To delete a branch in Git, use the command `git branch -d <branch-name>` for a safe delete, or `git branch -D <branch-name>` to forcefully delete a branch that hasn't been merged.
git branch -d <branch-name>
# or
git branch -D <branch-name>
Understanding Git Branches
What is a Git Branch?
A branch in Git is essentially a separate line of development. It allows developers to work on different features, bug fixes, or experiments without affecting the main codebase. This is crucial in a collaborative environment where multiple team members may be working on various parts of a project simultaneously. A branch serves as an isolated environment where changes can be made, tested, and reviewed before being merged back into the main code.
Types of Branches in Git
Git branches can be categorized mainly into two types: Local Branches and Remote Branches.
Local Branches
Local branches exist only on the user's machine. They are used for development purposes, allowing individual developers to create and modify branches for their own tasks. Once a feature is completed, the branch can be merged into the main branch (usually `main` or `master`) or deleted if not needed.
Remote Branches
Remote branches are found on the remote repository and are used to maintain a history of changes that may have been shared amongst different collaborators. These branches represent the various states of the project as seen by different users.
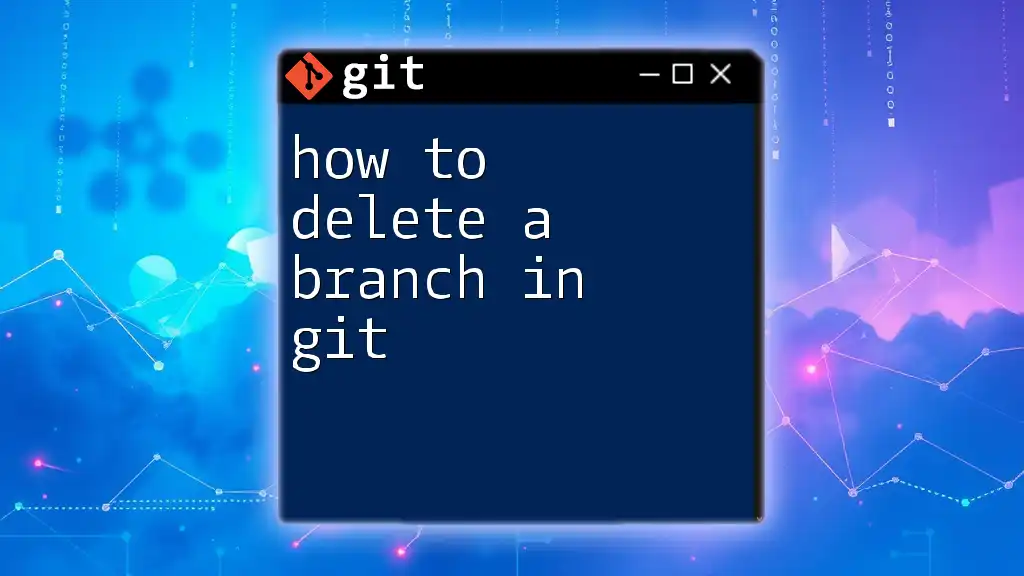
When to Delete a Branch
Common Scenarios for Deleting a Branch
Understanding when to delete a branch is vital for maintaining a clean and efficient Git workflow.
Merged Branches
Once a branch has been successfully merged into the main branch, it is often safe to delete it. This helps to keep the branch list uncluttered and prevents confusion about which branches are still active.
Stale Branches
Occasionally, branches may become obsolete due to changes in project direction or the completion of specific tasks. Deleting these stale branches is an important step in ensuring good repository hygiene.
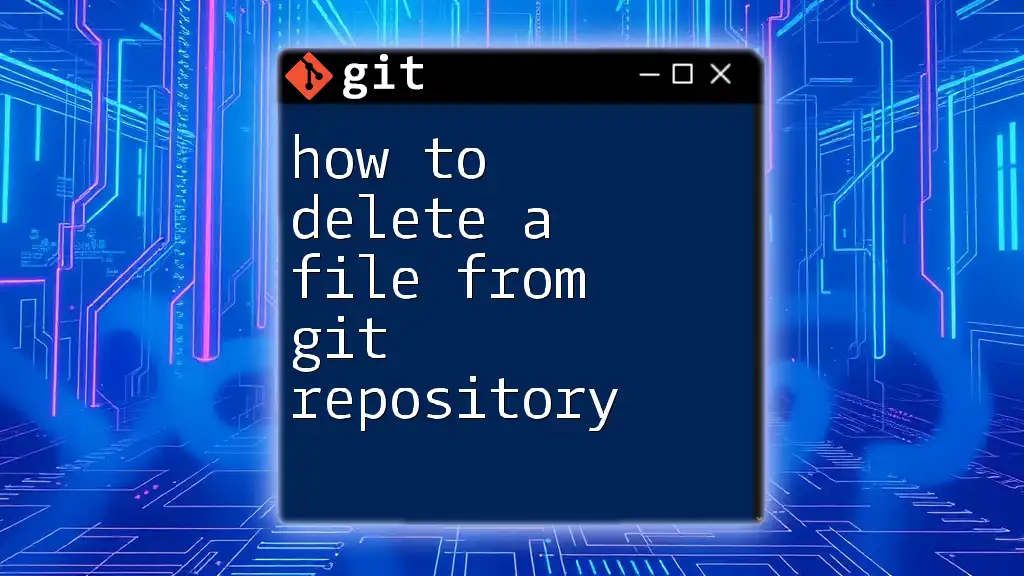
Steps to Delete a Branch
Deleting a Local Branch
To delete a local branch that you no longer need, you can use the Git command-line interface. The command to delete a local branch is as follows:
git branch -d <branch-name>
For example, to delete a branch called `feature/awesome-feature`, you would run:
git branch -d feature/awesome-feature
This command uses the `-d` flag, which stands for "delete". It ensures that you can only delete branches that have been successfully merged, preventing accidental loss of unmerged work.
Forcing Deletion of a Local Branch
Sometimes, you may find yourself needing to delete a branch that hasn't been merged yet. In this case, you can force the deletion with the `-D` flag:
git branch -D <branch-name>
For instance, if you want to delete a branch called `feature/broken-feature`, you would execute:
git branch -D feature/broken-feature
Be cautious when using this command, as it permanently removes the branch and its unmerged changes, making recovery difficult or impossible.
Deleting a Remote Branch
Deleting remote branches is a little different than deleting local ones. This step is crucial for maintaining an up-to-date project environment. You can delete a remote branch using the following syntax:
git push <remote-name> --delete <branch-name>
For example, to delete a remote branch named `feature/old-feature` on the `origin` remote, the command would look like this:
git push origin --delete feature/old-feature
This command signals to the remote repository to remove the specified branch. It's an essential task to keep your remote repository clean and relevant.
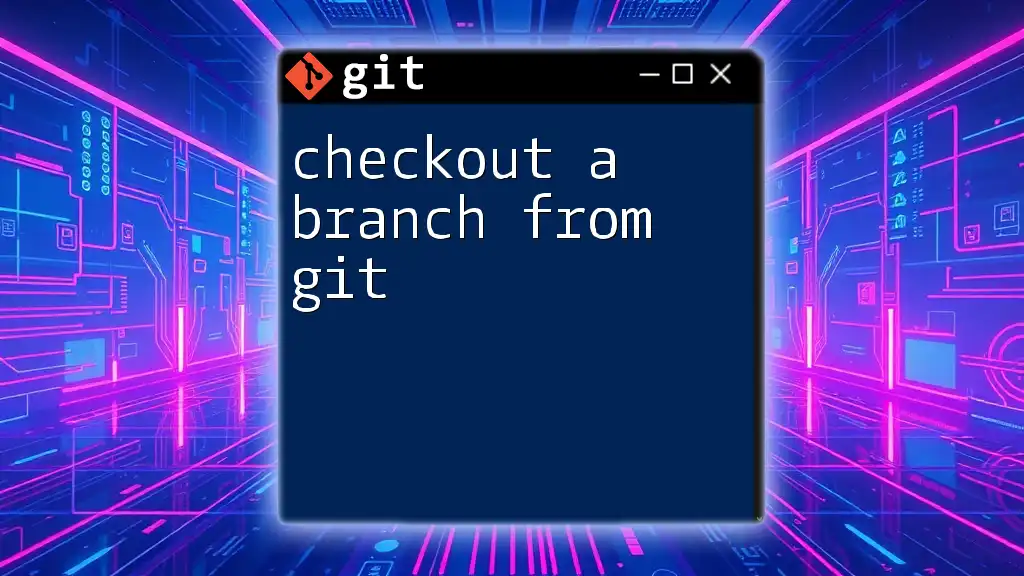
Best Practices for Deleting Branches
Regular Cleanup of Branches
Make it a habit to regularly review and clean up branches that are no longer needed. This not only declutters your Git environment but also minimizes confusion among team members.
Using Branch Naming Conventions
Implementing a consistent naming convention for branches can help keep your repository organized. For example, prefixes like `feature/`, `bugfix/`, or `hotfix/` can indicate the purpose of a branch, making it easier to identify which branches can be deleted after their corresponding changes have been merged.
Documenting Deletions
It's advisable to document branch deletions in project management tools or communication platforms used by your team. This ensures everyone is informed about which branches have been deleted and can prevent misunderstandings.
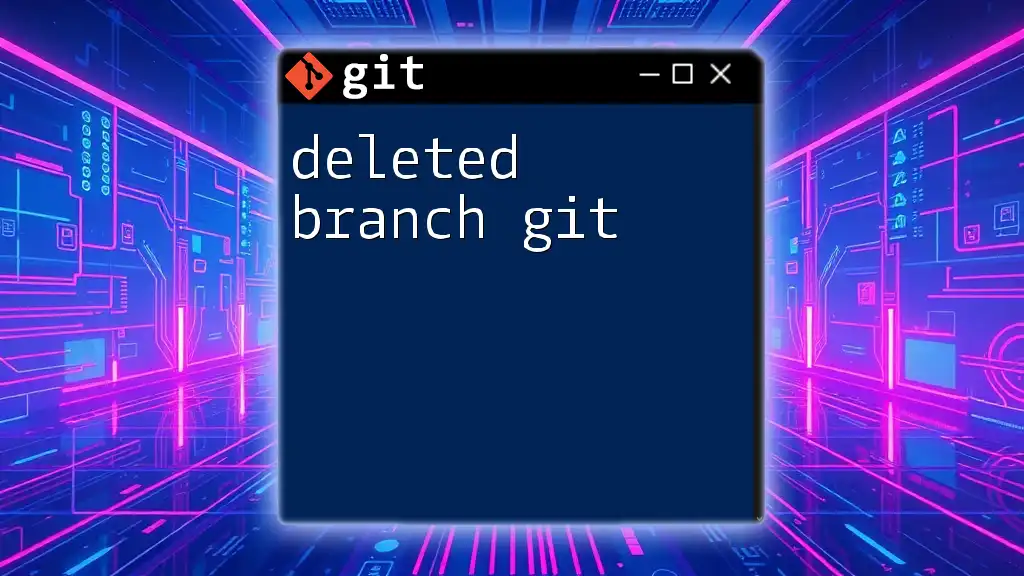
Common Mistakes to Avoid
Deleting Unmerged Branches
A common mistake among developers, especially those new to Git, is deleting branches that have unmerged changes. Always double-check if a branch has been merged into the main codebase before executing a deletion command.
Confusing Local with Remote Branch Deletion
Understand the difference between local and remote branch deletion. Deleting a local branch does not remove it from the remote repository and vice versa. Being clear on this distinction helps prevent accidental deviations in team workflows.
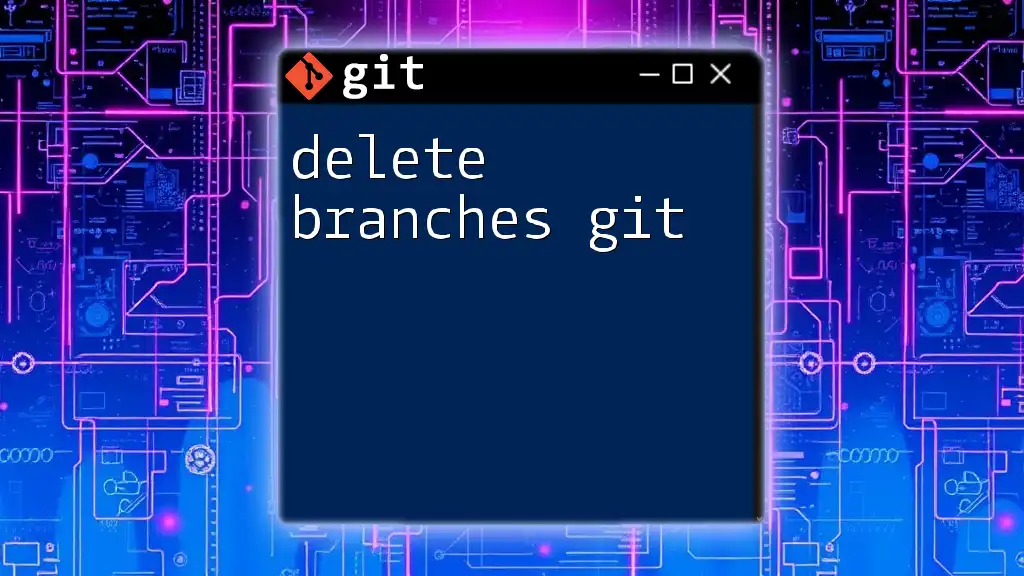
Conclusion
Managing Git branches effectively is crucial for any development team. Knowing how to delete a branch, whether local or remote, is a skill that supports maintaining a cleaner workspace and more efficient collaboration. Taking the time to manage your branches responsibly can lead to significant improvements in your Git workflow. Remember to regularly review your branches, implement consistent naming conventions, and document your deletions to keep everything organized. With the steps outlined in this guide, you're now equipped to master the essential skill of how to delete a branch from Git.