To restore a file from a previous commit in Git, you can use the command `git checkout <commit-hash> -- <file-path>`, replacing `<commit-hash>` with the hash of the desired commit and `<file-path>` with the path of the file you want to restore.
Here's how it looks in a code snippet:
git checkout abc1234 -- path/to/your/file.txt
Understanding Git Commits
What is a Git Commit?
A Git commit is a snapshot of your project's state at a specific point in time. It captures changes made to files and directories, along with a commit message that describes those changes. Each commit is unique and is identified by a commit hash, which is a string of characters that ensures the integrity of the changes.
When you create a commit, you include crucial information such as the author, timestamp, and a message explaining the purpose of the changes. This allows you to track the evolution of your project over time.
Viewing Commit History
To effectively navigate through your project's history, you can view the commit log using the `git log` command. This command provides a detailed list of commits in reverse chronological order.
To simplify the log output, you can use:
git log --oneline --graph
This displays the history in a compact format, showing each commit in a single line along with a graphical representation of branches and merges. Understanding how to interpret the output of `git log` is essential for finding the commit you want to revert to, as it will help you locate the specific commit hash associated with your changes.
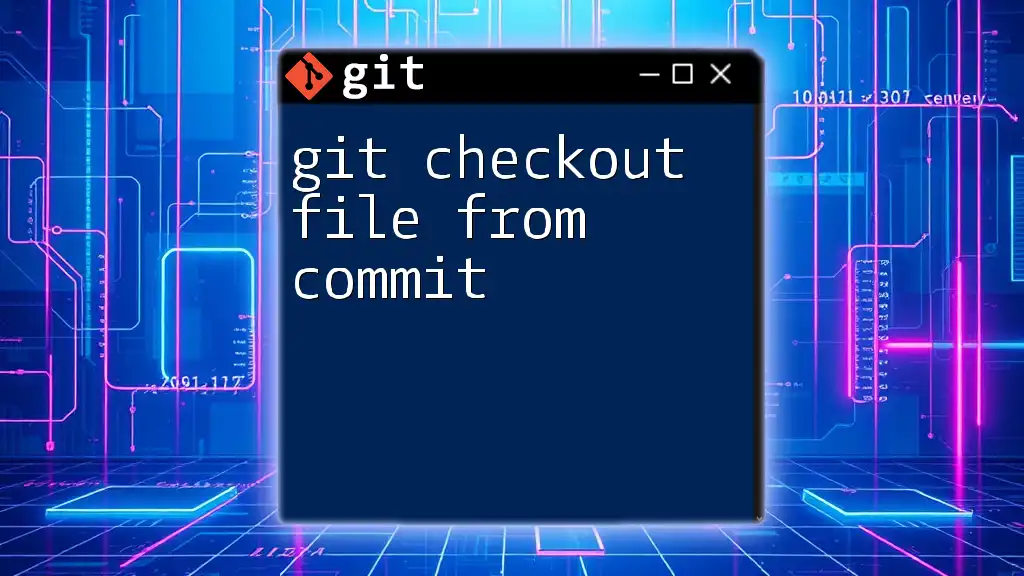
The Concept of Checkout
What Does `git checkout` Do?
The `git checkout` command serves multiple purposes in Git. At its core, it allows you to switch between different branches and restore files to a previous state. By checking out a file from a previous commit, you can revert that specific file without affecting the rest of the project.
Using `git checkout` can be likened to flipping through the pages of a book, where each page represents a different commit in your project’s history, allowing you to revisit and use the information contained in those snapshots.
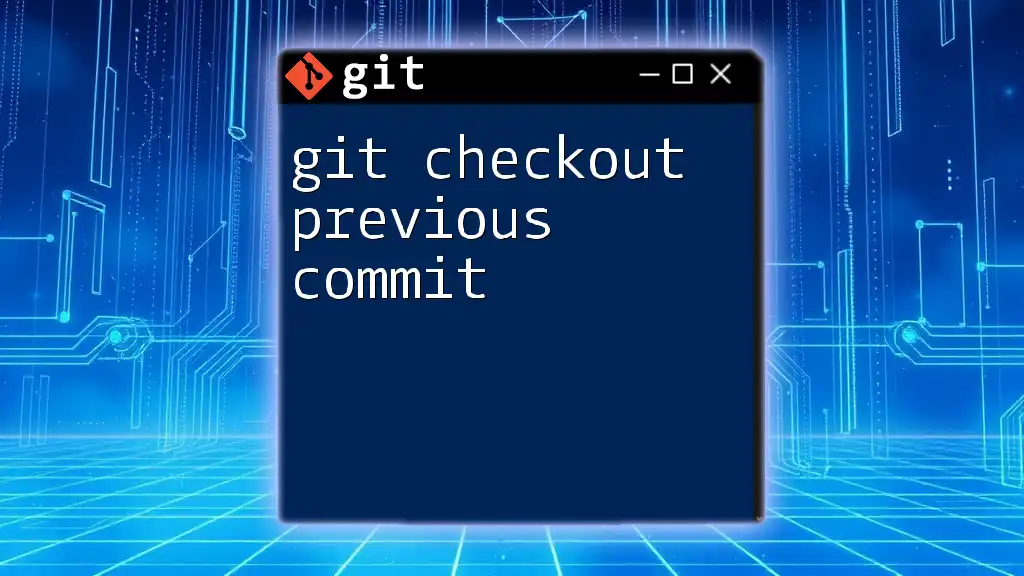
Checking Out a File from a Previous Commit
Syntax of the Checkout Command
The basic syntax for checking out a specific file from a previous commit is:
git checkout <commit_hash> -- <file_path>
- `<commit_hash>` refers to the unique identifier of the commit you wish to revert to.
- `<file_path>` is the path of the file you want to check out.
Finding the Commit Hash
To obtain the commit hash, you'll need to inspect your commit history. You can achieve this by running:
git log
As you scan through the log, look for the commit message and timestamp that corresponds to the state you want to restore. Each commit entry includes a unique hash, which you can copy for use in the checkout command.
Practical Example: Checking Out a File
Step-by-Step Walkthrough
-
View Commit History: Start by looking at your commit history to identify the commit that contains the version of the file you need.
git log
-
Use the Checkout Command: Once you find the desired commit hash (let's say it’s `a1b2c3d`), you can use the checkout command to restore a specific file:
git checkout a1b2c3d -- path/to/your/file.txt
-
Verify Changes: After executing the checkout command, you should verify that the file has changed to its previous state. One way to do this is by running:
git diff
This command will show you the differences between your working directory and the last commit, helping you confirm that the file has indeed reverted to the desired version.
Undoing a Checkout
If you realize that you’ve checked out the wrong file or want to return to the latest version of your project, you can undo your checkout. Simply execute:
git checkout HEAD -- <file_path>
This command restores the file to the most recent commit in your current branch, effectively undoing the changes made by the checkout process.
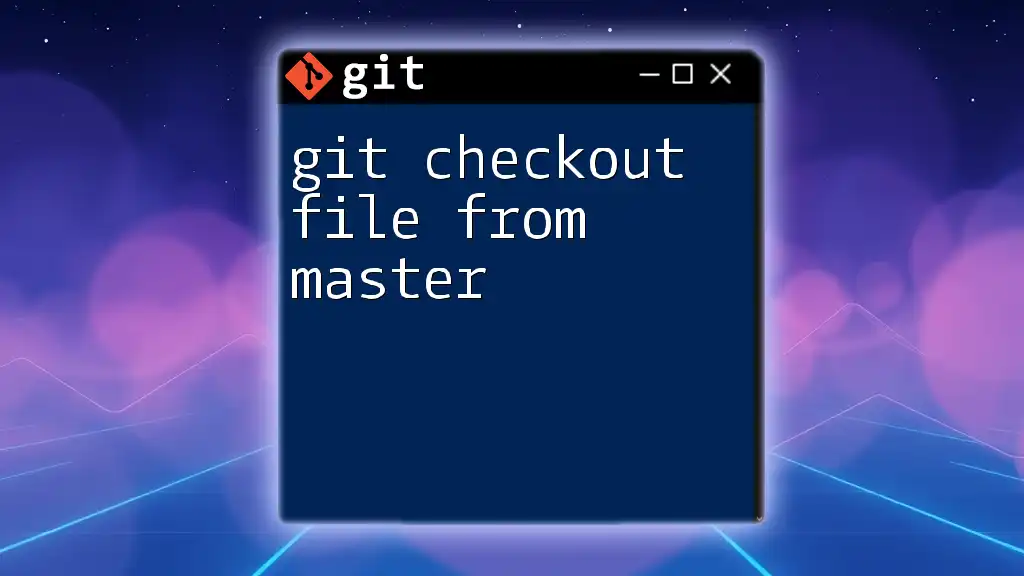
Best Practices for Using Checkout
Avoiding Common Pitfalls
While checking out files is a powerful feature, it comes with some risk. Always be cautious: if you check out a file, any unsaved changes in that file will be overwritten. To avoid data loss, consider committing your current changes or using `git stash` to temporarily save your work before proceeding.
Version Control Best Practices
- Regularly commit your changes: Frequent commits allow you to track your project effectively and revert to specific points in time without losing significant progress.
- Use meaningful commit messages: When creating commits, ensure to draft descriptive messages that accurately communicate what changes were made.
- Review changes before checkout: Always double-check which file you're about to replace with an older version, as this helps prevent unintended data loss.
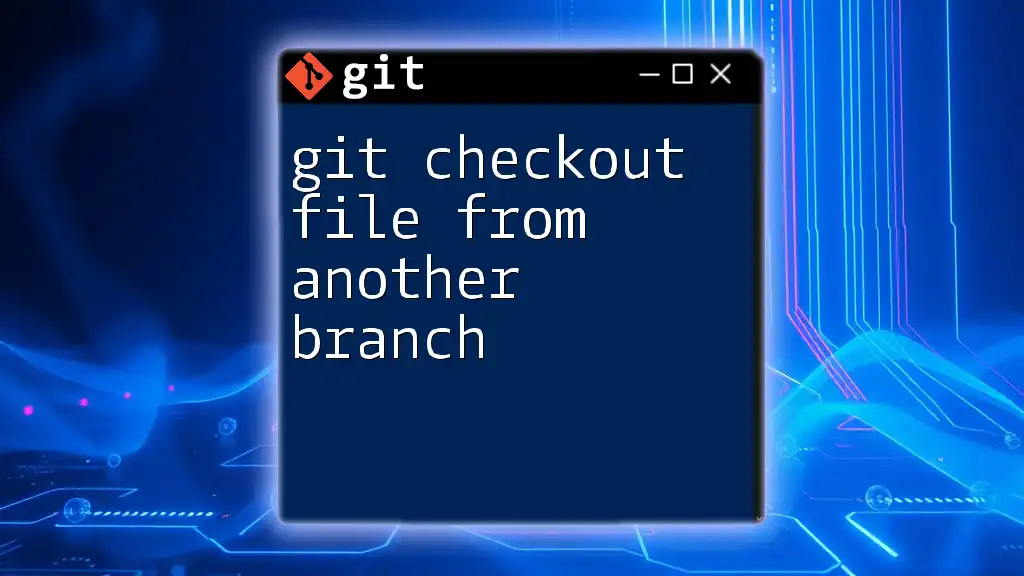
Conclusion
In this guide, we explored how to use the `git checkout` command to restore a file from a previous commit. You learned the significance of commits and the steps required to identify, select, and revert files. By integrating these practices into your workflow, you can efficiently manage the evolution of your projects and safeguard your work.
If you are serious about mastering Git, practice using `git checkout` regularly to familiarize yourself with the command and its implications. With time, you'll become more confident in navigating your project's history and utilizing Git's powerful features.
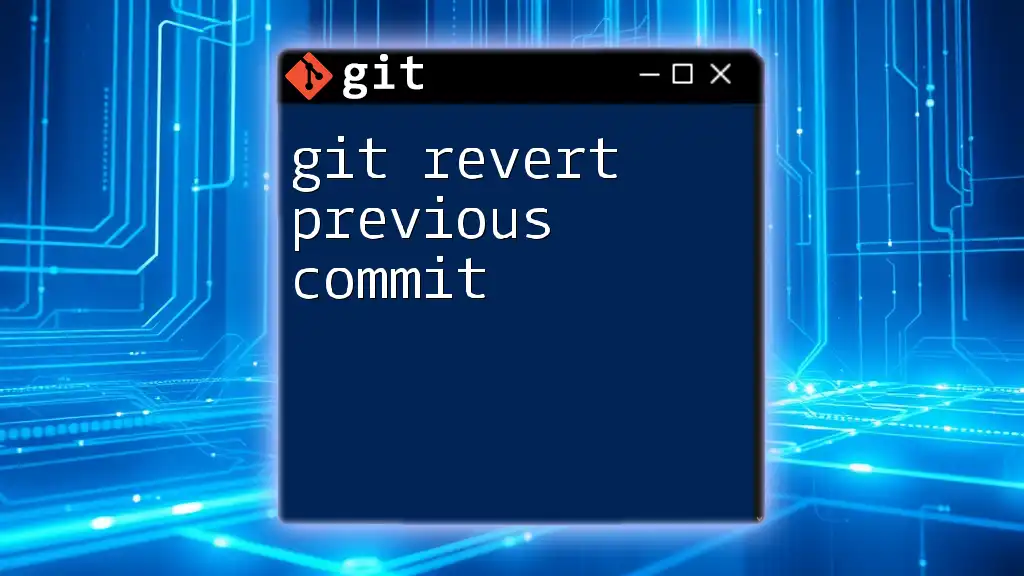
Additional Resources
Recommended Reading
- Git Documentation: Access the official Git documentation to deepen your understanding of commands and workflows.
- Online Courses: Consider taking an online course focused on Git to bolster your skills further.
FAQs
- What happens to the working directory when I checkout a file?
- The current version of the file is replaced with the version from the specified commit, resulting in all unsaved changes being lost.
- Can I checkout multiple files at once?
- Yes, you can specify multiple file paths in the `git checkout` command, separated by spaces.
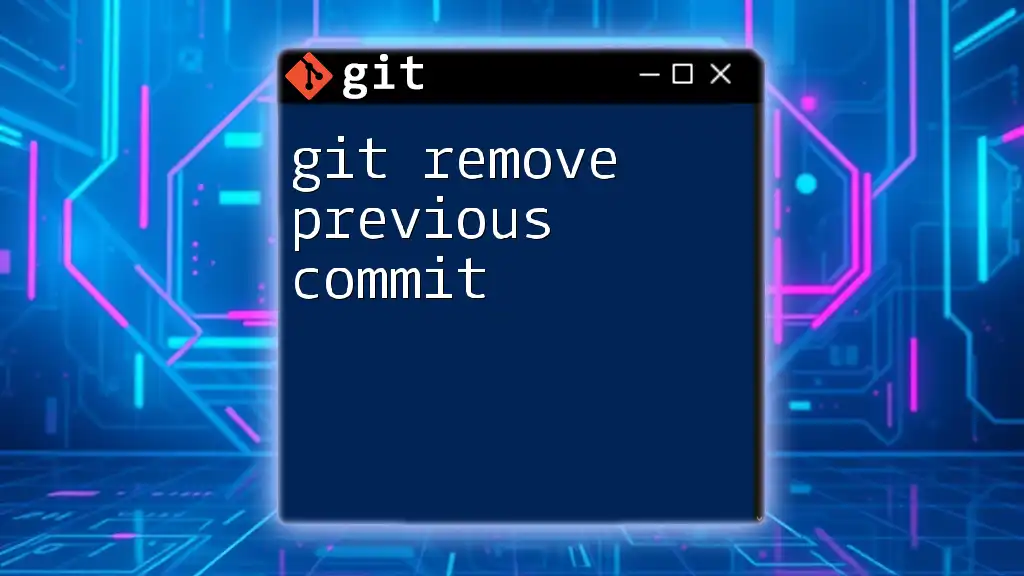
About the Author
A passionate developer and Git advocate, I am dedicated to simplifying complex workflows and helping others leverage version control to enhance productivity. My goal is to empower users to tackle their projects with confidence through practical guidance and clear insights.