To remove a pushed commit in Git, you can use the reset command along with the force push option to overwrite the remote history.
git reset --hard HEAD~1 && git push origin main --force
Understanding Git Commits
What is a Git Commit?
A Git commit represents a snapshot of your project at a certain point in time. Each commit includes metadata, such as the author, timestamp, and a unique commit ID. Understanding commits is fundamental because they form the backbone of version control, allowing you to track changes and collaborate effectively.
Why Would You Remove a Pushed Commit?
Removing a pushed commit is sometimes necessary for various reasons:
- Fixing Mistakes: A commit might contain bugs or incorrect information that you want to rectify.
- Removing Sensitive Data: If you accidentally pushed a commit containing passwords or API keys, it's crucial to remove it immediately for security reasons.
- Cleaning Up Commit History: Sometimes, a complex or cluttered commit history can make it difficult to navigate through changes. Cleaning it up can improve clarity for yourself and your team.
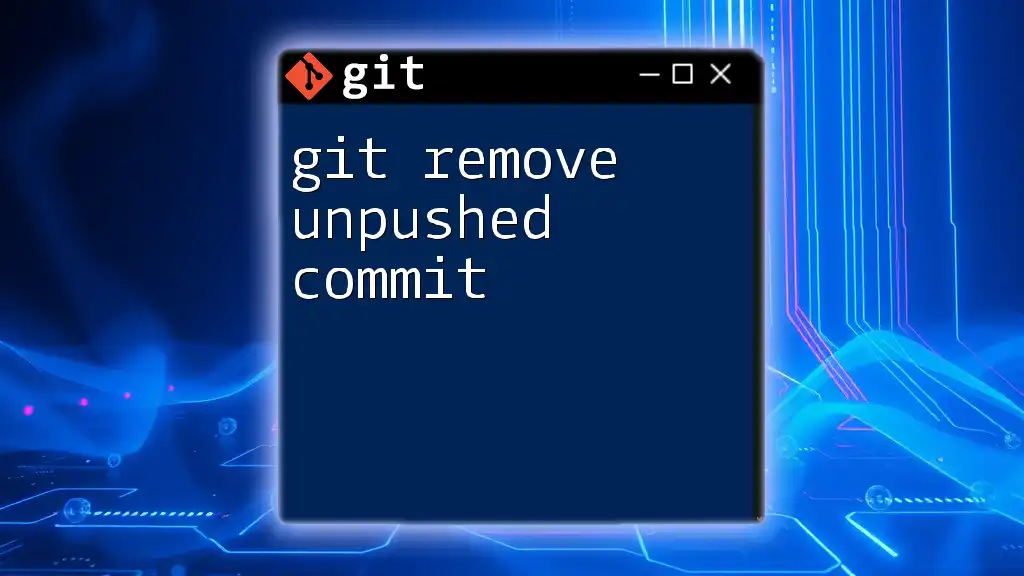
Ways to Remove a Pushed Commit
Using `git revert`
The `git revert` command allows you to reverse changes made by a previous commit, creating a new commit in the process. This method is beneficial because it keeps your commit history intact while performing an "undo" operation.
Pros:
- Maintains a complete history of changes.
- Safe to use in collaborative environments.
Cons:
- The history can become cluttered with "revert" commits.
To revert a commit, use the following command:
git revert <commit-id>
After running this command, Git will create a new commit that negates the changes of the specified commit. You can then push this new commit like so:
git push origin branch-name
Using `git reset`
The `git reset` command allows you to move the current branch pointer to a different commit, effectively removing commits from your branch's history.
Types of Reset:
- Soft Reset: Retains your changes in the staging area.
- Mixed Reset: Retains your changes in the working directory (default).
- Hard Reset: Discards all changes.
Pros:
- Allows you to selectively undo recent changes.
Cons:
- Using a hard reset can lead to data loss.
To perform a soft reset for the latest commit, use:
git reset --soft HEAD~1
This moves the HEAD pointer back one commit while keeping your changes in the staging area. If you make further adjustments, you can commit again.
For a hard reset, which removes changes entirely, run:
git reset --hard HEAD~1
WARNING: A hard reset is dangerous and should be used with caution, especially in a shared repository.
Using `git rebase`
The `git rebase` command allows you to rewrite commit history. This is particularly useful for cleaning up commits before merging branches.
To remove a specific commit, you can initiate an interactive rebase:
git rebase -i HEAD~3
This command brings up a text editor where you can modify commits. You can mark the commits to be removed. After saving, Git will replay the remaining commits.
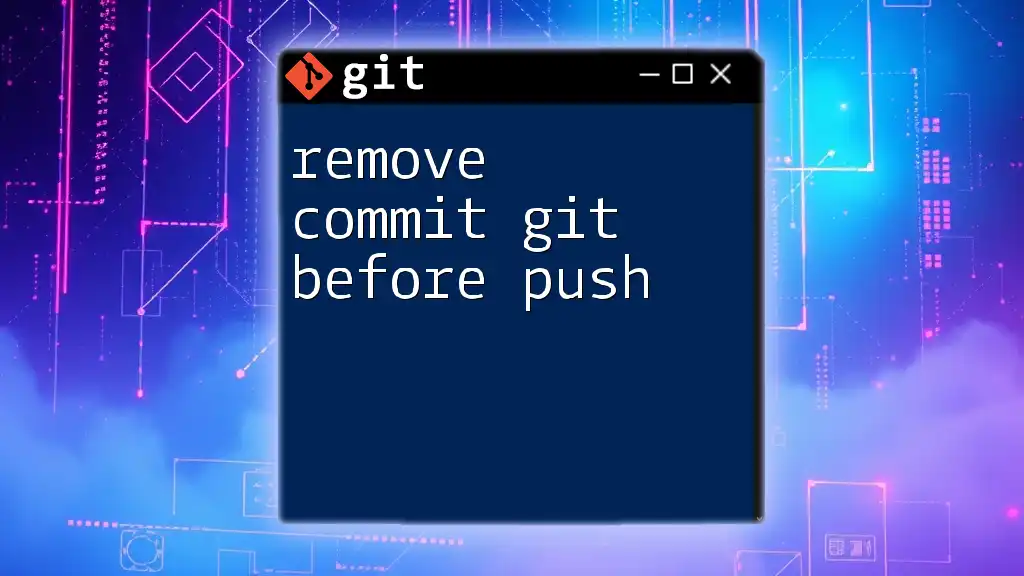
Best Practices for Removing Pushed Commits
Communicating with Your Team
Communication is key when working in collaborative environments. Inform your team before making changes to shared branches. This simple step can prevent confusion and potential conflicts in project history.
Back Up Your Work
Before making any significant changes, especially when removing commits, create a backup branch. This is a safeguard to ensure you can restore your work if needed:
git branch backup-branch-name
This command safeguards your progress and makes it easy to revert back if necessary.
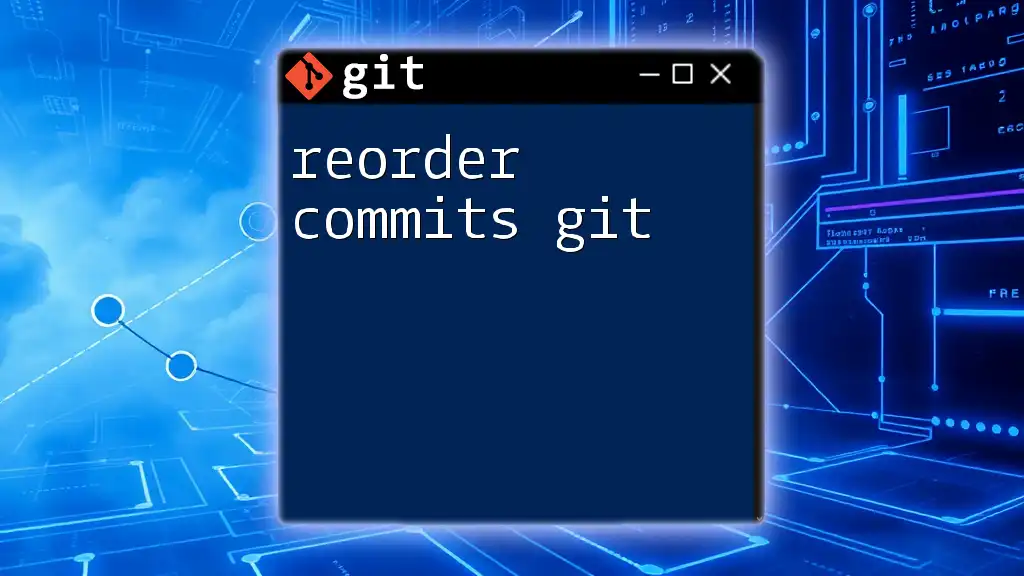
Potential Issues and How to Resolve Them
Conflicts During Rebase or Reset
When executing a rebase or reset, conflicts may arise if your changes overlap with those made by other collaborators. If this happens, Git will prompt you to resolve conflicts before continuing the operation.
To resolve conflicts:
- Identify the files with conflicts.
- Edit them to resolve differences.
- After resolving, stage the changes with:
git add <conflicted-file>
- Finally, continue the rebase with:
git rebase --continue
Force-Pushing Changes
Using `git push --force` can overwrite changes on the remote repository. This method is dangerous but sometimes necessary after a reset or rebase. Always ensure that your team is aware of such actions to avoid overwriting their work.
git push origin branch-name --force
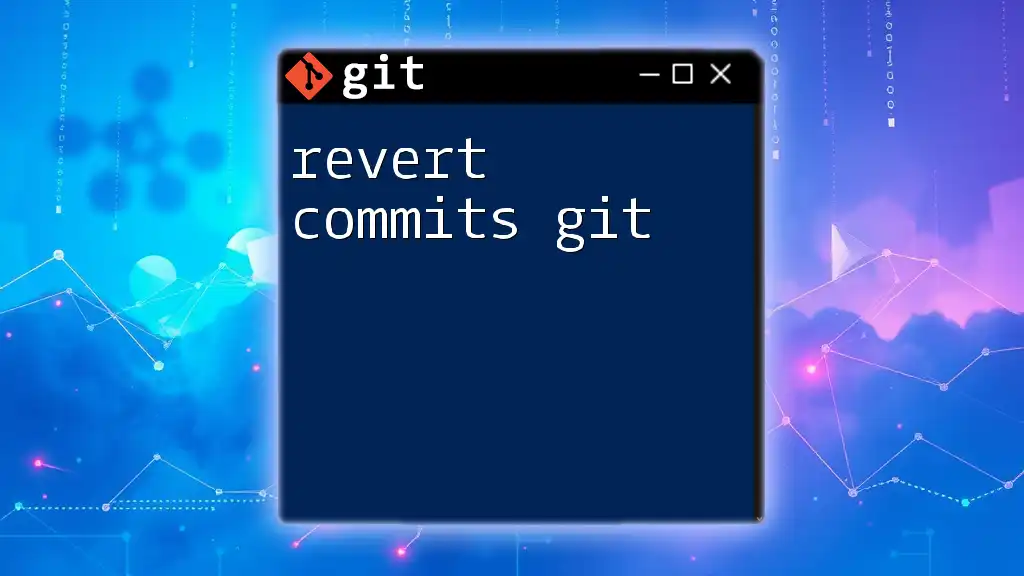
When Not to Remove a Pushed Commit
Avoid removing pushed commits in production environments without careful consideration. It may lead to broken builds or loss of significant history needed for troubleshooting. Always assess the implications on your workflow before proceeding with any removals.
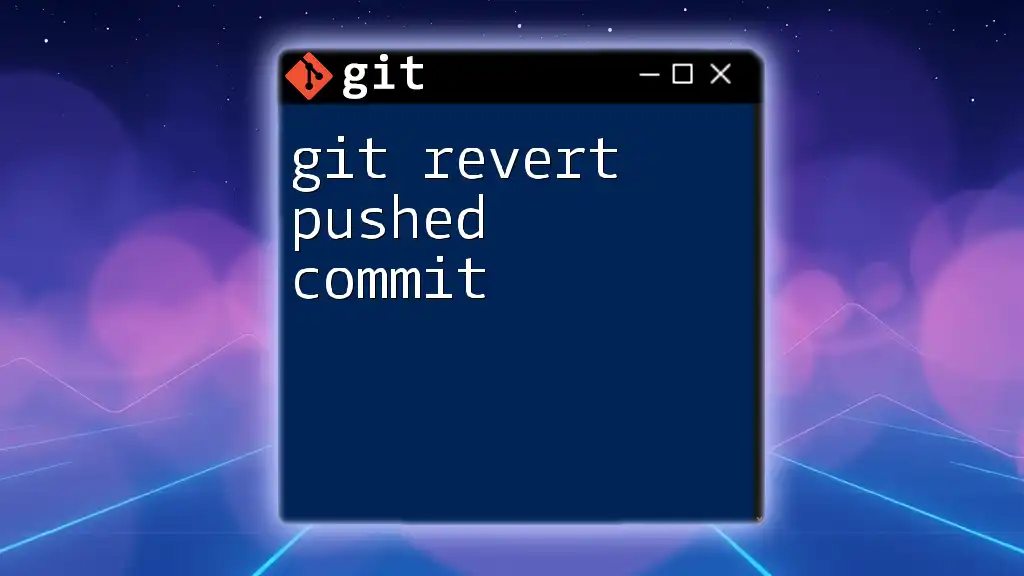
Conclusion
Understanding how to effectively and safely remove pushed commits in Git is an essential skill for developers. Whether you're fixing mistakes, maintaining commit history, or improving team collaboration, the methods outlined here will serve you well. Practice these techniques responsibly and always communicate with your team to ensure a smooth workflow.
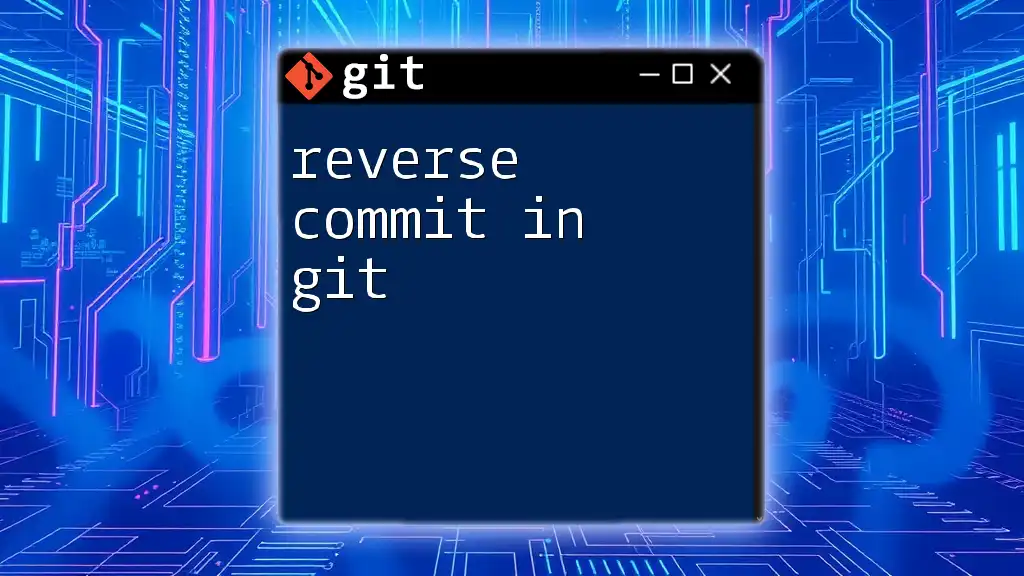
Additional Resources
For further exploration, visit the official Git documentation or consider taking advanced Git courses to deepen your understanding of version control. Happy coding!