The `git rm` command is used to remove a directory and its contents from both the working directory and the staging area in a Git repository.
git rm -r directory_name
Understanding `git rm`
What is `git rm`?
The `git rm` command is a key tool in Git that allows you to remove files or directories from your working directory and staging area. It serves as a way to tell Git that you want to keep track of the removal of a file in your version history. Importantly, `git rm` removes files from the working directory as well as from the staging area in preparation for the next commit, ensuring they will no longer be tracked in future updates.
How `git rm` Works
When you execute `git rm`, the command stages the removal of the specified files or directories for the next commit. This means that the files will be deleted from your filesystem, and Git will record this change so that the file no longer exists in the project’s history after the commit is made. Understanding the role of the staging area is crucial, as it holds the changes that will be recorded in the next commit.
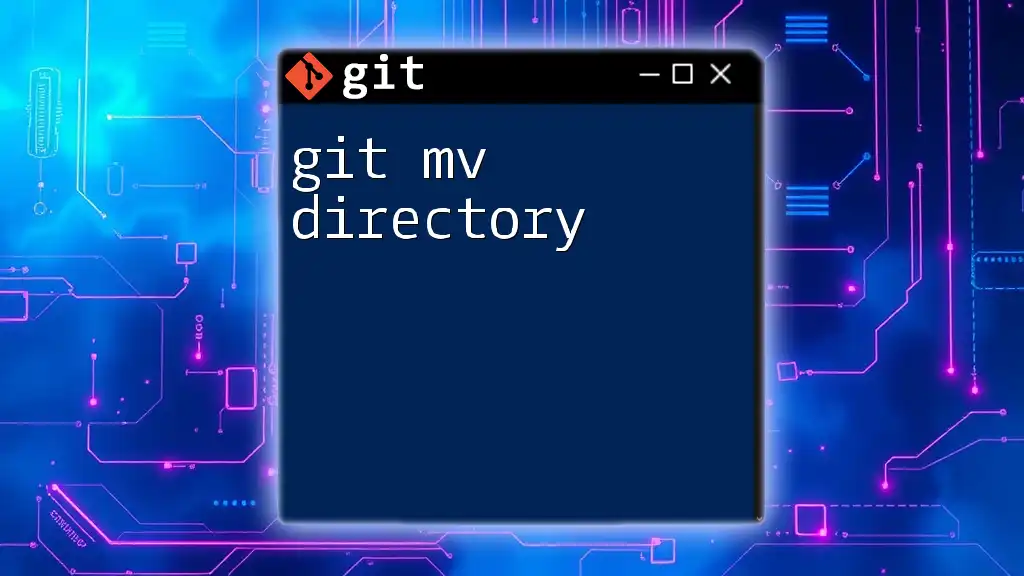
Using `git rm` to Remove a Directory
Syntax of the Command
The basic syntax for using the `git rm` command is straightforward:
git rm [options] <file or directory>
When it comes to directories, one of the most important options you will use is `-r`, which stands for recursive.
Removing a Non-Empty Directory
Important Note: Git does not allow you to remove a directory directly if it contains files. Instead, you must use the recursive option.
To remove a non-empty directory, you would use:
git rm -r my-directory/
In this command, the `-r` flag ensures that Git goes through the specified directory and removes all the contained files and subdirectories, marking everything for deletion in the Git index.
Removing an Empty Directory
While `git rm` is effective for removing files and non-empty directories, it does not support removing empty directories.
When Git tracks files, it does not track empty directories because it only keeps track of files that contain content. If you want to remove an empty directory, you can either remove it manually using standard file commands (like `rmdir` in Unix-based systems) or follow these steps:
- Create a `.gitkeep` file (or similar) in the directory you want to keep tracked.
- If you decide later to remove it, first stage the change and commit:
git rm --cached my-empty-directory/
- Finally, remove the directory manually:
rmdir my-empty-directory/
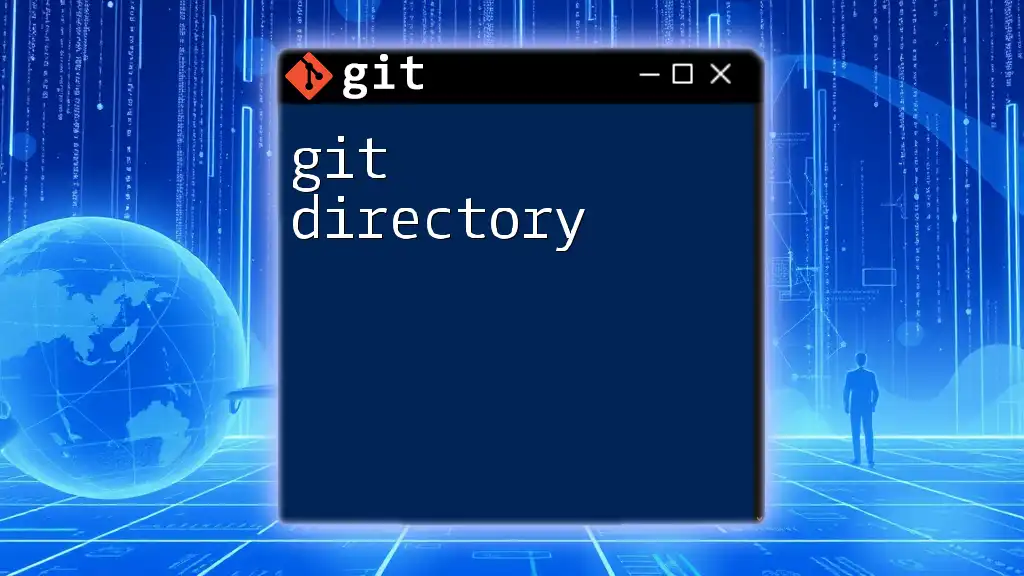
Practical Examples
Example 1: Removing a Directory and All Its Contents
Suppose you've created a directory and added some files. Here’s how you can remove it:
mkdir my-directory
touch my-directory/file1.txt
git add my-directory/
git commit -m "Added directory with file"
Now, if you decide to remove `my-directory` and everything inside it, you would run:
git rm -r my-directory/
git commit -m "Removed my-directory and its contents"
In this example, every command is significant. You create a directory and add a file, stage it with `git add`, and commit your changes to include the directory in your Git history. The removal commands then effectively delete the directory from both your filesystem and the tracked history.
Example 2: Removing Only Specific Files in a Directory
You may not always want to remove an entire directory. For instance, you might only want to delete specific files. Here’s how you would do that:
git rm my-directory/file1.txt
After executing this command, only `file1.txt` will be removed from the directory, while other files in `my-directory` will remain unaffected. This command provides flexibility in managing file tracking, allowing you to keep certain files while removing others as needed.
Example 3: Using `--cached` Option
Sometimes, you may want to remove a directory from tracking in your Git repository but not delete the actual files from your working directory. This is where the `--cached` option comes in handy. It tells Git to stop tracking the specified files without deleting them from your local filesystem.
You can achieve this with:
git rm --cached my-directory/
After running this command, `my-directory` will remain intact in your system, but Git will no longer track it. This is particularly useful when untracking files that should not be in version control, such as configuration files or local environment setups.
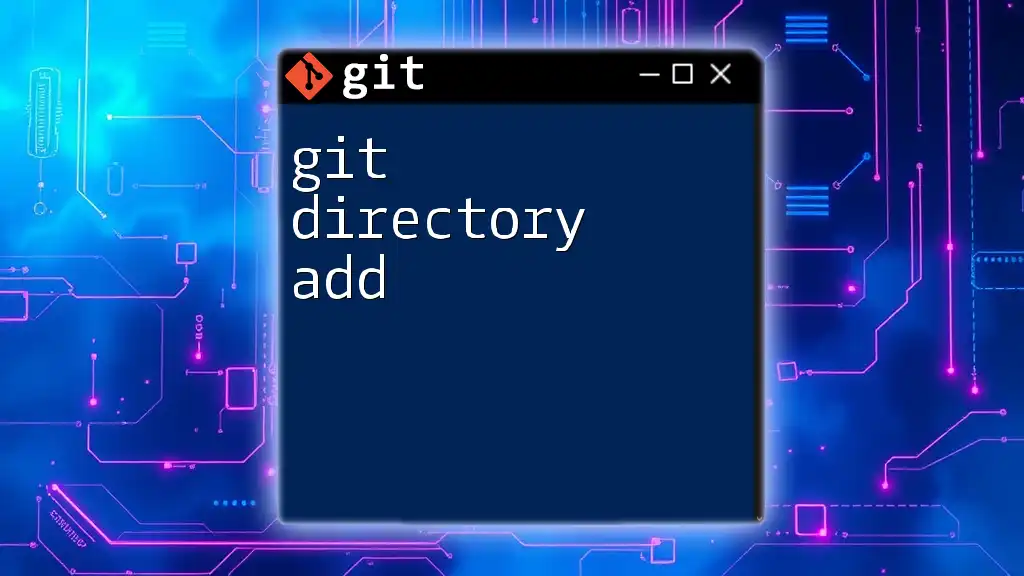
Important Considerations
Impact on the Repository
Understanding the impact of using `git rm` is crucial for maintaining a clean and useful project history. When you remove files using `git rm`, they will be deleted in the next commit. Keep in mind that this action is not easily reversible once committed, as previous versions will reflect the file's absence. It's important to consider whether you truly want to lose those files from the repository's history.
Best Practices When Using `git rm`
To avoid mishaps, here are some best practices to follow when using the `git rm` command:
- Always double-check directory contents: Before removing a directory, especially a non-empty one, ensure that there are no important files that you might need in the future.
- Back up files before deletion: If you’re unsure about the necessity of files, consider creating a backup or temporarily moving them to a different location.
- Understand the scope of your changes: Before committing after a removal, review your changes using `git status` to ensure that you're aware of what will be permanently removed.
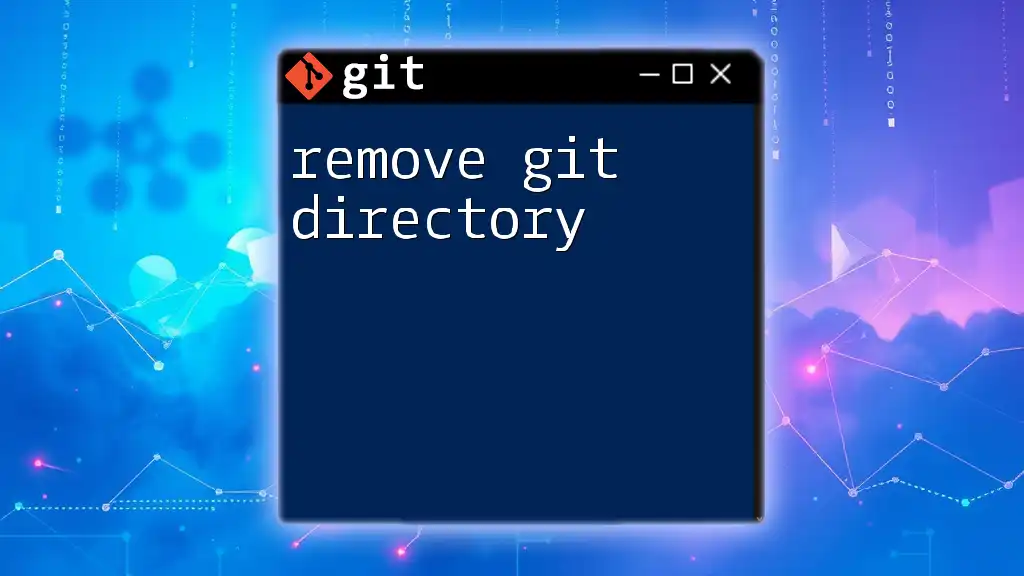
Conclusion
Using the `git rm directory` command is an essential operation for any Git user managing projects effectively. By understanding how `git rm` operates, practicing careful removal, and implementing best practices, you can ensure a streamlined workflow that keeps your version control organized and your repository clean. Remember, always stage your changes thoughtfully to maintain the integrity of your project history.
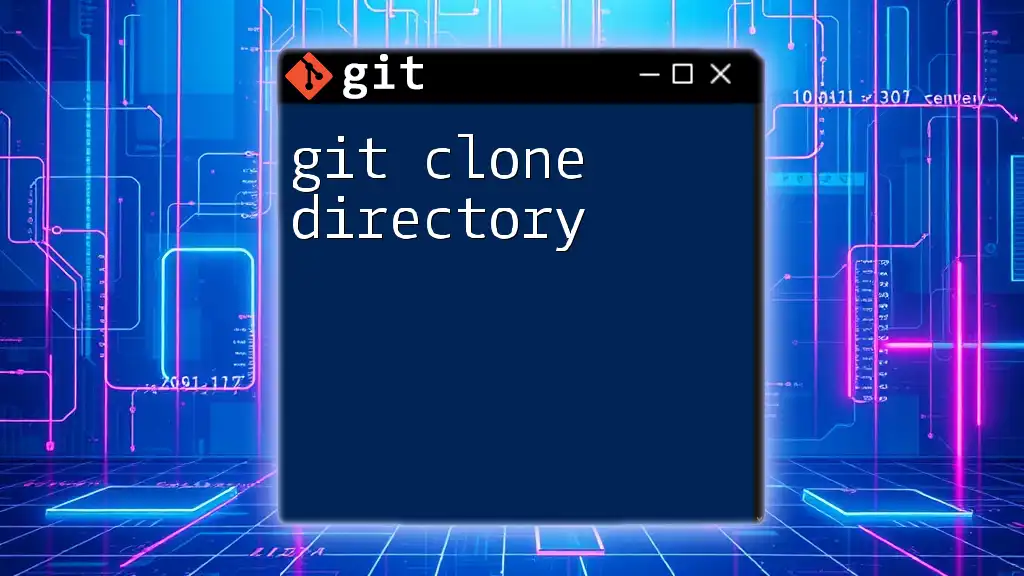
Additional Resources
For further exploration of Git operations, consider reviewing the official [Git documentation](https://git-scm.com/doc) and related tutorials that can offer deeper insights into comprehensive version control practices.