To resolve merge conflicts in Git, you need to manually edit the conflicting files, mark the conflicts as resolved, and then commit the changes using the following command:
git add <filename> && git commit -m "Resolved merge conflict in <filename>"
Understanding Merge Conflicts
What is a Merge Conflict?
A merge conflict occurs when two branches in Git attempt to modify the same line in a file or when one branch deletes a file that another branch modifies. In such cases, Git cannot automatically combine the changes, leading to a conflict that requires manual resolution. This situation often arises during collaborative projects where multiple developers work on the same codebase.
Why Do Merge Conflicts Occur?
Merge conflicts primarily happen during the merging process. When branches diverge significantly and changes are made to the same parts of a file, Git encounters discrepancies it cannot resolve on its own. To simplify, think of it as two teams trying to change the same document simultaneously without communicating their edits; the lack of coordination leads to confusion.
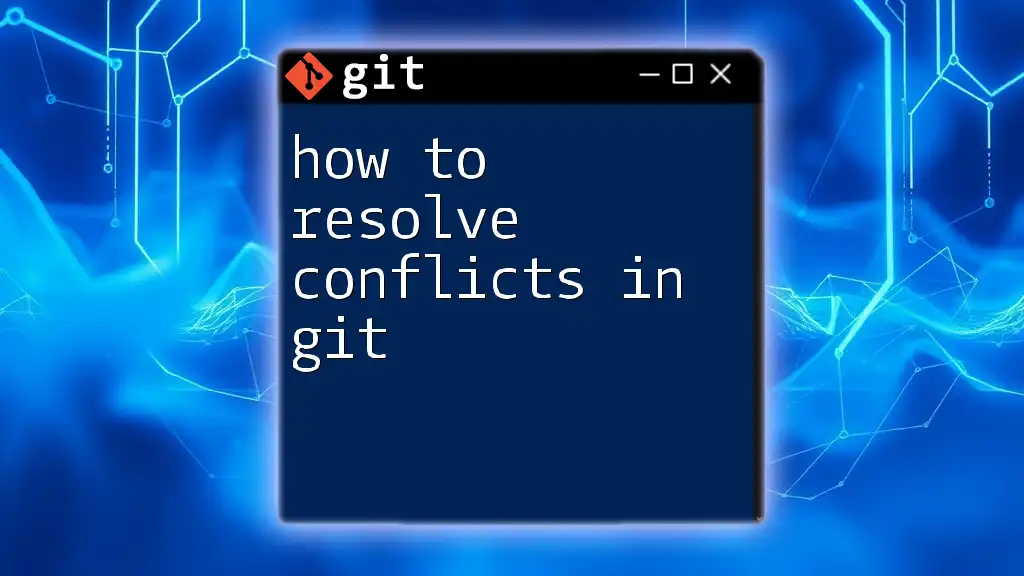
Preparing for a Merge
Best Practices to Avoid Merge Conflicts
To minimize the chances of encountering merge conflicts, consider implementing several best practices:
- Clear Communication: Ensure team members are aware of their changes and coordinate to avoid overlapping work.
- Frequent Pulling and Pushing: Regularly pull changes from the remote repository and push your local changes to reduce the likelihood of significant divergences.
- Organizing Code Reviews: Conduct code reviews before merging branches to catch potential conflicts early.
How to Check Your Current Branch Status
Before merging, it's essential to check your current branch status by using the `git status` command. This provides an overview of your branch and its relationship to the remote repository. Look for any uncommitted files or indications that an upstream branch has changes that need to be pulled.
git status
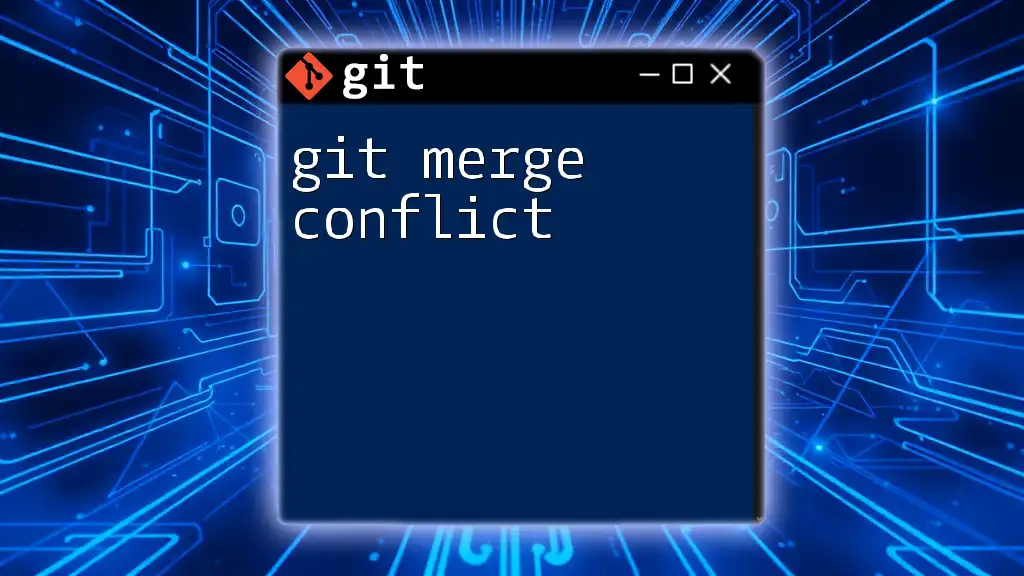
Detecting Merge Conflicts
Steps to Initiate a Merge
To merge a feature branch into the main branch, you would typically use the following command:
git merge feature-branch
During this process, Git will attempt to automatically combine changes. If it finds overlaps, you will receive a merge conflict message, indicating which files contain conflicts that need resolution.
Identifying Merge Conflicts in Git
When a merge conflict occurs, Git provides visible indicators in the terminal output. In addition to this, the affected files will be marked in the `.git` directory with conflict markers. It is crucial to identify these files quickly to address conflicts efficiently.
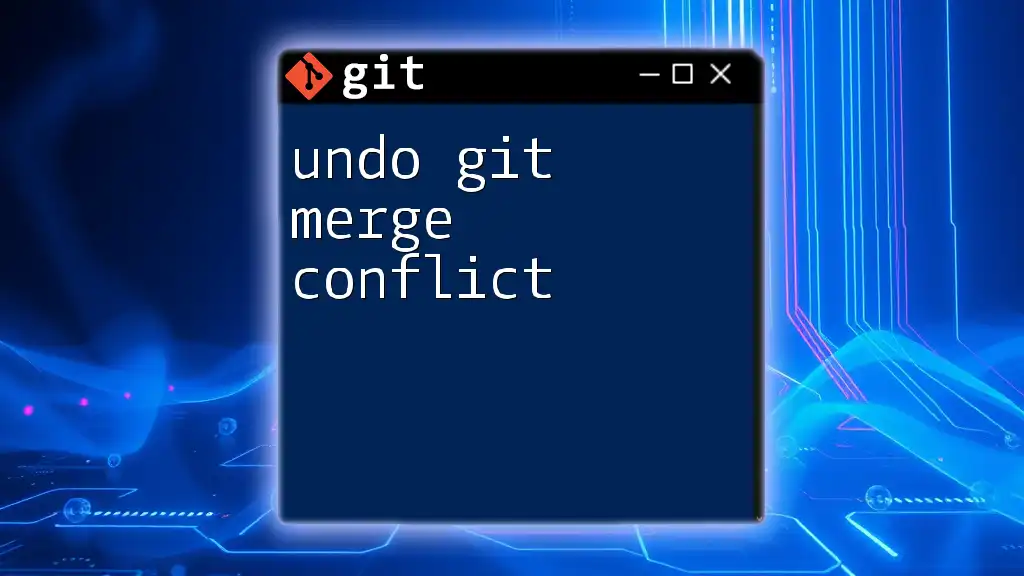
Resolving Merge Conflicts
Manual Resolution of Merge Conflicts
Manually resolving merge conflicts involves a careful review of the conflicting changes. Git uses specific markers to indicate where the conflicts arise:
<<<<<<< HEAD
Current change from the base branch
=======
Incoming change from the feature branch
>>>>>>> feature-branch
Here’s a step-by-step approach to manually resolve these conflicts:
- Open the Conflicting File: Identify and open the file marked by Git.
- Review the Changes: Analyze the changes under each conflict marker to understand how each branch modified the code.
- Edit the File: Decide how to combine or edit the conflicting changes. You can choose to accept one change, the other, or create a new integrated version that incorporates elements from both.
Once you have resolved the conflicts, it’s essential to remove the conflict markers (`<<<<<<<`, `=======`, `>>>>>>>`).
Using a Merge Tool
If manual resolution seems daunting, using a merge tool can streamline the process. Merge tools offer visual interfaces that display both versions of the code side by side, making it easier to identify differences and integrate changes. Some popular merge tools include KDiff3, Meld, and Beyond Compare.
To use a merge tool, simply execute:
git mergetool
This command launches your configured merge tool, allowing you to address conflicts in an intuitive manner.
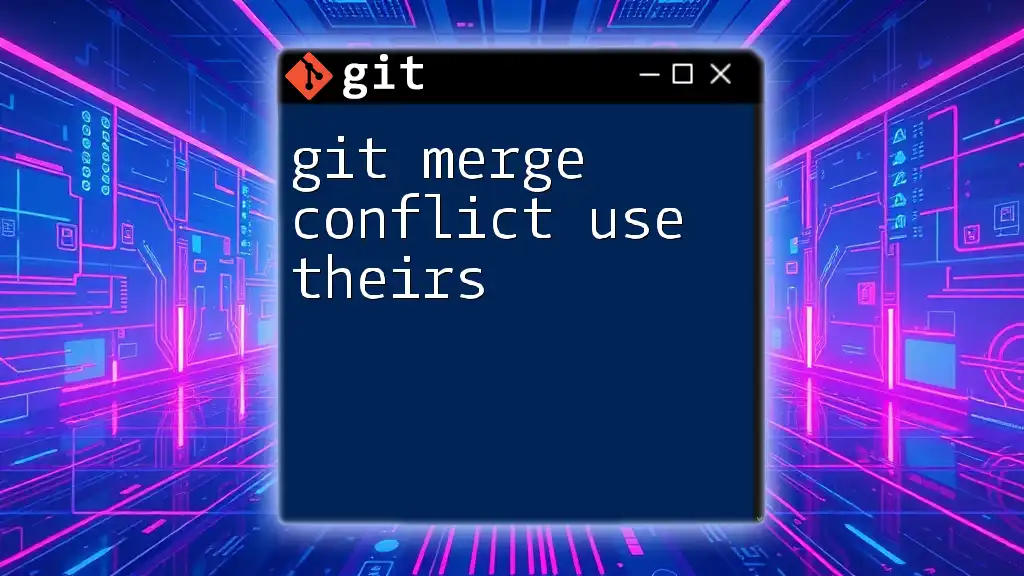
Committing Your Resolved Changes
Staging Resolved Files
After resolving the conflicts, the next step is to stage the resolved files. You can do this using the `git add` command:
git add <resolved-file>
Replace `<resolved-file>` with the name of the file you’ve resolved. This action informs Git that the conflicts have been addressed.
Finalizing the Merge
Once all conflicts are resolved and staged, you can commit the changes. A descriptive commit message clarifying the resolution process is always helpful:
git commit -m "Resolved merge conflicts between main and feature-branch"
By committing the changes, you complete the merge process, and your branch will reflect the new integrated code.
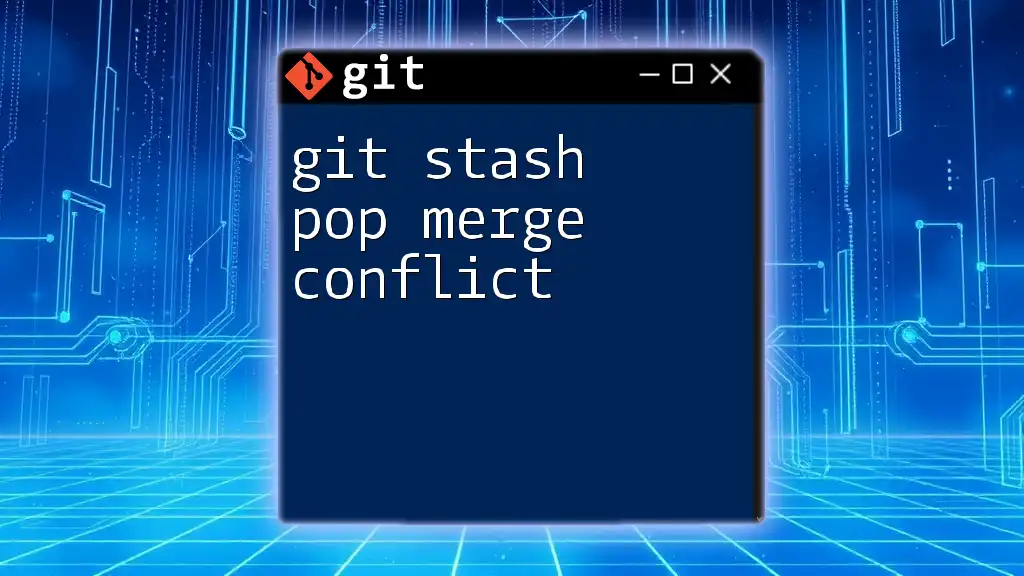
Post-Merge Practices
Verifying Your Changes
Testing is crucial after resolving merge conflicts to ensure that everything functions as expected. Run your tests or check your application manually to confirm that merging didn’t introduce any bugs.
Documenting Merge Conflicts for Future Reference
Keeping notes on merge conflicts can be beneficial for both individual learning and team coordination. Document the nature of the conflicts, how they were resolved, and any patterns observed to help your team in future projects.
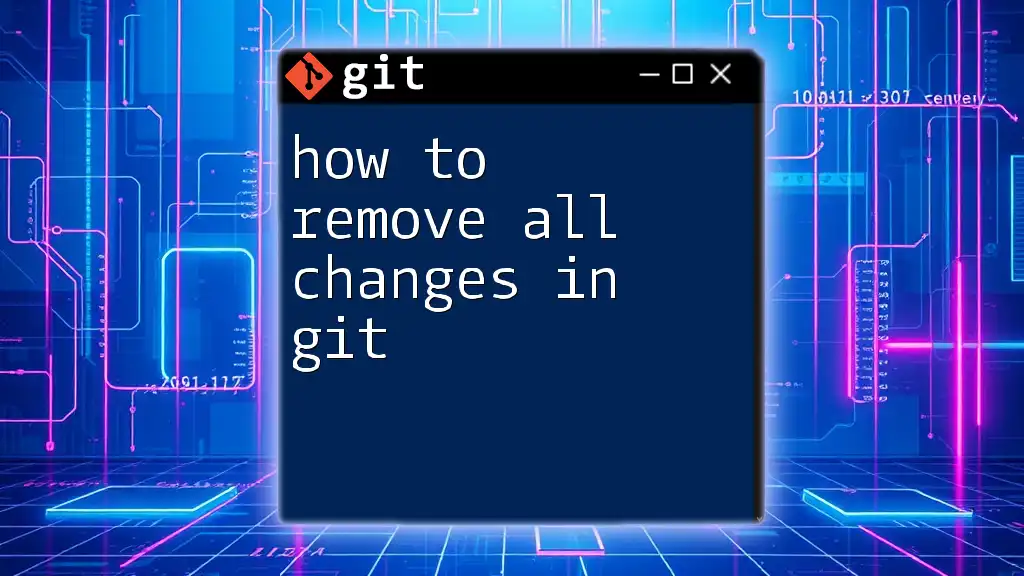
Conclusion
Recap of Best Practices in Conflict Resolution
To effectively manage and resolve merge conflicts in Git, understanding the underlying causes, practicing regular communication, and employing both manual and tool-assisted resolution techniques are essential.
Final Tips for Effective Collaboration Using Git
Encourage open conversations within your team to foster an environment where merging and collaboration are smooth processes. Resourceful individuals will find that the challenges posed by merge conflicts can become learning opportunities, ultimately enhancing overall team proficiency in using Git.
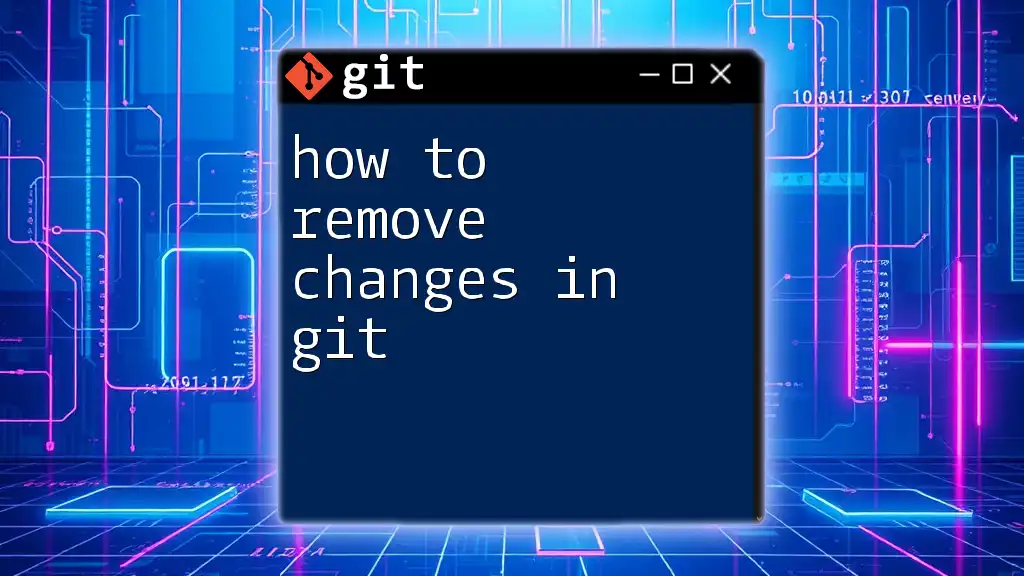
Additional Resources
Recommended Tools and Plugins
For further skill development in Git, consider exploring a variety of tools, references, and communities that can enhance your learning experience. This ongoing education can dramatically improve your efficiency in resolving merge conflicts and collaborating effectively.