To move a folder in Git, use the `git mv` command followed by the current folder path and the new folder path to rename or relocate it within your repository. Here's the syntax:
git mv old-folder-name new-folder-name
Understanding Git Directory Structure
What is a Git Repository?
A Git repository is where all the files and directories related to a project are stored along with their complete version history. It contains everything needed to track changes, collaborate with others, and manage different versions of your project. Understanding the structure of a Git repository is crucial because moving folders can impact not just the appearance of your project but also its functionality and organization.
Importance of Moving Folders
Moving folders within your Git repository can be essential for a variety of reasons, including:
- Organization: Keeping your project tidy by grouping related files together.
- Refactoring: Restructuring your project layout for improved maintainability.
- Collaboration: Adapting the directory structure based on team feedback or project requirements.
However, it's essential to remember that moving folders in a Git repository is more than just a filesystem operation; it also involves version control implications that must be managed correctly.
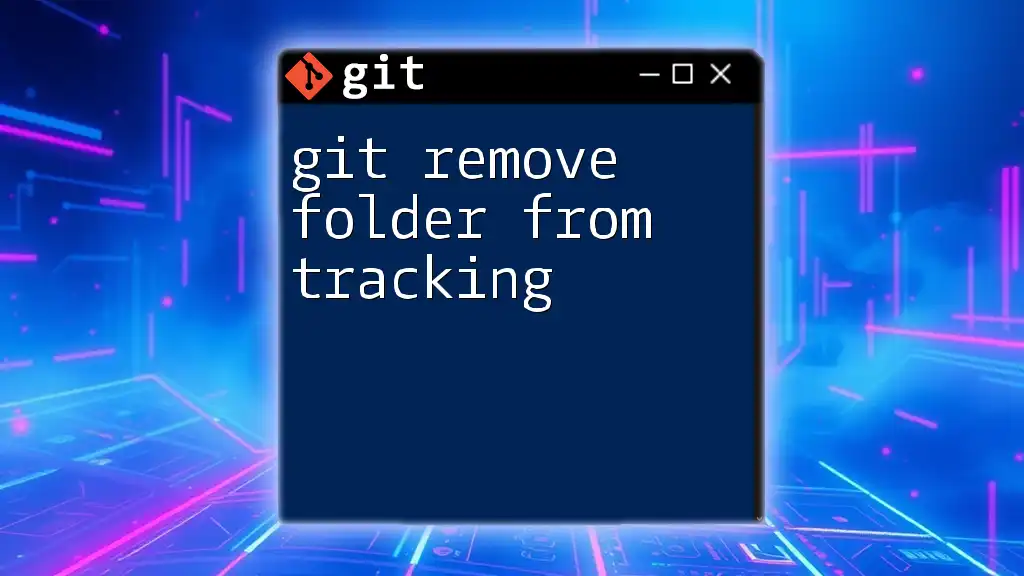
The `git mv` Command
What is the `git mv` Command?
The `git mv` command is a built-in function in Git that allows users to move or rename files and directories within a Git repository. This command not only moves the files but also stages the changes for the next commit, making it more efficient than manually moving files and then running `git add`.
Basic Syntax
The syntax of the `git mv` command is straightforward:
git mv <source> <destination>
Example of moving a folder:
git mv myFolder/ newLocation/myFolder/
In this example, `myFolder/` is the source folder being moved to `newLocation`, and it will retain its name at the new location.
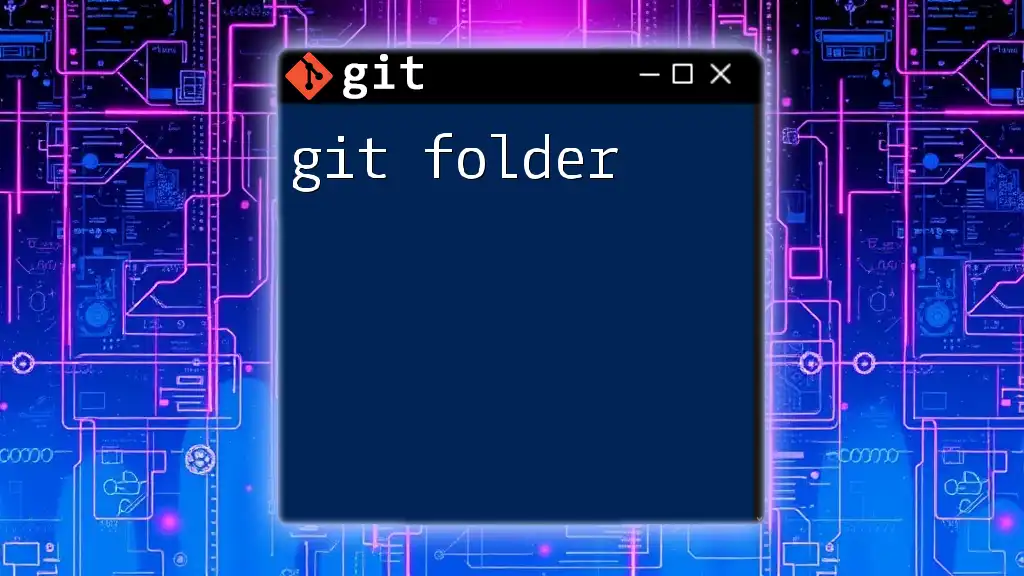
Step-by-Step Guide to Moving a Folder in Git
Preparing to Move the Folder
Before executing any commands, ensure you are on the correct branch in which you want to move the folder. Use the following command to check your current status:
git status
This command will show you the files tracked in your current branch and any changes made. It’s a best practice to confirm that you’re moving the correct folder and that there are no uncommitted changes that could complicate matters.
Executing the Move
To move the folder, simply use the `git mv` command followed by the source and destination folders:
git mv source_folder/ destination_folder/
Make sure to replace `source_folder/` and `destination_folder/` with the actual names and paths of the folders you are working with. This command will relocate the specified folder and its contents while tracking the change.
Reviewing Changes
Once you've executed the move, it's crucial to review the changes made to ensure everything has gone smoothly. You can do this by running:
git status
This command will show you that the folder has been moved and is pending further action. Additionally, you can preview your changes with:
git diff
This command will provide a visual breakdown of the changes made, giving you added assurance before committing.
Committing the Changes
After confirming the move, you'll need to commit your changes to finalize the move within version control. Use the following command to commit:
git commit -m "Moved folder from source to destination"
Importance: Committing your changes is crucial as it records the move in the repository’s history, ensuring that this organizational change is reflected in future developments.
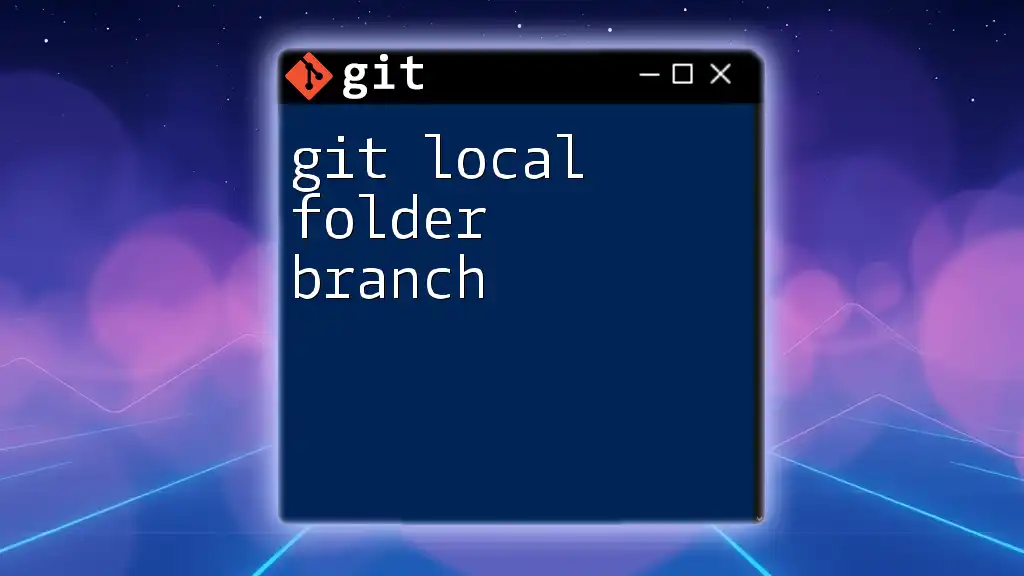
Common Scenarios and Considerations
Moving a Folder with Files
When you move a folder that contains multiple files, Git automatically tracks all contained files in its history. This means that moving `myFolder/` that includes various documents and subfolders will carry those files along without the need for manual staging.
Handling Merge Conflicts
After moving a folder, you may encounter merge conflicts, especially if others are working on the same project. It’s advisable to communicate with your team about major structural changes to avoid such conflicts. In case of conflicts, use:
git status
This command helps identify conflicting files, allowing you to resolve issues accordingly.
Moving Folders in a Submodule
In Git, a submodule allows you to keep a Git repository as a subdirectory of another Git repository. Moving a folder within a submodule follows the same principles as moving folders in a standard Git repository, with additional considerations for the parent repository. Use the `git mv` command as usual but verify that the parent repository recognizes the change.
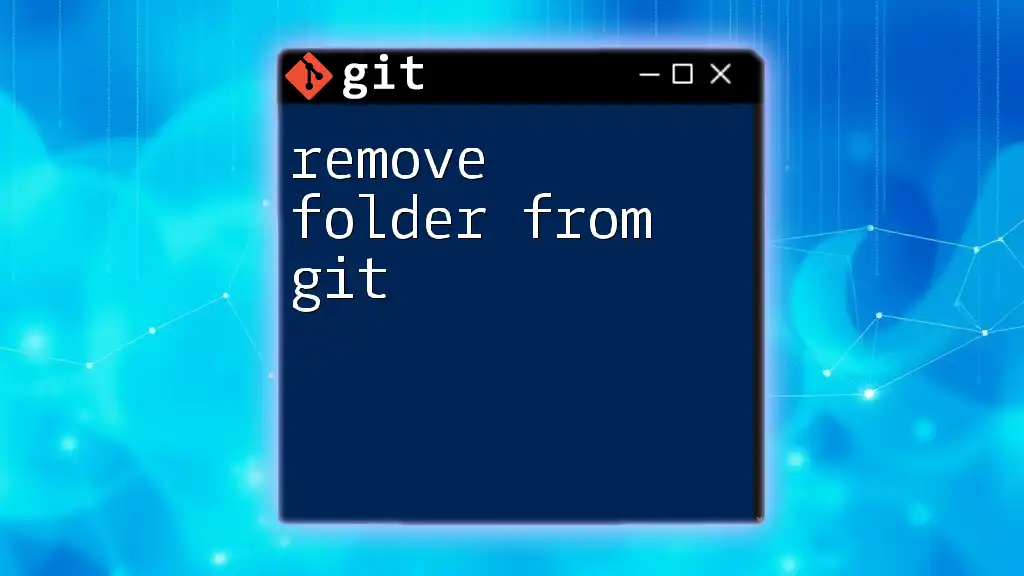
Best Practices for Moving Folders
Keep Commit History Clean
A clean commit history is crucial for future reference and collaboration. Avoid unnecessary noise in your repository by limiting moves to meaningful structural changes. Utilize branching for more significant restructurings and merge them only when ready.
Use Descriptive Commit Messages
When committing your changes, ensure that your commit messages are descriptive and convey the intent of the move. A message like "Moved folder `assets` to `src/assets` for better organization" is more useful than a generic "Updated files."
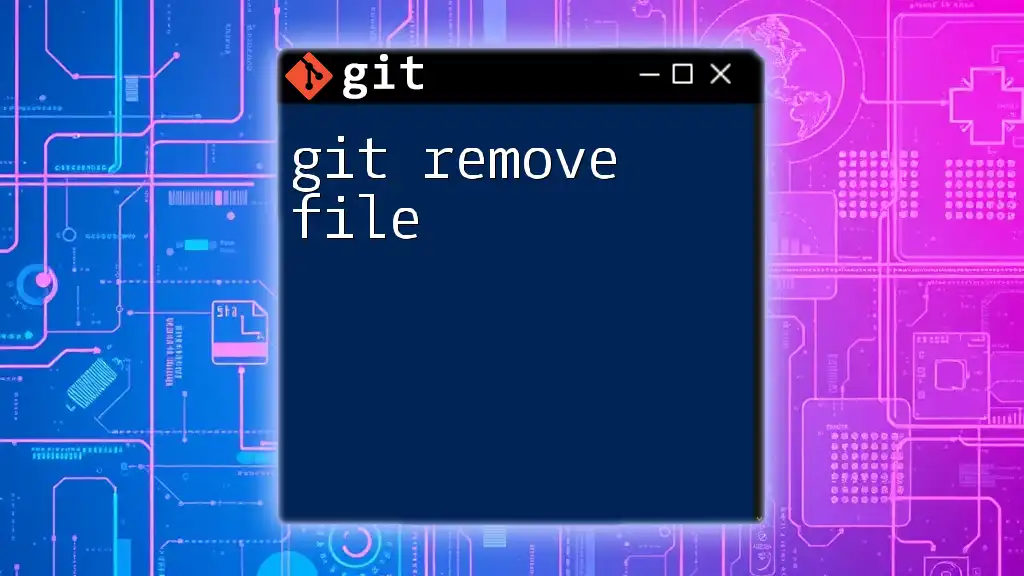
Troubleshooting Common Issues
Folder Does Not Exist
If you attempt to move a folder that does not exist, you’ll encounter an error message. Double-check your folder names and paths. Use:
ls
or
git ls-files
to verify the existence of the folder before trying to move it.
Permissions Issues
Sometimes, permission settings can prevent you from moving folders as expected. In such cases, ensure that your user has the necessary permissions to modify the files or consult system settings or your system administrator.
Mistakenly Moved the Wrong Folder
If you realize that you have moved the wrong folder, there’s no need to panic. You can revert your changes to the last committed state with:
git reset HEAD --hard
This command will discard all changes made after the last commit, so use it cautiously!
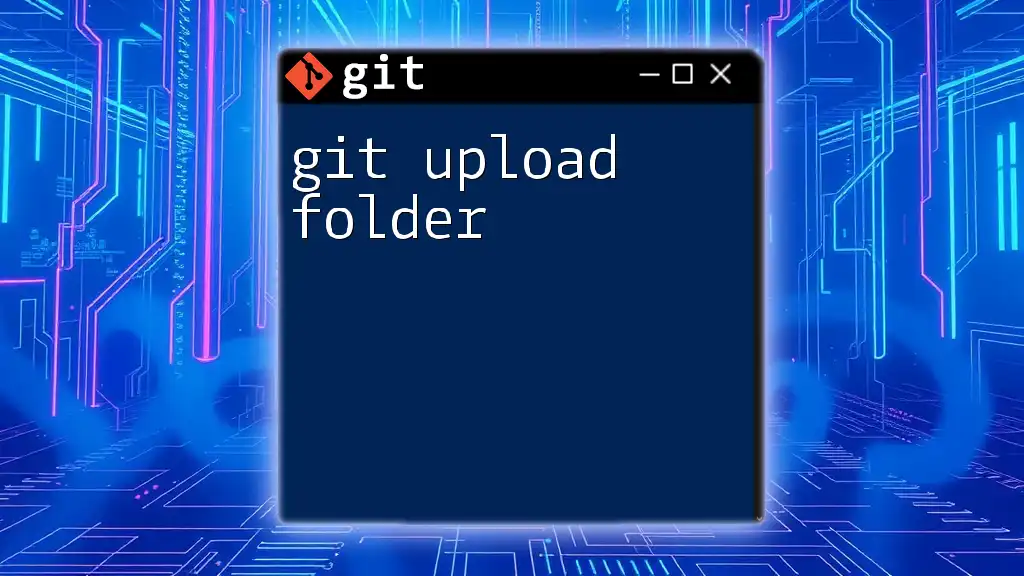
Conclusion
Moving folders in Git can enhance the organization of your repository and improve your productivity. By utilizing the `git mv` command effectively and following best practices, you can ensure your project remains coherent and accessible. Remember to leverage Git's version control capabilities to maintain smooth collaboration and documentation throughout your project's lifecycle.
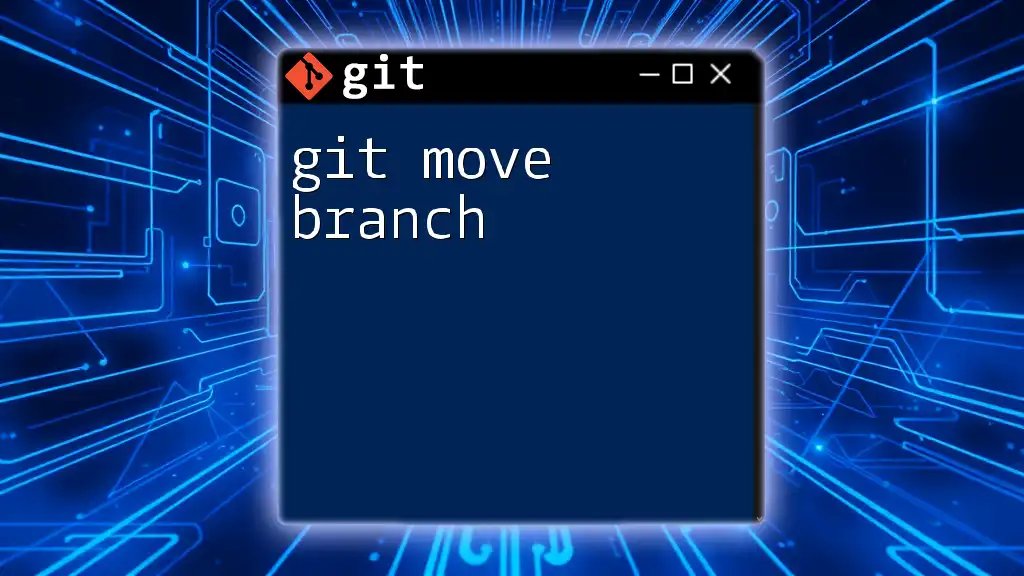
Additional Resources
For further learning, consider checking out comprehensive resources and tutorials available both on Git’s official documentation and various educational platforms specializing in version control systems.
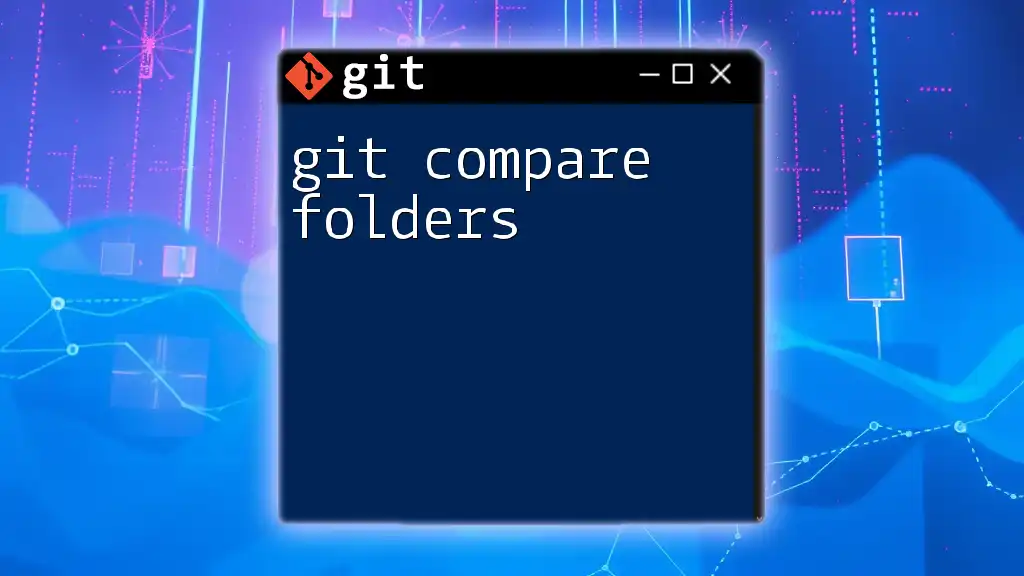
Call to Action
We encourage you to try moving folders using `git mv` in your projects. Share your experiences, challenges, and successes with us and feel free to subscribe for more Git tutorials and practical tips!