The `git checkout` command is used to switch branches or restore working tree files in a Git repository, and its flags allow for specific variations in behavior, such as creating new branches or discarding local changes.
git checkout -b new-branch # Create and switch to a new branch
git checkout -- filename.txt # Discard changes in the specified file
What is `git checkout`?
`git checkout` is a powerful command in Git primarily used for switching between branches or restoring working tree files. It serves as a core functionality that allows developers to navigate through different versions of their codebase efficiently. The versatility of this command makes it essential for anyone looking to master Git.
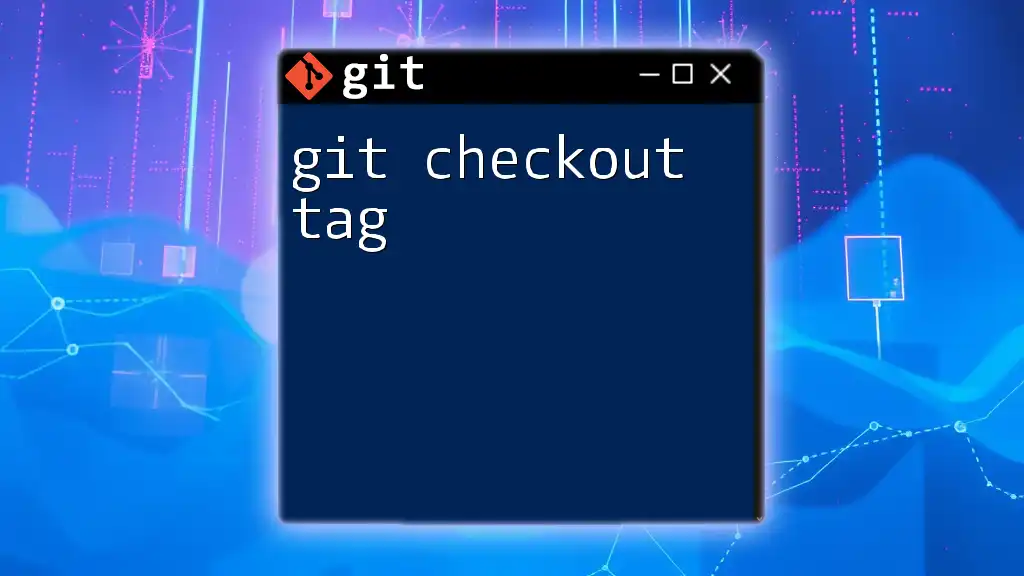
Common Use Cases for `git checkout`
Switching Branches
One of the most frequent use cases of `git checkout` is switching between branches in your repository. Branching allows multiple lines of development, enabling developers to work on features independently without interfering with each other. To switch to a branch named feature-branch, you would use the following command:
git checkout feature-branch
This command updates your working directory to reflect the state of the specified branch, allowing you to begin work immediately.
Restoring Files
If you've modified a file but wish to revert it back to its last committed state, `git checkout` can help with that too. To restore file.txt to its last committed version, you simply run:
git checkout -- file.txt
Note: Be cautious with this operation, as any unsaved changes will be lost.
Creating a New Branch
`git checkout` also allows you to create a new branch and switch to it in one command, making the process much more efficient. To create a new branch called new-branch, you use the `-b` flag:
git checkout -b new-branch
This command not only creates a new branch but also checks it out immediately, streamlining your workflow significantly.
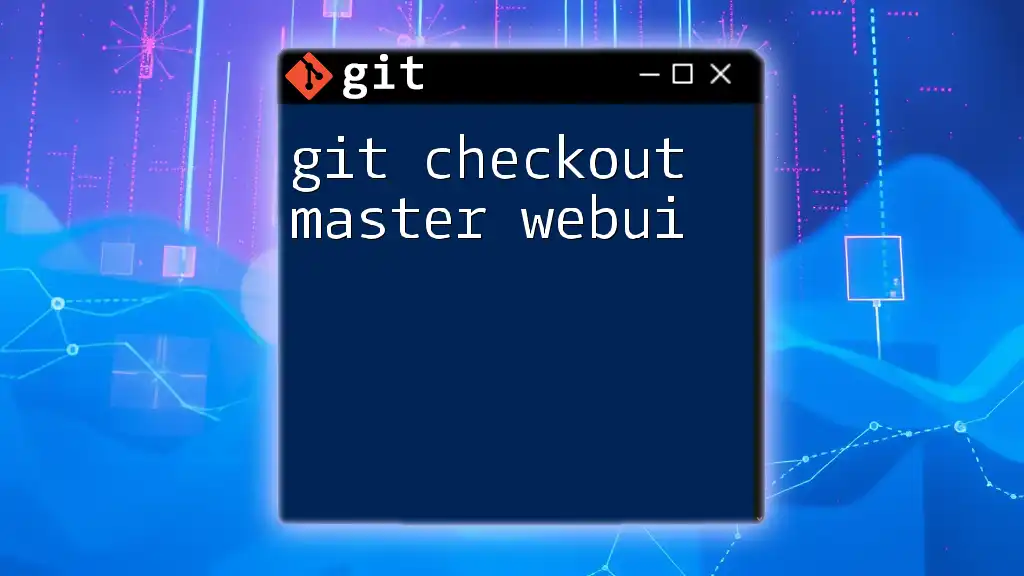
Overview of Git Checkout Flags
`-b` Flag
The `-b` flag is instrumental in creating a new branch and instantly switching to it. This single command integrates the workflow for new feature development. When you're ready to dive into a new feature, use:
git checkout -b featureX
This saves you time and helps in maintaining a well-organized branch structure.
`-f` Flag
The `-f` flag allows you to forcefully switch to a branch or file. This is particularly useful when your current branch has uncommitted changes. To switch to branch-name forcefully, you can use:
git checkout -f branch-name
This command forces Git to disregard any uncommitted changes, allowing you to proceed without worrying about losing your current work. However, be cautious—make sure you genuinely want to discard those changes.
`--detach` Flag
When you need to work with a specific commit rather than a branch, you can enter a detached HEAD state using the `--detach` flag. This allows you to experiment with your code without affecting any branches. To check out a specific commit, you can run:
git checkout --detach commit-hash
Working in a detached state can be useful for analyzing code or testing features without the risk of altering your main branches. Remember, though: if you create new commits in this state, they will not belong to any branch unless you switch back and create a new branch based on them.
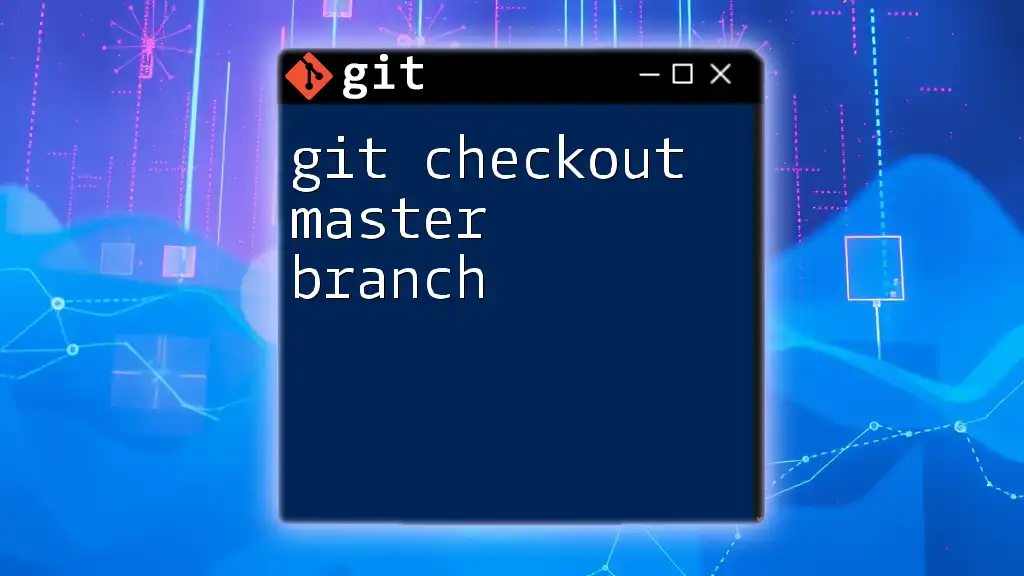
Understanding Other Flags
`--track`
The `--track` option is valuable when collaborating with remote repositories. By using this flag, you set tracking information for a new branch. To create a new branch that tracks a remote branch, execute:
git checkout --track origin/remote-branch
This establishes a relationship between your local branch and the corresponding remote branch, simplifying future pushes and pulls.
`--merge`
If you want to merge changes from one branch into your current branch without committing immediately, you can use the `--merge` flag. This gives you an opportunity to review changes before finalizing. To do so, run:
git checkout --merge branch-name
Using this command allows you to combine branches interactively, making it easier to handle conflicts or unexpected changes in the workflow.
`--no-track`
The `--no-track` flag is used to create a new branch without any associated tracking information. This can be useful when you don't want your new branch to be connected to a remote branch. For instance, to create a new branch without tracking, you can use:
git checkout --no-track -b standalone-branch
This allows you to work independently from the usual remote tracing mechanisms.
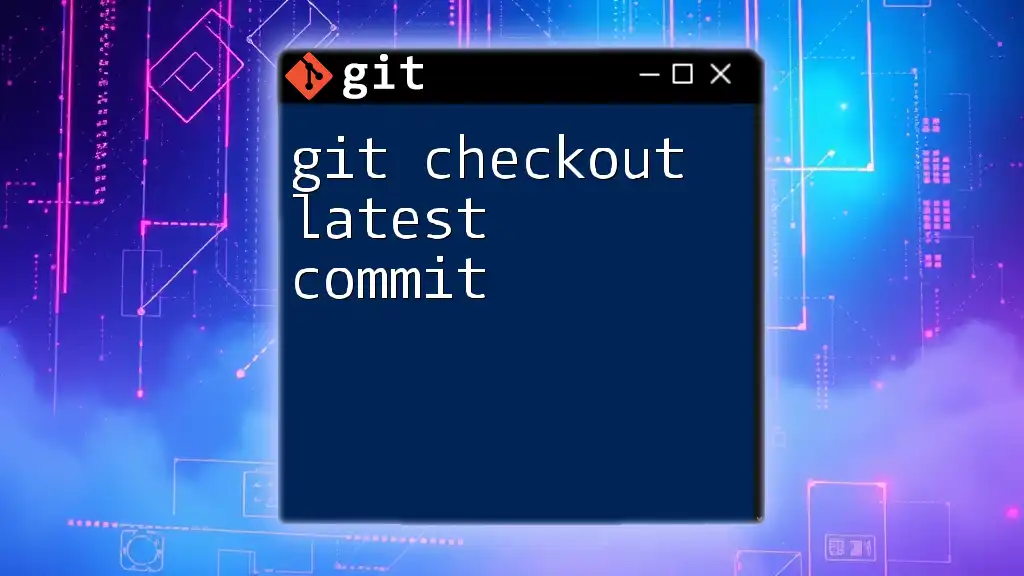
Practical Scenarios Using Checkout Flags
Case Study: Simplifying Workflow with Flags
Consider a developer juggling multiple feature branches while managing a codebase. By utilizing `git checkout flags`, the developer can streamline their workflow significantly. Here’s a potential scenario:
- Create a new feature branch: Use `git checkout -b new-feature` to start working on a new feature.
- Pull changes from a remote branch: Switch to the main branch with `git checkout main`, and pull the latest changes.
- Merge changes without committing automatically: After modifications, the developer can use `git checkout --merge feature-branch` to integrate changes before finalizing.
By efficiently using `git checkout flags`, developers can save time and maintain an organized workflow.
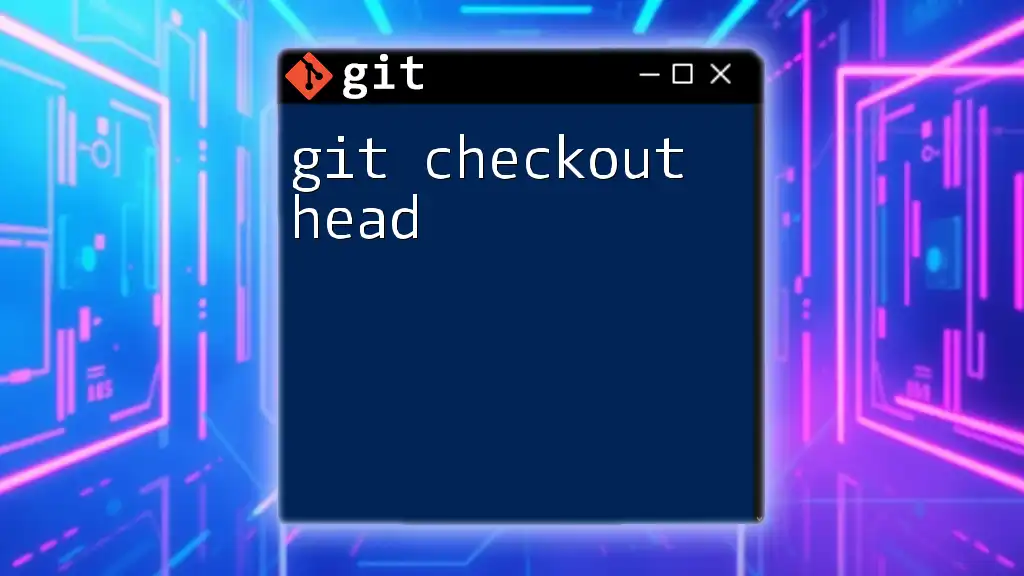
Common Pitfalls and Troubleshooting
Uncommitted Changes
When switching branches with uncommitted changes, Git will prevent you from checking out a different branch to safeguard your work. To resolve this, you might consider committing your changes or stashing them using `git stash` before switching branches.
Detached HEAD State
Operating in a detached HEAD state can be both a blessing and a risk. While it allows for experimentation, there's a chance of losing work if you forget to create a new branch for any commits made. Always keep track of your current state, and remember to use `git checkout -b new-branch` if you decide to keep your changes.
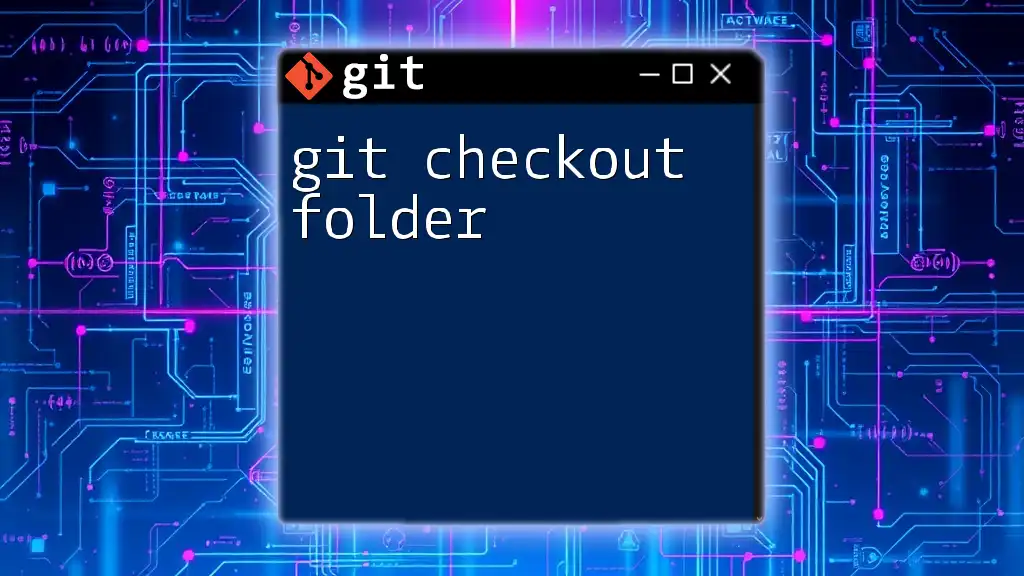
Conclusion
Understanding and mastering `git checkout flags` is essential for anyone looking to enhance their workflow in Git. By efficiently switching branches, restoring files, and managing remote tracking, you can significantly streamline your development process. Practicing these commands will not only boost your Git proficiency but will also make you more effective as a developer. Embrace the power of Git, and explore further resources for deepening your understanding of version control and collaboration.