To remove all changes in Git and revert the working directory to the last committed state, use the following command:
git checkout -- .
Understanding Your Current Git State
Before you take action on how to remove all changes in Git, it’s crucial to understand the current state of your repository.
Checking the Status
The first step is to see what changes exist in your working directory. Use the following command:
git status
This command gives you a clear overview of what has been modified, what is staged for commit, and what is untracked. Changes will typically fall into one of three categories:
- Staged: Changes that have been added and are ready to commit.
- Unstaged: Changes that haven’t been added to the staging area yet.
- Untracked: New files that Git is not tracking yet.
Identifying Changes
Recognizing the distinctions between tracked and untracked changes is vital. Tracked files are those that Git already manages and can be reverted to their last committed state. Untracked files, however, need to be explicitly managed, as they do not have previous versions saved in your Git history.
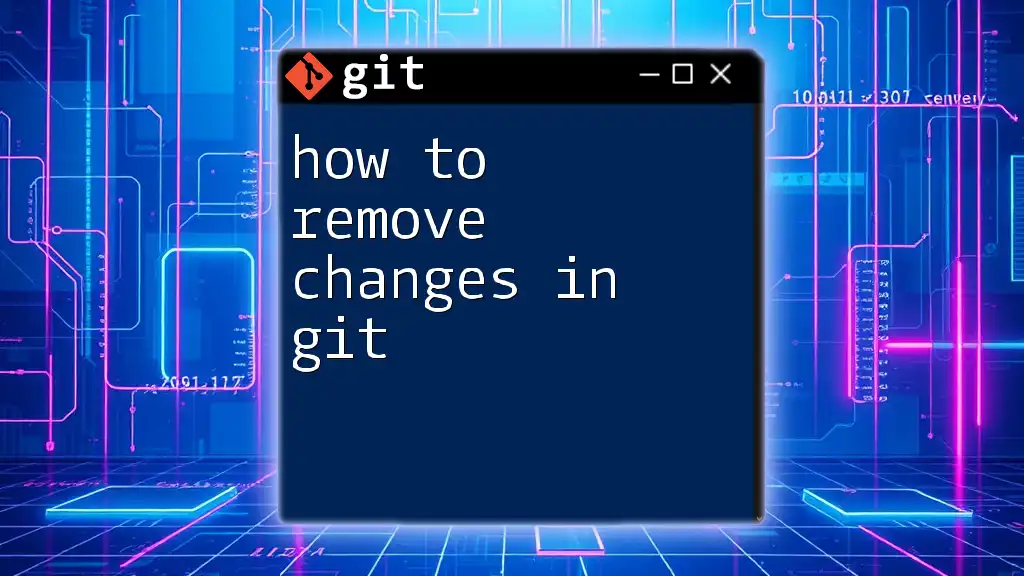
Methods to Remove Changes
When it’s time to clean up your repository, you have various options depending on your need to remove different types of changes.
Removing Changes in Tracked Files
Discarding Unstaged Changes
To revert any unstaged changes you've made to a tracked file, you can use the `git checkout` command. This command will eliminate changes and restore the file to its last committed state. Use it as follows:
git checkout -- <file-name>
Here, replace `<file-name>` with the name of the file you wish to restore. This action is effective for quickly undoing changes you haven’t staged yet.
Discarding Staged Changes
If you’ve staged changes but decide you want to revert them, the `git reset` command comes into play. This command will remove the specified file(s) from the staging area, allowing you to modify them again before committing.
To unstaged a file, use:
git reset HEAD <file-name>
Note: The staged changes will still exist in your working directory; they just won't be included in your next commit.
Complete Reversion of Tracked Files
If you wish to discard all changes in your working directory—both staged and unstaged—along with restoring your tracked files to the state of the last commit, the `git reset --hard` command is your best bet:
git reset --hard
Caution: This command will delete all your uncommitted changes permanently, so use it wisely, ensuring that any important work is backed up or committed first.
Removing Untracked Files
Sometimes, you might have files in your repository that are not being tracked by Git. If you want to delete these untracked files from your working directory, you'll use the `git clean` command.
Deleting Untracked Files
To remove untracked files, run:
git clean -f
The `-f` flag (force) is required to ensure you really want to delete those files. Without it, Git will ignore untracked files to prevent accidental deletions.
Dealing with Ignored Files
It’s possible you have ignored files in your repository that you may want to delete as well. These are typically specified in a `.gitignore` file.
Cleaning Ignored Files
To remove both untracked and ignored files, you can use:
git clean -fx
Here, the `-x` option will force the removal of ignored files. This command should be used with extreme caution, as it removes files that Git has been instructed to ignore.
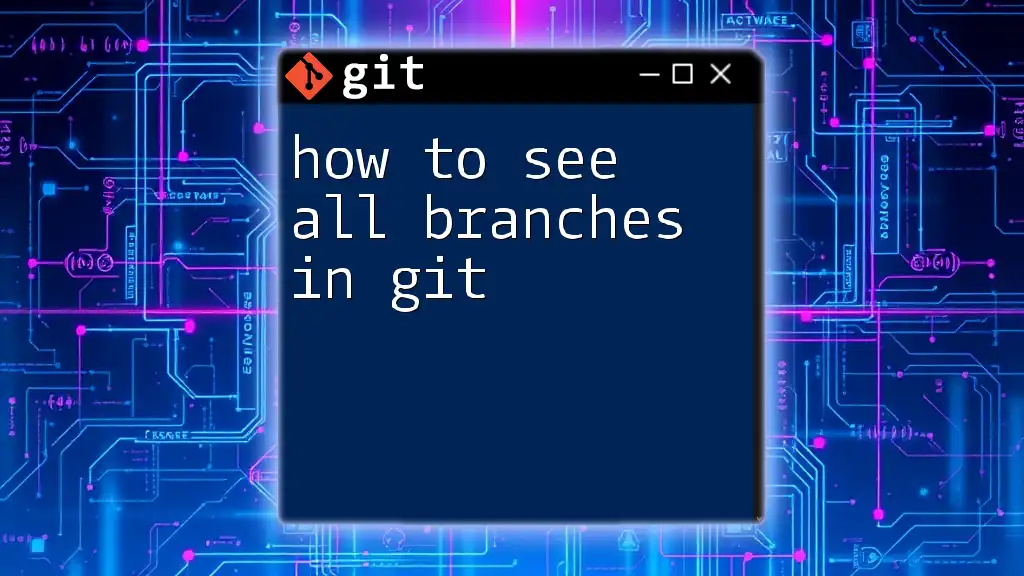
Best Practices
Before Removing Changes
Always check your status before making any changes. Running `git status` helps ensure you know what you are about to discard, allowing for more informed decision-making.
Backing Up Your Work
Consider using branches or stashes to preserve your modifications temporarily. If you're not ready to commit but don't want to lose your work, stashing is an excellent option:
git stash
This command saves your modifications and reverts your working directory to match the head commit, allowing you to come back to your changes later.
Understanding Commit History
Utilizing commit history can also aid in recovery. Always maintain a clean commit history; check your previous commits with:
git log
If you ever need to retrieve something you’ve discarded, understanding how to navigate your commit history is invaluable.
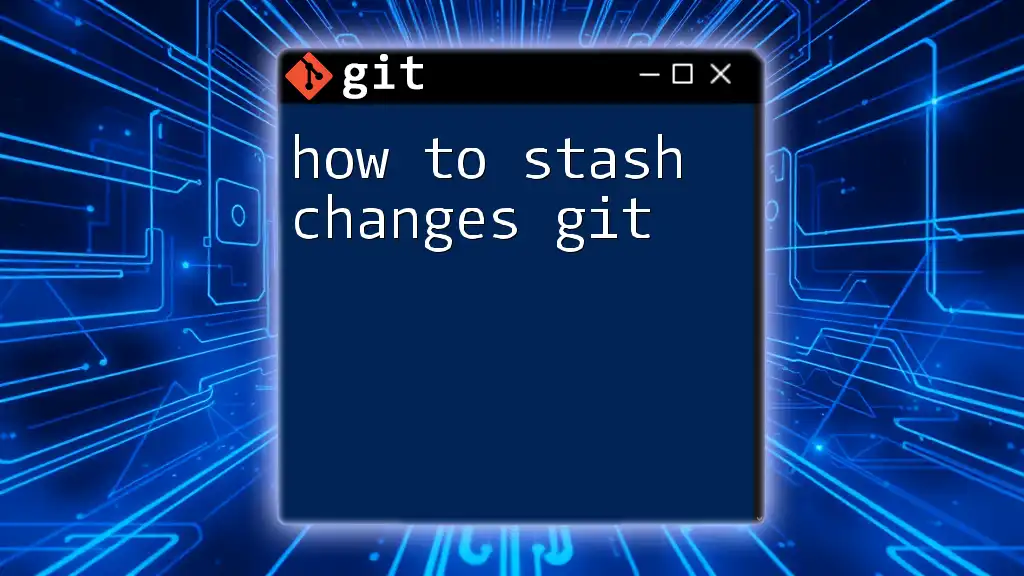
Common Mistakes to Avoid
Accidental Loss of Important Changes
One of the most significant risks when learning how to remove all changes in Git is inadvertently losing important files. Always double-check your working state before running commands like `git reset --hard`.
Not Recognizing Local Changes
Overlooking local changes can lead to unexpected outcomes. Mistakes often happen when users are unaware of their working directory’s state. Regularly using `git status` mitigates this risk.
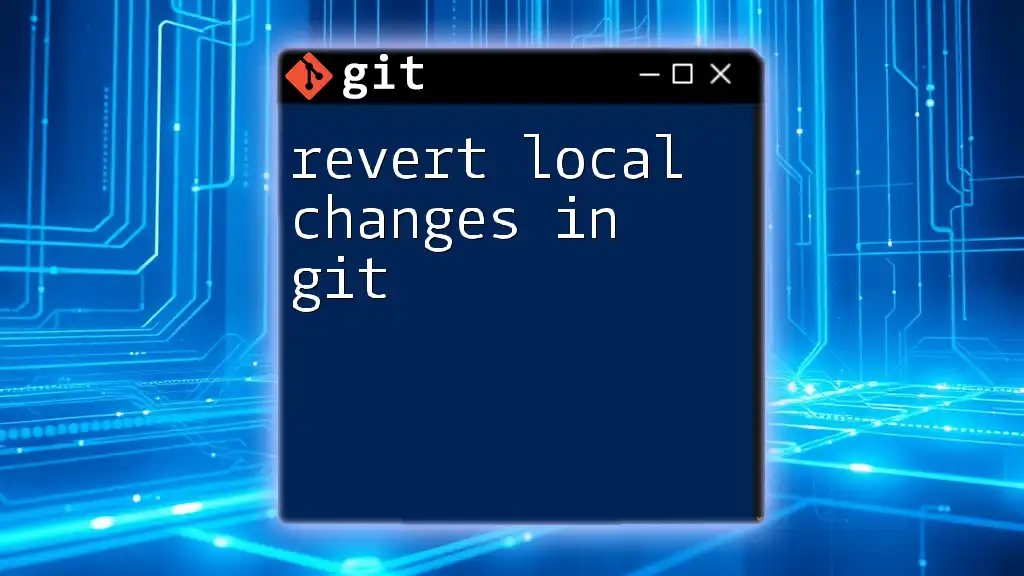
Conclusion
Understanding how to remove all changes in Git involves a clear grasp of your current repository state and utilizing the appropriate commands for your needs. By carefully considering each method and following best practices, you can effectively manage and revert changes without losing valuable work. Equip yourself with this knowledge, and practice in a safe environment to become proficient in Git commands.