Git commands are powerful tools that allow users to manage and track changes in their code repositories efficiently.
Here's a basic example of using Git to initialize a new repository:
git init
Understanding Git Structure
What is a Repository?
A Git repository is essentially a storage space for your project, which can be either on your local machine or on a remote server. You can think of it as a directory that contains all of your project files and their history.
-
Local Repository: This is stored on your own machine. Every time you initiate a repository with the command `git init`, you create a local repo that tracks changes made to your files.
-
Remote Repository: Stored on a server (like GitHub, GitLab, or Bitbucket), a remote repository allows multiple collaborators to access the same project.
To create a local repository, you can run:
git init
The Role of Branches
Branches in Git help manage different lines of development in your project. They allow developers to work on features independently without disturbing the main codebase.
When you create a new branch, you can isolate your changes, test new features, and ultimately merge back into the main branch (often called `main` or `master`) once your work is complete.
To create a new branch, use:
git branch [branch-name]
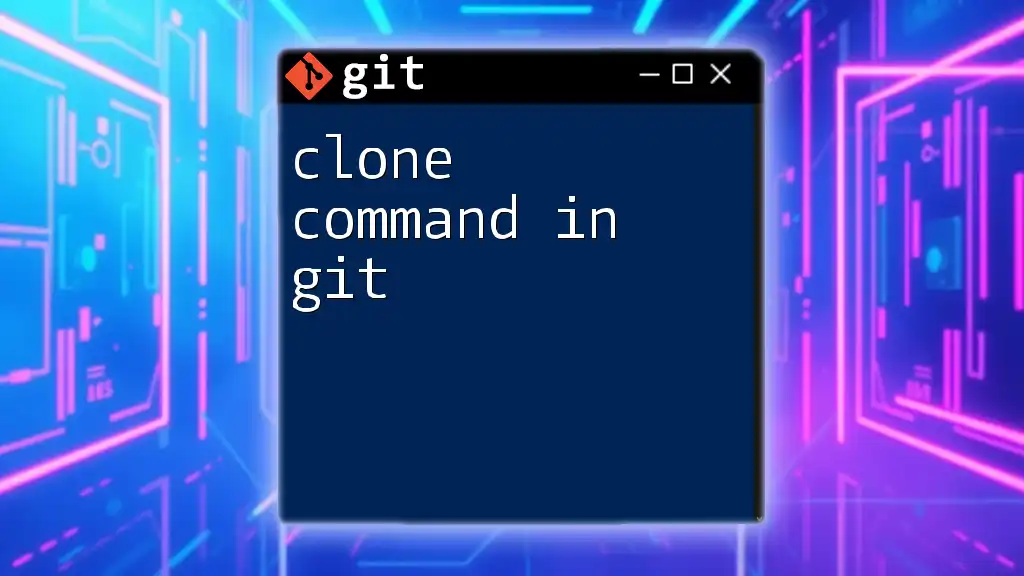
Essential Git Commands
Setting Up Git
Installing Git
To use the commands in Git, you need to install it on your machine. Git can be installed on various platforms:
-
Windows: Download and run the installer from the official Git website.
-
macOS: Use Homebrew with the command:
brew install git
-
Linux: Install Git using your package manager, such as:
sudo apt-get install git
Configuring Git
After installation, it’s essential to configure Git to personalize your user information. This can be done using the `git config` command. You can set a global configuration that applies to all repositories on your machine.
To set your user name and email, run:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
Basic Commands
git init
To create a new Git repository, the command `git init` will initialize an empty repository or convert an existing directory into a Git repository. You can execute this in your project folder:
git init
git clone
If you want to create a local copy of an existing remote repository, you use the `git clone` command. For example, to clone a repository from GitHub, execute:
git clone https://github.com/username/repo.git
git status
To check the current status of your files in the repository, the `git status` command is invaluable. It shows you which files have been modified, which are staged, and which are untracked. Simply run:
git status
This is crucial for understanding what changes have been made before committing or pushing.
git add
The `git add` command stages your changes, preparing them for the next commit. You can stage specific files with:
git add filename.ext
Or stage all modified files with:
git add .
git commit
Once you have staged your changes, it's time to commit them to your repository. The `git commit` command takes a snapshot of your staged changes. Committing requires a message to describe the changes:
git commit -m "Your commit message here"
Best practices suggest keeping commit messages concise yet descriptive to provide context for future references.
Branch Management
git branch
To list all the branches in your repository, simply use:
git branch
You can also create a new branch with:
git branch new-branch
git checkout
Switching between branches can be accomplished with the `git checkout` command. For example, to switch to a branch named `feature-branch`, run:
git checkout feature-branch
git merge
When you want to combine changes from one branch into another, you utilize `git merge`. For example, if you've finished working on `feature-branch` and want to merge it into `main`, check out the main branch and run:
git checkout main
git merge feature-branch
If there are conflicts, Git will guide you to resolve them before finalizing the merge.
Remote Repository Interaction
git remote
To work collaboratively, you will often need to interact with remote repositories. The `git remote` command allows you to manage remote connections. To add a new remote repository, you use:
git remote add origin https://github.com/username/repo.git
git fetch
The `git fetch` command helps you keep your local repository updated with changes that have been made in the remote repository. Fetching updates the remote tracking branches:
git fetch origin
Remember, `git fetch` does not merge changes; it merely receives updates.
git push
When you've made changes locally and are ready to send those changes to the remote repository, use the `git push` command:
git push origin main
Ensure that your local branch is up to date before you push to avoid unintended conflicts.
Viewing History and Changes
git log
To view the history of commits in your repository, the `git log` command is your go-to tool. It provides a detailed history of commits. For a simplified view, use:
git log --oneline
git diff
To see the differences between your files, the `git diff` command is useful. You can compare what you have modified against what is staged or your last commit:
git diff
This is especially handy for reviewing changes before staging and committing.
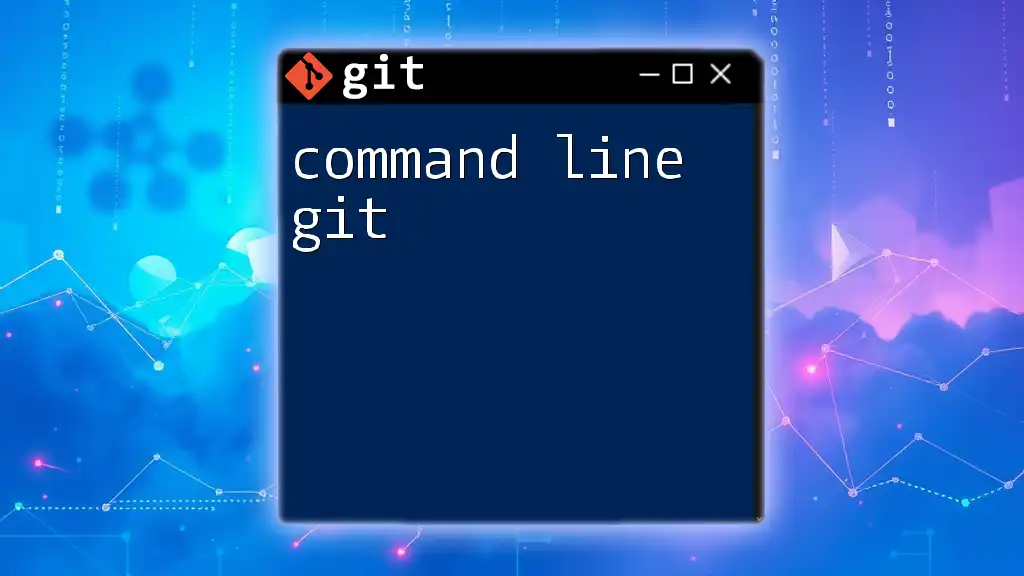
Advanced Commands
Stashing Changes
git stash
If you are working on something and can't commit your changes yet, you can use the `git stash` command to save your changes temporarily. You can later retrieve the stashed changes with:
git stash apply
Rebasing
git rebase
Rebasing is an alternative to merging branches. It allows you to integrate changes from one branch into another while maintaining a linear project history. Use:
git checkout feature-branch
git rebase main
Rebasing helps keep the project history clean but requires careful conflict resolution.
Tagging
git tag
Tags are a great way to mark specific points in your Git history, often used for version releases. To create a new tag, simply run:
git tag -a v1.0 -m "Release version 1.0"
You can list your tags with:
git tag
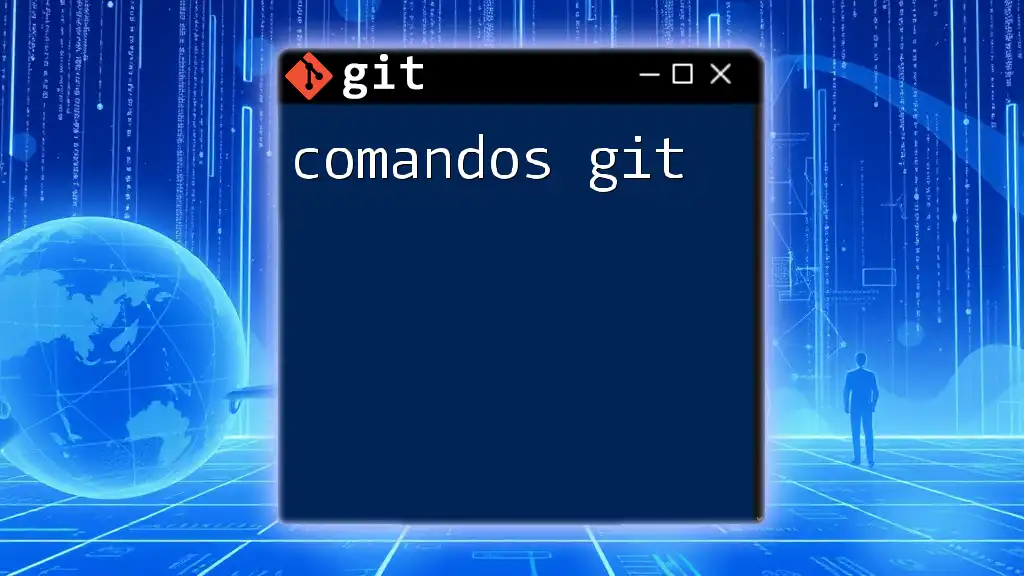
Conclusion
Mastering the commands in Git is essential for any developer looking to enhance their productivity and effectiveness in collaborative projects. With the commands outlined in this guide, you can efficiently manage your code, collaborate with others, and keep track of your changes. Practicing these commands will give you confidence in using Git as your source control management tool.
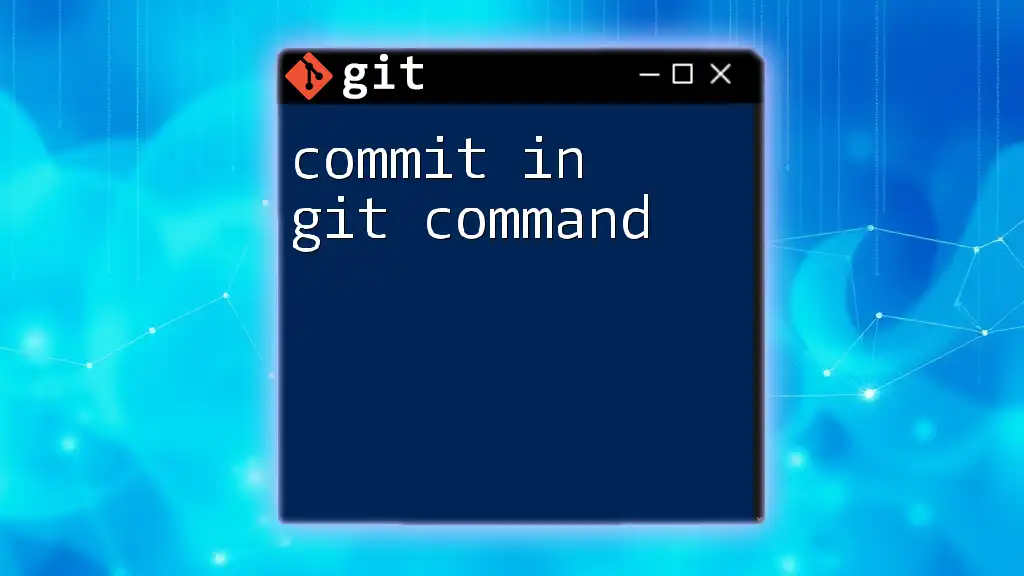
Additional Resources
For further learning, consider exploring the official [Git documentation](https://git-scm.com/doc), engaging with community forums, or enrolling in online courses that delve deeper into Git's capabilities and best practices. Consistent practice will elevate your understanding and proficiency in using Git commands.