A commit in Git is a snapshot of changes made to files in a repository, allowing you to track the history of your project and collaborate with others.
Here’s how you can create a commit in Git:
git commit -m "Your commit message here"
Understanding Commits
What is a Commit?
At its core, a commit in Git is a record of changes made to the files in a Git repository. It represents a snapshot of the project at a specific point in time. Every commit contains details about the changes, including which files were modified, added, or deleted, as well as metadata such as the author's name, email, and a timestamp.
The Role of Commits in Version Control
Commits are fundamental to the way Git operates as a version control system. They enable developers to track variations in their project over time by storing each change as a distinct snapshot. This historical record facilitates:
- Collaboration: Multiple contributors can work on the same project, and each commit includes contributions that can be attributed to specific authors.
- Revisions: Developers can revert to previous commits if a change causes issues, allowing easy management of project states.
Commit Objects in Git
In Git, a commit is more than just a simple record; it is a structured object consisting of:
- Commit hash: A unique identifier for the commit, generated by hashing the commit's contents.
- Author: The name and email of the person who made the commit.
- Date: The timestamp of when the commit was made.
- Commit message: A short description explaining the purpose of the commit.
- Parent commit(s): A pointer to the commit(s) that preceded it, creating a linked history of commits.
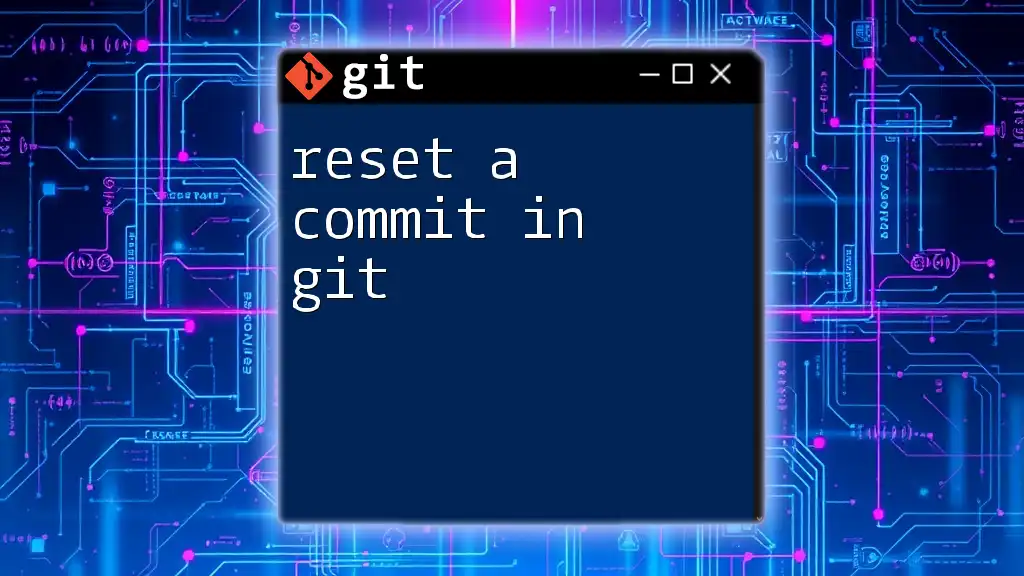
Creating a Commit
The Git Workflow
To create a commit, it is essential to grasp the broader Git workflow, which involves steps that lead to a commit. Before committing changes, files must be modified and staged in what is known as the index.
Step-by-Step Guide to Creating a Commit
Step 1: Modify Files
Begin by making changes to the files in your project. For example, you might create a new file with the following command:
echo "Hello, World!" > hello.txt
Step 2: Stage Changes
Once you've made changes, you need to stage those changes. This process informs Git which modifications you want to include in your next commit. The command below stages the new `hello.txt` file:
git add hello.txt
Step 3: Commit Changes
After staging the changes, you can create the commit. Use the command below to commit changes along with a descriptive message:
git commit -m "Add hello.txt file"
Here, the `-m` flag tells Git to take the following string as the commit message, which serves as a brief identifier for the commit.
Best Practices for Writing Commit Messages
Writing effective commit messages is an essential skill. Good commit messages convey the purpose of your changes clearly, and they follow certain conventions. Here are some key elements to consider:
- Use the imperative mood (e.g., "Fix bug" instead of "Fixed bug").
- Keep the messages concise yet descriptive, ensuring that they provide context.
- If needing to explain substantial changes, consider adding a more detailed description in the body of the commit message.
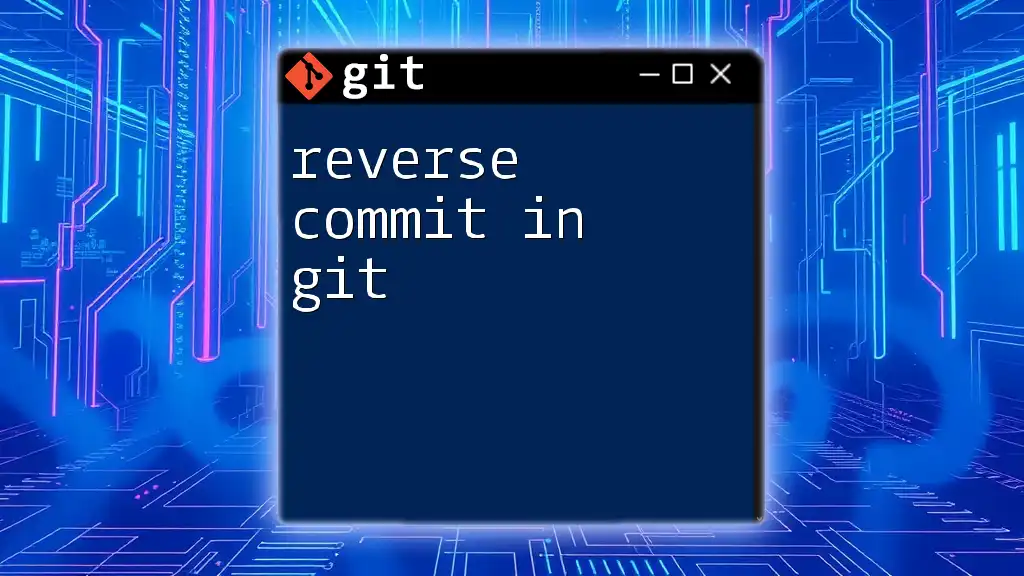
Viewing Commit History
The Git Log
To view the history of commits, use the `git log` command. This command lists all commits in the current branch, providing an overview of the project's development. You can invoke it with:
git log
Understanding Commit Output
The output from `git log` includes critical information for each commit:
- Commit hash: A unique identifier for the commit.
- Author: Displays the name and email of the person who made the commit.
- Date: Shows when the commit was made.
- Commit message: Provides context on what changes were made.
When analyzing a commit log, it is possible to see the evolution of the project, identify problem areas, and understand how features were developed.
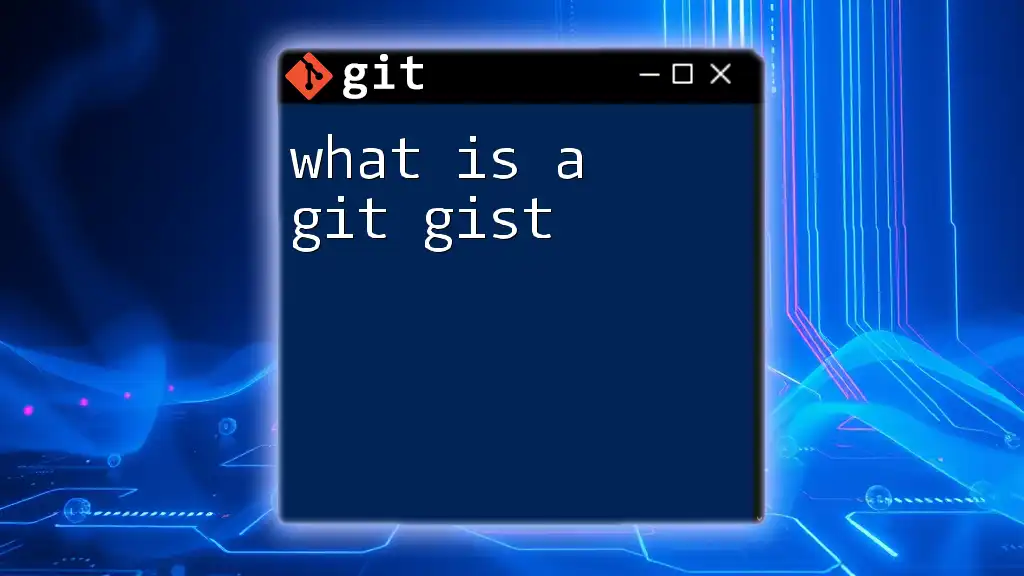
Modifying and Removing Commits
Amend Last Commit
In situations where you need to adjust the last commit—perhaps to correct a commit message or include additional changes—Git allows you to amend that commit. This can be done with:
git commit --amend -m "Updated commit message"
Amending is particularly useful for small changes; however, be cautious when amending commits that have already been pushed to a shared repository, as this can disrupt the commit history for others.
Undoing Commits
If you need to revert changes made by a previous commit, Git offers flexible methods:
-
`git reset`: This command allows you to unstage or reset your commit history.
- Use `git reset --soft <commit>` to remove the commit while keeping changes in the staging area.
- Use `git reset --hard <commit>` to delete the commit and the associated changes entirely.
-
`git revert`: Unlike `reset`, `git revert` creates a new commit that undoes the changes made by a previous commit. This method is safe for shared repositories:
git revert <commit>
This approach is ideal for maintaining a clean project history while still addressing issues.
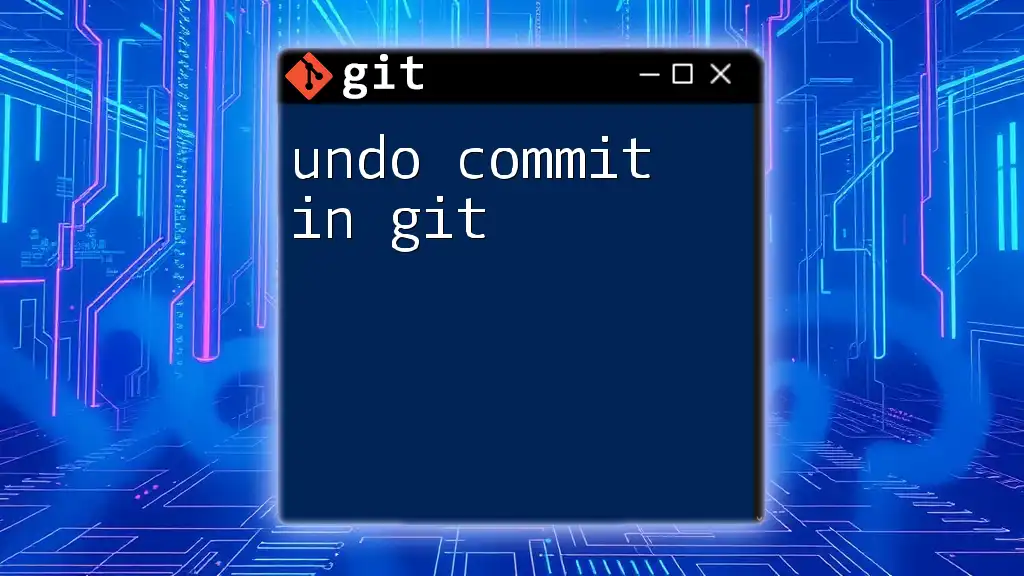
Commits in Team Collaboration
Sharing Commits
Once you have made your commits locally, you may want to share your changes with collaborators. This is done using the `git push` command, which uploads your local commits to a remote repository:
git push origin main
This command uploads all your local commits to the `main` branch in the remote repository called `origin`.
Pull Requests and Reviews
In collaborative projects, commits often pave the way for pull requests. When you create a pull request, you can request that your changes be reviewed and merged into the main codebase. This process allows your team to review your commit history, ensuring that all changes have been vetted and discussed.
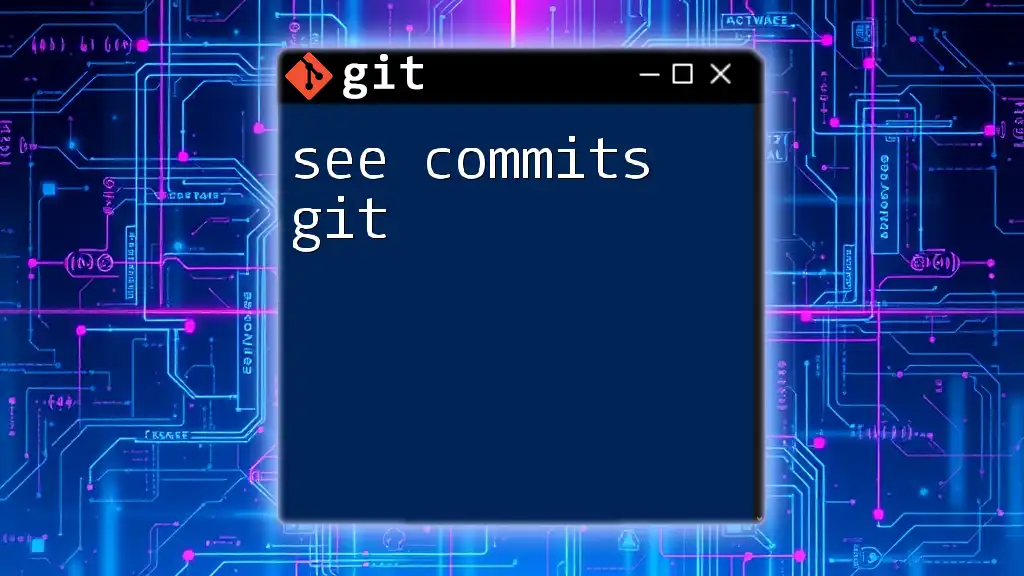
Conclusion
Committing in Git is a fundamental process that underlines the power of version control. By understanding what a commit in Git truly represents, its structure, functions, and best practices for creation, you can harness the full potential of Git to manage your projects effectively. Embrace the practice of making well-documented commits, and you will find yourself well-equipped to tackle collaborations and project history management. For continuous improvement, consider exploring additional resources to advance your Git skills further.