To resolve conflicts in Git, you can manually edit the conflicting files to reconcile changes, and then stage the resolved files before committing them, as shown in the following example:
git add <file_with_conflict>
git commit -m "Resolved merge conflict in <file_with_conflict>"
Understanding Git Conflicts
What is a Git Conflict?
A Git conflict occurs when two branches have made changes to the same line or lines in a file, and Git does not know which version to keep during a merge or a rebase. It can also happen when one person deletes a file that another person has modified. Conflicts often lead to the daunting task of determining which code to retain and which to discard, making understanding and resolution crucial skills for any developer.
The Importance of Conflict Resolution
Resolving conflicts swiftly is vital for maintaining code integrity and ensuring seamless collaboration among team members. Conflicts left unresolved can lead to broken builds, lost work, and a generally chaotic development environment. Therefore, mastering how to resolve conflicts in Git not only enhances productivity but also fosters teamwork.
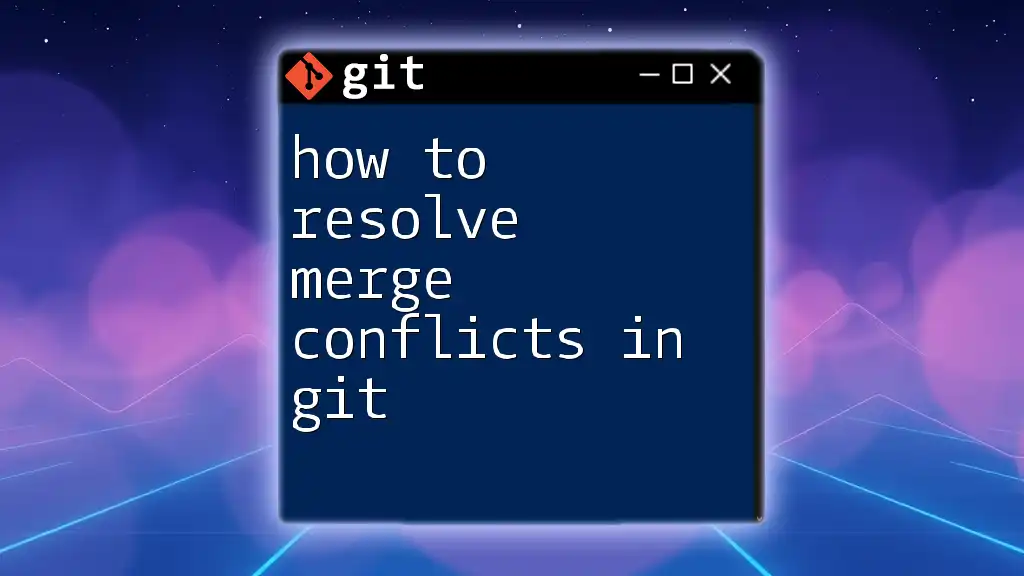
Identifying Conflicts
How to Recognize a Conflict
When a conflict arises during a merge or a rebase, Git provides clear messaging to highlight the conflicting files. For example, when running a merge command, you may see output messages indicating that certain files need your attention, like so:
Automatic merge failed; fix conflicts and then commit the result.
Common Signs of Conflicts in Git Log
Another way to identify conflicts is by checking your repository status. You can use:
git status
If there are conflicts, you will see a list of files marked as "both modified." This tells you exactly where Git is facing challenges.
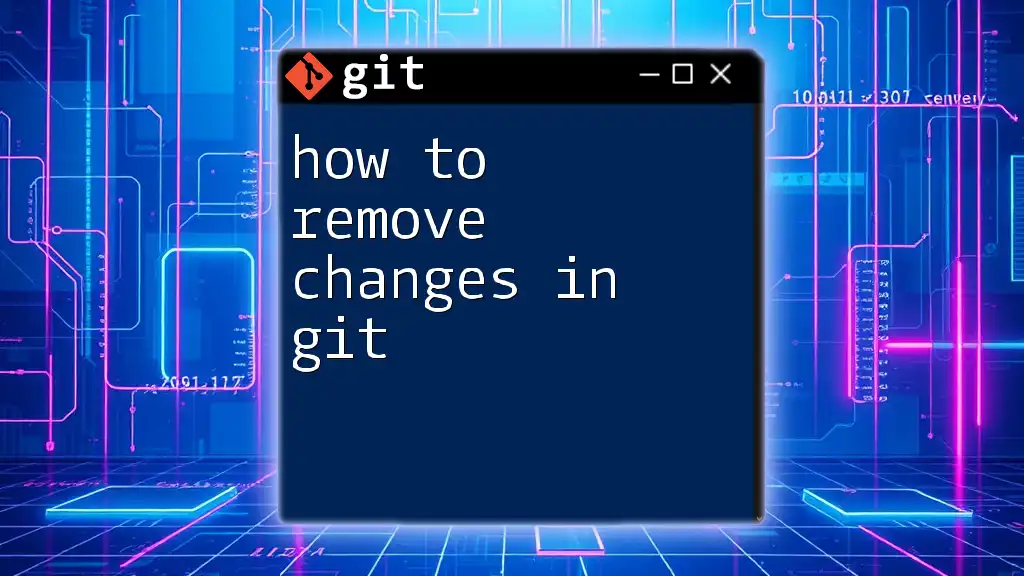
Resolving Conflicts
Preparing for Conflict Resolution
Before diving into conflict resolution, it's essential to ensure your local repository is up to date. Running the following command will help you synchronize your changes with the remote repository:
git fetch
The Workflow for Resolving Conflicts
Step 1: Triggering the Conflict
To understand how conflicts occur, consider an example where we have two branches, branchA and branchB, both changing the same line in a file. You can create a conflict by attempting to merge them:
git checkout branchA
git merge branchB
You will likely encounter a conflict if changes in branchB conflict with those in branchA.
Step 2: Checking the Conflict
Once a conflict has occurred, Git will modify the affected files to include conflicts. You'll see conflict markers like this in the code:
<<<<<<< HEAD
int a = 5;
=======
int a = 10;
>>>>>>> branchB
These markers indicate which lines are from your current branch (`HEAD`) and which lines are from the merging branch (`branchB`).
Step 3: Manual Conflict Resolution
The next step is to manually resolve the conflict. Open the file in a code editor and decide which code change to keep or how to combine changes. After editing, your code should look like this:
int a = 5; // Choose the desired value
By removing the conflict markers, you've resolved the conflicting code.
Step 4: Marking Conflicts as Resolved
After resolving the conflicts in one or more files, you need to stage these changes. Use the following command:
git add <filename>
This command tells Git that you have resolved the conflict in the specified file.
Step 5: Finalizing the Merge or Rebase
Once all conflicts have been resolved and staged, you can finalize the merge or rebase process by committing your changes:
git commit -m "Resolved merge conflict"
This commitment marks the end of the conflict resolution process, and your branch now contains the integrated changes.
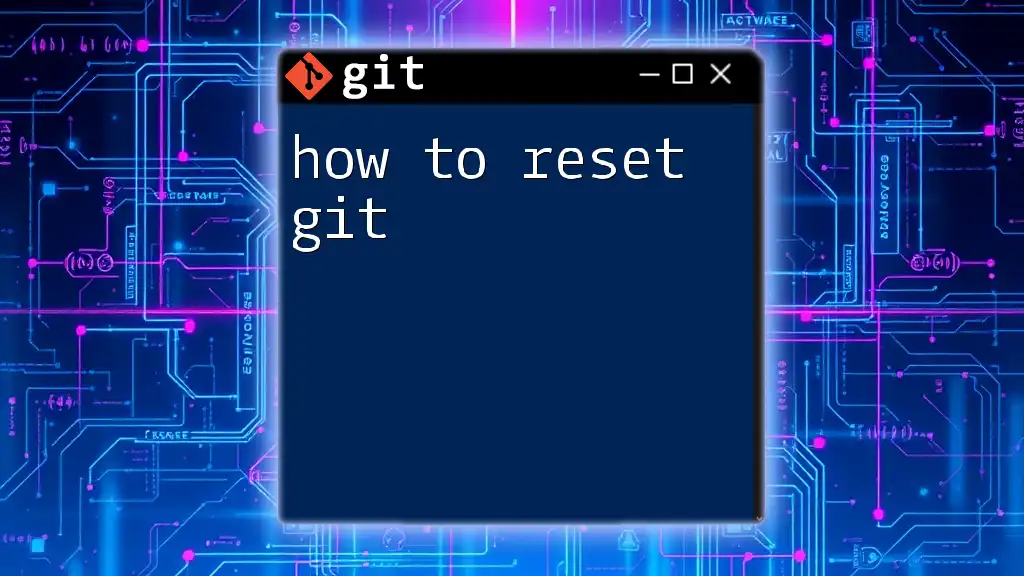
Advanced Conflict Resolution Techniques
Using Merge Tools
For those who prefer a graphical or more visual representation during conflict resolution, consider utilizing merge tools like KDiff3 or Meld. To configure a merge tool, you can set it in your git configuration:
git config --global merge.tool kdiff3
Once configured, running the merge command will open the merge tool, allowing you to resolve conflicts intuitively.
Cherry-Picking and Reverting
Cherry-picking is a technique to apply specific commits from one branch to another. This can sometimes lead to conflicts, particularly if the commits involve changes to the same lines of code. The command looks like this:
git cherry-pick <commit_hash>
If a conflict arises during a cherry-pick, follow the same resolution steps: edit the file, stage it, and commit the changes.
Conflict Resolution Strategies
When resolving conflicts, it’s essential to employ the right strategy. The "theirs" and "ours" strategies dictate which branch's changes will prevail in case of a conflict. Use `--strategy-option theirs` in a merge to favor changes from the branch you're merging in, while `--strategy-option ours` favors the current branch’s changes.
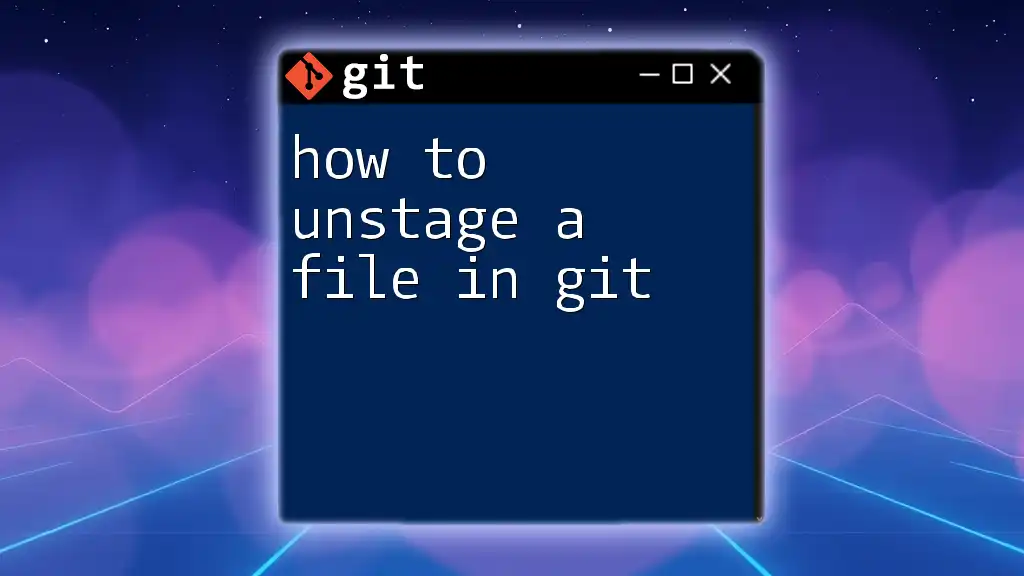
Best Practices for Avoiding Conflicts
Regular Pulling and Merging
To minimize conflicts, it's advisable to frequently pull and merge changes from the remote repository. This practice ensures your local branch remains current, reducing the chances of running into conflicts down the line.
Clear Communication with Team Members
Maintaining clear communication with your team about which aspects of the codebase you're working on can significantly diminish conflicts. Using project management tools or setting weekly stand-ups helps keep everyone on the same page.
Smaller, Frequent Commits
Encourage making smaller and more frequent commits instead of one large commit at the end of a feature. This approach not only mitigates the likelihood of conflicts but also makes it easier to identify and fix issues.
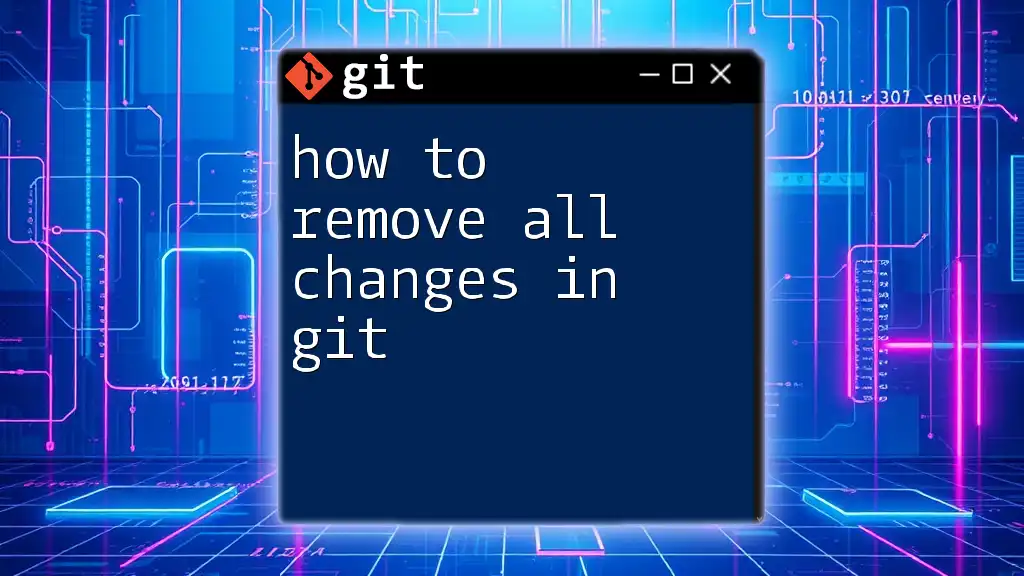
Conclusion
Mastering how to resolve conflicts in Git is a fundamental skill that enhances teamwork and code quality. With effective strategies and practices at your disposal, you can navigate potential conflicts with confidence. Regularly practicing these techniques will prepare you for any collaboration challenges and help you maintain a smoother development workflow.
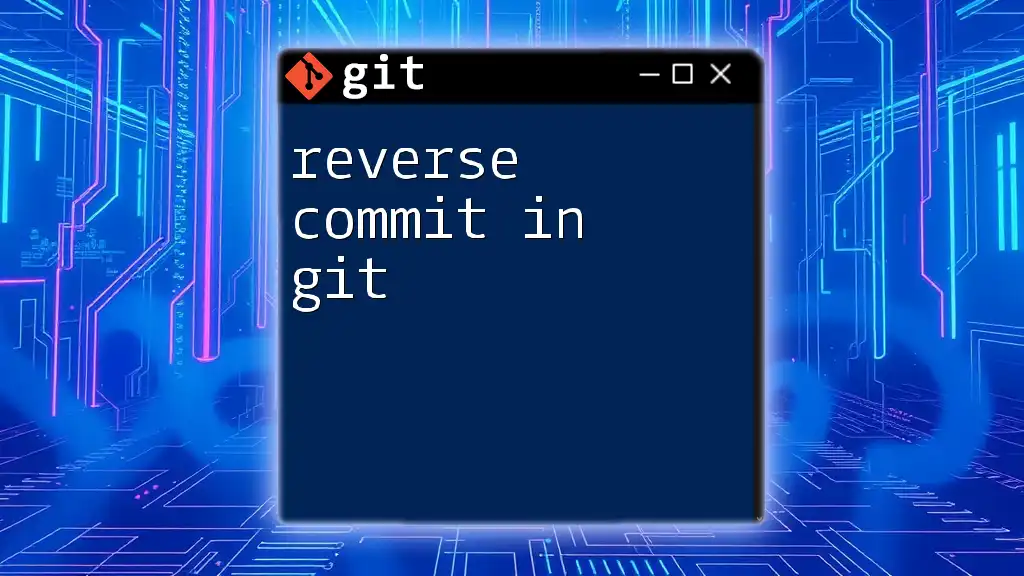
Additional Resources
For those looking to expand their understanding further, explore the official [Git documentation](https://git-scm.com/doc) and check out recommended tools for conflict resolution. Taking proactive steps to enhance your Git skills will only lead to long-term benefits!