The `git mv` command is used to move or rename a file or directory within a Git repository, ensuring that Git tracks the changes and maintains the version history.
git mv old_directory new_directory
Understanding `git mv`
What is `git mv`?
`git mv` is a command in Git that allows users to move or rename files and directories within a Git repository. Unlike the traditional Unix/Linux `mv` command, `git mv` integrates the move operation directly into the version control system. When you use `git mv`, it not only moves the files or directories but also stages the changes for committing, ensuring that the Git history remains intact.
Why Use `git mv`?
Utilizing `git mv` comes with several advantages:
-
Version Control Benefits: When files or directories are moved using `git mv`, Git tracks these changes efficiently. This method preserves the history of the moved items, making it easier to navigate project history later on.
-
Ease of Use: The command simplifies the process of moving files in a manner that aligns with version control principles. It reduces the risk of leaving untracked files or creating confusion about file changes.
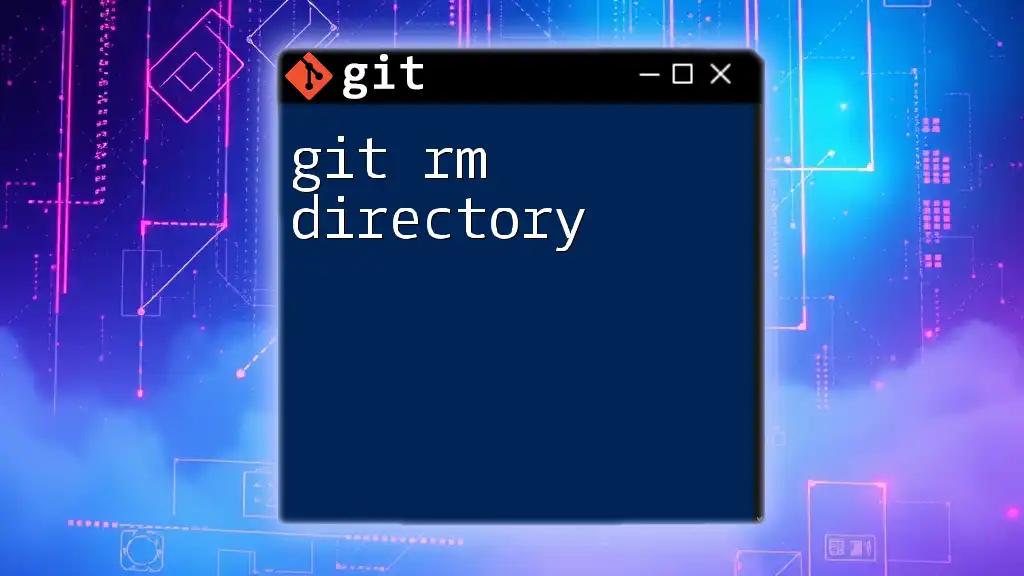
Syntax of `git mv`
General Syntax
The syntax for using `git mv` is straightforward:
git mv [options] <old-directory> <new-directory>
In this command:
- `<old-directory>` is the current path of the directory you wish to move.
- `<new-directory>` is the desired new path for that directory.
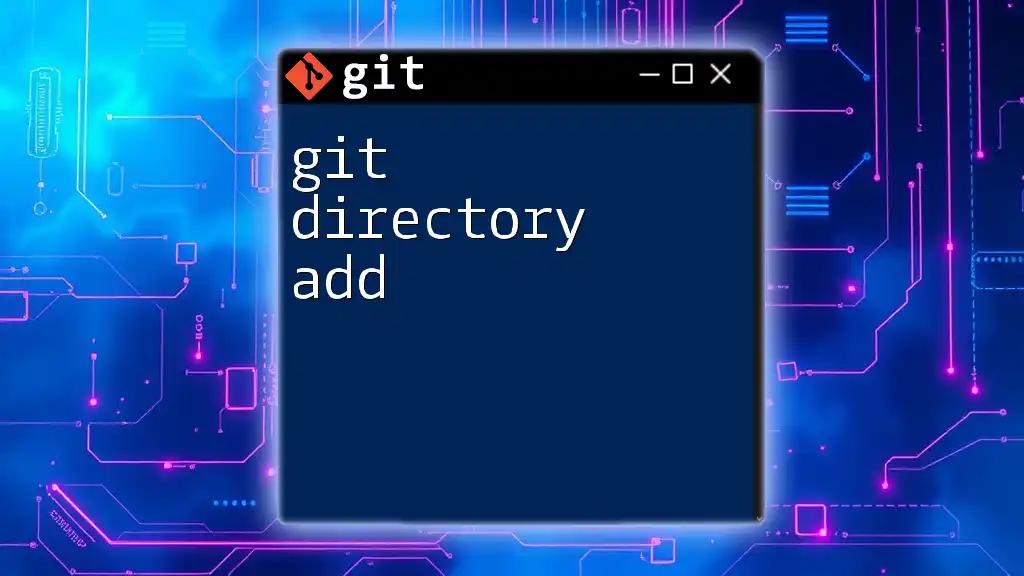
How to Use `git mv` for Directories
Moving a Directory
To move a directory from one path to another, you simply execute:
git mv myOldDir/ myNewDir/
This command takes the directory `myOldDir` and moves it to the new location `myNewDir`. After executing this command, you can run `git status` to see that the move has been tracked. Git will show that `myOldDir` has been deleted and `myNewDir` has been added, both ready to be committed.
Moving a Directory with Subdirectories and Files
If the directory contains files and subdirectories, you can still use the same command:
git mv project/src/ project/source/
This command will seamlessly relocate `src` to `source`, along with all its contents. Even with this complex hierarchy, Git will accurately track the move, preserving the history of the files involved.
Renaming a Directory
Renaming a directory is a specific type of move. For example:
git mv oldDirectoryName newDirectoryName
This command renames `oldDirectoryName` to `newDirectoryName` while maintaining the complete history of files within that directory. Understanding this distinction helps you keep your project organized efficiently.
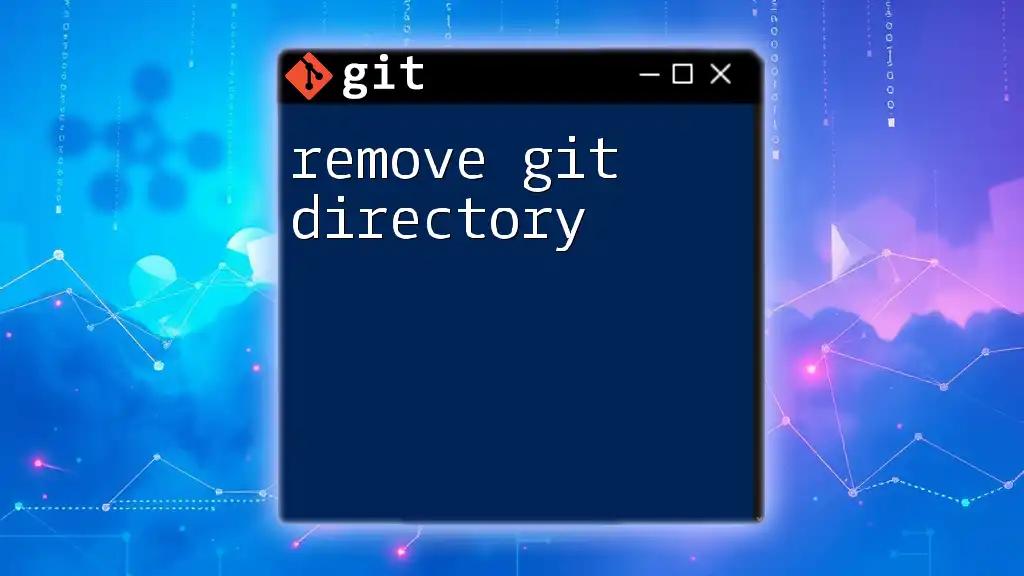
Common Options for `git mv`
`-f` (force)
You may occasionally need to override an existing directory or file without confirmation. The `-f` option allows you to force the move:
git mv -f sourceDirectory/ destinationDirectory/
Using this option can be helpful but should be approached with caution as it will overwrite existing files without prompting.
`-n` (dry-run)
If you're unsure of the move and want to see the outcome before making changes, the `-n` option provides a dry run:
git mv -n source/ destination/
This will simulate the move operation, allowing you to review the results without executing any changes. It's an excellent way to avoid mistakes.
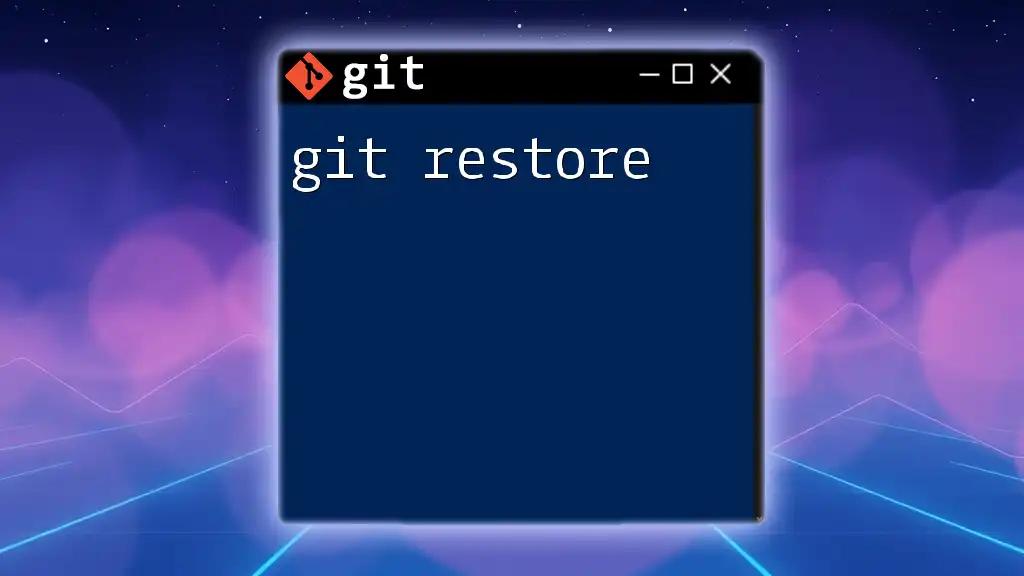
Checking the Status After Moving Directories
Verifying Changes
After executing a move command, checking the status of the repository is crucial. You can verify the changes using:
git status
This command will show the current state of the repository. You can expect to see output indicating that the original directory has been removed and the new directory is staged for commit.
Viewing Commit History
To see how the moved directory fits into the project's history, you can use:
git log --follow <new-directory>
The `--follow` flag helps track the history of the directory through renames and moves, giving you a comprehensive view of its evolution in the repository.
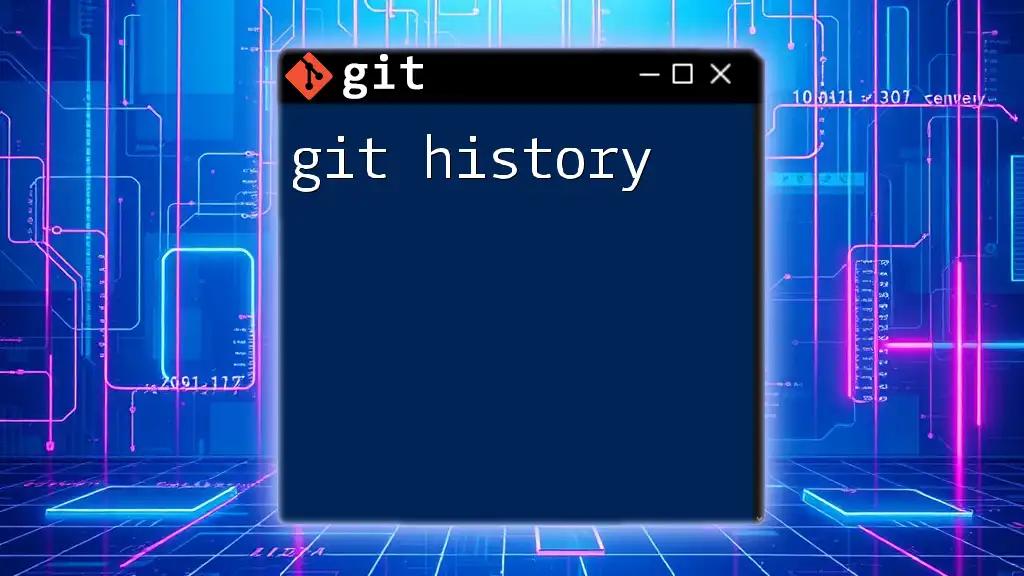
Undoing a Move
Reverting a Directory Move
If you change your mind before staging the move, you can quickly revert it using:
git mv newDirectory/ oldDirectory/
This command simply moves the directory back to its original location, ensuring that any unwanted changes are easily undone.
Using `git reset`
If you've already staged the change but haven’t committed it yet, you can unstage it with:
git reset HEAD <new-directory>
This command effectively undoes the move at the staging level, allowing you to make adjustments or perform other operations without affecting your commit history.
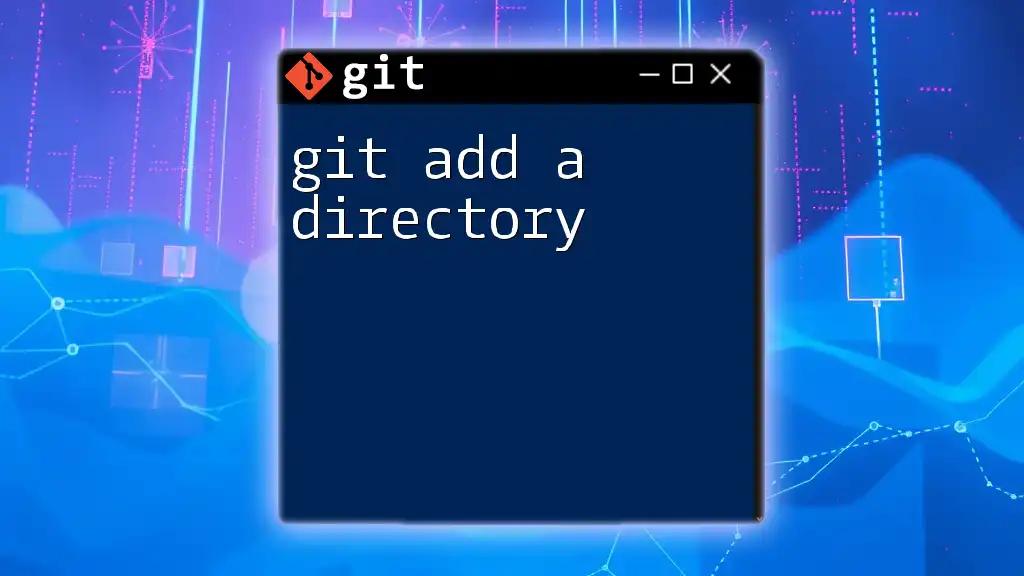
Best Practices for Using `git mv`
Commit Regularly
After moving directories, it's essential to commit your changes promptly. Regular commits maintain a clear and organized history. Crafting expressive commit messages, such as “Moved `src` folder to `source` for better clarity,” will enhance collaboration and understanding.
Consistency in Directory Naming
Maintaining a consistent naming convention for your directories promotes readability across the project. It becomes easier for collaborators to navigate.
Collaboration Considerations
When working in a team, coordinating moves is essential to avoid conflicts. Communicate any significant changes to the directory structure in team meetings or through project management tools.
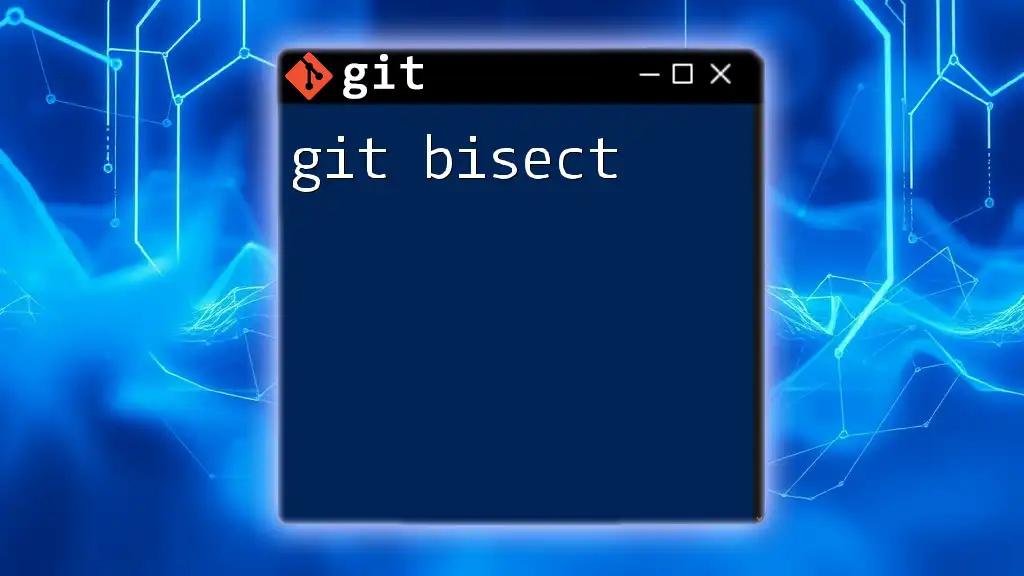
Conclusion
In summary, mastering the `git mv directory` command is vital for any developer working with Git. It offers significant advantages in managing files and directories while ensuring the integrity of version history. With practice, using `git mv` will streamline your workflow, help maintain organization within your repositories, and simplify the collaborative development process.
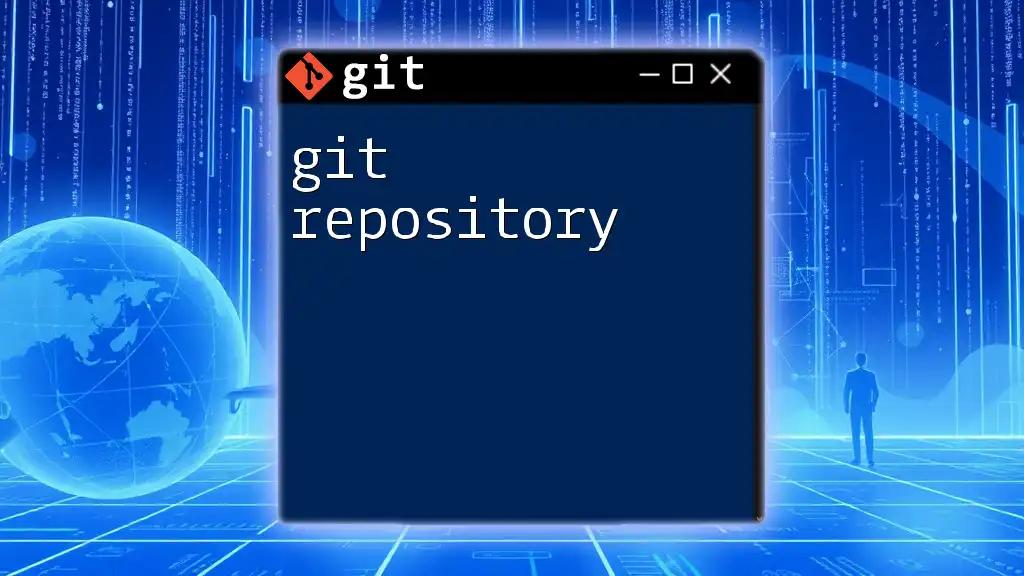
Additional Resources
Reference Links
- For an in-depth understanding, refer to the official [Git Documentation](https://git-scm.com/doc).
- Explore additional tutorials and resources about Git commands and best practices for a more comprehensive learning experience.
Related Commands
Familiarizing yourself with other relevant Git commands such as `git rm`, `git add`, and `git commit` will enhance your overall command of Git, especially when managing directories.
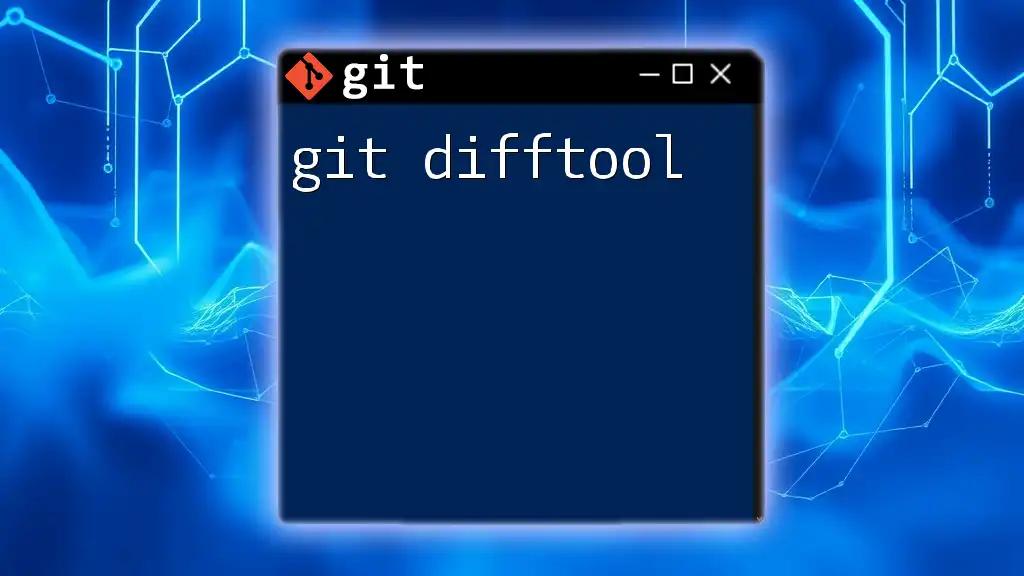
FAQs
What happens if I move a directory that is being tracked?
If you move a tracked directory using `git mv`, Git will automatically detect the move, ensuring the history and versioning of its contents are retained.
Can I move directories across different branches?
Yes, you can move directories across branches, but make sure to commit your changes before switching branches to avoid losing work.
How does `git mv` differ from conventional file system moves?
Using `git mv` incorporates move operations into the Git history, meaning it tracks changes and maintains a comprehensive versioning of files, unlike a regular file system move that does not consider version control.