To revert the effects of a specific pushed commit in Git, you can use the `git revert` command followed by the commit hash.
Here's a code snippet to illustrate:
git revert <commit-hash>
Understanding Git Basics
What is Git?
Git is a distributed version control system that enables multiple developers to work on a project simultaneously without overriding each other’s changes. Its primary purpose is to keep track of alterations in source code throughout the development lifecycle. With Git, you can revisit any alterations made at any point in time, fostering collaboration and enhancing productivity within teams.
Importance of Reverting Changes
In any collaborative environment, mistakes are inevitable. Whether you mistakenly push an unstable feature, merge in conflicting changes, or accidentally include sensitive files, being able to revert changes is crucial. The reliability of your codebase depends on how effectively you can manage and rectify such errors.
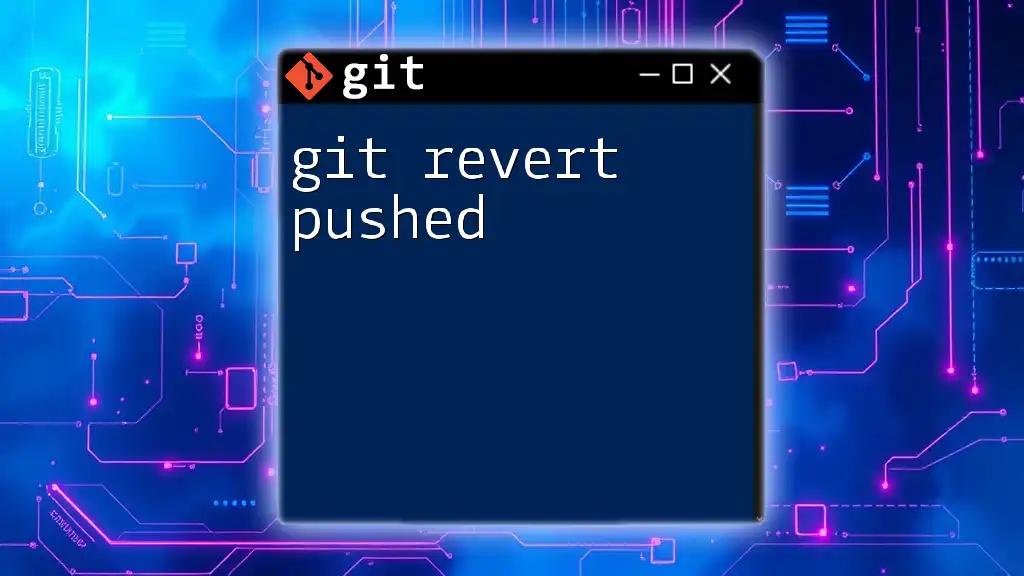
Types of Pushes in Git
Local Push vs Remote Push
Understanding the distinction between local and remote pushes is essential when discussing how to revert a push in Git:
- Local Push: Changes pushed from your local repository to the remote server. This is where you commit changes to your local repository before sending them to the remote.
- Remote Push: This is the final action that updates the remote repository with the changes. Once the push is successful, your changes become part of the project accessible to all collaborators.
Identifying the Need to Revert
Before executing a revert, it's essential to assess whether it is necessary. Common scenarios where you might need to revert include:
- Introducing a Bug: If you accidentally pushed code that causes a malfunction.
- Unintended Files: When sensitive information was included in a commit.
Evaluate the impact of the change before proceeding to revert.
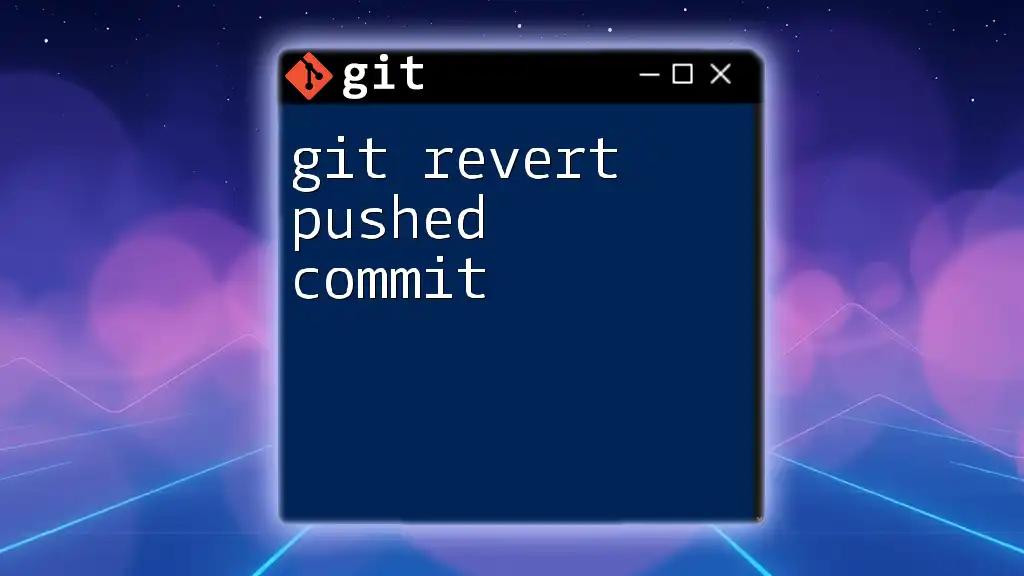
How to Revert a Git Push
Step-by-Step Guide
Identifying the Commit to Revert
Begin by determining which commit needs to be reverted. You can use the `git log` command, which displays a chronological list of commits:
git log
This command will show you the commit history along with their unique commit hashes. Identify the hash of the commit that brought about the need for reversal.
Reverting a Commit
To revert a specific commit, employ the `git revert` command. This command is particularly powerful because it creates a new commit that undoes the changes made in the specified commit. The syntax is as follows:
git revert <commit_hash>
Replace `<commit_hash>` with the actual hash from the previous step. For instance, if you identified a hash like `abc1234`, you’d execute:
git revert abc1234
Once executed, Git will create a new commit that reverses the changes. Be sure to provide an appropriate commit message during this process to explain why the revert was necessary.
Pushing the Revert
After creating the revert commit, you’ll need to push this new state to the remote repository:
git push origin <branch>
This action will apply the revert to the branch you’re currently working on, effectively rolling back the unintended changes for all collaborators.
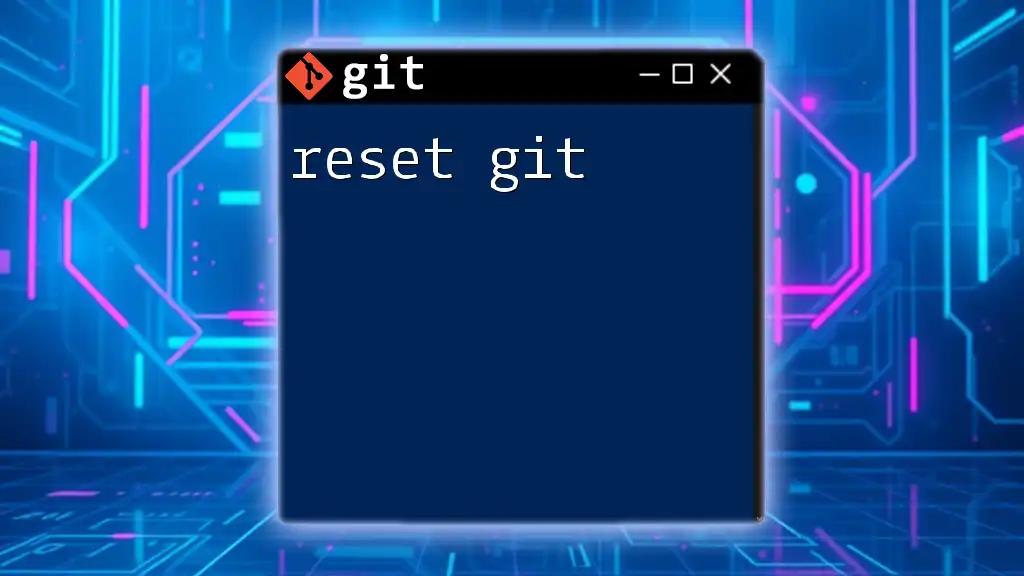
Alternative Methods to Revert a Push
Resetting a Commit
Understanding `git reset`
The `git reset` command is another method to consider, although it’s crucial to understand its implications. This command manipulates the commit history and can lead to data loss if used carelessly.
- Hard Reset: Reverts the working directory and the index (staging area) to the specified state. This will permanently remove the commits following the specified commit.
- Soft Reset: Keeps your changes staged for commit, reverting only the commit history.
Example of a Hard Reset
If you decide to go with a hard reset, you can do so with the following command:
git reset --hard <commit_hash>
For instance, if your commit hash is `xyz9876`, use:
git reset --hard xyz9876
Because this action alters the commit history, it's essential to proceed with caution. After executing a hard reset, you'll need to force the push to the remote repository:
git push origin <branch> --force
Using `git reflog`
What is `git reflog`?
`git reflog` is an invaluable tool that allows you to keep track of where your HEAD has been, enabling a recovery option even if you can't find a commit in the regular log. This command can help in situations where commits are seemingly lost due to resets or other Git operations.
Retrieving a Previous State
You can use `git reflog` to view the history of all your commands and find lost commits easily:
git reflog
The output will provide a list of actions taken in your repository, including the commit hashes that you can revert to or reset from.
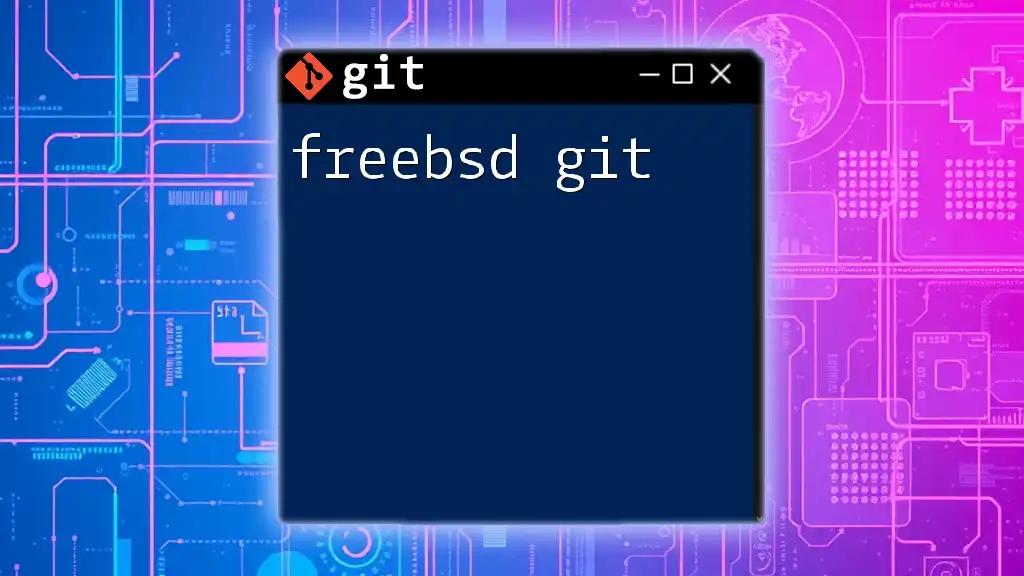
Best Practices for Avoiding Mistakes
Regular Commit Practices
To minimize the need for reverts, adopt a workflow that emphasizes frequent commits. Smaller, consistent commits allow for easier management of changes and help you quickly isolate problematic commits when issues do arise.
Branching Strategies
Another best practice involves utilizing feature branches. Instead of committing directly to the main branch, create dedicated branches for new features or bug fixes. This isolated environment reduces the risk of introducing errors into the primary codebase.
Testing Before Pushing
Encourage code reviews and testing before committing changes to the remote repository. This collaborative approach helps identify mistakes early on and ensures that the code being pushed is of high quality.
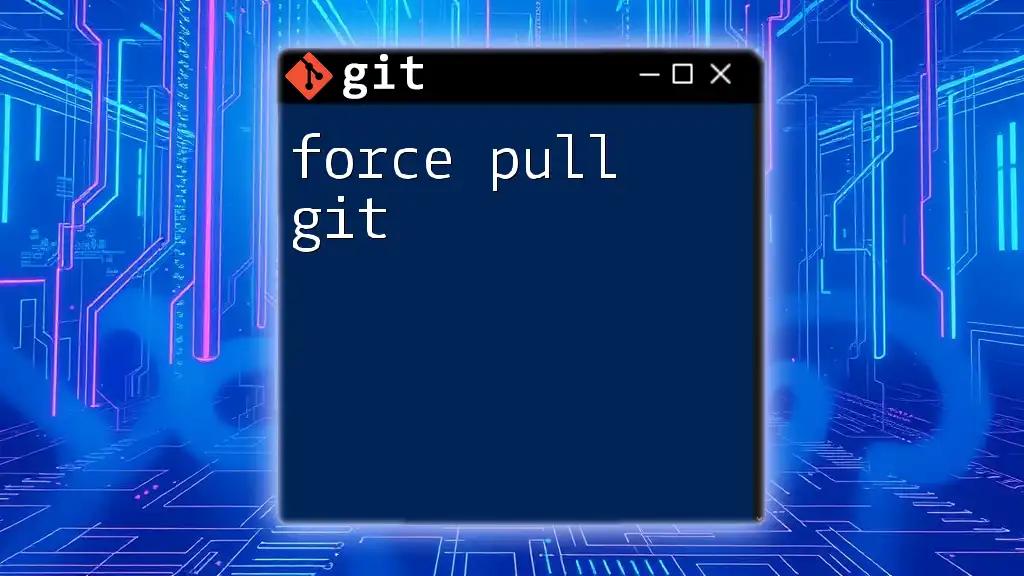
Conclusion
Recap of Key Points
Throughout this guide, you've learned the difference between reverting and resetting a commit, and when each method is appropriate. Understanding both processes allows for greater flexibility and control in managing your Git repository.
Call to Action
Ready to master Git commands and workflows? Join our Git Mastery Course to deepen your skills and ensure effective version control in your projects.
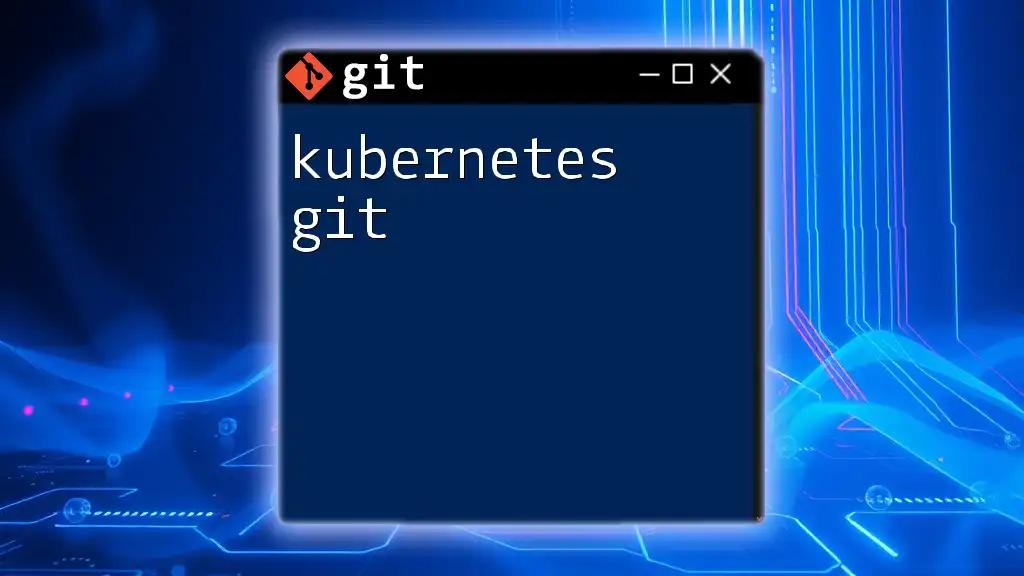
Frequently Asked Questions
What happens to my remote repository when I revert?
When you perform a revert, a new commit is added to the remote repository that undoes the specified changes. This preserves the commit history while effectively rolling back the impact of the previous commit.
Can I revert more than one commit at a time?
Yes, you can use the `git revert` command with a commit range. For example:
git revert <oldest_commit>^..<latest_commit>
This command will create revert commits for each commit in the specified range.
Is there a way to permanently delete a commit?
While you can use `git reset` for this purpose, keep in mind that it can lead to data loss. Make sure that you only use this option if you are certain that you won’t need the commit in the future.
How does reverting affect collaboration?
Communicate with your team whenever you revert a commit, as it can affect other team members who are basing their work on the commit you modified. Maintaining clear communication helps ensure that everyone is aligned on the project's status.