To reset a commit in Git, use the `git reset` command followed by the commit reference to move the HEAD pointer and potentially modify the staging area or working directory.
git reset --hard <commit-reference>
What is Git Reset?
Git reset is a powerful command used in Git to undo changes made in your commit history. It primarily allows users to move the HEAD pointer and optionally change the staging area and working directory to reflect that move. The ability to reset commits is crucial for maintaining a clean and precise commit history, helping developers manage the evolution of their codebase effectively.
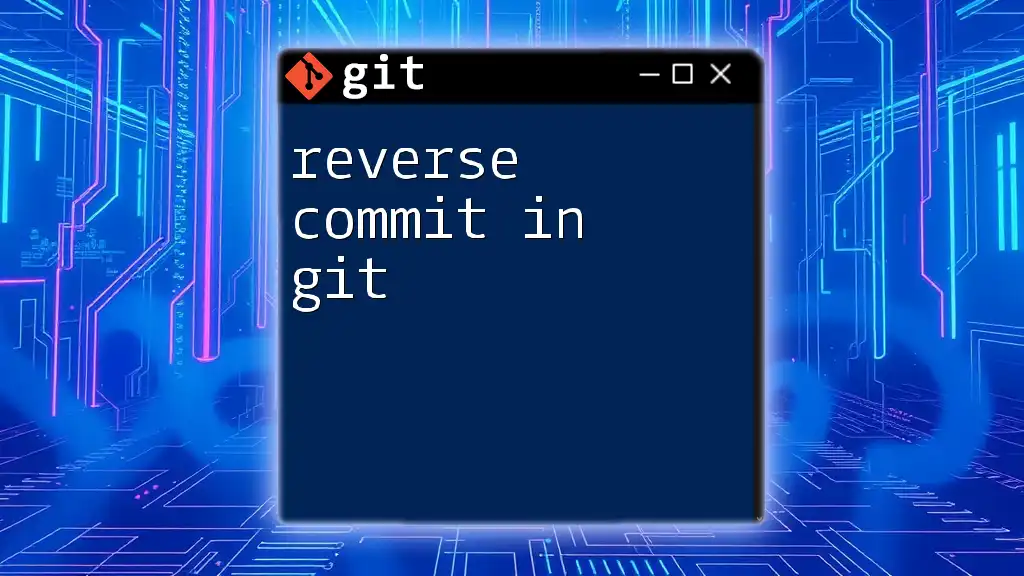
When to Use Git Reset
You might find yourself needing to reset a commit in Git under several circumstances, such as:
- Removing unwanted changes: After realizing a commit contains mistakes or unnecessary files.
- Adjusting commit history: When you want to combine multiple changes into a single commit or need to split large commits into smaller ones.
- Undoing mistakes: If you accidentally commit sensitive information or incorrect code.
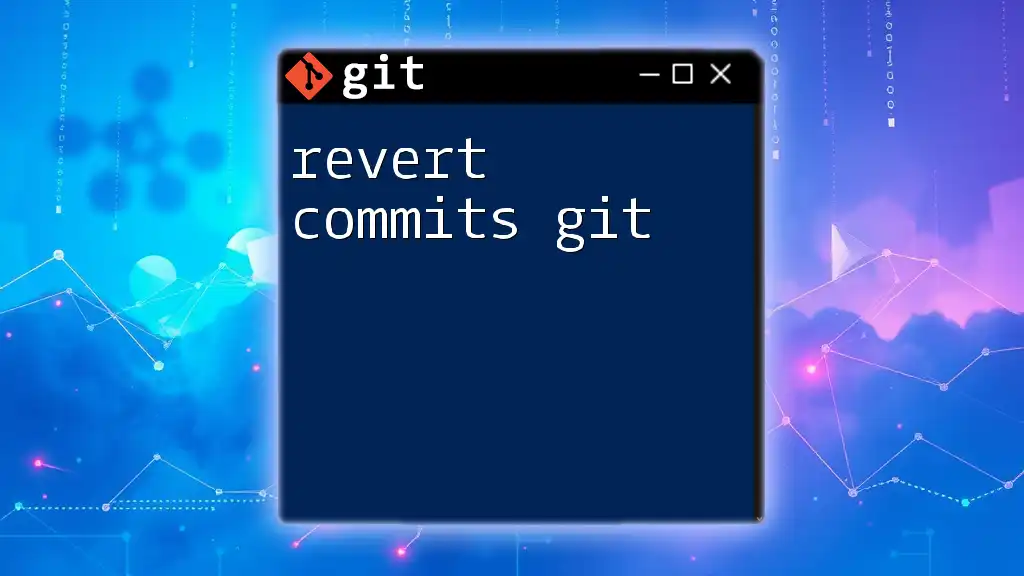
Types of Git Reset
Soft Reset
Definition and Purpose
A soft reset in Git allows you to move the HEAD pointer back to a specified commit while leaving your working directory and staging area untouched. This means the changes from the reset commit will remain staged and ready for further modifications or recommitting.
Command Syntax
git reset --soft <commit>
Example Usage
Imagine you've just made a commit and realized that you need to make some more changes. For instance:
git commit -m "Initial commit"
git reset --soft HEAD~1
In this example, the HEAD is moved back one commit. The changes from that initial commit will still reside in the staging area, allowing you to amend them before committing again. After executing the command, you can use `git status` to see that your previous changes are still staged for a new commit.
Mixed Reset
Definition and Purpose
A mixed reset is the default mode of git reset. It moves the HEAD pointer back to a specified commit and resets the staging area to match, but it leaves the working directory intact. As a result, the changes will still be present in your files, but they will now be uncommitted and unstaged.
Command Syntax
git reset --mixed <commit>
Example Usage
Suppose you have a commit you want to undo but keep your changes for further edits:
git commit -m "Add feature"
git reset --mixed HEAD~1
After executing this command, your changes will still be in your working directory, but not in the staging area. You can freely modify, stage, and commit these changes again as needed.
Hard Reset
Definition and Purpose
A hard reset is the most severe option in Git reset functionality. When you reset to a commit using the `--hard` option, it not only moves the HEAD pointer but also changes your working directory and staging area to match the state of the specified commit. This makes it irreversible; any uncommitted changes will be lost.
Command Syntax
git reset --hard <commit>
Example Usage
If you find yourself in a situation where you’ve committed changes that you completely want to discard, you can use:
git commit -m "Fix bugs"
git reset --hard HEAD~1
This command will delete the changes from the last commit and reset your working directory to match the state of your repository before that commit. Be extremely cautious with this command, as you could easily lose work you may have intended to keep.
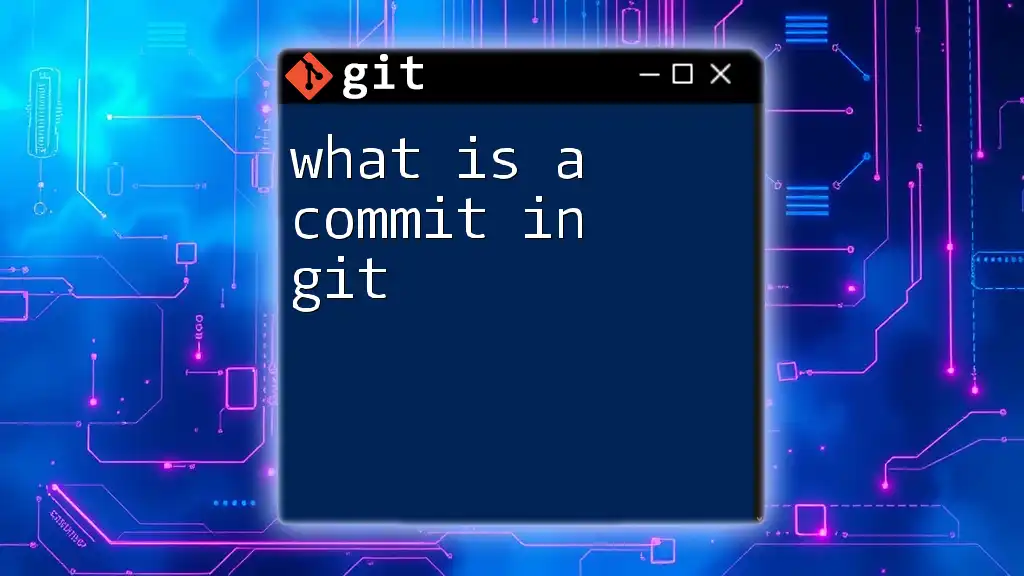
Understanding Git Commit References
How to Identify Commit References
Each commit in a Git repository is identified by a unique SHA-1 hash. You can view your commit history to find these hashes by using:
git log
This command presents a list of commits, their authors, dates, messages, and hashes. Additionally, `HEAD` represents the current working area in the repository, and you can refer to it using related terms like `HEAD1` (the previous commit) or `HEAD2` (the commit before that).
Commonly Used References
Using references such as `HEAD`, `HEAD^`, and `HEAD~1` can simplify your Git commands, making it easier to navigate through the commit history. The `git reflog` command is also useful for tracking where your HEAD has been, allowing you to find past commits more easily.
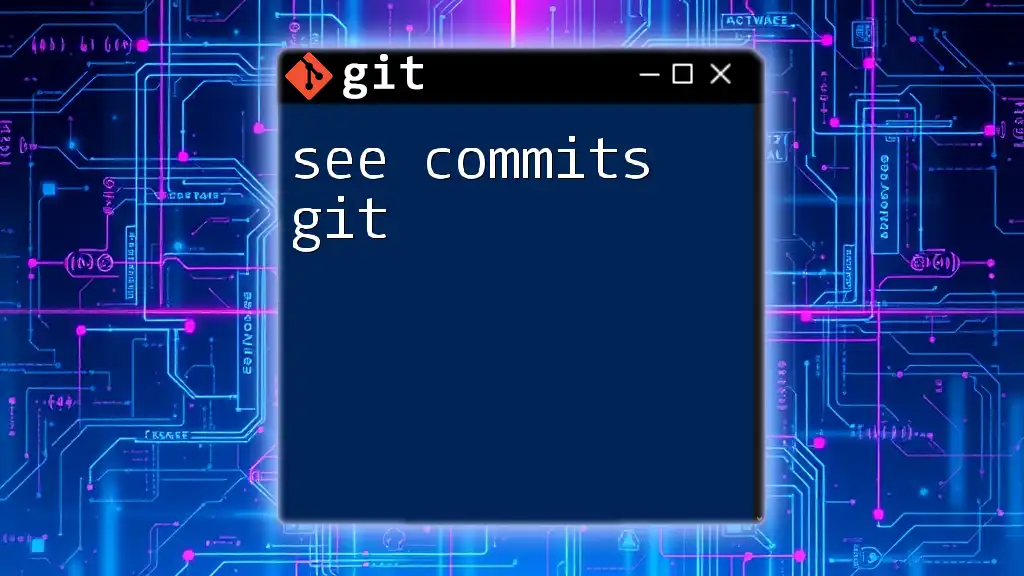
Best Practices and Precautions
When Not to Use Git Reset
While git reset can be handy, it isn’t always the best tool. When collaborating in a team environment, avoid using git reset on commits that have already been pushed. Doing so can lead to complications for other developers who are working from the same codebase, resulting in confusion and orphaned commits.
Alternatives to Git Reset
If you need to undo changes without affecting the commit history in a shared environment, consider using `git revert`. This command creates a new commit that undoes the changes made by a previous commit. For example:
git revert <commit>
This method preserves history and is a safer option when working collaboratively.
Backing Up Before Resetting
Before performing a reset, especially a hard reset, it’s advisable to create a backup branch to protect your work. You can easily do this with:
git branch backup-branch
Creating a backup ensures that you have a point to return to if you decide you need what was reset.
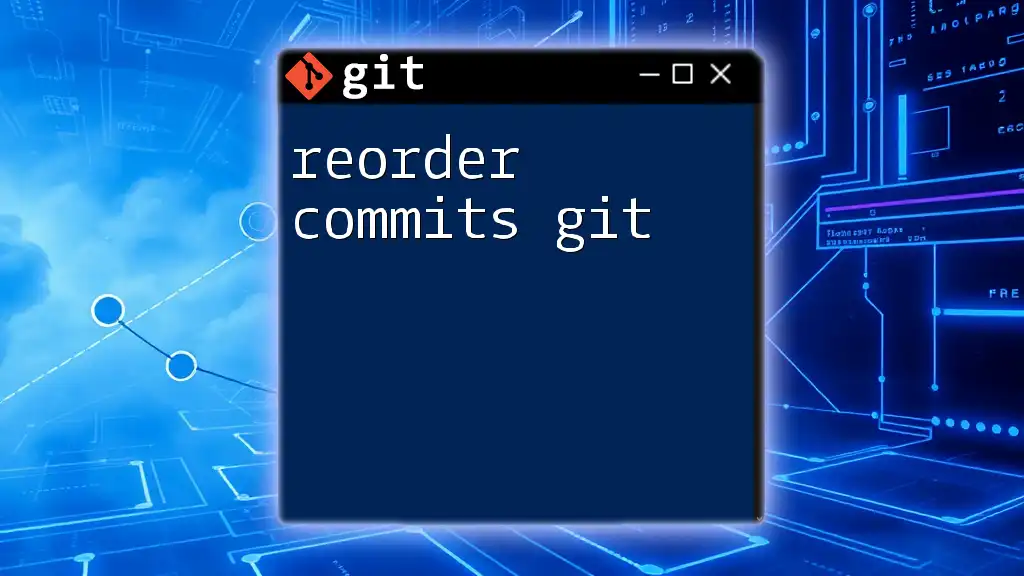
How Resetting Affects Branches and Collaboration
Impact on Shared Repositories
Using git reset in a shared repository can affect your teammates. When the history is rewritten (especially with hard resets), it can lead to discrepancies between the local copies of the repository. As a best practice, always communicate with your team before using reset commands that affect shared branches.
Maintaining Commit History
A clear and informative commit history is essential for collaboration. Whenever possible, consider alternatives to resetting and aim to maintain the integrity of the commit history. Using meaningful commit messages and regular communication on your changes will help ensure everyone stays on the same page.
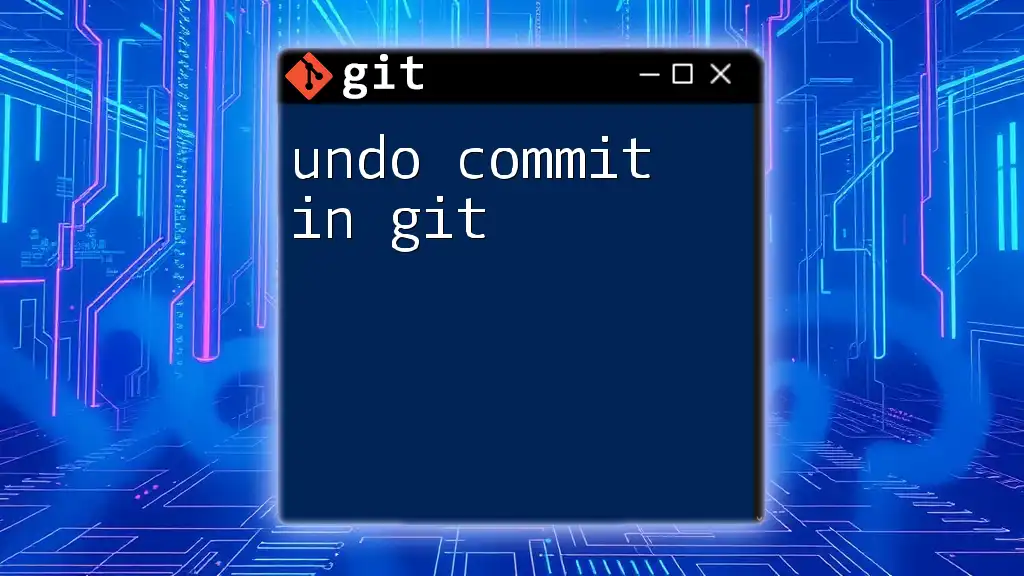
Conclusion
Understanding how to reset a commit in Git is a vital skill for any developer. By recognizing the differences between soft, mixed, and hard resets, you can wield this power responsibly, maintaining a clean and harmonious repository while ensuring your work is well-managed.
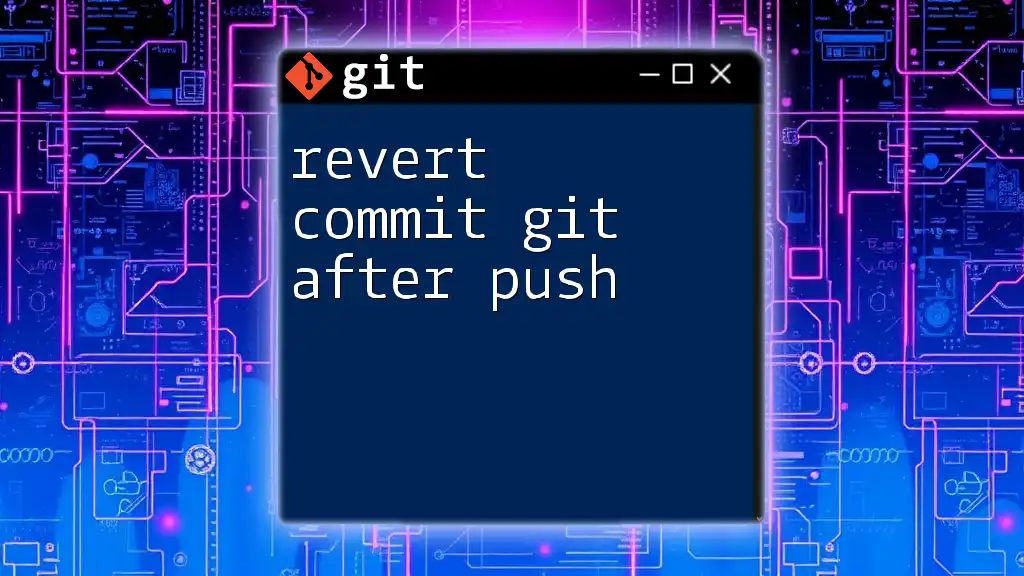
Additional Resources
For deeper dives into Git commands and techniques, refer to the official Git documentation, which provides extensive details about every aspect of Git command usage. Embrace the learning journey, and don't hesitate to practice these techniques in a safe environment. If you have questions or seek clarifications, feel free to reach out for assistance.