To rollback a commit in Git, you can use the `git reset` command to move the branch pointer back to the desired commit, effectively undoing any changes made in later commits.
git reset --hard HEAD~1
Understanding Git and Commits
What is Git?
Git is a powerful version control system that helps developers manage changes to their codebase. It allows multiple contributors to work on different parts of a project simultaneously while tracking every modification made over time. Key features of Git include branching, merging, and its ability to handle large projects effectively, making it a preferred choice among software developers.
Defining a Commit
In Git, a commit represents a snapshot of your project at a specific point in time. Each commit contains metadata, including a unique identifier (commit hash), the author's information, and a timestamp. Commits are crucial for tracking changes and collaboration in a project, as they allow teams to revert to earlier versions of files easily and maintain a history of modifications.
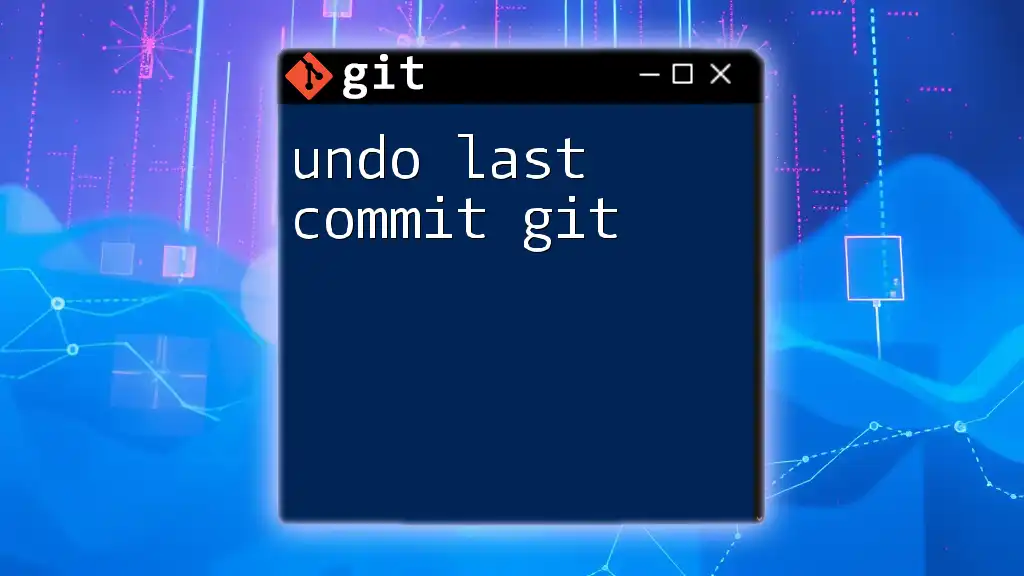
What is Rollback?
Definition of Rollback in Version Control
Rollback in the context of version control refers to the process of reverting the state of your repository to a previous commit. This is particularly useful when you have accidentally introduced errors or when a new feature does not function as intended. Rolling back helps maintain the integrity of your code and provides a way to recover your project from unwanted changes.
Difference between Rollback and Revert
While both rollback and revert aim to undo changes, they operate differently in Git. Rollback typically means resetting your repository to a previous state, often discarding changes after a specific commit. In contrast, reverting creates a new commit that undoes the changes made by a particular commit, preserving the commit history.
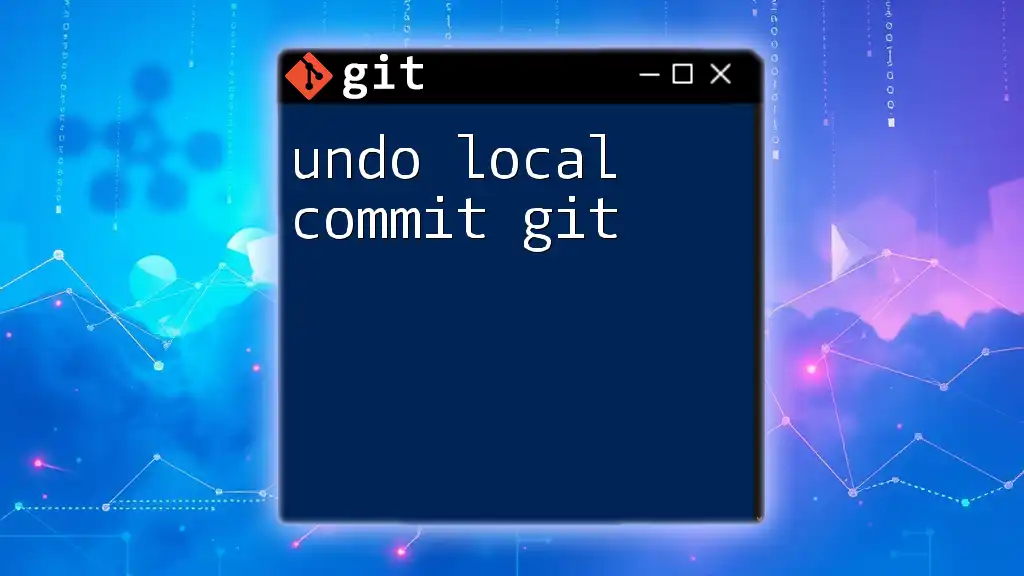
Types of Rollbacks in Git
Using `git reset`
The `git reset` command allows you to move the HEAD pointer to a specified commit, effectively changing the current state of your working directory. There are three types of reset:
-
`--soft`: Moves HEAD to a specified commit without altering the staging area or working directory. Use this option when you want to make additional changes before re-committing.
git reset --soft <commit_hash>
-
`--mixed` (default): Resets the staging area, keeping your working directory intact. It’s useful if you want to unstage changes without losing them.
git reset --mixed <commit_hash>
-
`--hard`: This option resets the index and working directory to match the specified commit. Warning: This will permanently delete any changes after the specified commit.
git reset --hard <commit_hash>
Using `git checkout`
The `git checkout` command allows you to navigate to a previous commit without modifying the current branch. This is particularly useful for testing or exploring past states without affecting the current state of your repository.
git checkout <commit_hash>
Using `git revert`
`git revert` is a safe way to undo changes made by a specific commit by creating a new commit that negates the changes. This is valuable when you want to keep a record of what has been done while still rolling back a faulty commit.
git revert <commit_hash>
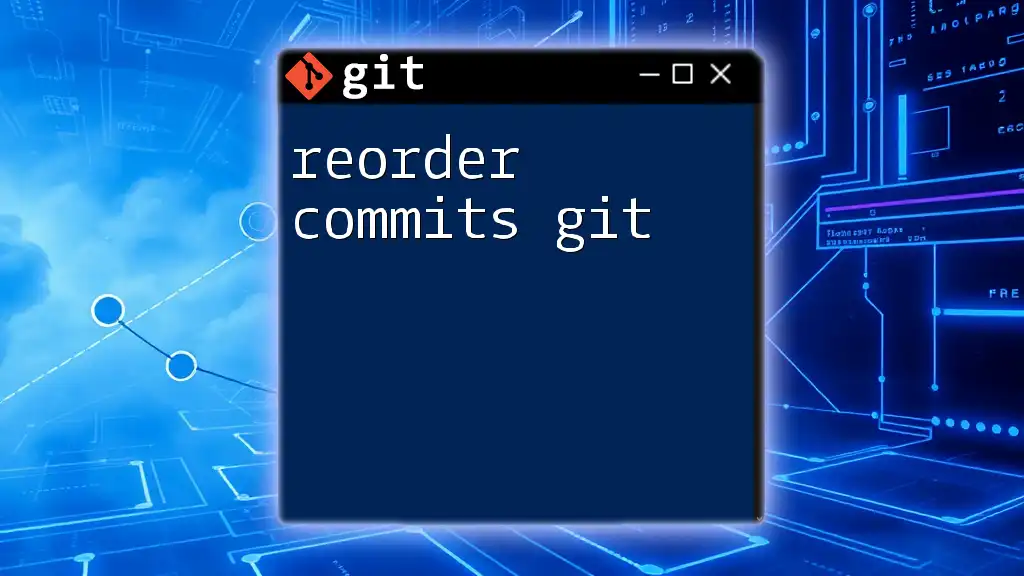
When to Use Each Rollback Method
Advantages and Disadvantages of `git reset`
- Advantages: Quick way to remove unwanted commits and can be tailored to leave changes written either to the staging area or working directory.
- Disadvantages: High risk of losing data, particularly with the `--hard` option. Use it with caution, especially when working with shared repositories.
Advantages and Disadvantages of `git revert`
- Advantages: Maintains a complete history of changes, making it ideal for shared repositories.
- Disadvantages: Can clutter commit history if used frequently, as each revert creates a new commit.
Advantages and Disadvantages of `git checkout`
- Advantages: Enables exploration of previous states without modifying the current branch, allowing you to experiment freely.
- Disadvantages: Can lead to a detached HEAD state if you forget to return to your branch, which can be confusing.
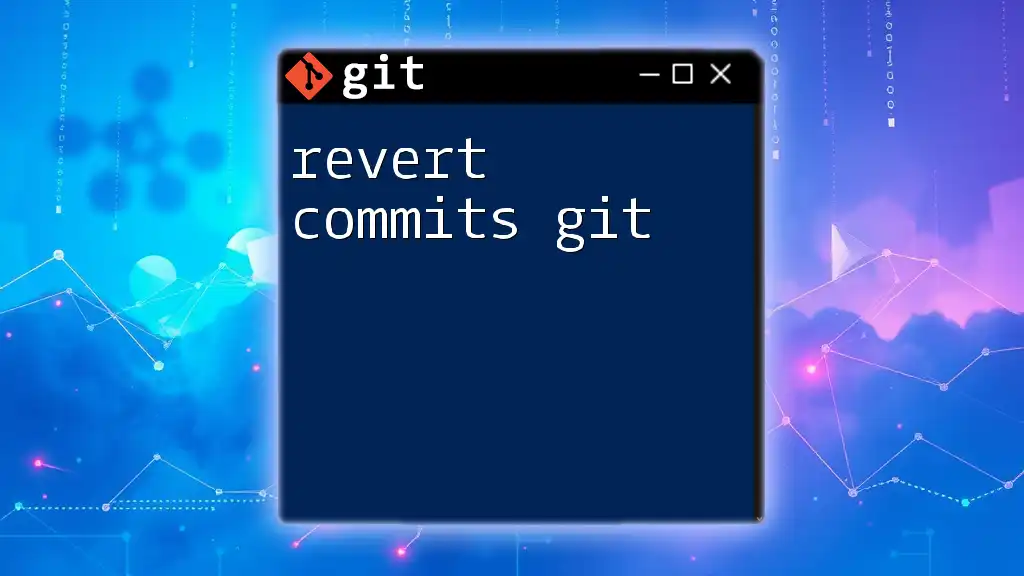
Step-by-Step Guide to Rolling Back a Commit
Pre-Rollback Considerations
Before rolling back a commit, ensure all changes are committed or safely stashed. This precaution helps prevent loss of work and prepares your repository for a smooth rollback process.
Step-by-Step Process for Each Method
Rolling Back with `git reset`
- Identify the commit hash to which you want to revert.
- Choose the appropriate reset option (`--soft`, `--mixed`, or `--hard`).
- Execute the command.
For example, to reset the last three commits:
git reset --hard HEAD~3
Rolling Back with `git revert`
- Find the commit hash of the changes you want to undo.
- Use the revert command to create a new commit negating the specified changes.
To revert a specific commit:
git revert <commit_hash>
- If conflicts arise, resolve them before finalizing the revert.
Rolling Back with `git checkout`
- Determine the target commit hash.
- Checkout the commit, remembering that you will enter a detached HEAD state.
git checkout <commit_hash>
- Make sure to return to your branch once you finish to avoid confusion.
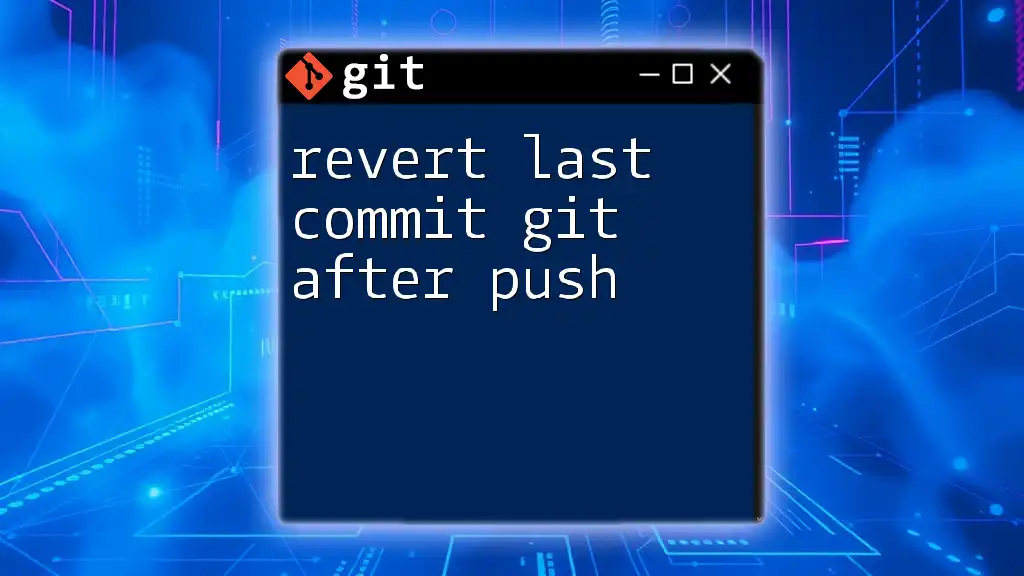
Common Scenarios for Rollback
Undoing a Recent Commit
Imagine you pushed a commit that introduced bugs. By using `git reset` with `--hard`, you can remove that commit altogether.
Recovering from a Mistaken Merge
If you merged a branch that caused issues, find the merge commit hash, and either use `git revert` to negate the merge or `git reset` if you want a clean slate.
Testing and Experimentation
During feature development, it can be easy to divert from your original plan. Utilize `git checkout` to test previous commits without affecting ongoing work.
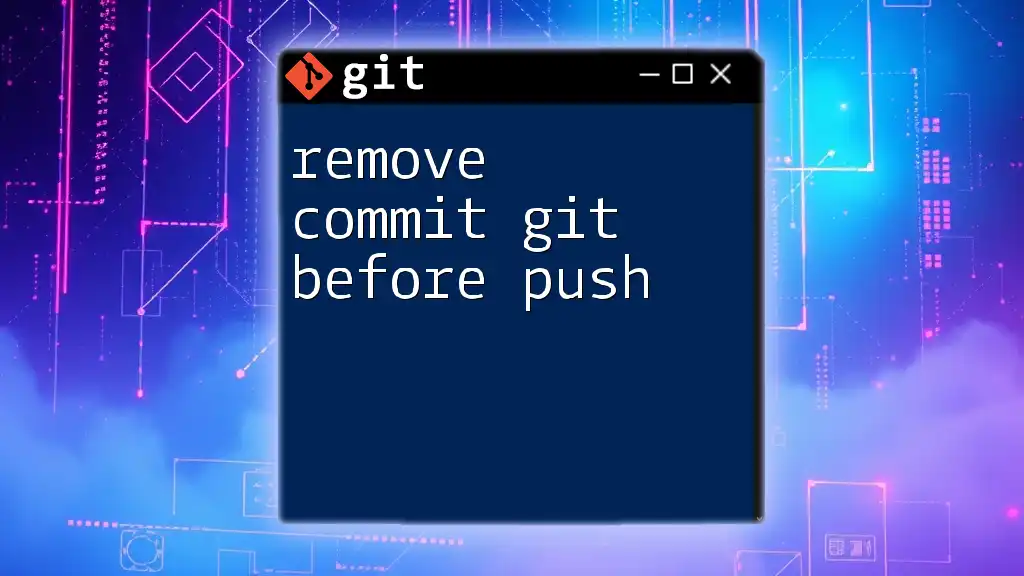
Best Practices for Working with Git Rollbacks
Documenting Commit Messages
Ensure that commit messages are clear and descriptive. This practice helps identify which changes may need to be reverted or modified later.
Utilizing Branches for New Features
Always develop new features in separate branches. This strategy keeps your main branch stable and makes rollbacks simpler if experiments fail.
Regular Backups and Stashing
Use `git stash` to temporarily save your changes instead of committing incomplete work. This practice minimizes the risk of losing data when rolling back commits.
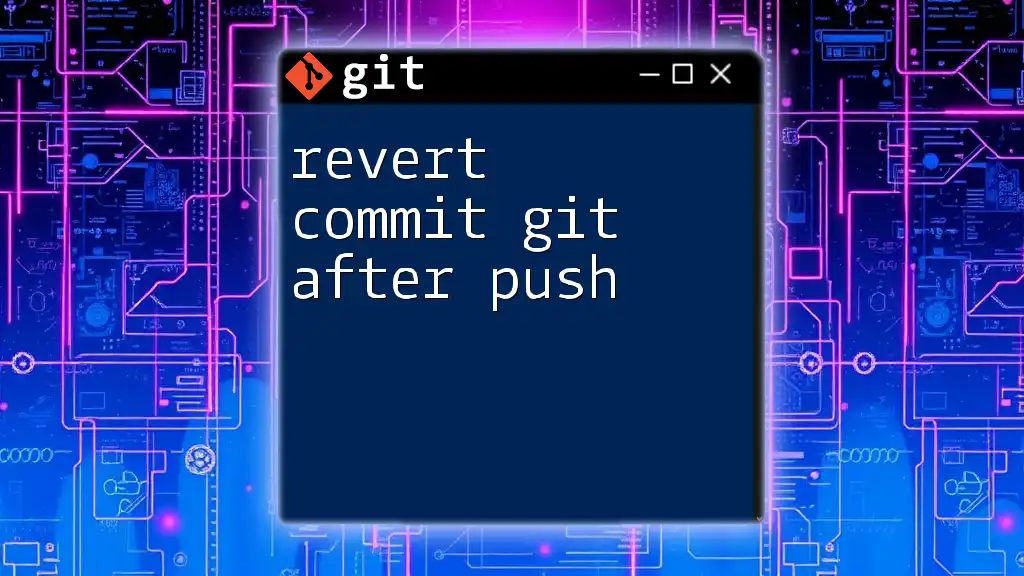
Troubleshooting Rollback Issues
Common Errors
Common mistakes include the accidental deletion of changes when using `git reset --hard`. Always double-check which commits you are resetting to and ensure that you have backups in place.
Helpful Git Commands
- `git reflog`: This command helps you recover lost commits by showing a log of where your repos have pointed in the past.
- `git status`: Use this to check the current state of your repository, ensuring that you have not left any important changes unstaged or uncommitted.
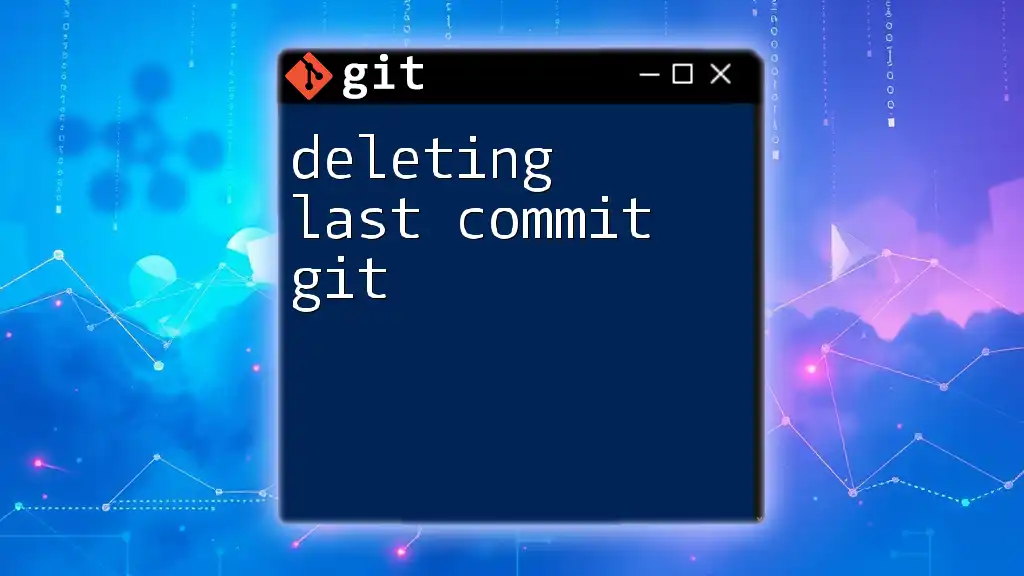
Conclusion
Rolling back commits in Git is a powerful feature that empowers developers to manage their code effectively. Understanding the differences between methods such as `git reset`, `git revert`, and `git checkout`, as well as knowing when to use each, will keep your workflow smooth and efficient. By adhering to best practices and utilizing helpful commands, you'll ensure a robust, reproducible development experience.