To restore a file in Git to its last committed state, you can use the following command:
git restore <file>
Understanding Git States
What Are Git States?
Git operates with files across different stages: the Working Directory, the Staging Area, and the Repository. Understanding these states is crucial when you need to restore a file in Git.
-
Working Directory: This is where you are actively editing your files. Changes made here are not yet tracked or saved for future commits.
-
Staging Area: Also known as the index, this is where you place files that you intend to commit. It's like a preview of what will be included in your next commit.
-
Repository: This is the stored history of your project, which contains all the commits and their respective states.
Importance of Knowing the Current State
Being aware of which state you are in has a direct impact on your ability to restore files. If you don’t know whether a file is in the Working Directory or Staging Area, you may choose the wrong command and end up creating confusion.
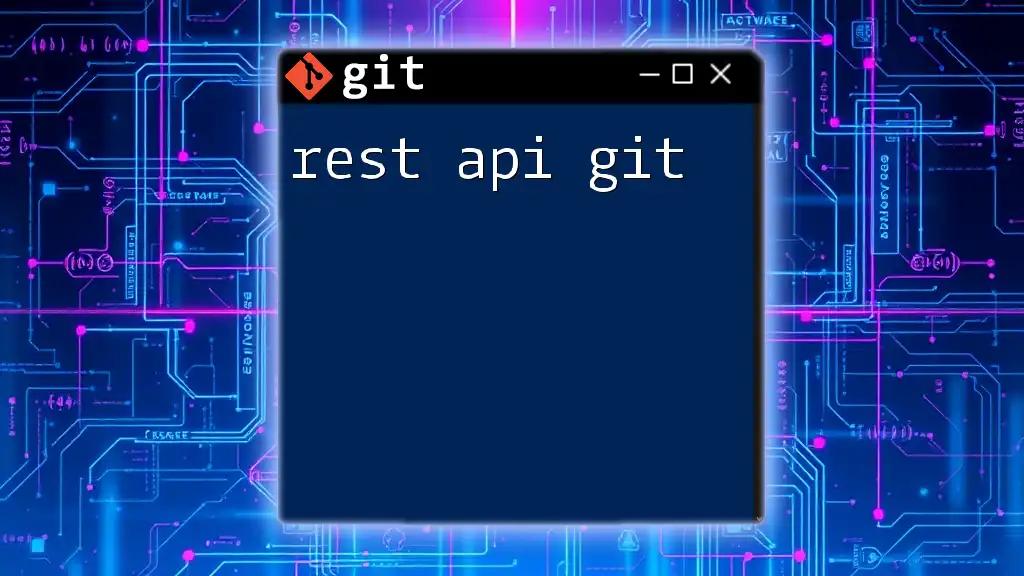
Common Scenarios for Restoring Files
Accidental Deletion of Files
It’s common to accidentally delete files when working on a project. Knowing how to restore these files can save a lot of time and frustration.
Reverting Changes to a Previous Version
Sometimes, changes made to files introduce unwanted behavior. Restoring files to an earlier version allows you to revert to a known good state.
Restoring Files from Uncommitted Changes
If you've modified a file but haven't committed the changes, you might want to discard those modifications. This can happen when a change doesn't work out, and you need the original file back.
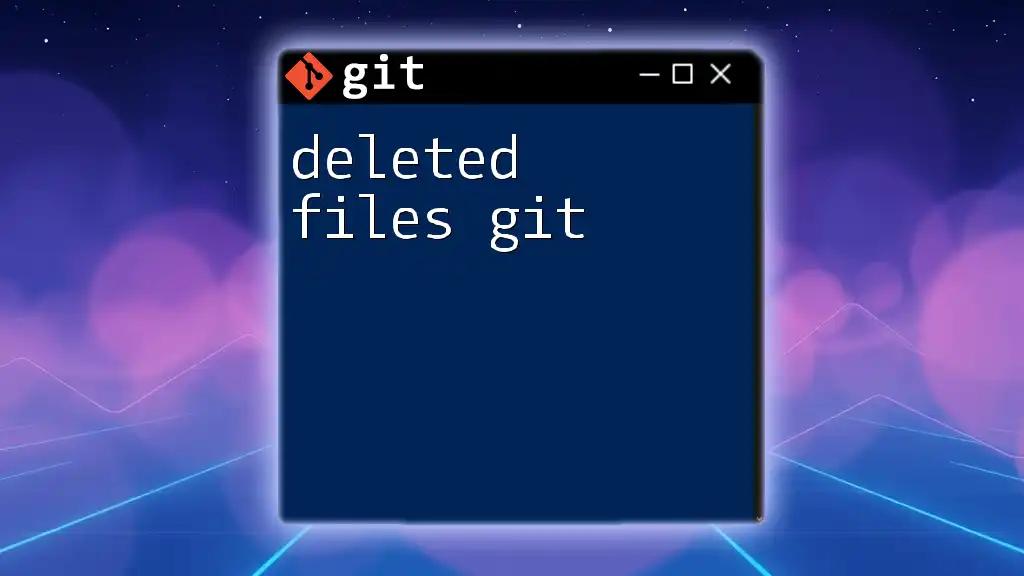
Methods to Restore Files in Git
Restoring a File from the Staging Area
If a file has been staged but not yet committed, you can unstage it using the following command:
git restore --staged <file>
This command essentially moves the file from the Staging Area back to the Working Directory, allowing you to make further modifications or discard changes entirely.
Restoring a File from the Working Directory
If you want to discard all changes made to a file since the last commit, restoring it is as simple as running:
git restore <file>
This command will replace the file in your Working Directory with the last committed version, effectively erasing any unsaved changes you've made.
Recovering a File from a Specific Commit
To restore a file to the state it was in at a specific point in time, you can use the `checkout` command:
git checkout <commit_hash> -- <file>
You can find the commit hash by checking your commit history with `git log`. This method allows you to pull any version of the file that exists in your project’s history.
Using `git reflog` to Recover Deleted Files
In instances where you deleted a file unintentionally, you can track your recent actions with `git reflog`.
git reflog
This command provides a history of all the references (or actions) in your Git repository. By examining this output, you can find the commit prior to the deletion and restore the file by checking it out or resetting to that state.
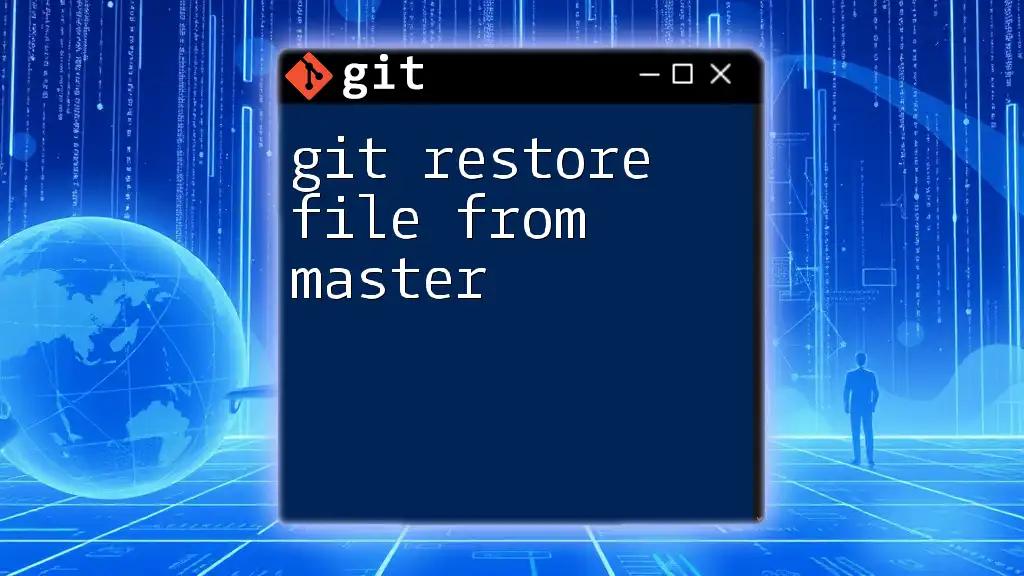
Practical Examples
Scenario 1: Recovering a Deleted File
Suppose you accidentally deleted `example.txt`. You can restore it quickly using the command:
git restore example.txt
This returns the file to your Working Directory with its last committed state, saving valuable time.
Scenario 2: Reverting File Changes after a Pull
You’ve just pulled updates from a remote repository, and a file you are working on has conflicted changes. To revert it to how it was before the pull, you can restore it using:
git restore <file>
This effectively discards the unwanted changes while retaining your last committed work.
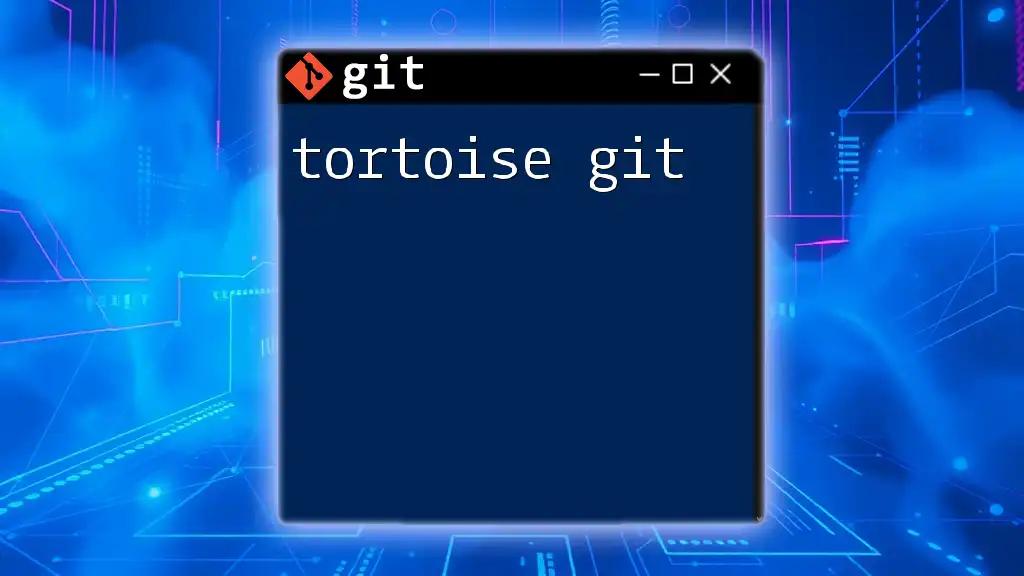
Tips and Best Practices
Always Make Regular Commits
Regular commits not only document your progress but also provide more restore points. The more frequently you commit, the easier it will be to revert to a specific version if needed.
Utilizing Branches
Branching is a powerful feature in Git that allows you to work on new features without disrupting the main codebase. By keeping changes isolated on a separate branch, you minimize the risk of losing important work.
When to Use `reset` vs. `restore`
It’s important to know when to use `git reset` versus `git restore`. Use `reset` for rolling back commits or un-staging files, while `restore` is ideally suited for recovering files to their last committed state in the Working Directory or Staging Area.
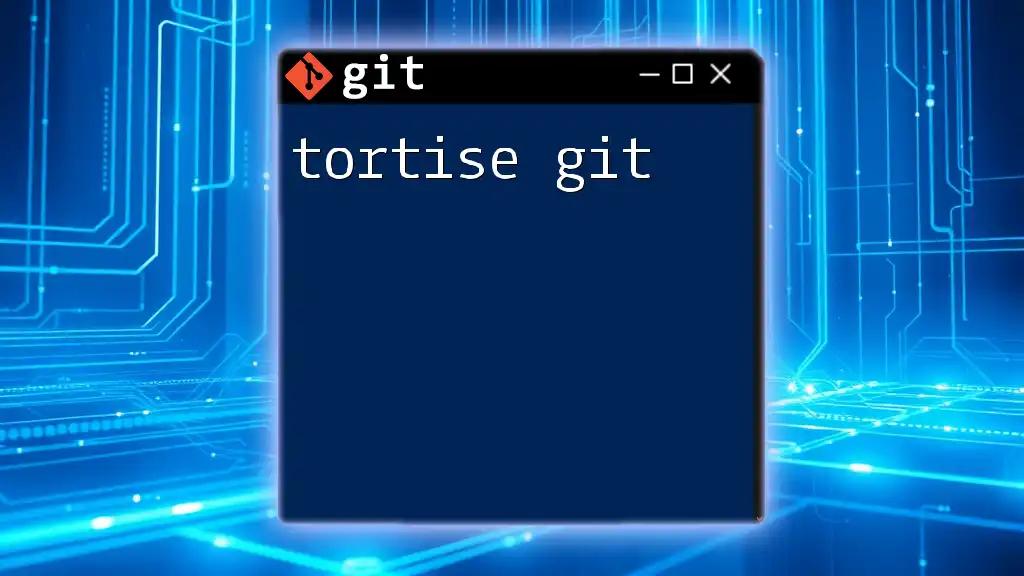
Conclusion
Understanding how to restore a file in Git is essential for effective version control. Knowing which commands to use in various scenarios can save time and help maintain the integrity of your code. Practice these methods in a safe environment to build your confidence and proficiency in handling file restorations in Git. Being adept in these techniques greatly enhances your ability to manage and recover lost work efficiently.
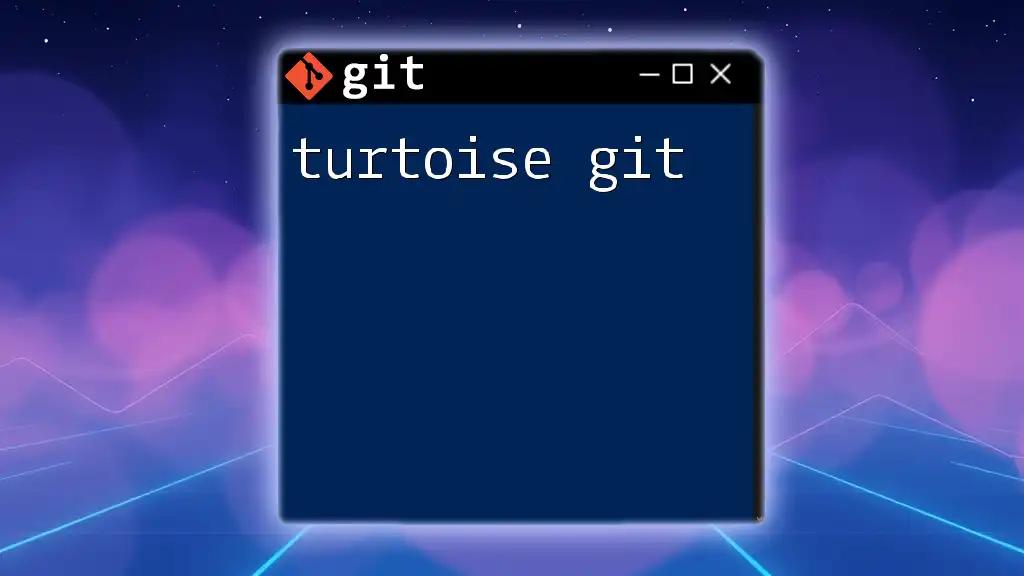
Additional Resources
To further deepen your understanding of Git and its commands, refer to the official [Git documentation](https://git-scm.com/doc). Additionally, consider using graphical user interfaces (GUIs) for Git like GitKraken or SourceTree, which can simplify many of these processes.