You can create a new branch from an existing one and switch to it using the `git checkout` command with a different name by specifying the `-b` option followed by the new branch name.
git checkout -b new-branch-name existing-branch-name
What is git checkout?
`git checkout` is a versatile command in Git used primarily to switch between different branches or restore working tree files. It plays a crucial role in your version control workflow, allowing developers to navigate the project's history and manage different lines of development easily.
Common use cases for `git checkout` include:
- Switching to an existing branch.
- Creating a new branch and switching to it simultaneously.
- Restoring a file from a previous commit.
Understanding how to effectively utilize `git checkout` can significantly enhance your Git proficiency.
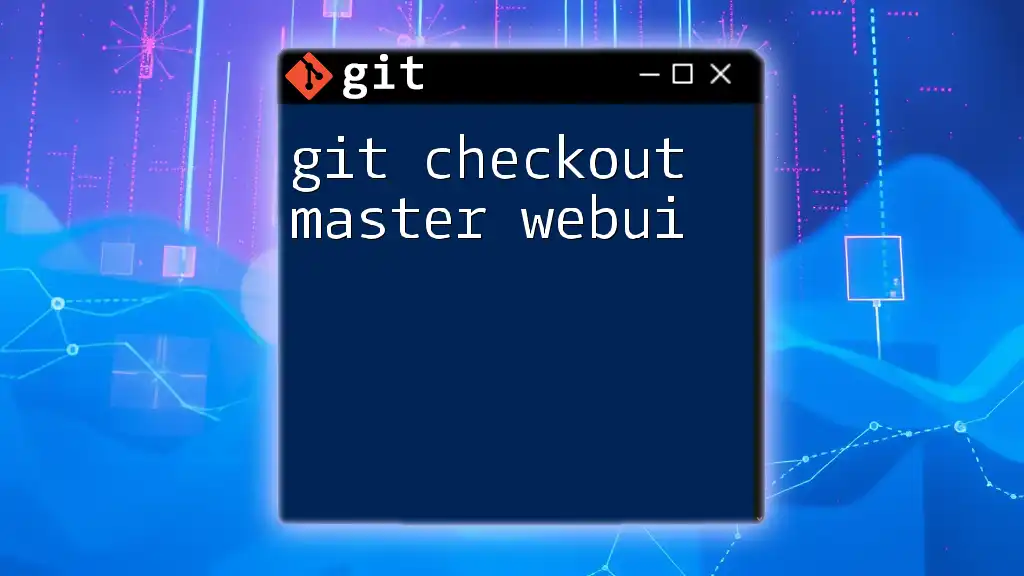
Understanding Branch Switching
Switching branches is one of the fundamental features of Git that allows developers to work on different tasks in isolation. The basic syntax for switching branches using `git checkout` is as follows:
git checkout <branch-name>
For instance, if you want to switch to a branch named `feature-xyz`, you would use:
git checkout feature-xyz
It's important to distinguish between local and remote branches during this process. If you attempt to switch to a branch that doesn't exist locally but does exist on a remote repository, you won't be able to switch until you create a local copy of it.
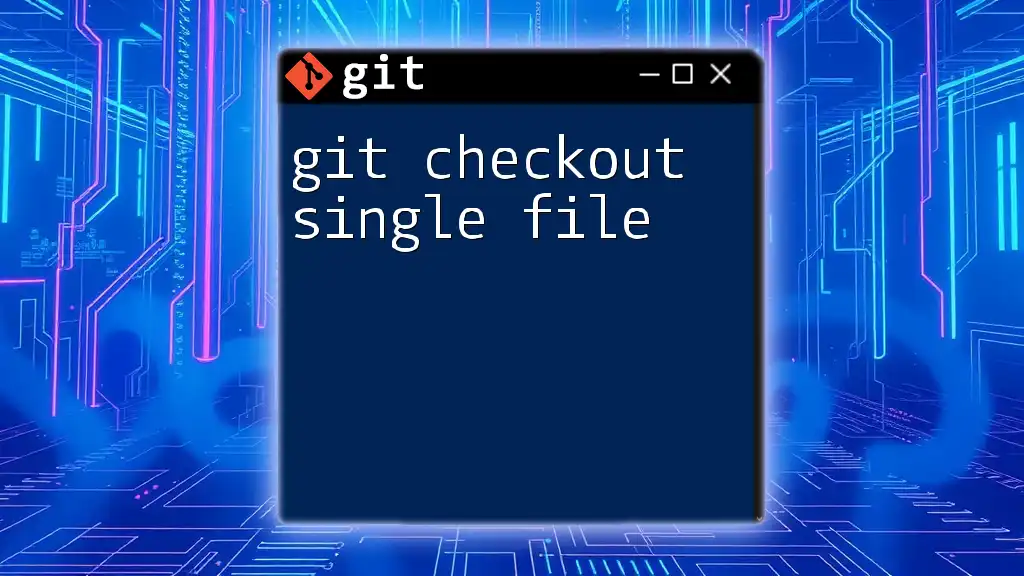
Creating a New Branch with a Different Name
Creating a new branch and checking it out in one step can streamline your workflow. The command to achieve this is:
git checkout -b new-branch-name
For example, to create and switch to a new branch called `enhancement/login`, you would execute:
git checkout -b enhancement/login
The `-b` option tells Git to create a new branch and switch to it immediately. This is highly efficient, as it saves you from executing two separate commands.
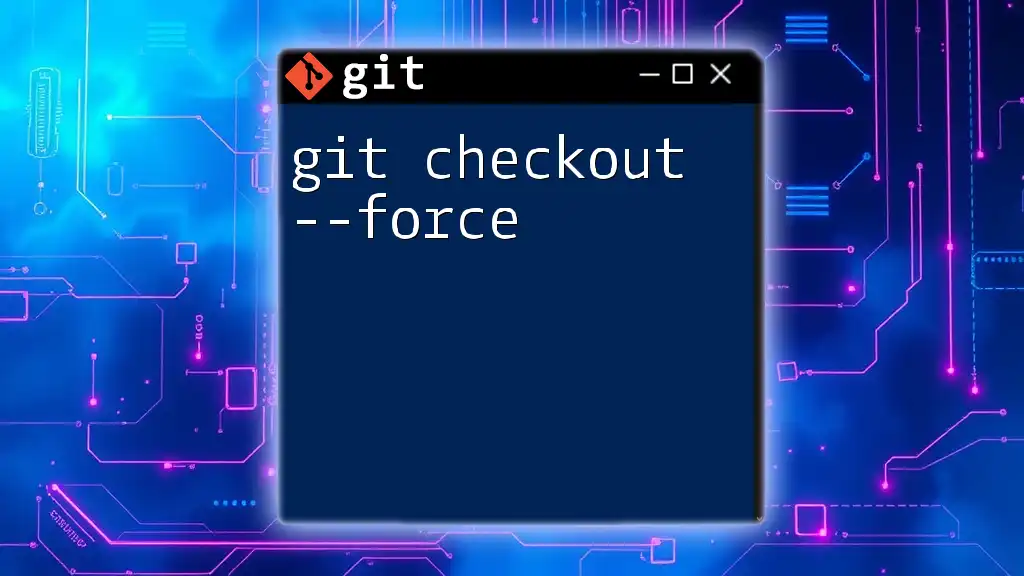
Checking Out a Branch Under a New Name
Sometimes, you may want to create a local branch based on a remote branch but give it a different name for clarity. This situation often arises when collaborating in a team environment. You can achieve this by using the following syntax:
git checkout -b local-branch-name origin/remote-branch-name
For instance, if you want to create a local branch named `feature-abc` from a remote branch called `feature-abc-remote`, you would run:
git checkout -b feature-abc origin/feature-abc-remote
This practice enables you to work on a specific feature without cluttering your local branch names with the remote naming conventions.
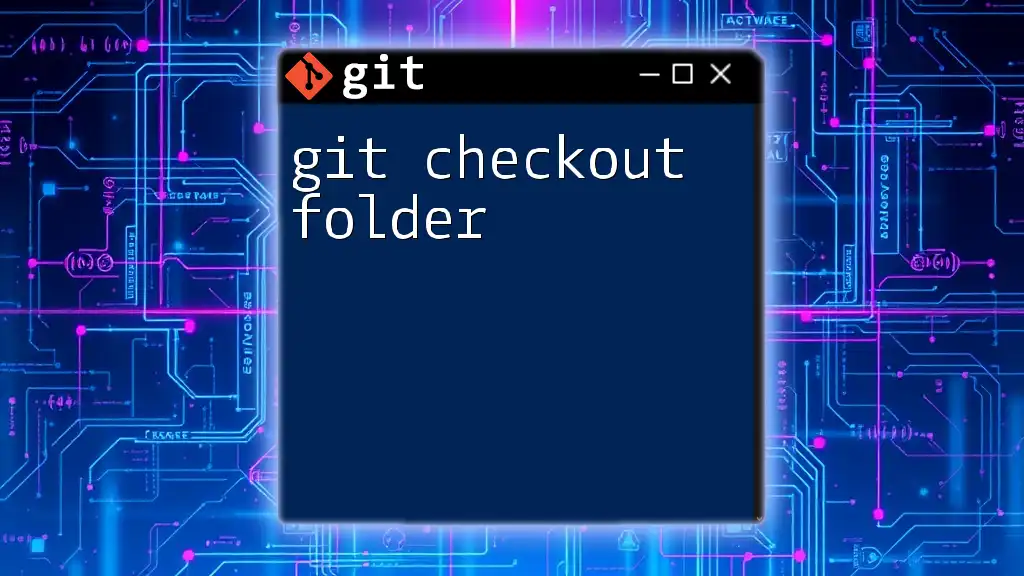
Renaming Branches with git checkout
Renaming an existing branch can be necessary as projects evolve. You can rename a branch using `git checkout` with the following syntax:
git checkout -b new-branch-name old-branch-name
For example, if you currently have a branch called `old-feature` and want to rename it to `updated-feature`, you would run:
git checkout -b updated-feature old-feature
Important Consideration: You cannot rename a branch while currently checked out to that branch. You must switch to another branch first (usually the `main` or `master` branch).
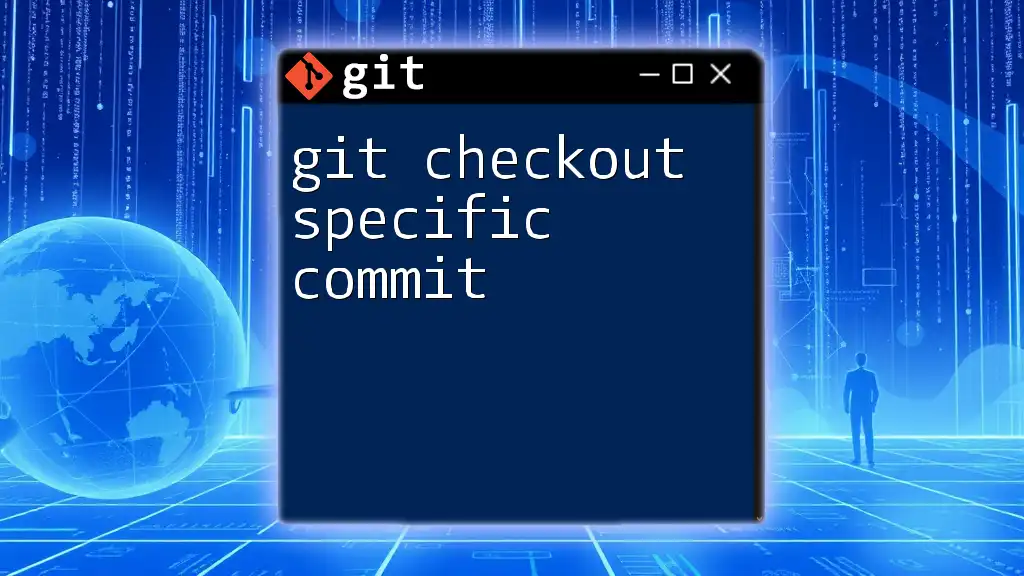
Using git checkout to Restore Files
Another powerful feature of `git checkout` is its capability to restore specific files from previous commits. This is particularly useful if you accidentally modified or deleted a file. The command structure for restoring a file is as follows:
git checkout <commit-hash> -- <file-name>
For example, if you wanted to restore a file called `app.py` from a commit with the hash `abc123`, you would execute:
git checkout abc123 -- app.py
This command retrieves the state of `app.py` from the specified commit, effectively rolling back any changes made to that file since then.
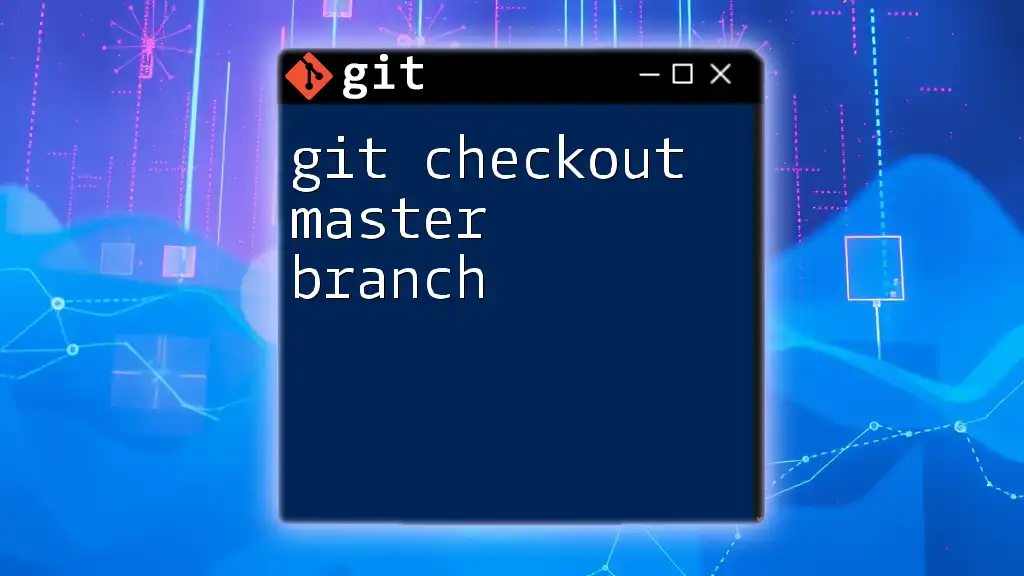
Common Issues and Troubleshooting
While using `git checkout`, you may encounter some common issues.
Branch Not Found Errors: If you attempt to check out a branch that does not exist, Git will return an error. Always double-check branch names and ensure they are available locally or on the remote repository.
Merge Conflicts During Checkout: If you have uncommitted changes that conflict with the branch you want to check out, Git will prevent the checkout. Stash or commit your changes before switching branches to avoid this issue.
Detached HEAD State: When you check out a specific commit instead of a branch, you enter a detached HEAD state. To resolve this, create a new branch from that commit to retain your changes:
git checkout -b new-branch-from-detached
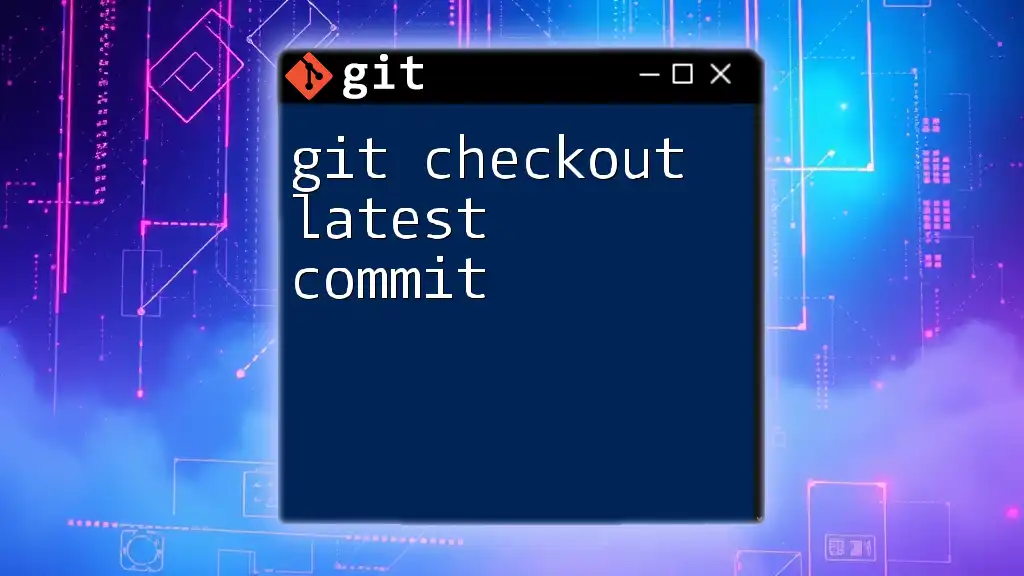
Useful Tips for Mastering git checkout
To master `git checkout`, consider the following best practices:
- Be intentional with branch names: Clear naming conventions prevent confusion and facilitate collaboration.
- Utilize `git switch`: The `git switch` command is a more user-friendly alternative for switching branches, designed to enhance usability.
- Leverage tab completion: Most terminal interfaces support tab completion for branch names, reducing typing errors.
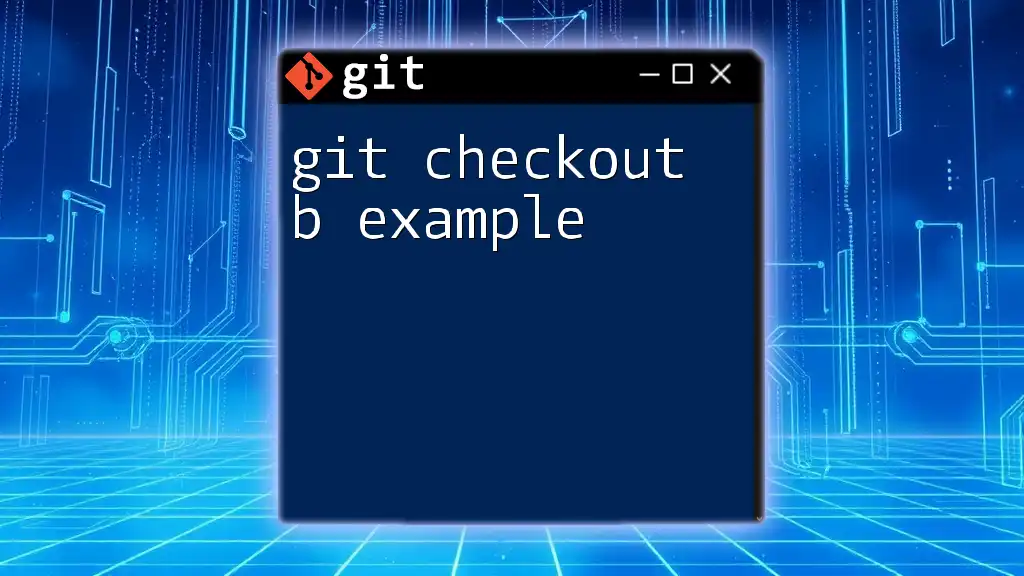
Conclusion
Understanding how to use git checkout as a different name enhances your Git skills and enables effective management of your development workflow. By mastering this command, you can streamline your branch management, enhance collaboration, and navigate your project's history with confidence.
Continue practicing these concepts to reinforce your understanding, and explore further resources to deepen your Git knowledge.