To revert local changes in Git, you can use the `git checkout -- <file>` command to discard changes in a specific file or `git reset --hard` to reset your working directory to the last committed state.
git checkout -- <file>
or
git reset --hard
Understanding Local Changes
What are Local Changes?
Local changes refer to the modifications made in your working directory that have not yet been committed to the repository. These changes can be categorized into two types:
- Unstaged Changes: Modifications that you have made but have not yet staged for a commit. They exist only in your working directory.
- Staged Changes: Changes that you have marked to be included in the next commit using the `git add` command.
Understanding local changes is crucial for managing your project effectively and ensuring that only intended updates are saved in your Git history.
The Importance of Reverting Changes
Reverting changes is an essential feature of Git that allows developers to roll back any unwanted modifications. Common scenarios where reverting changes may be necessary include:
- Mistakes in Code: When you realize that your recent changes have introduced bugs or issues.
- Unplanned Additions: If you accidentally added files or code that should not be part of the current feature development.
- Clean Working Directory: Maintaining a clean working directory is vital for productivity and clarity when working on multiple features or bugs.
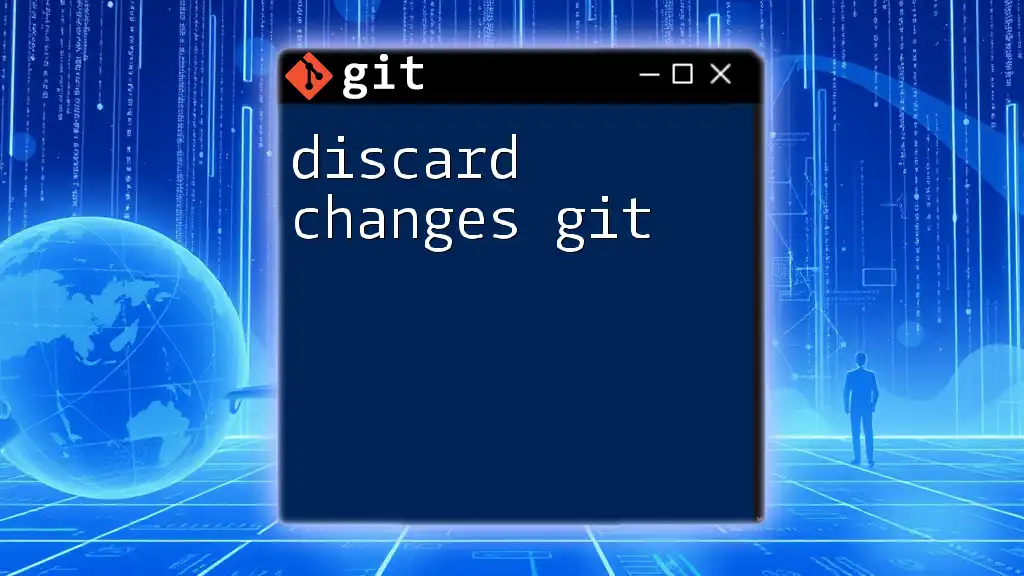
Reverting Unstaged Changes
Using git checkout
To revert unstaged changes in a specific file, you can use the `git checkout` command. Its syntax is as follows:
git checkout -- <file>
Example: If you want to revert changes made to a file named `myfile.txt`, you would use:
git checkout -- myfile.txt
This command tells Git to ignore the local changes made to `myfile.txt` and revert the file to its last committed state. It is essential to note the double hyphen `--`, which indicates that what follows is the name of a file.
Using git restore
With newer versions of Git, you can use the `git restore` command to achieve the same effect. The syntax is:
git restore <file>
Example: Reverting changes to `myfile.txt` can be accomplished by:
git restore myfile.txt
This command effectively resets the specified file to its last committed version. Using `git restore` can be more intuitive for those just starting with Git, as it more clearly indicates that the intention is to restore a file.
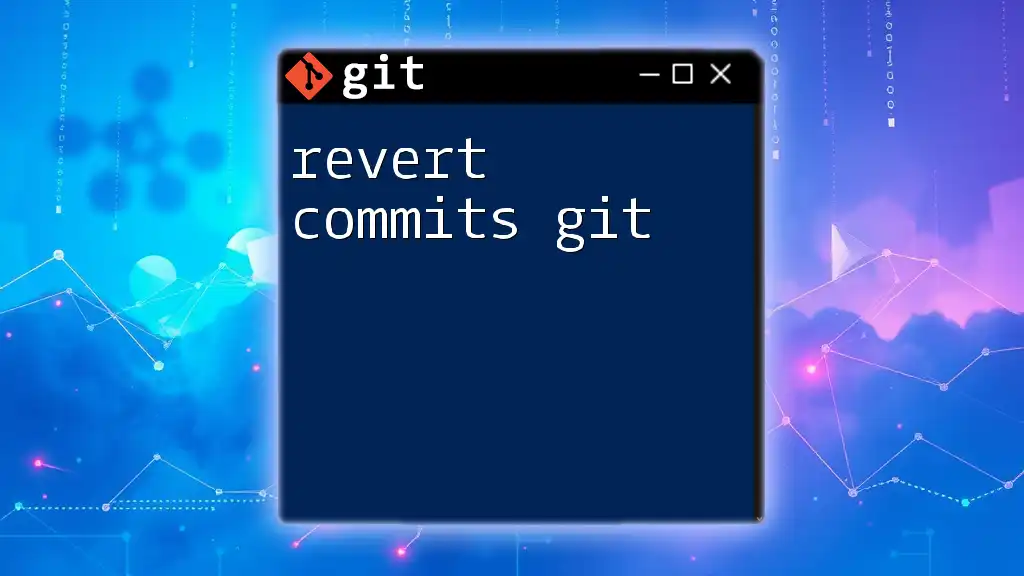
Reverting Staged Changes
Using git reset --mixed
If you've already staged your changes using `git add` but wish to revert them, you can use the `git reset` command with the `--mixed` option. The syntax is:
git reset HEAD <file>
Example: To unstage changes made to `myfile.txt`, execute:
git reset HEAD myfile.txt
Executing this command unstages the specified file, effectively moving it back to the unstaged state while keeping the changes in your working directory intact. This is particularly useful if you realize you want to modify the changes further before committing them.
Using git restore for Staged Files
Similar to unstaged changes, you can also use `git restore` for staged files. The syntax for unstaging is:
git restore --staged <file>
Example: To unstage `myfile.txt` while keeping your edits, run:
git restore --staged myfile.txt
This command removes the file from the staging area but does not alter the changes you've made.
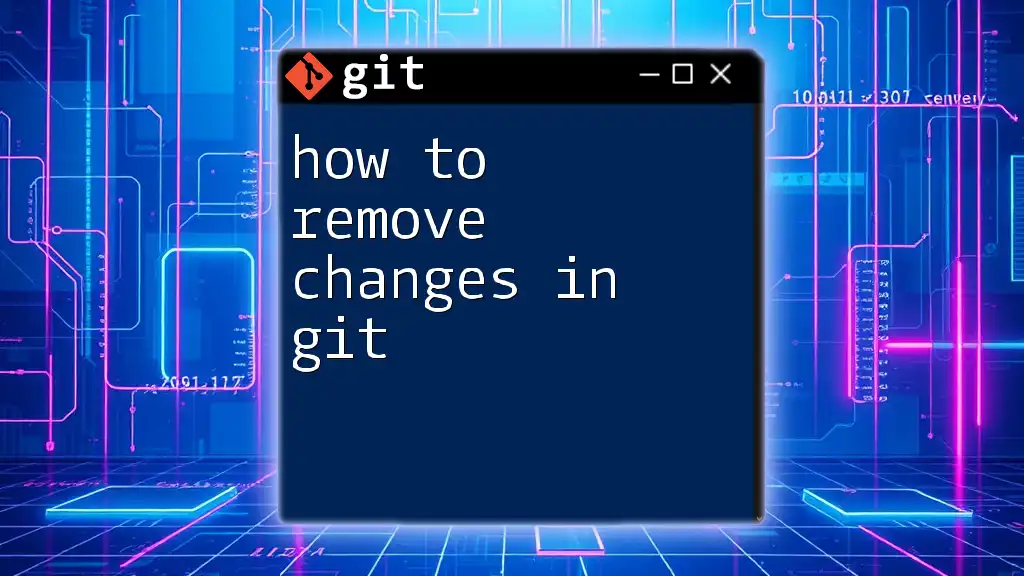
Reverting Committed Changes
Using git revert
When you need to undo a committed change, the `git revert` command is your ally. This command creates a new commit that undoes the changes of a specified commit while preserving the history. Its syntax is:
git revert <commit>
Example: To revert a specific commit identified by its hash (e.g., `abc1234`), you would use:
git revert abc1234
This command generates a new commit that effectively undoes the changes made in the specified commit, allowing the history to remain intact and ensuring that there are no lost changes.
Creating a New Commit
The power of `git revert` lies in its ability to maintain your project's commit history. Unlike `git reset`, which can overwrite history, `git revert` creates a new commit that indicates what was undone. This is especially vital when multiple developers are collaborating on a project, as it maintains clarity about what changes have been made and deleted.
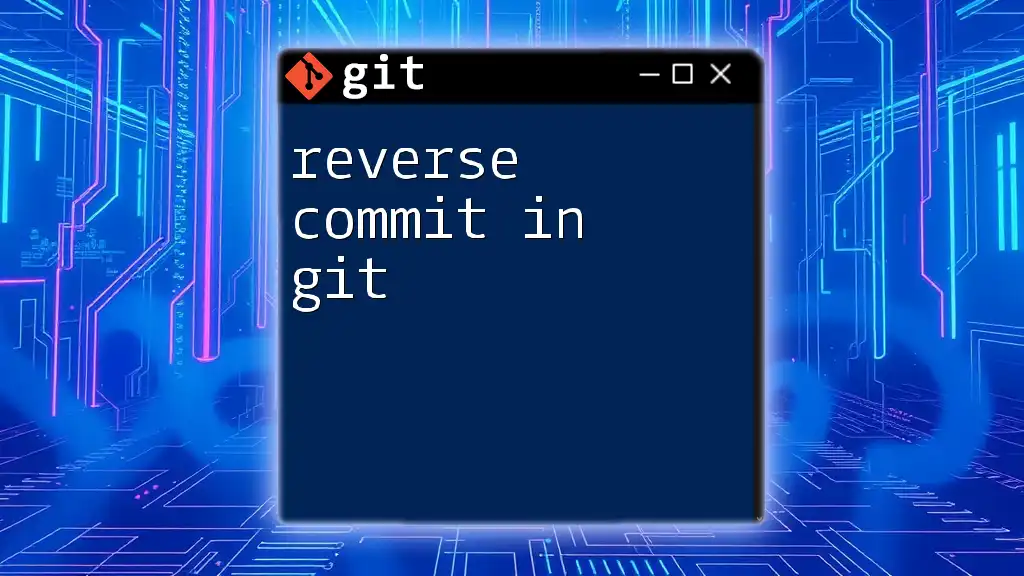
Undoing All Local Changes
Using git reset --hard
If you need to completely discard all local changes across your working directory and revert to the last commit, the `git reset --hard` command is a solution. The syntax is:
git reset --hard
Warning: This command will permanently erase all changes made since the last commit, both staged and unstaged. Use it with caution, as it cannot be undone.
This command is beneficial when you want to reset everything, especially after experimenting with changes that you no longer wish to keep.
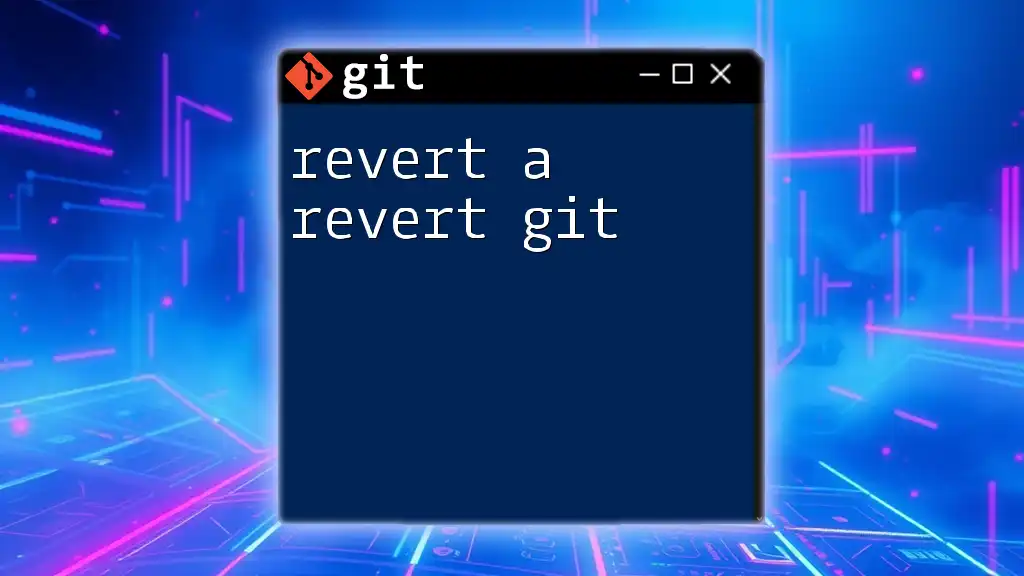
Best Practices for Managing Local Changes
To effectively handle local changes in Git:
- Regularly Commit Changes: Making frequent commits not only provides a safety net but also helps track progress and rollback if necessary.
- Utilize Branches: When working on new features or fixes, consider creating a branch to isolate changes. This allows you to revert or discard a branch without affecting the main codebase.
- Maintain a Clean Commit History: Before pushing changes, ensure that you have reverted unwanted local changes to keep your commit history clean and meaningful for collaborators.
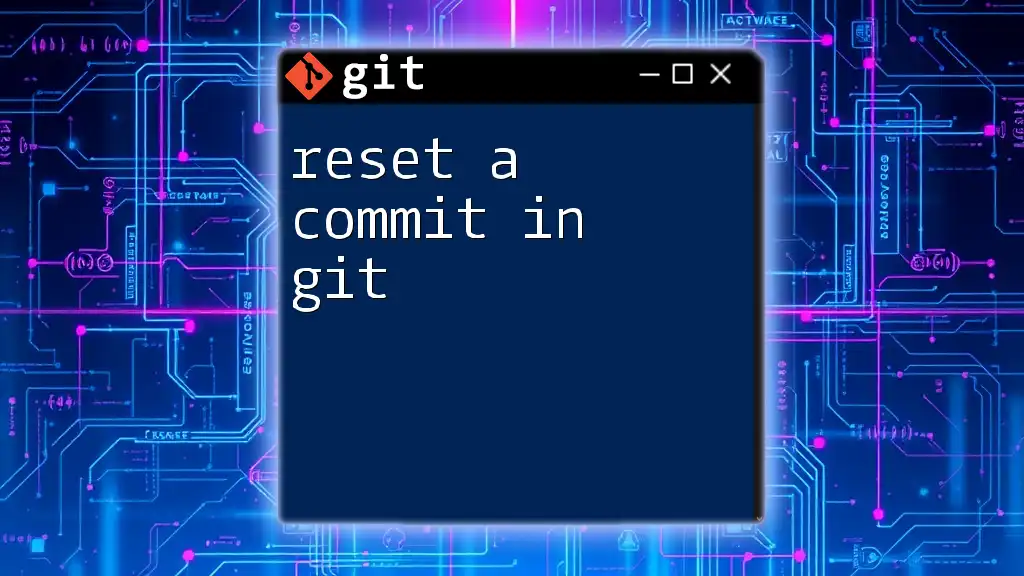
Conclusion
Reverting local changes in Git is a vital skill for any developer who wants to manage their code effectively. Understanding how to deal with unstaged, staged, and committed changes enhances your workflow and helps maintain a clean project history. Whether using commands like `git checkout`, `git reset`, or `git revert`, mastering these techniques will bolster your confidence as you navigate the intricacies of version control.
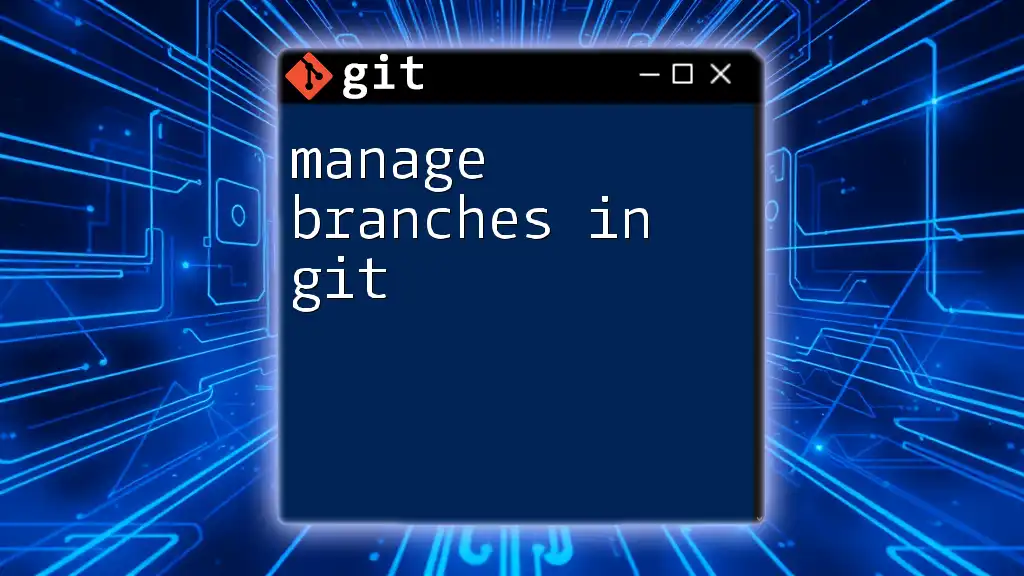
FAQs
Can I recover changes after using git reset --hard?
The `git reset --hard` command is powerful but destructive. Once executed, the changes cannot be retrieved using Git’s capabilities. However, if you have backups or if your changes were previously committed, you may recover them from those sources.
What is the difference between git reset and git revert?
The primary difference lies in how the two commands affect the commit history. `git reset` modifies your history and goes back to a previous state, which can be confusing for others working with the same repository. In contrast, `git revert` creates a new commit that undoes the changes of a specific commit, preserving the historical context.
How can I see what local changes I have before reverting?
You can view your current local changes by using the following commands:
- Check Status: This command shows you the status of your files and lists which ones are staged or unstaged.
git status
- View Differences: To see the actual changes made in your files compared to the last commit, use:
git diff
These commands provide a clear snapshot of your working directory, making it easier to decide what needs to be reverted.