You can reorder commits in Git using an interactive rebase, which allows you to change the order of commits in your branch’s history.
Here's the command to initiate an interactive rebase for the last 5 commits:
git rebase -i HEAD~5
Understanding the Commit History
What is a Commit?
In Git, a commit is essentially a snapshot of your project at a specific point in time. Each commit contains information about changes made, the author, and a unique identifier (hash). The structure of a commit typically includes:
- A unique SHA-1 checksum
- Author and committer details
- The commit message describing the changes
- A reference to the previous commit
Viewing Commit History
Before reordering commits, it’s essential to understand the existing commit history. You can use the `git log` command to view your commits. A basic example of this command is:
git log --oneline
This will show a condensed summary of your commit history, which can help you identify the commits you may want to reorder.
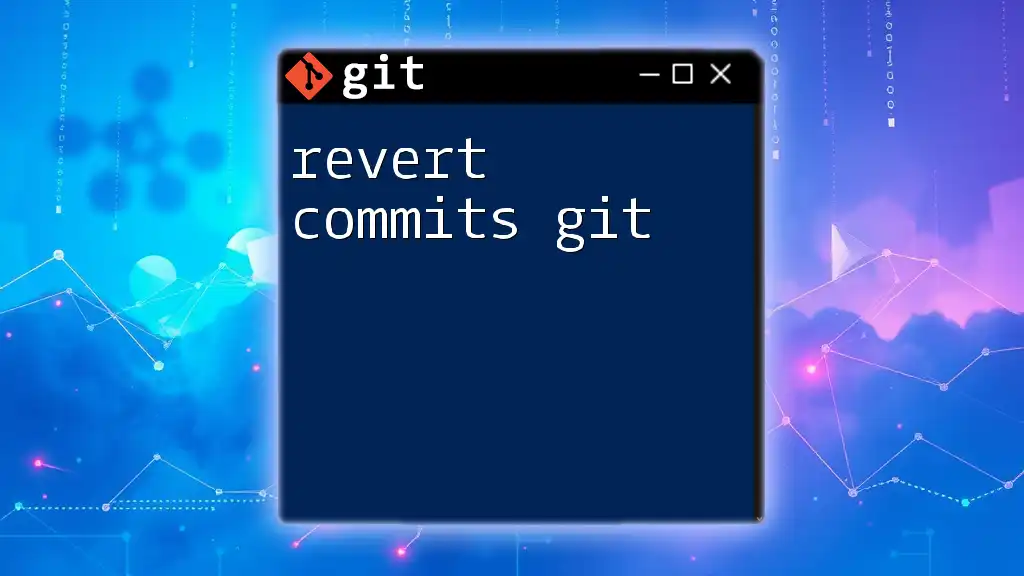
Why Reorder Commits?
Improving Readability of History
One of the primary motivations for reordering commits in Git is to enhance the readability of your commit history. A well-structured commit history provides a clear narrative of how a project evolved over time. By reordering commits, you can ensure that related changes are grouped together logically, making it easier for you and your team members to track development progress.
Fixing Mistakes in Commit Order
When working on multi-commit contributions, it’s common to make mistakes regarding the order of commits. Perhaps a feature was developed before its corresponding tests or earlier commits should appear later in your history. Reordering commits can fix these inaccuracies, providing a more coherent timeline.
Enhancing Collaboration
In collaborative projects, clear communication through commit messages is vital. Reordering commits allows teams to follow the thought process behind changes. By structuring commits in a logical order, you facilitate better understanding and collaboration among team members.
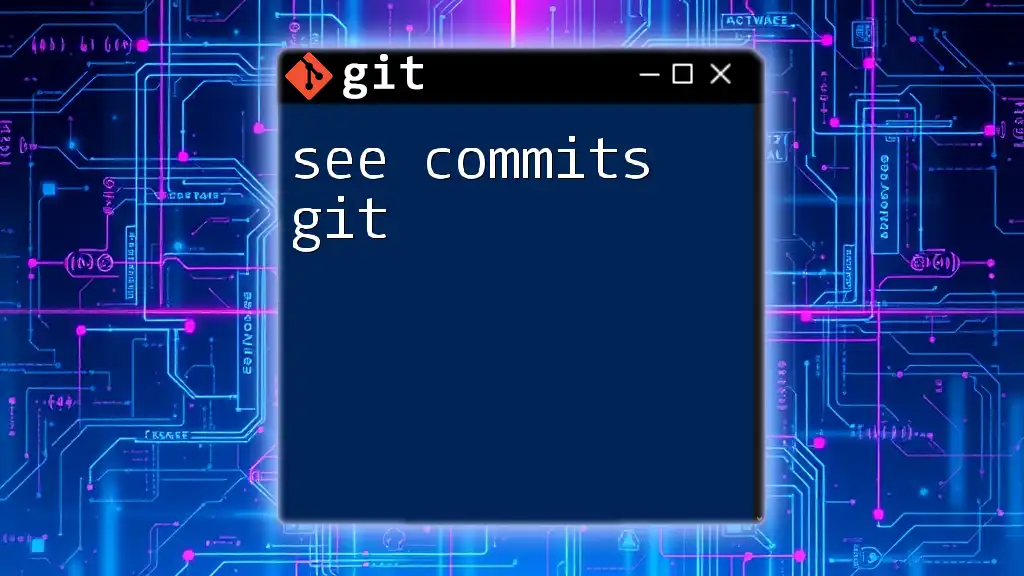
Methods to Reorder Commits
Using Interactive Rebase
What is Interactive Rebase?
Interactive rebase is a powerful feature in Git that allows you to edit your commit history. It provides a way to reorder, squash, or fix up commits.
Command Syntax
To initiate an interactive rebase, you can use the command:
git rebase -i HEAD~N
Replace `N` with the number of recent commits you wish to consider for reordering.
Step-by-Step Process
-
Launch Interactive Rebase
When you run the command, Git opens your default text editor with a list of commits to edit. -
Understanding the Editor Interface
Each commit starts with the word 'pick'. This indicates that the commit should be included in the new history. To reorder commits, simply move the lines up or down as desired. -
Changing 'pick' to 'reword,' 'edit,' or 'drop'
If you wish to change a commit message or remove a commit, replace 'pick' with 'reword' or 'drop', respectively. -
Example: Reordering Commits
Here’s what the editor might look like:pick 1234567 First Commit pick 890abcd Second Commit
If you want to move "Second Commit" before "First Commit," you would rearrange it as:
pick 890abcd Second Commit pick 1234567 First Commit
-
Commit Order Adjustment
After saving and closing the editor, Git will apply the changes. Follow any prompts that may appear regarding merge conflicts or other issues.
Consistent Communication with Team
Whenever you reorder commits in a shared repository, keep your team informed. Notify them about the changes and the reasoning behind your reordering to maintain transparency.
Using the `git cherry-pick` Command
What is Cherry-Picking?
Cherry-picking is another method to reorder commits. It allows you to apply commits from one branch to another without merging the entire branch.
When to Use Cherry-Picking
Cherry-picking is especially useful when you want to take specific commits from one branch and apply them to another, maintaining a clean commit order or isolating certain features.
Code Example: Cherry-Picking a Specific Commit
Here is a basic example of using cherry-pick:
git cherry-pick <commit-hash>
Replace `<commit-hash>` with the SHA-1 hash of the commit you want to include. This approach effectively allows you to reorder commits by creating a new linear sequence of commits.
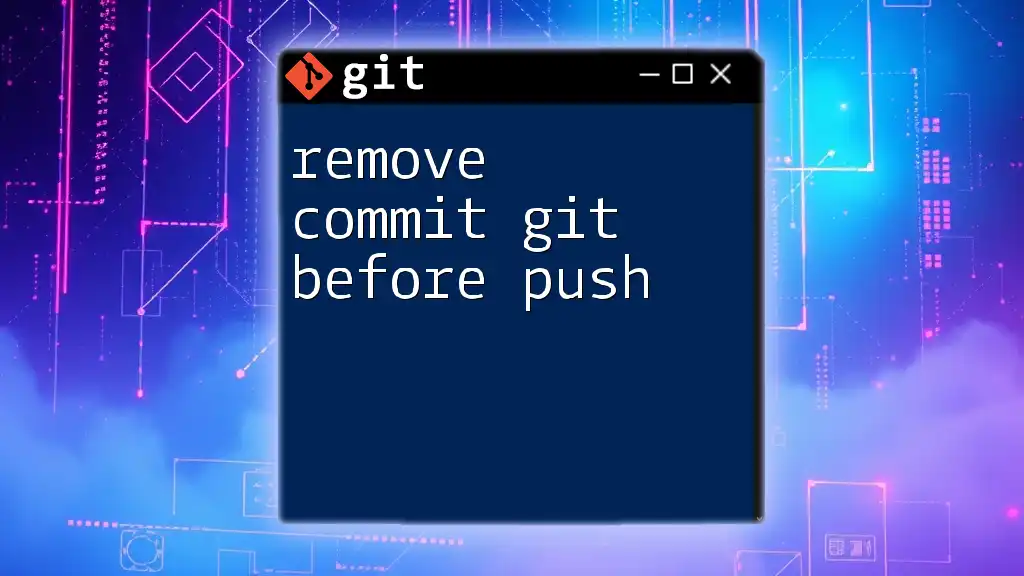
Important Considerations
Understanding of `ORIG_HEAD`
After successfully performing a rebase or cherry-pick, you may want to reference the original position of `HEAD`. The special reference `ORIG_HEAD` helps revert to previously checked-out commits if needed.
Dealing with Merge Conflicts
When reordering commits, especially during a rebase, you may encounter merge conflicts. It’s crucial to resolve these conflicts correctly before continuing with the rebase process. Address any conflicts by carefully reviewing and selecting the appropriate changes, then continue the rebase with:
git rebase --continue
Impact on Shared Repositories
Reordering commits can significantly impact shared repositories. Git history rewriting alters the timeline, which can confuse collaborators if not communicated properly. Always consider if a commit has been pushed to a shared repository and proceed with caution.
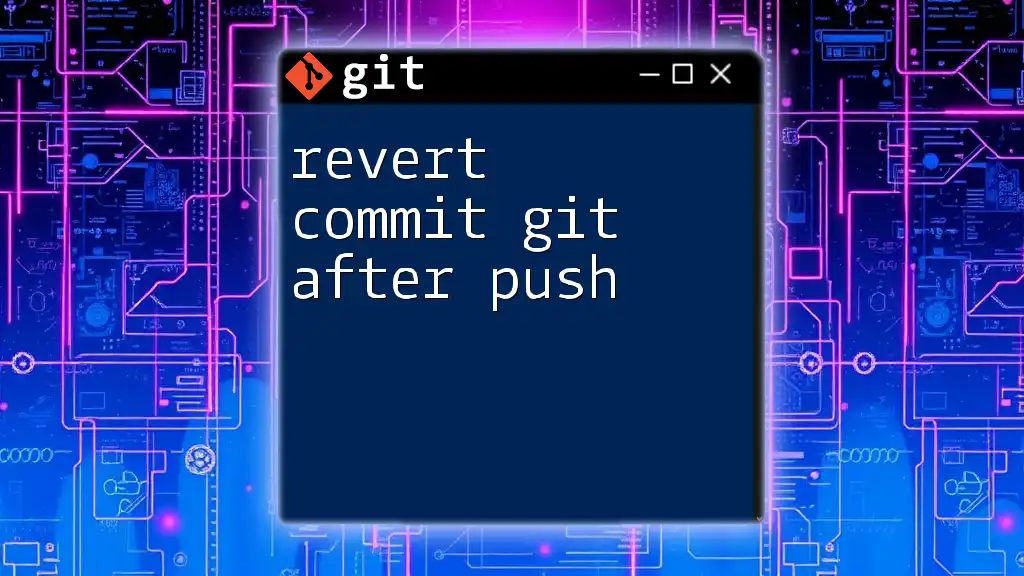
Reordering Commits in a Positive Workflow
Establishing a Commit Message Style
A consistent commit message style enhances the readability of your project history. Consider adopting a standard format, such as mentioning the related issue number or a brief summary of changes.
Tip: Use Descriptive Commit Messages
Good commit messages are essential for clarity. Craft messages that concisely reflect the nature of changes, the rationale, and any relevant context. For instance:
git commit -m "Add user authentication feature #42"
In this example, the message provides insight into what the commit entails and references a related issue.
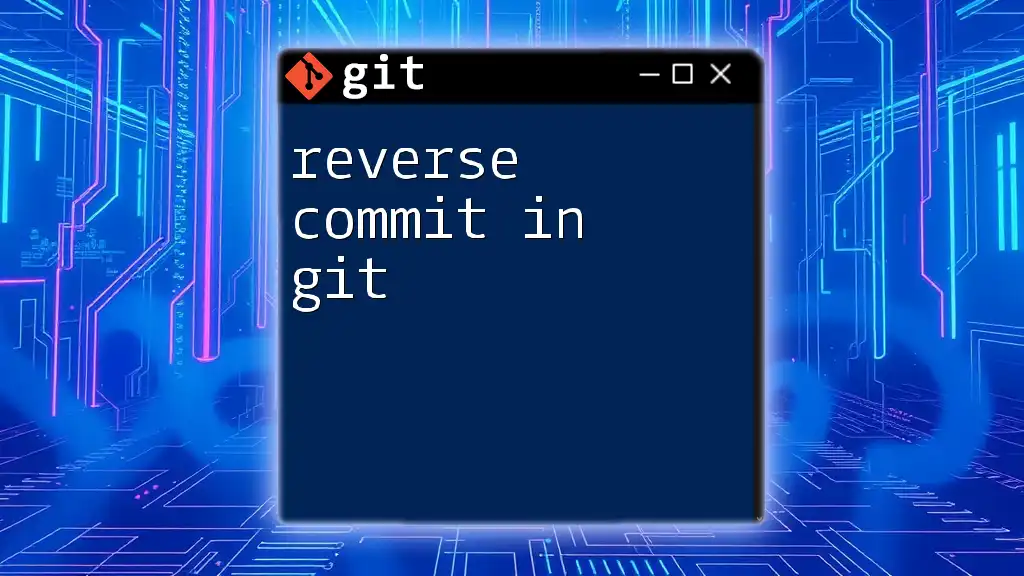
Conclusion
In summary, understanding how to reorder commits in Git is a valuable skill for any developer. By enhancing the readability and coherence of your project history, you can significantly improve collaboration and maintainability. As you practice these techniques, you'll appreciate the clarity and precision they bring to your version control process. Remember, a thoughtful commit history reflects a well-structured workflow, benefiting both individual developers and teams alike.
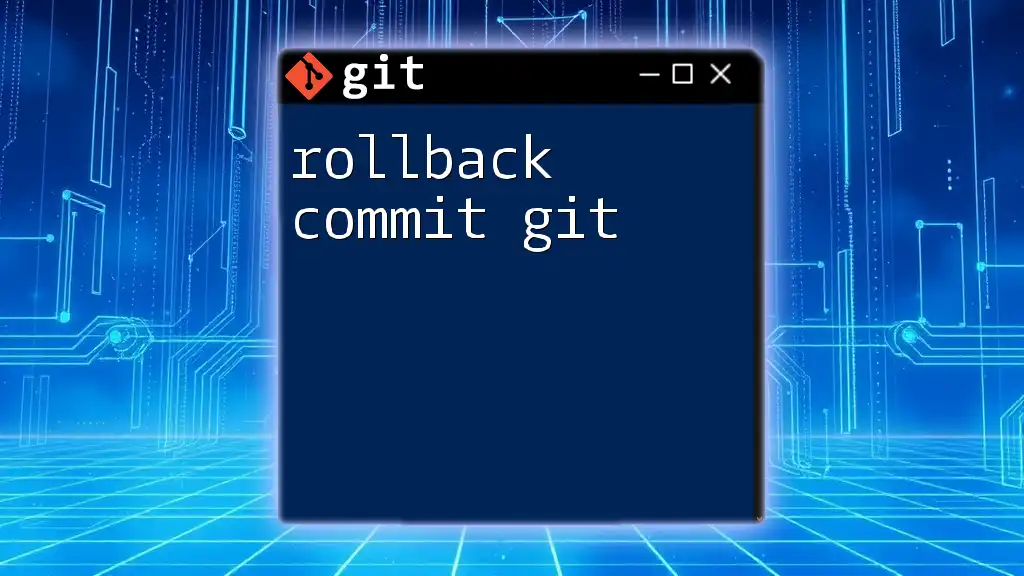
Additional Resources
- Links to Official Git Documentation for detailed commands and options.
- Recommended Git Tutorials for further learning on related topics.
- Community Forums for Git Support where you can ask questions and share experiences with other developers.