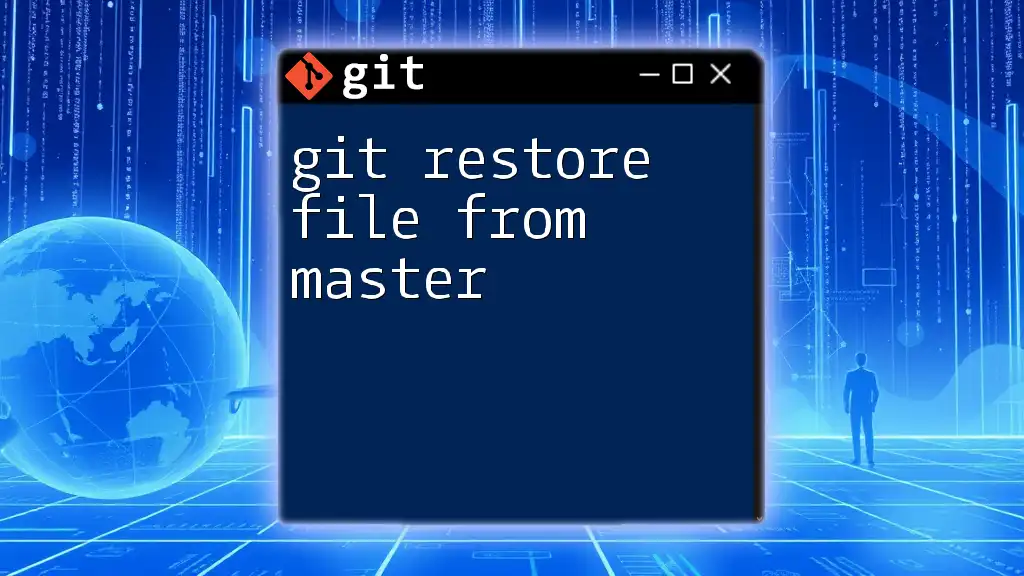
Alternative to Checkout: Using `git restore`
Introduction to `git restore`
With newer versions of Git, the command `git restore` has been introduced to make it easier for users to manage file states. This command is specifically designed to restore working tree files and can serve as an alternative to `git checkout`.
Example of Using `git restore` for a File
To achieve the same effect as checking out a file from the master branch, you can use:
git restore --source master -- path/to/file.txt
This command clarifies your intent to restore the file from a specific source (i.e., master) without the ambiguity that sometimes accompanies the `checkout` command. It’s a cleaner and more intuitive way to revert files.
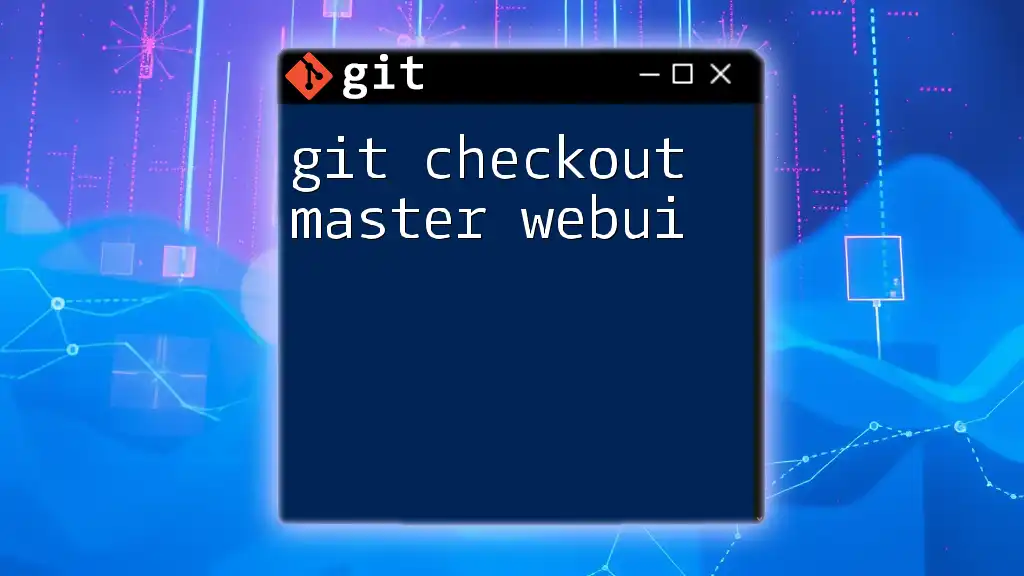
Conclusion
Mastering how to use the git checkout file from master command is a vital skill for any developer working with Git. Whether you need to fix a bug, revert changes, or fetch updates, understanding this command and its implications will elevate your version control proficiency. Practice using this command in a safe environment to ensure you're comfortable executing it when real issues arise.
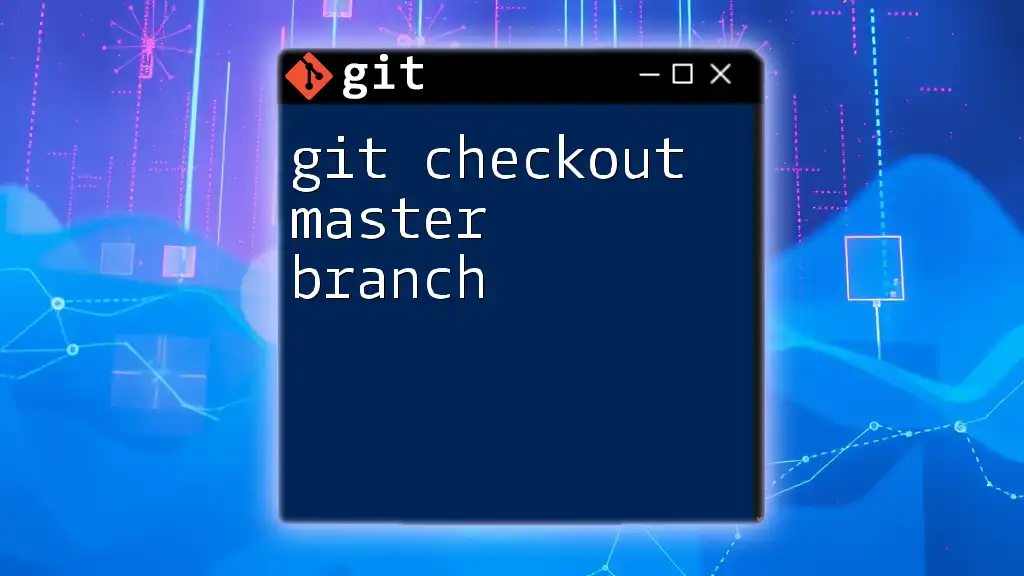
Additional Resources
Links to Further Reading
- Official Git documentation for in-depth understanding.
- Recommended tutorials and guides on branching and checkout commands can deepen your knowledge and skills.
Community Support
Joining forums or communities for Git users can greatly expand your learning experience. Engaging with other developers provides valuable insights and answers to any questions you may have.
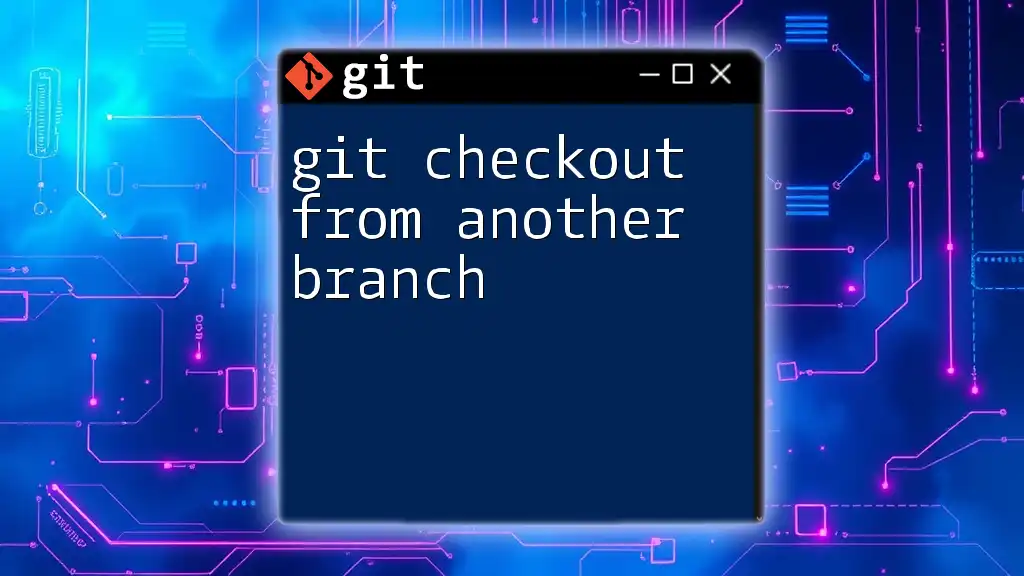
FAQs
Common Questions about Checking Out Files in Git
- What happens to my local changes after checking out a file? Any uncommitted changes in the file you’re replacing will be lost.
- Can I checkout a file from another branch instead of master? Yes, simply replace `master` with the name of your desired branch.
- How do I undo a checkout if I make a mistake? If you check out a file and realize it's not what you wanted, you can use `git checkout -- path/to/file.txt` to revert back to the last committed version of that file.
This guide serves to equip you with the necessary tools and understanding to efficiently use the `git checkout` command, ensuring you can easily navigate your Git repository's files.