Kubernetes Git integration allows you to manage your Kubernetes configurations and deployments through Git, enabling version control and collaboration for your containerized applications.
Here's an example of a command to sync your Kubernetes manifests with a Git repository:
git clone https://github.com/yourusername/your-k8s-repo.git
Integrating Git with Kubernetes
Understanding Continuous Integration/Continuous Deployment (CI/CD)
Continuous Integration/Continuous Deployment (CI/CD) is a set of practices designed to improve software development processes by allowing frequent and reliable software releases. CI/CD practices are crucial in modern development environments, particularly for cloud-native applications managed in Kubernetes.
In this context, Git plays a pivotal role in automating deployments and tracking code changes. When developers push code to a Git repository, it can trigger a series of automated processes that:
- Build the application
- Run tests to ensure functionality
- Deploy changes to Kubernetes seamlessly
By integrating Git into CI/CD pipelines, teams can shorten their development cycles and improve product quality.
GitOps: The Working Principle
GitOps is a modern practice deriving from the principles of DevOps, where Git is the single source of truth for infrastructure and application configurations. In GitOps, all Kubernetes resources are stored as code in a Git repository. This method not only provides a clear audit trail of infrastructure changes but also enhances collaboration among team members.
Core principles of GitOps include:
- Declarative infrastructure: All configurations are specified in declarative files, making it easy to track changes and configure environments consistently.
- Versioned deployment: Using Git to version deployments allows for easy rollbacks and visibility into the histories of deployments.
- Automation: Continuous deployment tools monitor changes in the Git repository and apply those changes to the Kubernetes cluster automatically.
Benefits of adopting GitOps include enhanced productivity, improved reliability through automation, and increased system transparency.
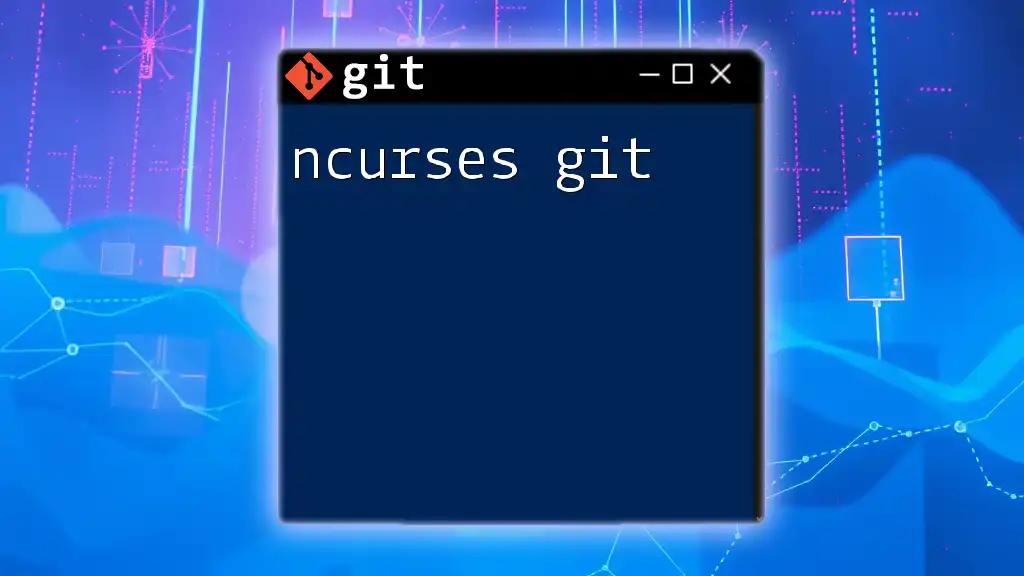
Setting Up Your Kubernetes Environment
Prerequisites
Before diving into using Kubernetes along with Git, make sure you have the following tools installed on your local environment:
- Kubernetes CLI (kubectl): Used to interact with Kubernetes clusters.
- Docker: Containers are the building blocks in Kubernetes.
- Git: Essential for version control.
- Kubernetes Cluster: This can be created using tools such as Minikube, Google Kubernetes Engine (GKE), Amazon EKS, or Azure AKS.
Creating a Kubernetes Cluster
Creating a Kubernetes cluster can be easily accomplished using Minikube, which runs a single-node Kubernetes cluster on your local machine for development purposes. To create a Minikube cluster, use the following command:
minikube start
After successfully starting Minikube, you can access your local Kubernetes cluster using `kubectl`.
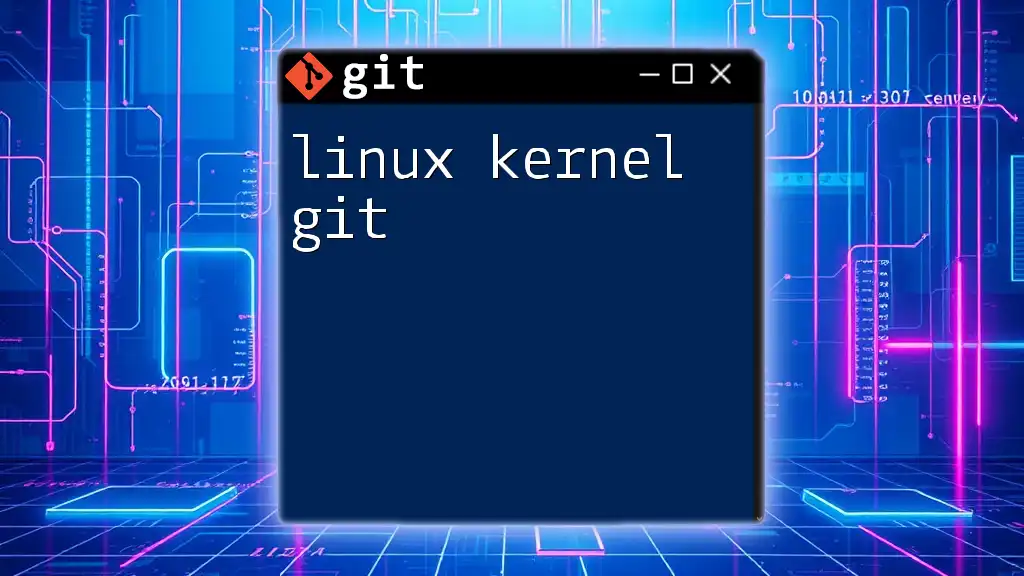
Version Control with Git in Kubernetes
Creating a Git Repository
To manage your Kubernetes resources effectively, initialize a Git repository for your project. Run the following command to create a new Git repository:
git init my-k8s-project
This command sets up a new Git repository in a folder named `my-k8s-project`.
Structuring Your Kubernetes Resources
A well-structured folder organization for your Kubernetes manifests enhances maintainability and collaboration. A recommended folder structure might look like this:
my-k8s-project/
├── deployments/
├── services/
├── configmaps/
└── secrets/
This structure allows anyone to locate specific resources quickly and understand the purpose of each directory.
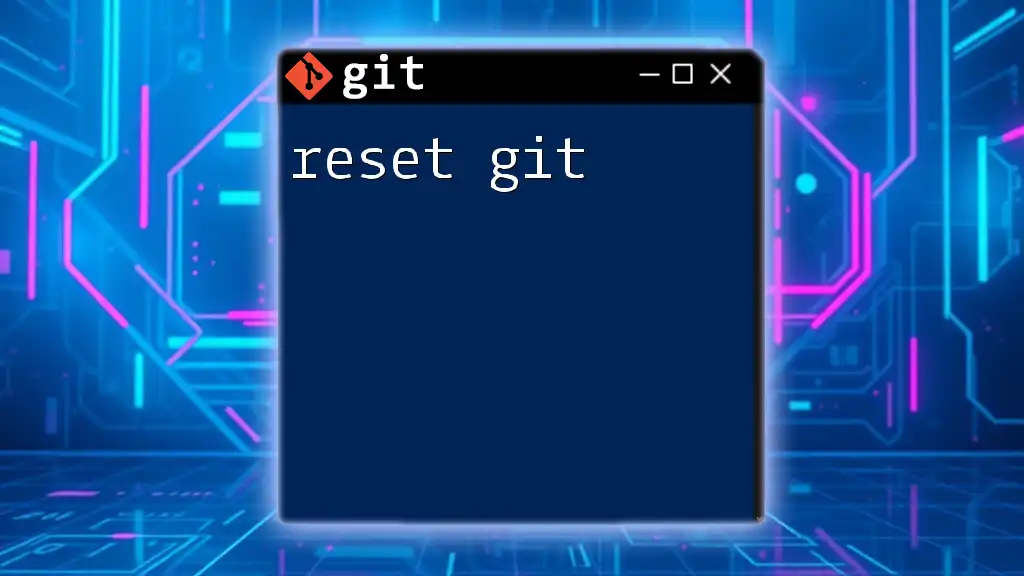
Managing Kubernetes Manifests with Git
Writing Your First Kubernetes Manifest
Kubernetes resources are defined using YAML manifest files. Each manifest describes a desired state of a specific resource type. For example, below is a simple Deployment manifest for an application:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app
spec:
replicas: 3
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-app-container
image: my-app-image:latest
This manifest defines a Deployment that manages a set of replicas for your application. Check the Kubernetes documentation for additional resource types, each with its own set of required fields.
Committing Changes to Your Repository
As you make changes to your Kubernetes manifests, it’s essential to commit those changes to your Git repository. Here are some best practices:
- Write meaningful commit messages that explain the changes clearly.
- Commit frequently to keep track of changes at granular levels.
Example commands for committing changes include:
git add .
git commit -m "Initial commit of the my-app deployment"
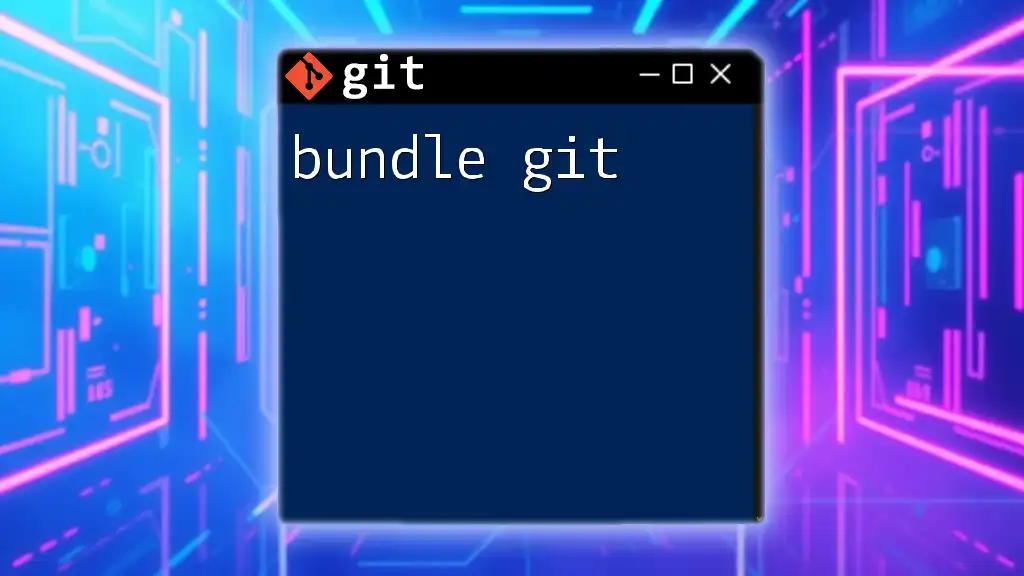
Automating Deployments with Git and Kubernetes
Choosing a CI/CD Tool
Selecting an appropriate CI/CD tool is crucial for automating your Kubernetes deployments. Popular options include Jenkins, GitHub Actions, and GitLab CI. Each has its strengths and weaknesses based on team workflows, preferences, and existing infrastructure.
For example, GitHub Actions integrates seamlessly with GitHub repositories, providing an easy way to automate deployments triggered by Git events like a `push` to the main branch.
Creating CI/CD Pipelines
To implement automation using GitHub Actions, you can create a workflow file in your repository. Below is an example of a GitHub Actions workflow to deploy an application to Kubernetes:
name: Deploy to Kubernetes
on:
push:
branches:
- main
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- name: Check out code
uses: actions/checkout@v2
- name: Set up Kubeconfig
run: |
echo "${{ secrets.KUBECONFIG }}" > kubeconfig.yaml
export KUBECONFIG=$(pwd)/kubeconfig.yaml
- name: Apply Kubernetes Manifests
run: kubectl apply -f ./deployments/
This workflow checks out the code, sets up the Kubernetes configuration, and applies YAML manifests to the Kubernetes cluster when changes are pushed to the `main` branch.
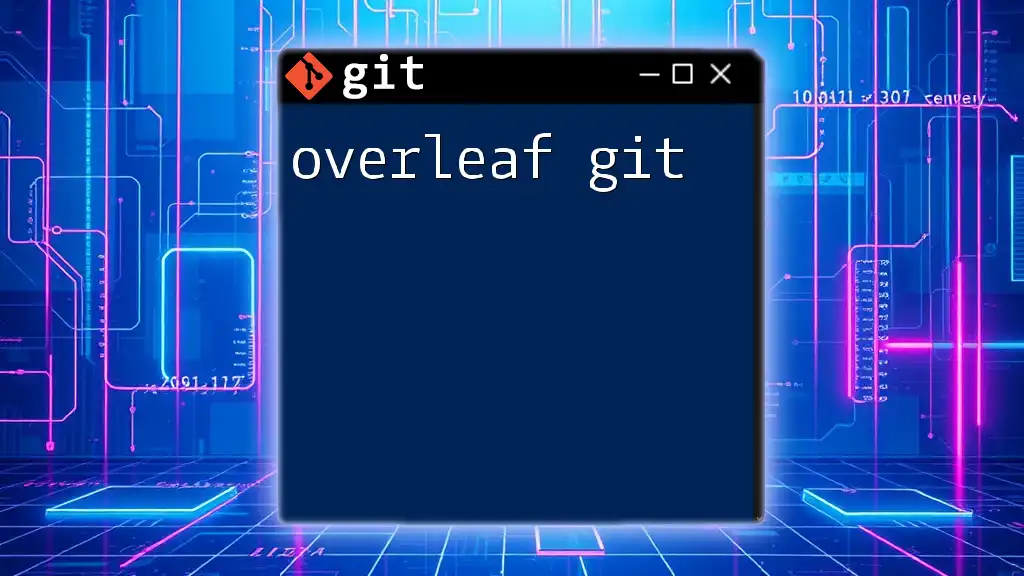
Monitoring and Troubleshooting
Setting Up Monitoring
Effective monitoring is critical in production environments. Tools like Prometheus and Grafana provide excellent capabilities to monitor application performance and health within Kubernetes clusters. Implementing observability will help you gain insights into your applications and optimize performance over time.
Troubleshooting Deployment Issues
Even with a robust CI/CD setup, issues can arise during deployments. Familiarizing yourself with common problems and their resolutions is essential. Use the `kubectl` command-line tool for checking the status of resources:
kubectl get pods
kubectl logs <pod-name>
Common issues might include:
- Incorrect configurations in your manifests
- Resource quota limits being exceeded
- Problems with image pulling or container startup
By examining logs and resource statuses, you can pinpoint and address issues promptly.
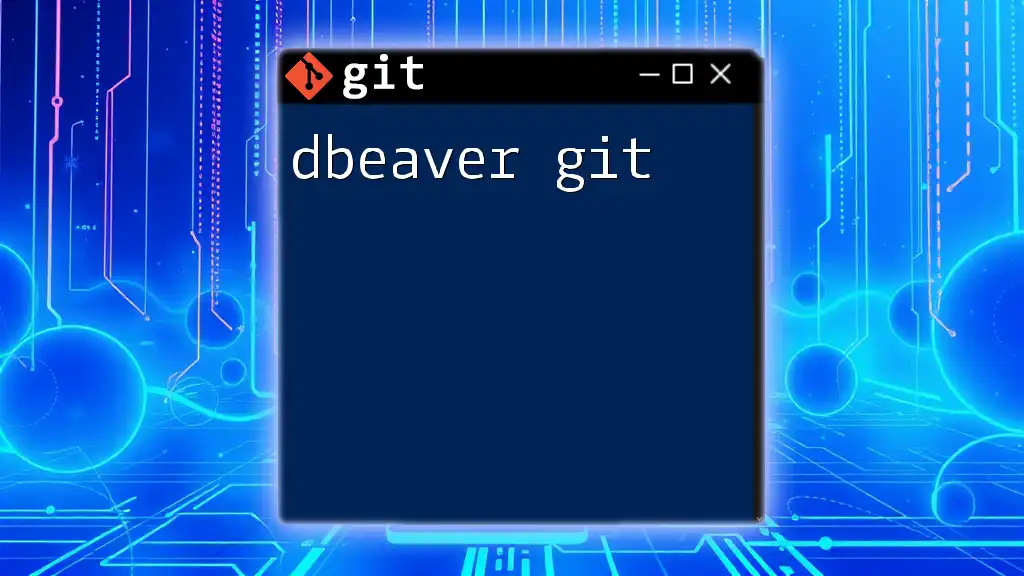
Conclusion
Integrating Kubernetes git practices into your development workflows significantly enhances your ability to manage deployments effectively. By leveraging Git for version control and adopting GitOps principles, you can ensure your infrastructure remains consistent, auditable, and easy to collaborate on.
Embracing GitOps not only streamlines deployment processes but also empowers teams to iterate rapidly and deliver value more efficiently. With these practices in place, you'll be well on your way to mastering the synergy between Kubernetes and Git.
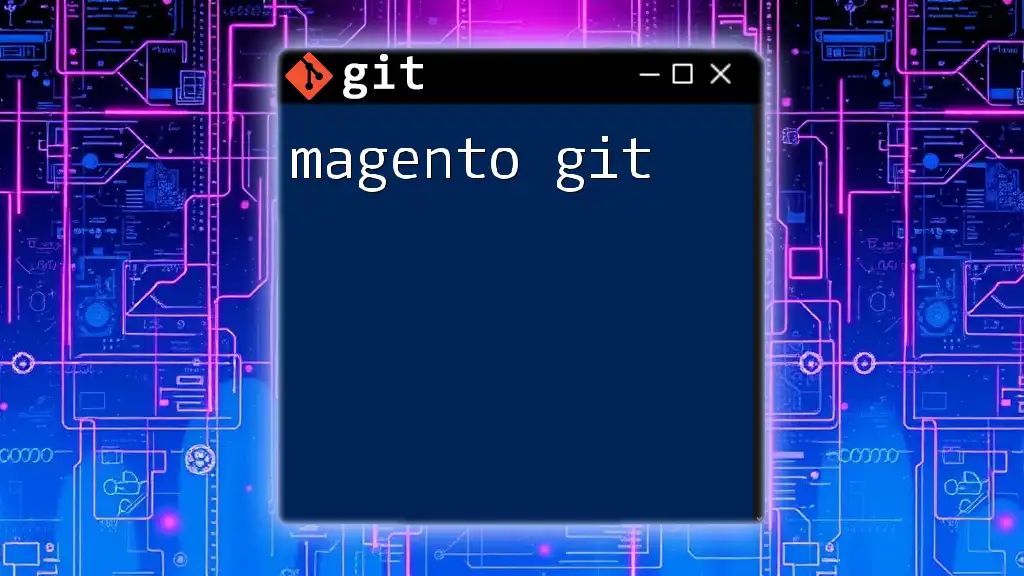
Additional Resources
To further enhance your understanding and implementation of Kubernetes and Git, consider exploring the following resources:
- Official [Kubernetes documentation](https://kubernetes.io/docs/home/)
- Sample Kubernetes projects on [GitHub](https://github.com/)
- Online courses focused on GitOps and Kubernetes development
With these tools and knowledge, you can deepen your expertise and drive impactful results in your software development journey.