You can sign the previous commit in Git by using the `--amend` option along with the `-S` flag to create a signed version of the latest commit. Here's how you can do it:
git commit --amend -S
Understanding Git Commit Signing
What is a Git Commit?
In Git, a commit is a snapshot of your changes. It serves as a marker in your project’s history. Each commit contains a unique ID, metadata (like the author and date), and the actual changes made to the files. Understanding how to create and manage commits is fundamental to using Git effectively.
What Does It Mean to Sign a Commit?
Signing a commit adds an additional layer of security by allowing authors to verify that the commit was indeed made by them and hasn’t been tampered with afterward. A signed commit uses GPG (GNU Privacy Guard) to encrypt the commit's metadata and ensures its authenticity, providing trust in collaborative environments.
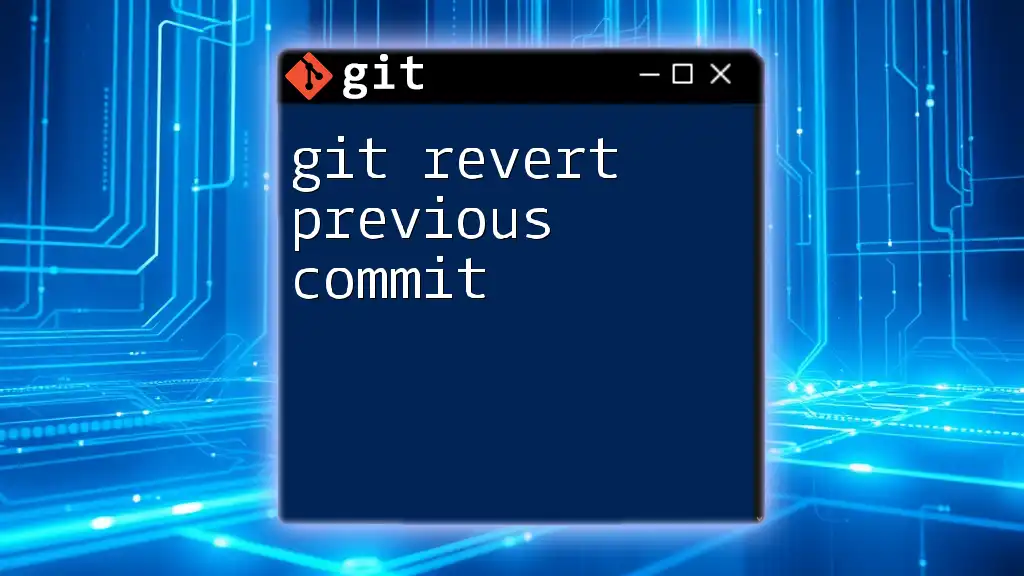
Prerequisites for Signing a Commit
Setting Up GnuPG
To sign commits in Git, you’ll need GnuPG. It is a tool for secure communication that allows you to generate private/public key pairs, which are essential for signing commits. Installation varies by operating system:
- Windows: You may install Gpg4win, which includes GnuPG, or use Windows Subsystem for Linux (WSL).
- macOS: You can install GnuPG using Homebrew with the command:
brew install gnupg
- Linux: GnuPG is typically pre-installed. If not, you can install it using your package manager:
sudo apt-get install gnupg
Generating a GPG Key
Once GnuPG is installed, you need to create a GPG key. Follow these steps:
- Open your terminal and run:
gpg --full-generate-key
- You’ll be prompted to select the key type; typically, the default (RSA and RSA) is sufficient.
- Choose a suitable key size (2048 bits or higher is recommended).
- Enter your identification information, like your name and email address.
- Finalize your key creation by entering a passphrase.
Configuring Git for GPG Signing
After creating your GPG key, you need to link it to your Git configuration. Use the command below, replacing `[YOUR_GPG_KEY_ID]` with the key ID you generated:
git config --global user.signingkey [YOUR_GPG_KEY_ID]
To make it easier, you can set Git to sign all commits by default with the following command:
git config --global commit.gpgSign true
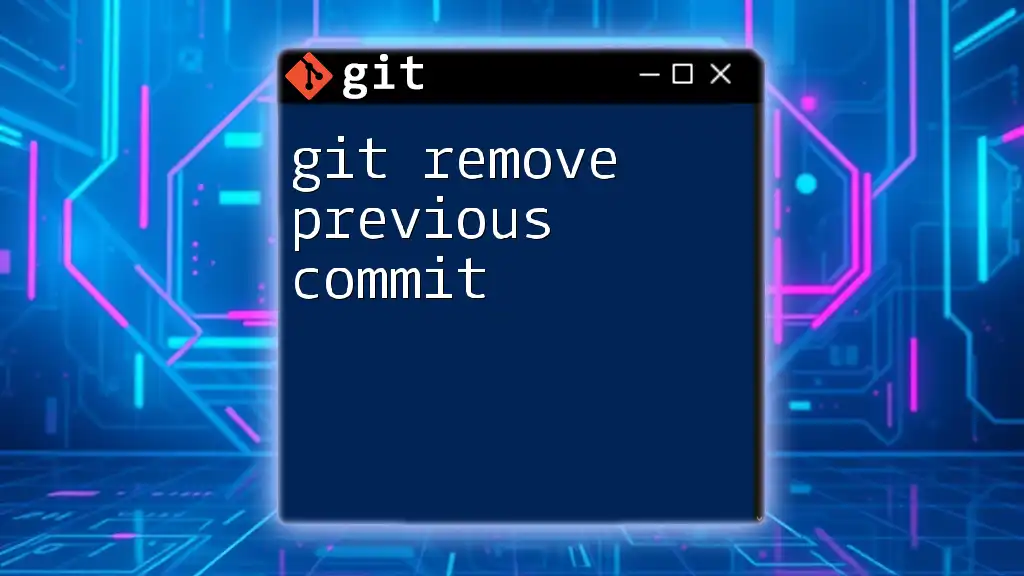
How to Sign a Previous Commit
Understanding the Process
Signing a previous commit involves modifying past commits to include your signature. This can be particularly useful if a commit was made without signing, and you want to correct that oversight to enhance the integrity of your project history.
Using `git commit --amend`
To sign the most recent commit, you can amend it. This is accomplished using the following command:
git commit --amend -S
The `-S` flag indicates that you want to sign the commit. This command opens your commit editor, allowing you to make adjustments to your commit message if needed before saving.
Signing a Specific Commit
Git Rebase
If you want to sign a specific commit further back in your history, you can use the rebase command. Here’s how to do it:
git rebase --exec 'git commit --amend -S --no-edit' -i [COMMIT_HASH]^
In this command, replace `[COMMIT_HASH]` with the hash of the commit you want to start with. This command will apply the signing operation to each commit while preserving the commit message.
Interactive Rebase
Another method involves using interactive rebase for finer control over which commits to sign:
- Start an interactive rebase with the following command:
git rebase -i [COMMIT_HASH]^
- In the text editor that opens, you’ll see a list of commits. Change the word `pick` to `edit` for the commit you wish to sign.
- When prompted during the rebase, run:
git commit --amend -S
- After signing, to continue the rebase, use:
git rebase --continue
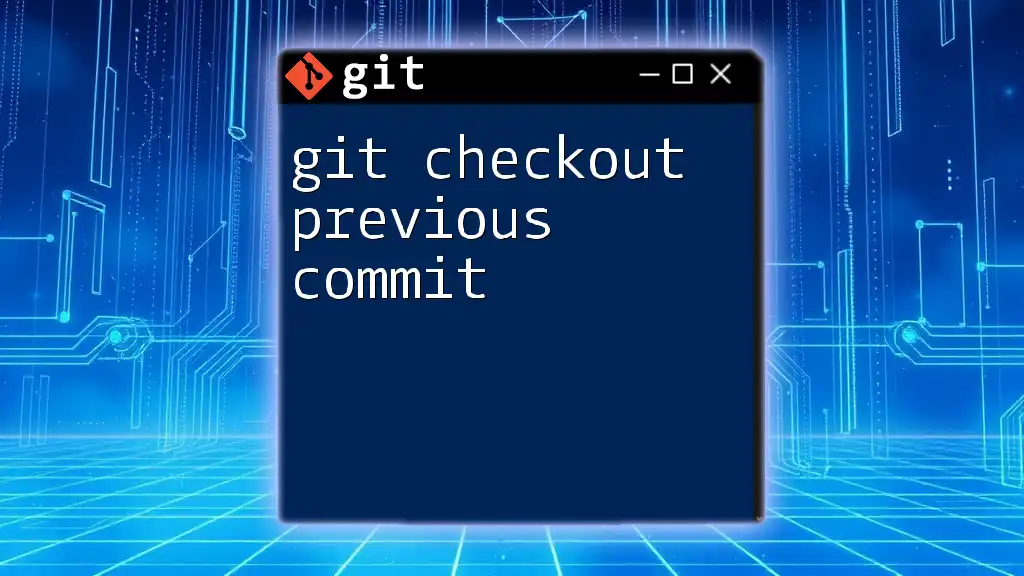
Verifying Signed Commits
To verify a commit’s signature, you can use the following command:
git verify-commit [COMMIT_HASH]
Replace `[COMMIT_HASH]` with the commit’s hash. Git will check the signature and return the status, confirming the authenticity of the commit.
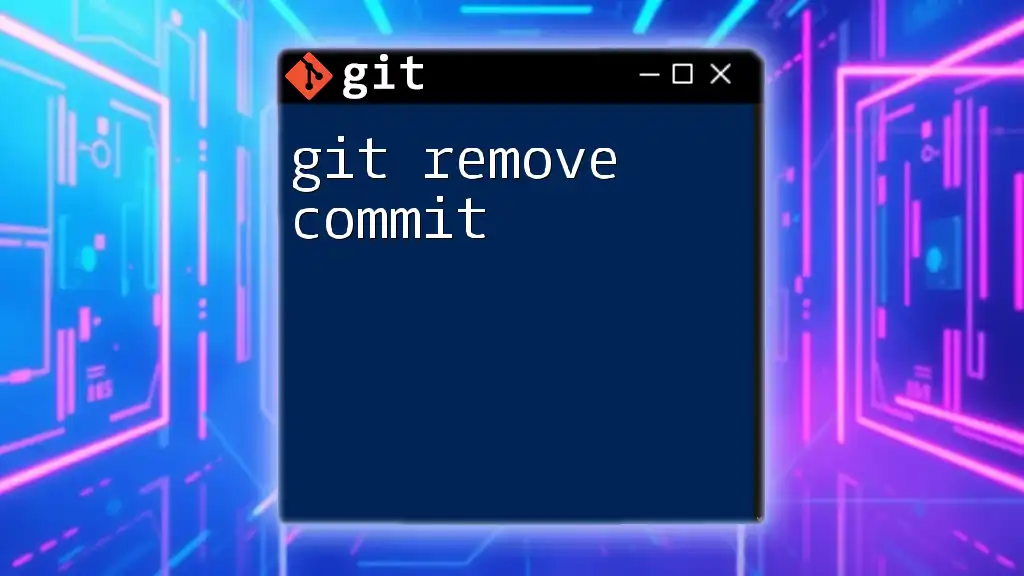
Best Practices and Tips
When to Sign Commits
It is a good practice to sign your commits, especially in collaborative and open-source projects. Signing provides assurance to others that your code is authentic and has not been altered maliciously.
Common Pitfalls and Troubleshooting
Whenever you encounter issues, such as a GPG agent not functioning or a signature verification failure, ensure that your GPG key is correctly linked in your Git configuration. Also, verify that your GPG agent is running, which can often resolve signature issues quickly.
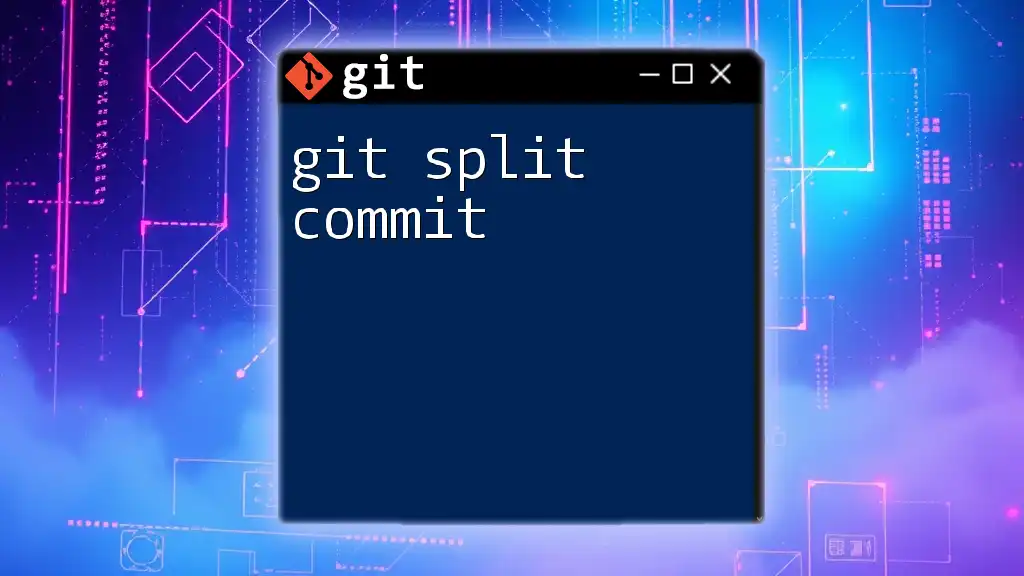
Conclusion
Signing your Git commits enhances the security and authenticity of your project history. By following the steps outlined in this guide, you'll not only learn how to implement signing but also understand its importance in maintaining the integrity of your code. Start signing your commits today to cultivate a trusted and verifiable development process!
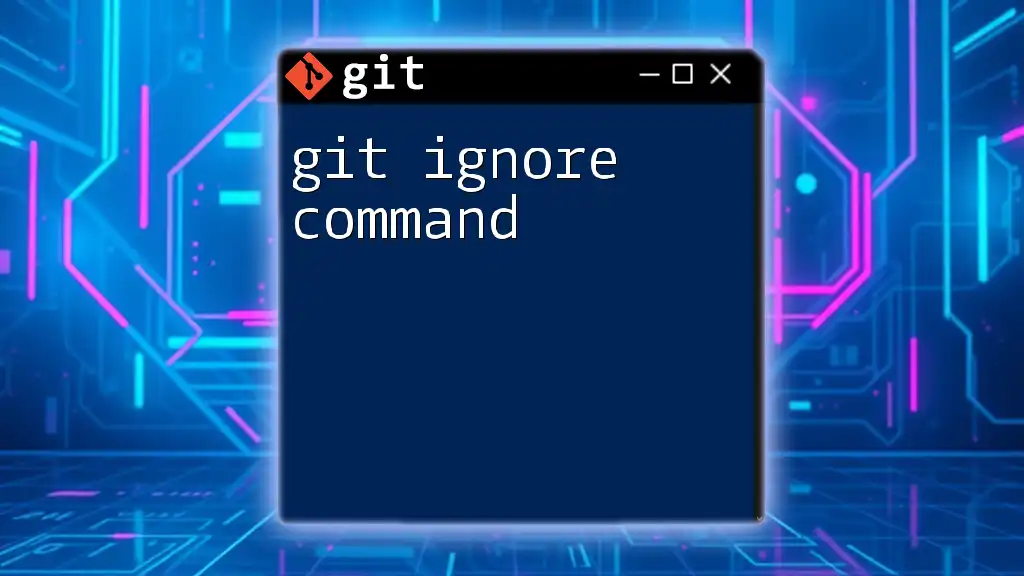
Additional Resources
- For further reading, refer to the [official Git documentation on commit signing](https://git-scm.com/book/en/v2/Git-Tools-Signing-Your-Work).
- Learn more about GnuPG in the [GnuPG official documentation](https://www.gnupg.org/documentation/).
- Explore additional Git processes and workflows to enhance your Git proficiency.