The `git clone` command is used to create a local copy of a remote Git repository, including all its history and branches.
Here’s the basic syntax:
git clone <repository-url>
Understanding the Git Clone Command
What is the Git Clone Command?
The clone command in git is a fundamental tool used to create a local copy of a remote repository. When you clone a repository, Git not only downloads the files but also retrieves the entire version history, allowing you to work seamlessly in your local environment. This capability is essential for collaborative development, as it gives contributors full access to all the project data.
Why Use the Git Clone Command?
The clone command in git provides several vital benefits that enhance your development workflow:
- Local Development: By cloning a repository, developers can work offline, making changes without needing an active internet connection.
- Contribution to Projects: Cloning allows you to engage with open-source projects or team repositories easily, fostering collaboration.
- Easy Access to Code and Version History: You get a complete history of the project, enabling you to explore past commits, revert changes, or understand the evolution of the codebase.
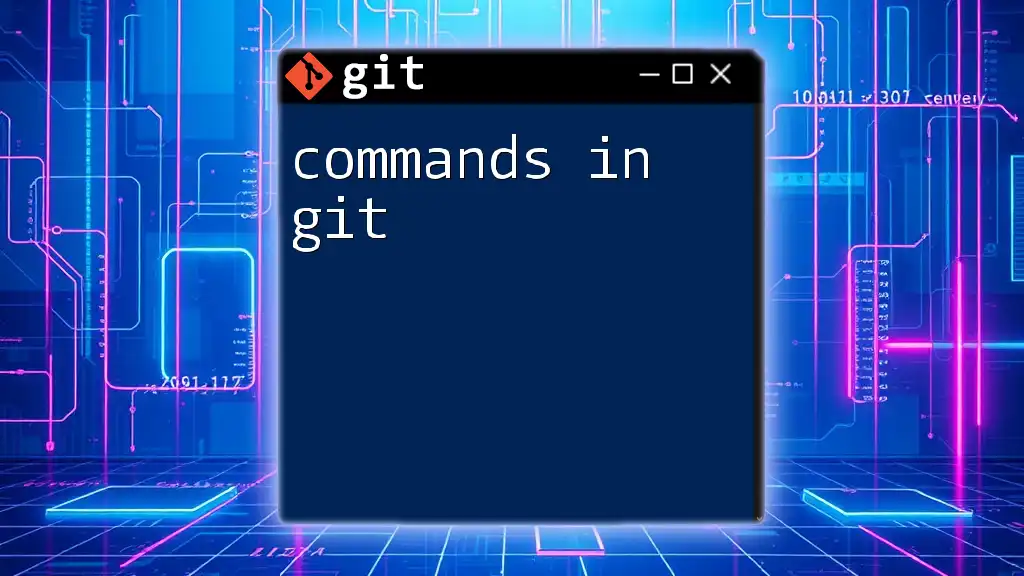
Basic Syntax of the Git Clone Command
Command Structure
The basic syntax for the clone command in git is as follows:
git clone <repository_url> [<directory>]
In this command:
- repository_url: This is the location where the repository resides, which can be a URL pointing to a hosted service like GitHub, GitLab, or Bitbucket.
- directory: This is optional. If specified, it indicates the name of the directory where you want to clone the repository. If omitted, Git will create a directory with the same name as the repository.
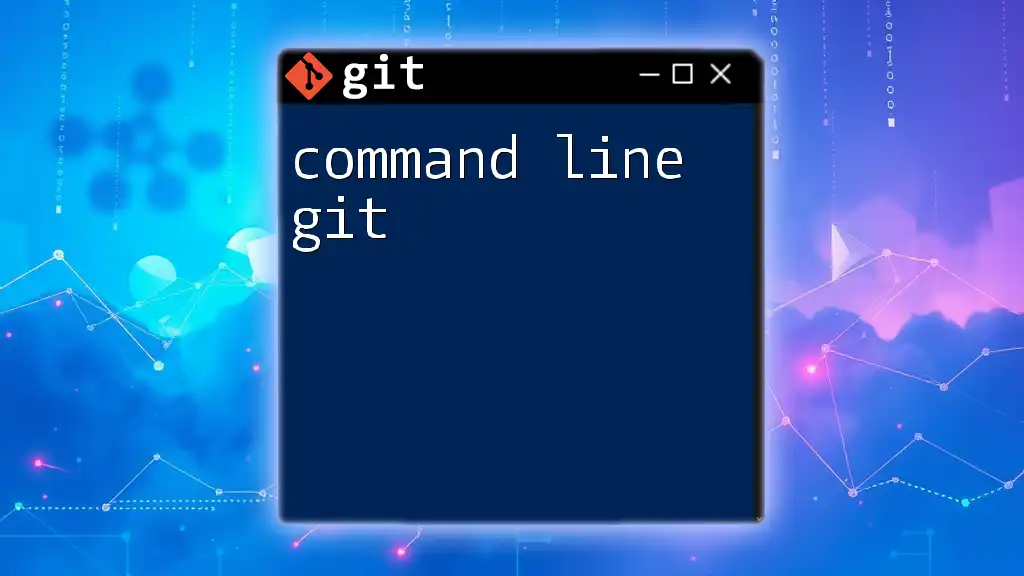
How to Clone a Repository
Cloning a Public Repository
Cloning a public repository is straightforward, allowing anyone to access and replicate the project. Here’s how to do it:
Open your terminal and run the following command:
git clone https://github.com/username/repo.git
In this command:
- Replace `https://github.com/username/repo.git` with the actual URL of your desired repository.
Once executed, Git will create a local copy of the repository in a directory named `repo` within your current working directory. The output will include information about the cloning process, indicating how many objects were received and processed.
Cloning a Private Repository
To clone a private repository, you need to have appropriate access permissions. Typically, this means generating and using an SSH key or personal access token for authentication. Here’s an example of how to do it using SSH:
git clone git@github.com:username/repo.git
Make sure that your SSH key is added to your GitHub or relevant Git service account for successful authentication.
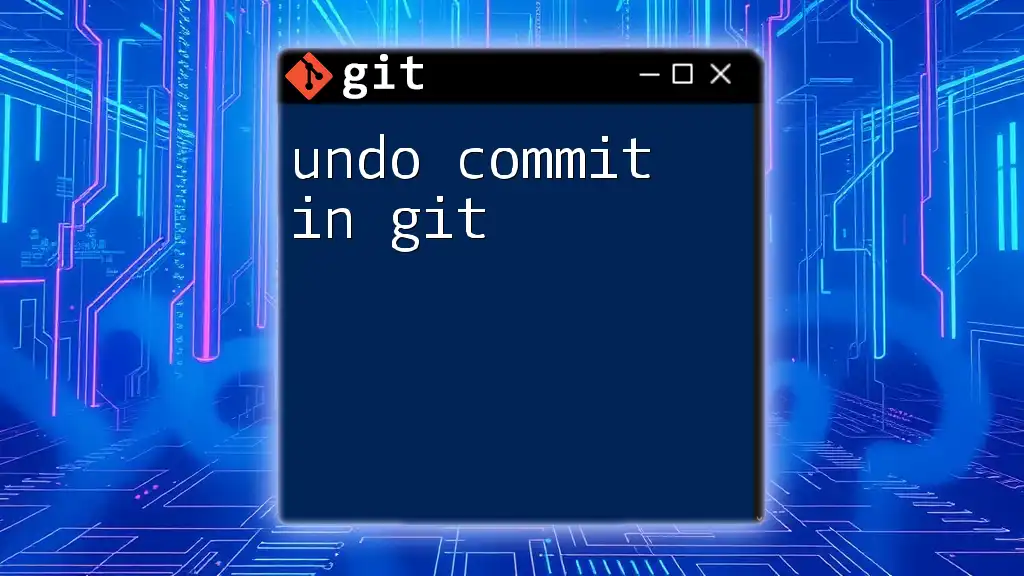
Cloning with Different Options
Cloning a Specific Branch
Sometimes, you may only want to clone a specific branch of a repository instead of the entire project history. You can do this with the following command:
git clone -b <branch-name> <repository_url>
In this command:
- Replace `<branch-name>` with the branch you want to clone.
- This option is particularly useful for large repositories where the master branch is not needed, or you are only interested in development on a specific feature branch.
Cloning with Depth
If you want to clone only the latest snapshot of a project without the entire commit history, you can perform a shallow clone using the `--depth` option. This is especially helpful for large repositories, as it significantly reduces download time.
git clone --depth 1 <repository_url>
Here, `1` indicates that only the latest commit will be retrieved. Use shallow cloning when you’re interested in the current state of the repository without needing the full history.
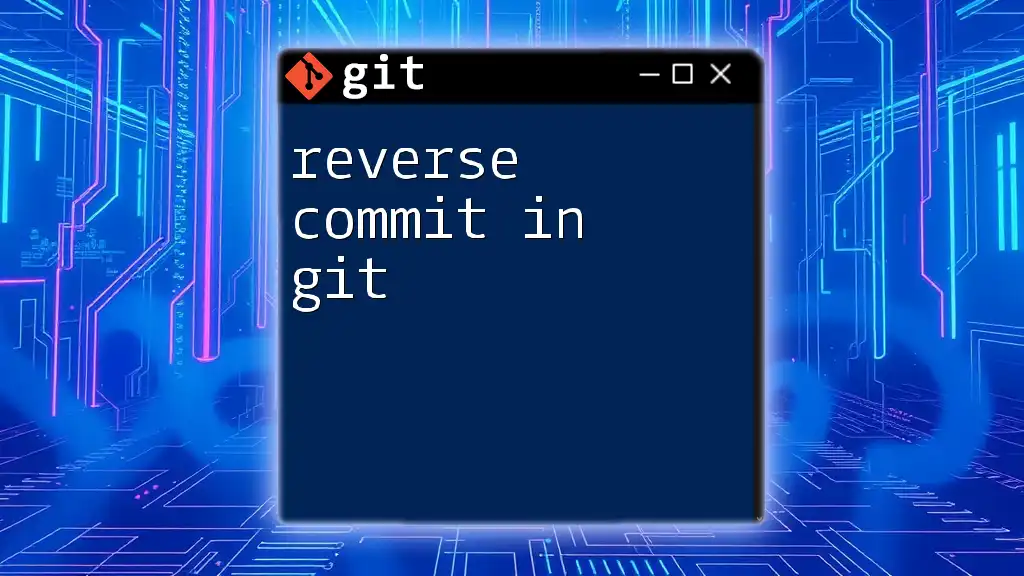
Post-Cloning Actions
Navigating Into the Cloned Repository
Once your repository is successfully cloned, you will want to navigate into it. You can do this with the `cd` command:
cd repo
Checking Remote Repository Information
To verify that your local copy is correctly linked to the remote repository, use the following command:
git remote -v
This command will display the remote URLs associated with your local repository. Ensure that these match with the intended source to confirm that your clone was successful.
Initializing Local Development
After cloning, it’s common to set up your local development environment. Begin by creating a new branch for your changes:
git checkout -b <new-branch>
This will help keep your modifications organized and facilitate smoother collaboration when pushing changes later.
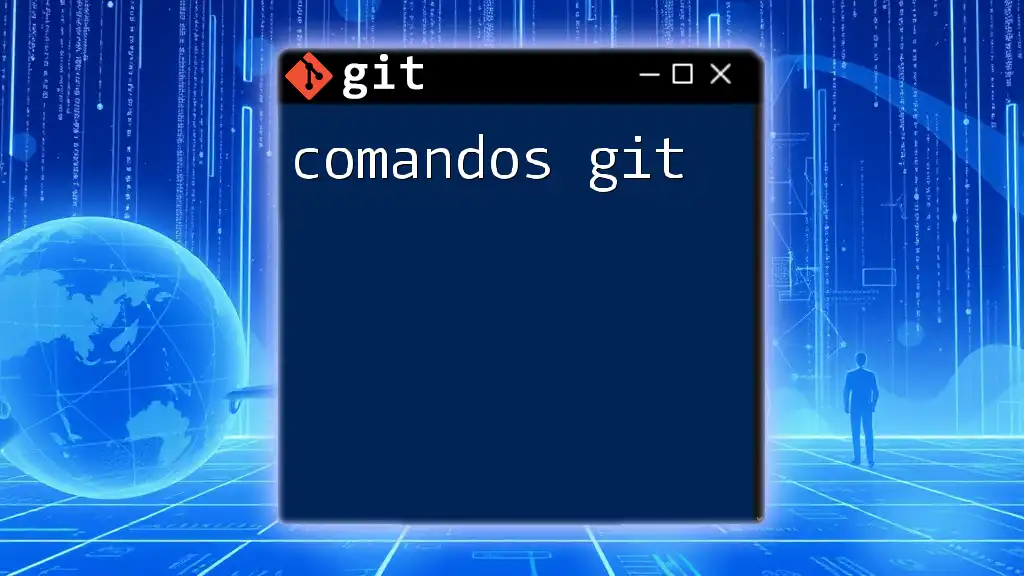
Common Issues and Troubleshooting
Errors During Cloning
While using the clone command in git, you might encounter various errors. Here are a few common ones and how to troubleshoot them:
- Permission Denied: This usually occurs when trying to clone a private repository without proper access rights. Check your authentication setup or verify that you have the right permissions.
- Repository Not Found: Ensure that you’re using the correct URL and that the repository exists.
Ensuring Up-to-Date Clones
After cloning a repository, it's important to keep your local version synchronized with the remote. To fetch the latest changes and updates from the remote repository, use the command:
git pull
This command will ensure your local copy reflects any new changes made by other collaborators.
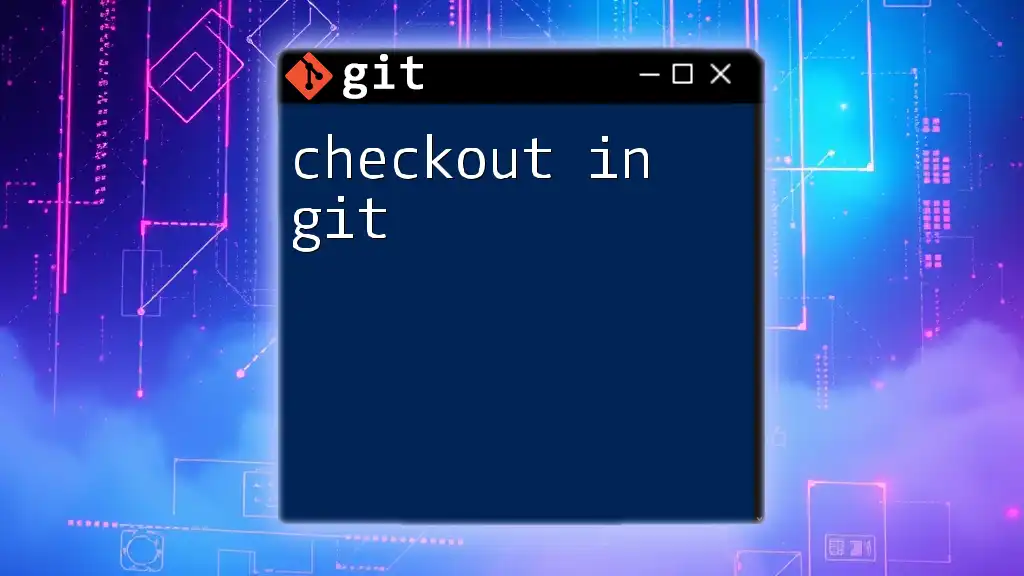
Conclusion
Mastering the clone command in git is essential for anyone looking to engage in collaborative software development. By understanding its syntax, options, and typical use cases, you can efficiently manage your workflow and contribute to projects with confidence. Whether you are cloning public repositories or collaborating on private ones, this powerful command will be a key tool in your development toolkit.
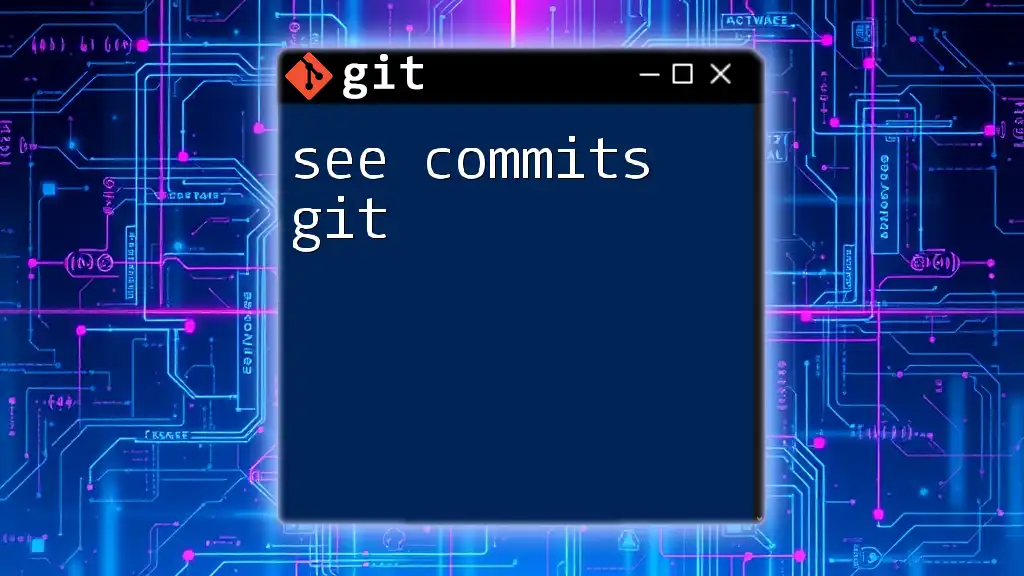
Additional Resources
- For further reading, consult the [official Git documentation](https://git-scm.com/doc).
- Explore online tutorials and videos that provide hands-on demonstrations of the clone command in git and other Git functionalities.