To revert a commit in Git after it has been pushed, you can use the `git revert` command followed by the commit hash, which creates a new commit that undoes the changes made by the specified commit.
Here's the command in markdown format:
git revert <commit-hash>
Replace `<commit-hash>` with the actual hash of the commit you want to revert.
Understanding Git and Its Repositories
What is Git?
Git is a distributed version control system that enables individuals and teams to track changes in files and collaborate on projects. Its primary purpose is to manage source code during software development, but it can also be used for other file types. By using Git, teams can maintain a complete history of their projects, making it easier to revert to previous versions or collaborate across different environments.
What Happens When You Push?
When you execute a `git push` command, you transfer committed changes from your local repository to a remote repository. This action affects both local and remote branches, and it effectively updates the remote state with any new commits you've added locally. Understanding this process is crucial, as it establishes a path for cooperation among team members while also putting the responsibility of maintaining commit integrity on the developer.
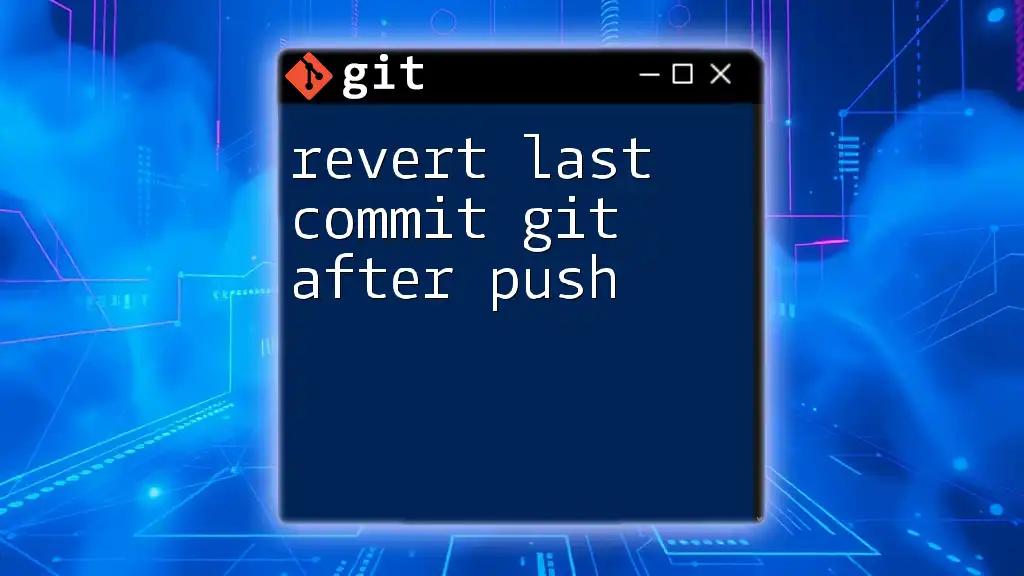
Reasons to Revert a Commit
Common Scenarios for Reverting
There are several scenarios in which you might need to revert a commit:
- Mistaken commits: You accidentally committed the wrong files or added leftover debug code.
- Errors in code: A commit introduces bugs that break the application.
- Version corrections: You need to revert to a stable version after introducing an unstable or incomplete feature.
The Importance of Clean History
Maintaining a tidy commit history is vital for clarity in collaborative projects. A well-managed history makes it easier to track changes, understand decisions made over time, and isolate issues as they arise. Adhering to best practices, such as committing only completed and tested features, helps ensure that your project maintains its integrity.
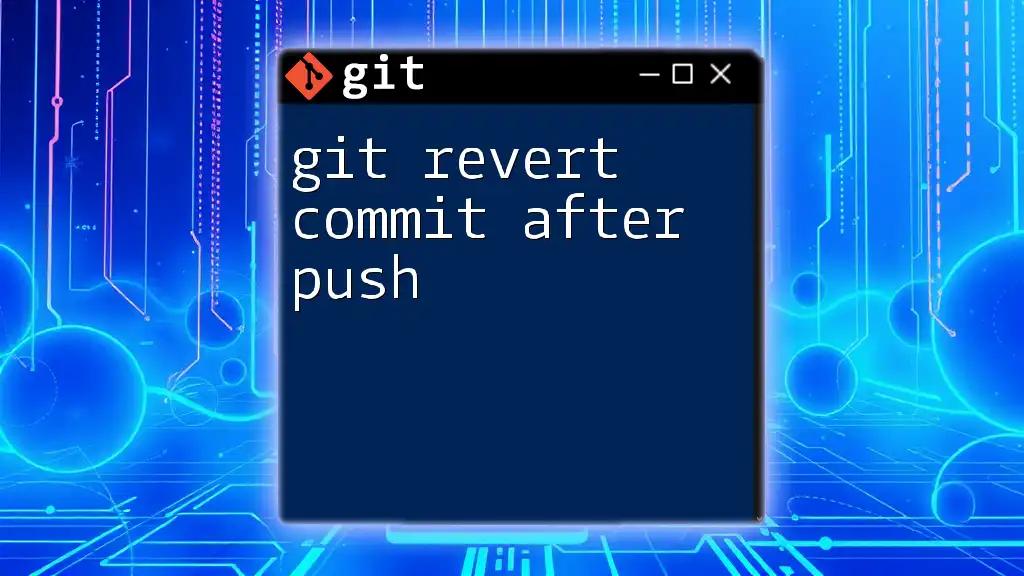
Reverting a Commit: An Overview
What Does It Mean to Revert a Commit?
To revert a commit in Git means to create a new commit that undoes the changes introduced by a previous commit. Unlike resetting a commit, which removes history, reverting preserves the integrity of the commit history. This distinction is critical, particularly when working in collaborative environments.
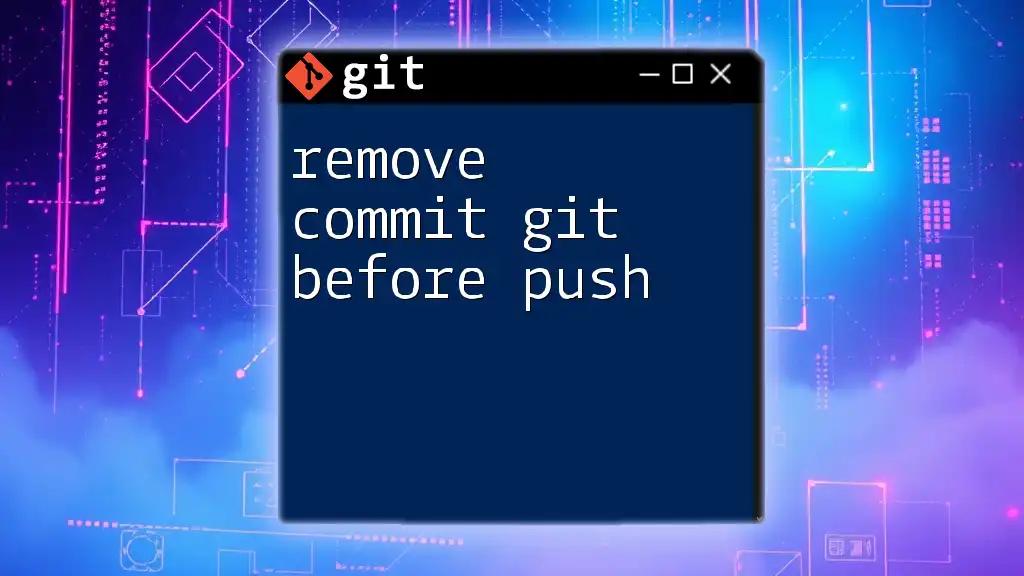
Pre-requisites for Reverting a Commit
Permissions and Access
Before reverting a commit, it is essential to ensure that you have the necessary permissions for the remote repositories. Check your access rights to confirm that you can push changes back to the remote repository. This helps prevent unexpected issues when attempting to revert directly on shared branches.
Confirming Your Repository Status
Using `git status` will help you verify the current state of your repository, including which branch you are on and any changes that are staged for commit. Knowing this information is essential to ensure you are targeting the correct version of your code.
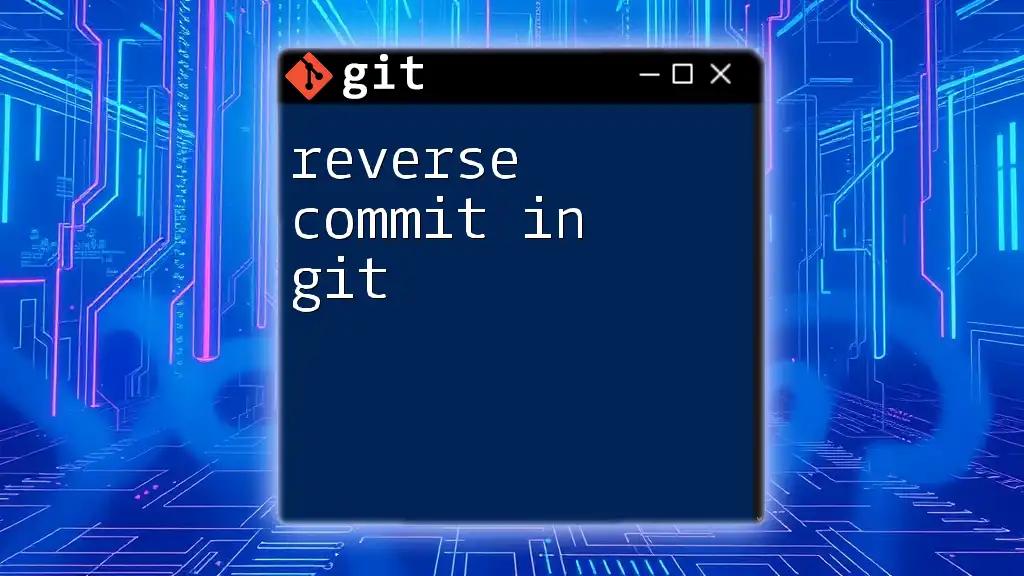
Steps to Revert a Commit After Push
Identify the Commit to Revert
To revert a commit, you first need to identify which commit you intend to undo. You can find the commit hash by using the `git log` command, which displays the history of commits along with their hashes.
To see your commit history, run:
git log
This command will output a list of commits, showing commit hashes, author information, and commit messages. Once you locate the hash of the commit you wish to revert, take note of it.
Reverting the Commit
Using the Git Revert Command
You can revert a commit using the `git revert` command, which allows you to undo changes made by the specified commit.
The syntax is as follows:
git revert <commit_hash>
Code Example
If you want to revert a commit with hash `a1b2c3d`, you would execute:
git revert a1b2c3d
After this command is run, Git will create a new commit that reverses the changes made in the specified commit. Git will prompt you to enter a commit message, which you should do to provide clarity on why the revert occurred.
Dealing with Merge Conflicts
Understanding Merge Conflicts
When reverting a commit, especially if the changes you're reverting have since been modified in other commits, you could encounter merge conflicts. Merge conflicts occur when Git cannot automatically reconcile changes from different commits.
Resolving Merge Conflicts
When a conflict arises, Git will inform you, and you must manually resolve these conflicts. Use `git status` to see which files are in conflict. After resolving the conflicts directly within the code files (e.g., using a text editor), you can stage the changes by running:
git add <file_name>
Finally, commit the resolved changes:
git commit
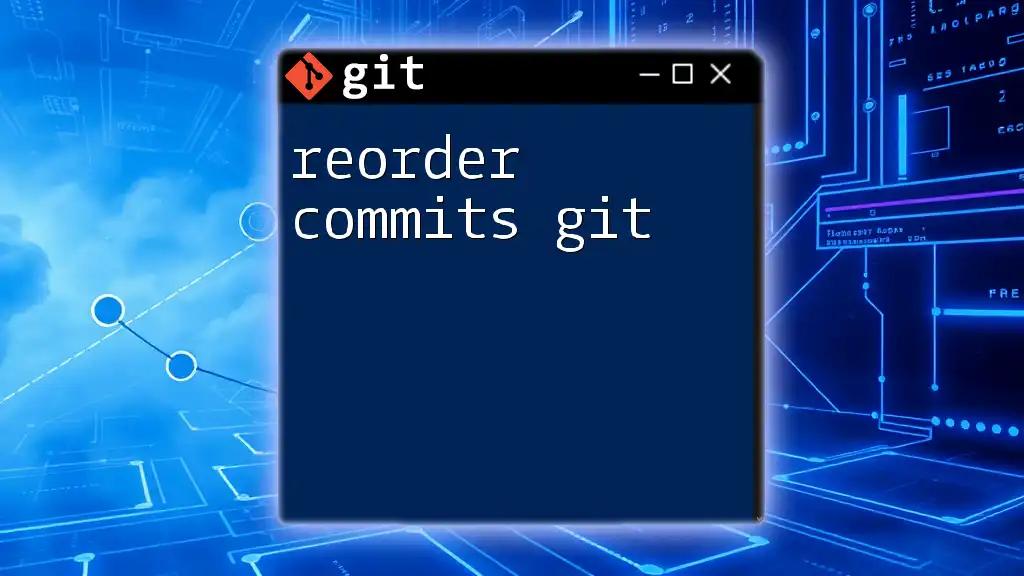
Pushing the Reverted Commit
How to Push Changes to Remote
After successfully reverting a commit, you need to push your changes to the remote repository. Ensure you are on the branch that corresponds to your work.
Use the following command to push:
git push origin <branch_name>
Confirming the Changes
To ensure that your revert has been successfully applied, you can review your commit history again using `git log`. This will show the new commit that has been created as a result of the revert, allowing you to verify that the changes are reflected in the remote repository.
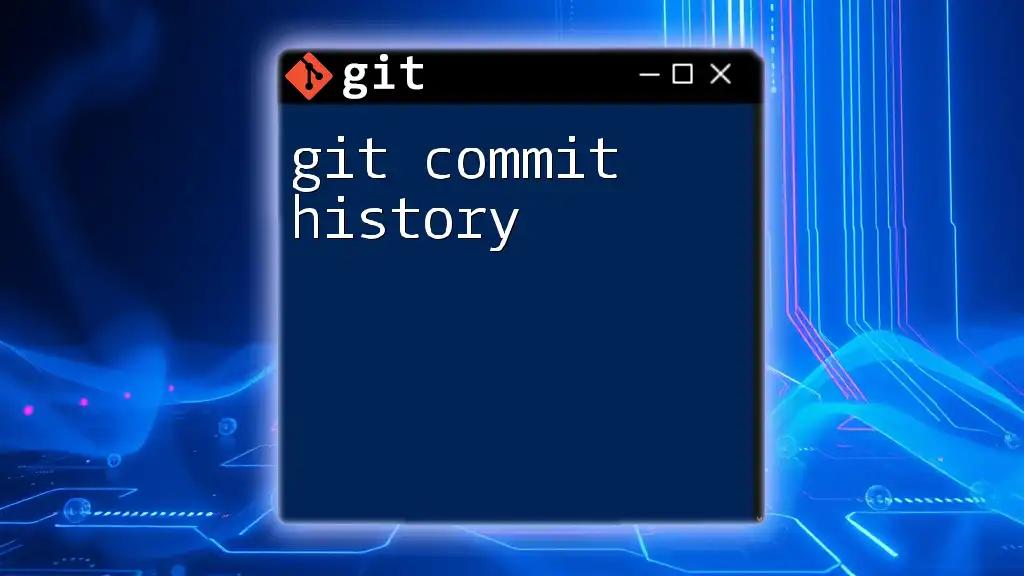
Alternative Methods to Undo a Commit
Using Git Reset (with caution)
An alternative to reverting is using `git reset`, which can also undo changes but may cause issues in collaborative environments. This command can remove commits entirely, changing history.
Difference between Revert and Reset
- Revert: Preserves history by creating a new commit that undoes changes.
- Reset: Alters history by removing commits.
To reset to a specific commit:
git reset --hard <commit_hash>
Be extremely cautious with this command, as it will delete data and cannot be easily recovered.
Amend Last Commit
If you need to make changes to the last commit before pushing, you can use the git commit --amend command. This is useful to adjust commit messages or include forgotten changes.
To amend the latest commit:
git commit --amend
This command allows you to incorporate any unstaged changes into the last commit or modify its message.
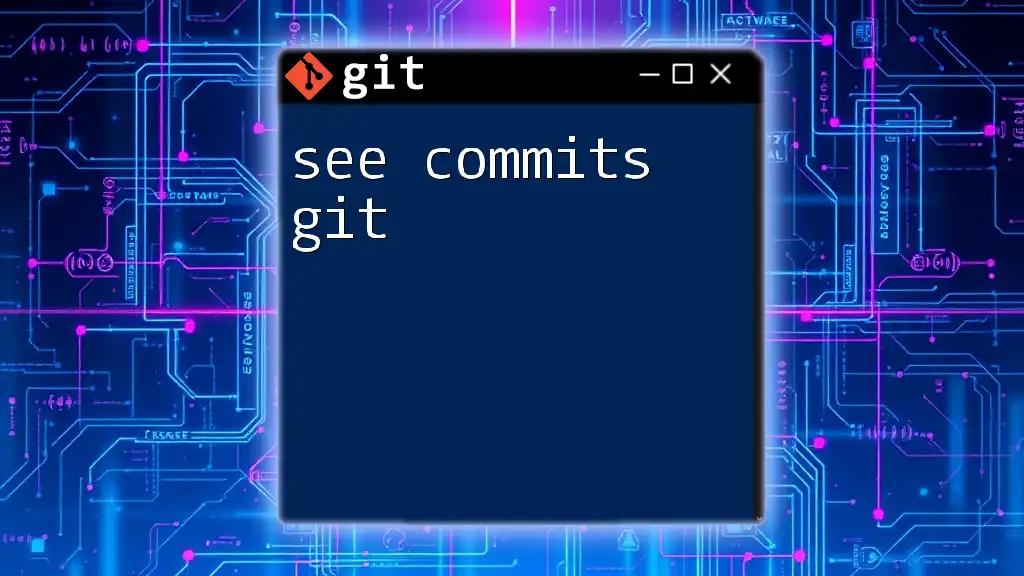
Best Practices for Git Commit Management
Regularly Check Your Commit History
Make it a habit to frequently review your commit history. This practice helps you stay aware of the evolution of your project and can illuminate potential areas for improvement or correction.
Use Meaningful Commit Messages
Clear, descriptive commit messages communicate the purpose of your changes to your team and future contributors. When writing a commit message, consider using the imperative mood and be specific about the intent, which helps maintain professionalism and clarity.
Create Branches for New Features
Creating separate branches for new features or bug fixes keeps the main branch secure and stable. Once the feature is complete and tested, it can easily be merged back into the main branch.
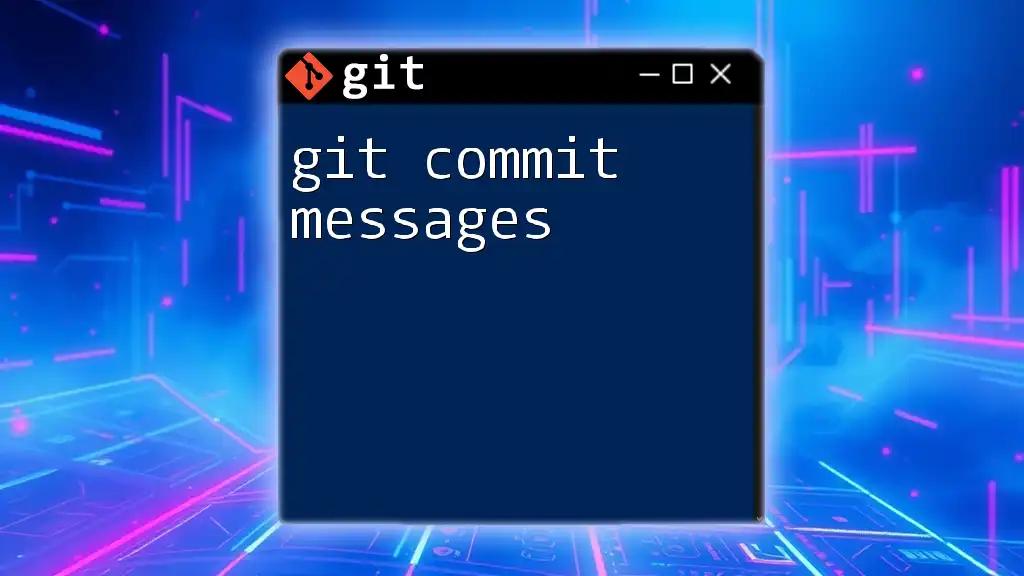
Conclusion
Summary of Steps to Revert a Commit
Reverting a commit after pushing involves identifying the commit hash, executing the `git revert` command, and pushing the changes back to the remote repository. This process is essential for maintaining a clean project history and for managing complex codebases.
Encouragement for Best Practices
Adopting best practices such as branching, reviewing commit history, and writing clear commit messages will lead to more effective collaboration and a successful version control strategy in your projects.
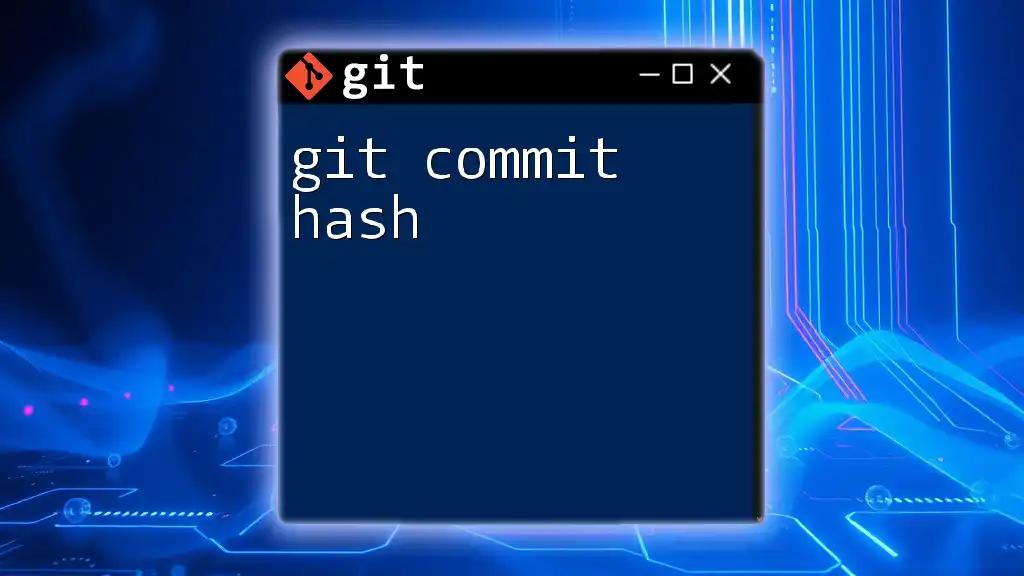
Additional Resources
Documentation and Support
For more detailed information on Git commands and practices, refer to the [official Git documentation](https://git-scm.com/doc). This resource provides comprehensive guidance and examples for every aspect of using Git effectively.