Managing branches in Git involves creating, switching, and merging branches to streamline development and collaborate effectively within a project.
Here's a quick overview with example commands:
# Create a new branch
git branch my-new-branch
# Switch to the new branch
git checkout my-new-branch
# Merge changes from another branch (e.g., main) into the current branch
git merge main
# Delete a branch after merging
git branch -d my-new-branch
Understanding Git Branches
What is a Git Branch?
A Git branch is essentially a lightweight movable pointer that serves as a line of development. When you create a new branch, you are creating a separate context to work in. This allows developers to diverge from the main codebase, engage in feature development, or fix bugs without impacting the primary work until it’s ready to be merged. Branches facilitate parallel development, making it easier for multiple team members to contribute to a project simultaneously.
Benefits of Using Branches
Utilizing branches in Git enhances collaboration significantly. It allows team members to work on different features or fixes simultaneously without stepping on each other’s toes. Here are some reaped benefits:
- Isolation of Work: Develop features or fix bugs in isolation. This prevents unfinished work from interfering with the stable version of code.
- Experimentation: Create experimental features without worrying about affecting the main codebase.
- Simplified Collaboration: Team members can collaborate on different branches and merge changes only when needed.
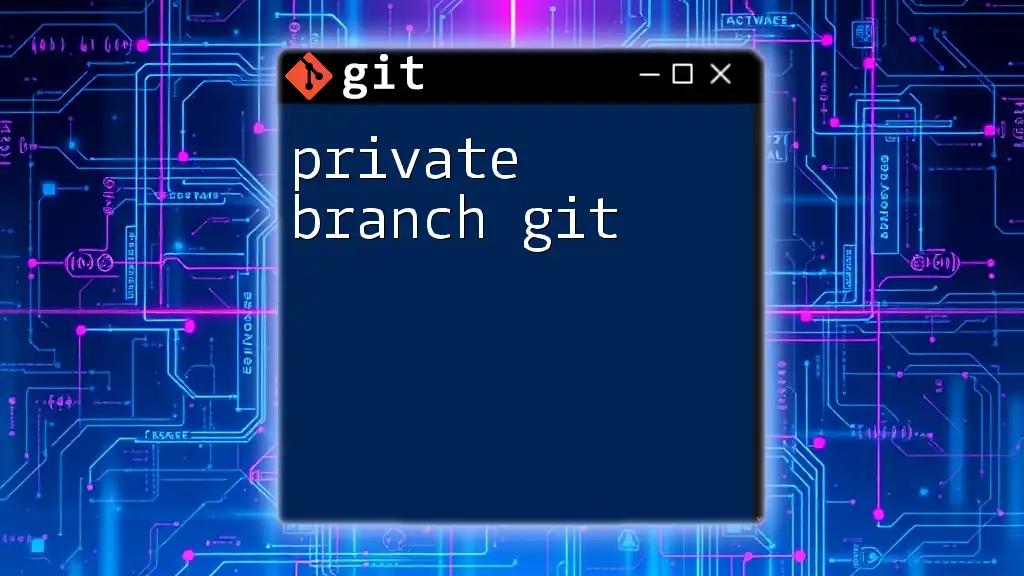
Creating Branches
Command to Create a New Branch
To create a new branch, you can utilize the `git branch` command as follows:
git branch <branch-name>
In this command, replace `<branch-name>` with a descriptive name that clearly indicates the purpose of the branch, such as `feature/login-form` or `bugfix/navbar-issue`. Adopting a consistent naming convention enhances transparency and reduces confusion.
Creating and Switching to a Branch in One Command
You can create a new branch and switch to it in a single command by using the `-b` option with `checkout`:
git checkout -b <branch-name>
This command is efficient and is often used when starting new features, allowing you to dive right into coding without switching context afterward.

Listing Branches
Viewing All Branches
To view all the branches, use:
git branch
Executing this command will list all local branches in your repository. The current active branch will be highlighted, allowing you to quickly identify where you are in your development.
Showing Remote Branches
To see the branches available on a remote repository, you can run:
git branch -r
This command displays remote branches, which is essential for understanding available work shared among your team.
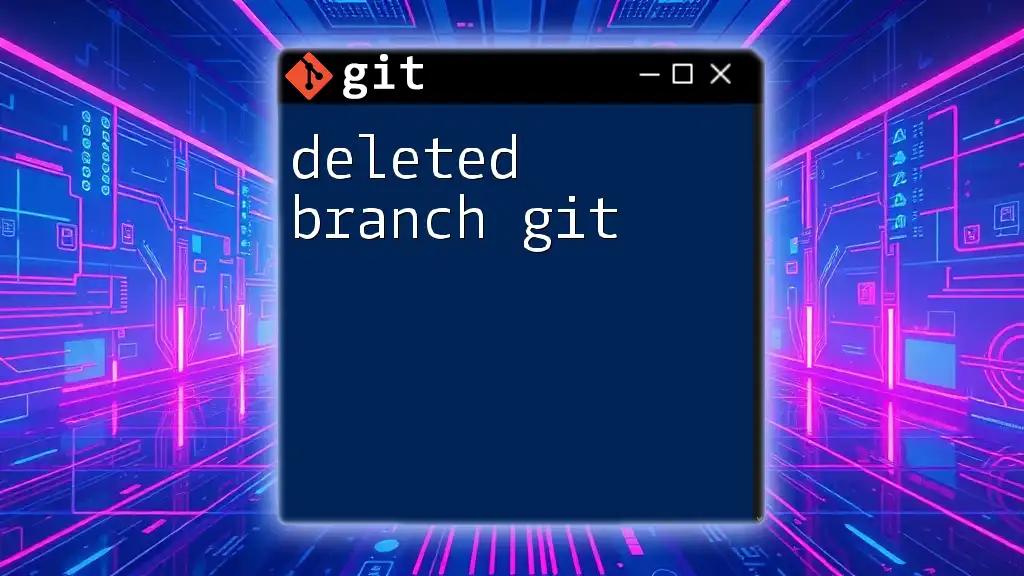
Switching Between Branches
Basic Command to Switch Branches
Switching between branches is accomplished with the `checkout` command:
git checkout <branch-name>
This command changes your working directory to the specified branch. Be aware that uncommitted changes may block switching. If so, you will need to either commit those changes or stash them before switching branches.
Using Git Switch
As of newer Git versions, you can use a dedicated command for switching:
git switch <branch-name>
This is a simpler and more intuitive way to switch branches, improving command clarity. It reduces the potential for errors inherent in using `checkout` for multiple purposes.
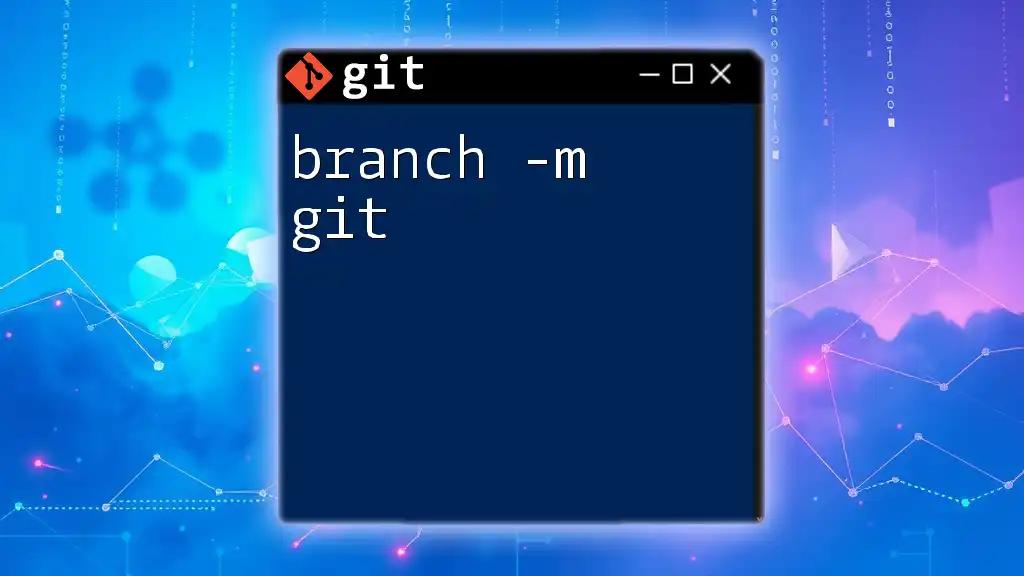
Merging Branches
What is Merging?
Merging is the process of integrating changes from one branch into another. This is crucial for consolidating work done in feature branches back into the main codebase. Understanding the distinct methods of merging, namely merging and rebasing, is vital for effective Git usage.
Merging Branches in Git
To merge changes from one branch into another, follow these steps:
git checkout <target-branch>
git merge <source-branch>
Here, `<target-branch>` is where you want the changes to be integrated, while `<source-branch>` is the branch you’ve worked on. It’s critical to ensure that the target branch is up-to-date before merging to minimize conflicts.
Fast-Forward vs. Three-Way Merge
When merging, you may encounter two primary types:
- Fast-Forward Merge: If the target branch hasn't diverged, Git will simply move the branch pointer forward to the latest change. This keeps the history linear and clean.
- Three-Way Merge: If both branches have new commits, Git will create a new merge commit to consolidate changes. Understanding how to resolve conflicts in this scenario is critical.
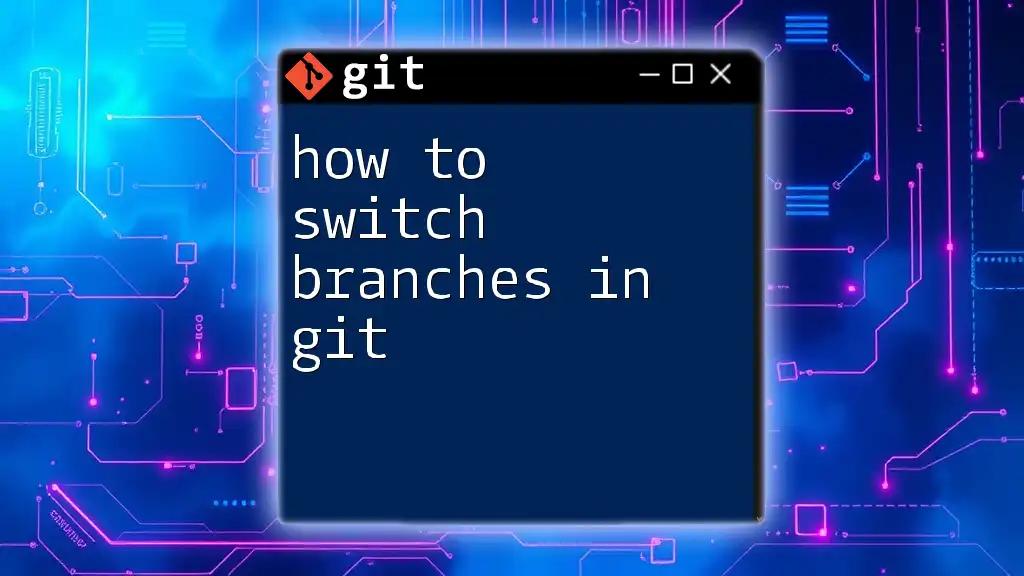
Deleting Branches
Deleting Local Branches
To delete a local branch that is fully merged:
git branch -d <branch-name>
This command ensures you only delete branches that are no longer needed, preserving your project's integrity. If the branch hasn’t been merged, Git will prevent deletion, requiring you to take caution before removing unmerged branches.
Force Deleting Local Branches
In scenarios where deletion is necessary despite unmerged changes, use:
git branch -D <branch-name>
This type of deletion bypasses the safety check, but exercise caution as it can lead to loss of uncommitted work.
Deleting Remote Branches
To remove branches from the remote repository, the following command is used:
git push origin --delete <branch-name>
Always ensure to communicate this action with your team to prevent confusion, as removing remote branches can affect collaborative efforts.
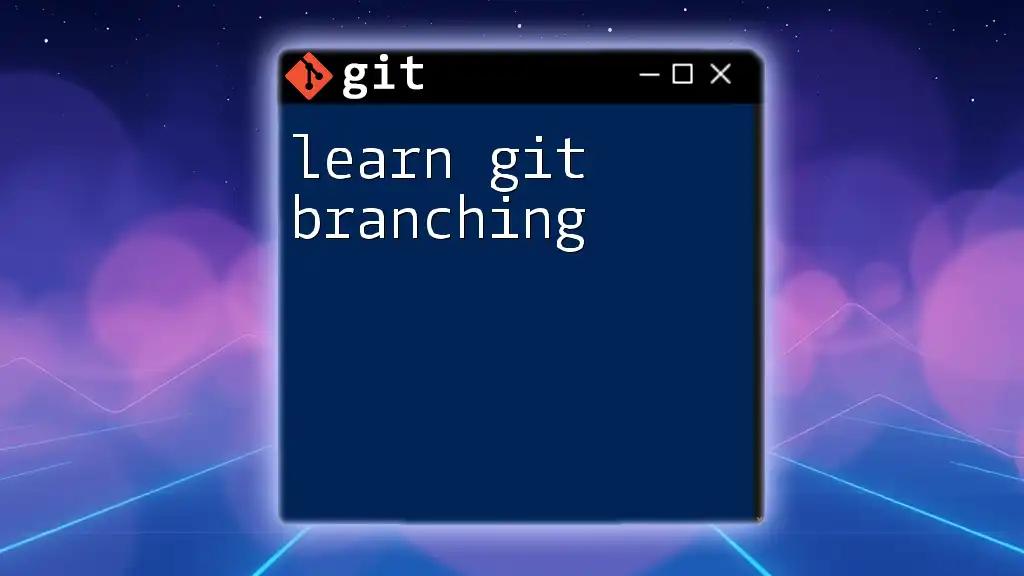
Best Practices for Branch Management
Naming Conventions for Branches
Establishing a clear and consistent naming convention for branches enhances the understandability of the project's development. Good examples include prefixes like `feature/`, `bugfix/`, or `hotfix/`. Avoid vague names—clear, descriptive titles help maintain clarity and purpose.
Keeping Branches Up to Date
Maintain branch relevance by frequently merging updates from your main branch to your feature branches. Doing this helps minimize conflicts and keep your work aligned with the latest project updates.
Regular Cleanup of Old Branches
Old branches can clutter your repository and lead to confusion. Make a habit of reviewing and removing stale branches regularly. Setting up reminders for cleanup can help keep your branches organized and reduce clutter.
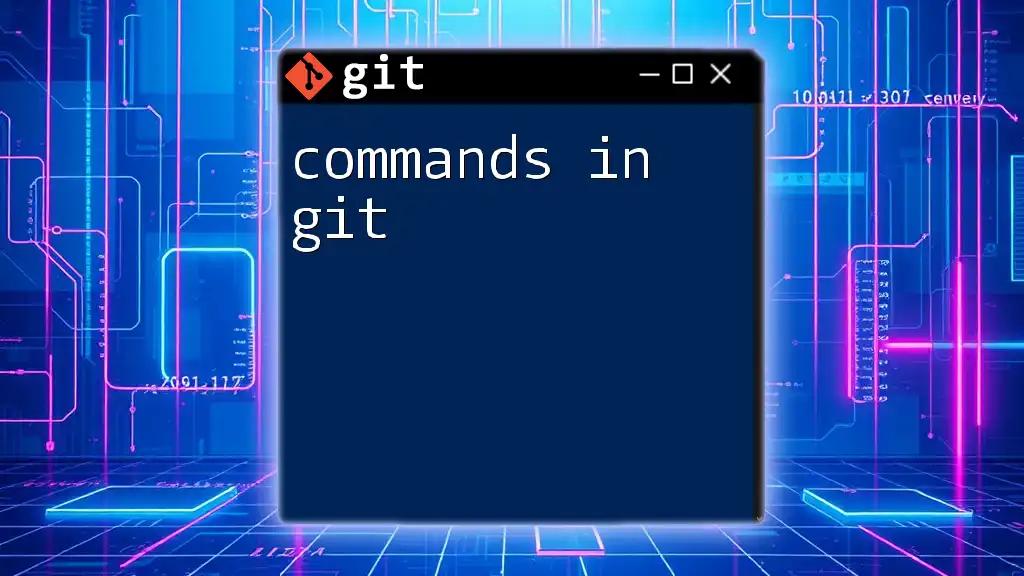
Conclusion
Managing branches in Git is an essential skill for developers and teams collaborating on software projects. By understanding how to create, merge, switch, and delete branches, along with adhering to best practices, you can significantly improve your team's workflow and project efficiency. Practice these skills to enhance your Git proficiency, and consider engaging in training sessions designed to boost your command of such crucial concepts.