A Git folder, also known as a repository, is a directory that contains all your project files and the necessary metadata for version control along with the Git history of changes.
Here's an example of how to clone a Git repository into a folder:
git clone https://github.com/username/repository.git
Understanding Git Folders
What is a Git Folder?
A Git folder is the core structure that Git uses to keep track of the history and state of projects. Whenever you initialize a Git repository, it creates a `.git` directory that contains all the necessary information about the versions, branches, and commits of your project.
Importance of Directory Structure in Git
Maintaining a structured folder becomes crucial, especially in team environments. A well-organized directory not only helps streamline collaboration but also minimizes confusion about where to find particular files or resources. Each file and folder can play a pivotal role in the version control process, affecting how changes are tracked and integrated over time.

The Components of a Git Folder
The .git Directory
The `.git` directory is the backbone of your Git project. It holds vital metadata necessary for version control. When you initialize a repository, here's what is created inside the `.git` directory:
- objects/: This subdirectory contains all the data blobs, which are the actual content of your files as well as all past versions of your repository.
- refs/: This holds the pointers to branches and tags in your repository, helping Git know what changes belong where.
- HEAD: This file is crucial as it points to the latest commit in your currently checked-out branch, allowing Git to know which files to track and update based on changes.
Working Directory vs. Staging Area
It's essential to distinguish between the working directory and the staging area. The working directory is the workspace where you actively edit files, while the staging area acts as a buffer.
When you make changes to your working directory, those alterations do not automatically become a commit. They first need to be added to the staging area. This two-step process allows you to group changes logically before finalizing them with a commit.

Navigating and Managing Git Folders
Initializing a Git Repository
To create a new git folder, you need to initialize a Git repository. This can be done with the following command:
git init my-repo
This command creates a new directory named `my-repo` and sets it up as a Git repository. Inside this directory, the `.git` folder will now contain all the necessary subfolders and files for version control.
Cloning a Git Repository
If you wish to duplicate an existing repository, you can use the `git clone` command. This action copies all the contents of the repository, including its `.git` directory. Here’s how you do it:
git clone https://github.com/username/repo.git
When executed, Git creates a local copy of the entire project, including all its history, branches, and tags, organized into a corresponding folder.
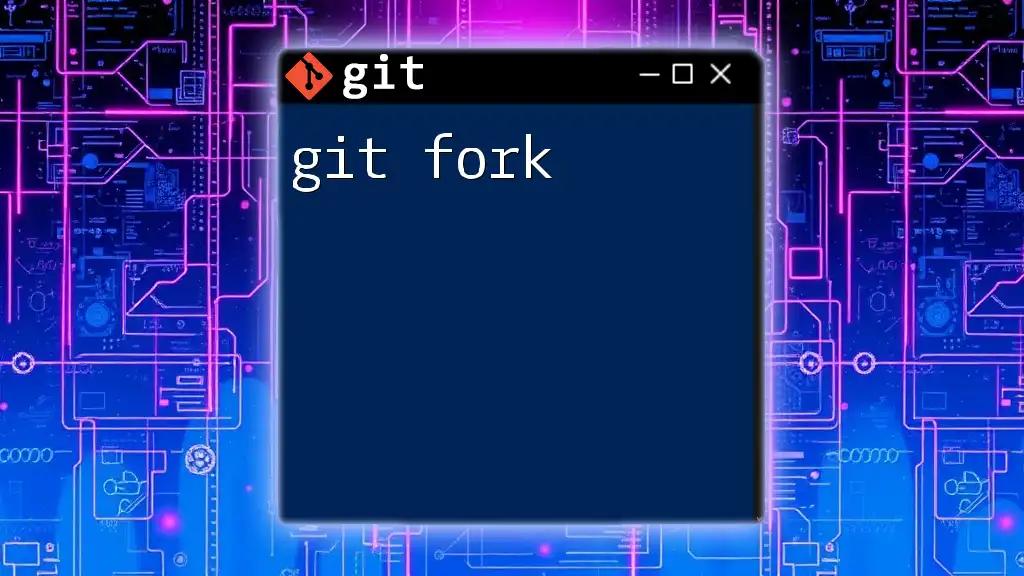
Working with Git Folders
Adding and Committing Changes
When you've made changes to files and want to include them in your repository, you must first add them to the staging area. The command to do this is:
git add <file_name>
This command informs Git that the specified file is ready to be committed. It’s important to choose meaningful file names to keep your changes organized.
Once files have been added to the staging area, you can commit those changes as follows:
git commit -m "Your commit message"
A good commit message is crucial; it should clearly describe the changes made, allowing others (and yourself) to easily understand the why behind your modifications.
Viewing the Status of Your Git Folder
To keep track of the current state of your git folder, use the `git status` command:
git status
This command provides an overview of which files have been changed, which are staged for commit, and which are still untracked, helping you stay organized and aware of your project's progress.
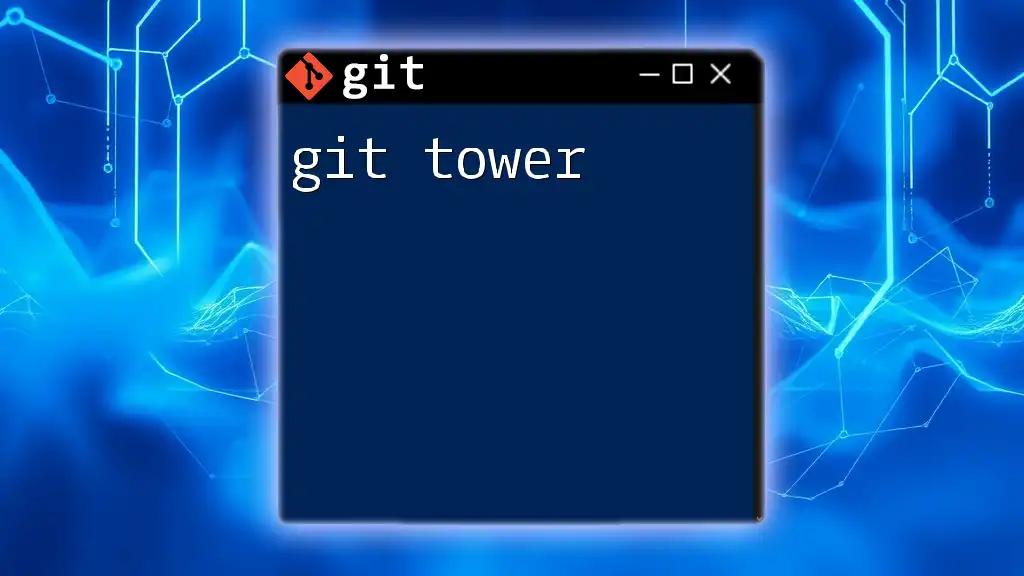
Advanced Git Folder Management
Branching and Merging
Branching is one of Git's most powerful features, allowing you to create divergent versions of your project. To create and switch to a new branch, use the following commands:
git branch new-branch
git checkout new-branch
This enables you to work on features or fixes independently without affecting the main codebase.
Once your changes have been finalized in a branch, you can merge them back into the main branch. Use the following command:
git merge new-branch
Be prepared for potential merge conflicts—Git will highlight the portions of your files that need to be reconciled. Handling these conflicts requires a clear understanding of what changes should remain in the final version.
Deleting Files and Directories
To remove files from your git folder, you can use:
git rm <file_name>
git commit -m "Removed file"
This action deletes the specified file from both the working directory and the index, creating a record of the deletion within your commit history.
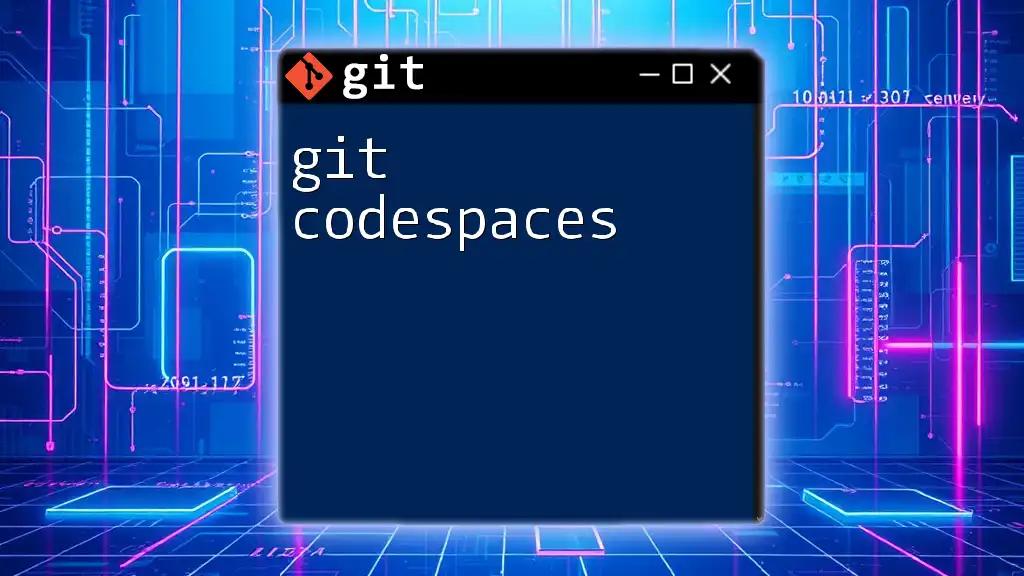
Best Practices for Git Folder Management
Organizing Your Project Structure
A well-thought-out project directory structure can make a significant difference. Consider establishing subdirectories for different components such as `src` for source code, `tests` for testing scripts, and `docs` for project documentation. Each of these organizes the project logically and helps collaborators understand its structure clearly.
Keeping Your Repository Clean
Regular maintenance can prevent git folders from becoming cluttered. Utilizing the `git gc` command can help optimize your repository, cleaning up unnecessary files and reducing overall size.
Documentation and README Files
Including a `README.md` file is essential for any Git repository. This document should outline the project's purpose, how to install and run it, dependencies, and examples. Documentation is vital for onboarding new collaborators and maintaining long-term project viability.
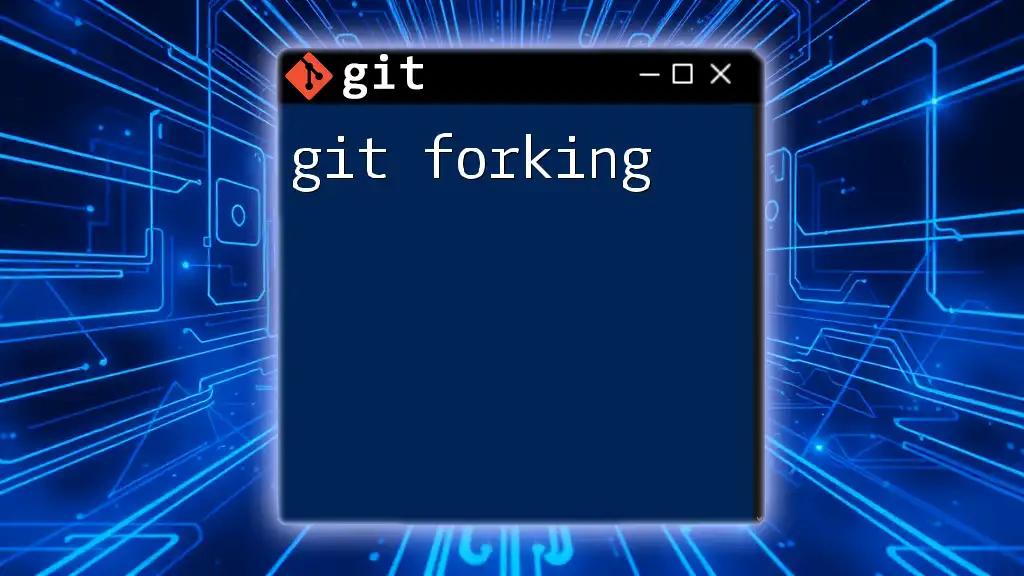
Conclusion
Recap of Git Folder Best Practices
To effectively utilize a git folder, you should understand its components, maintain an organized structure, keeping the repository clean and well-documented, and ensuring collaborative improvement through effective branching practices.
Encouragement to Practice
As you embark on your journey with Git, remember that practice is pivotal. Start applying these commands within your own projects to discover the benefits of using Git for version control and collaboration.
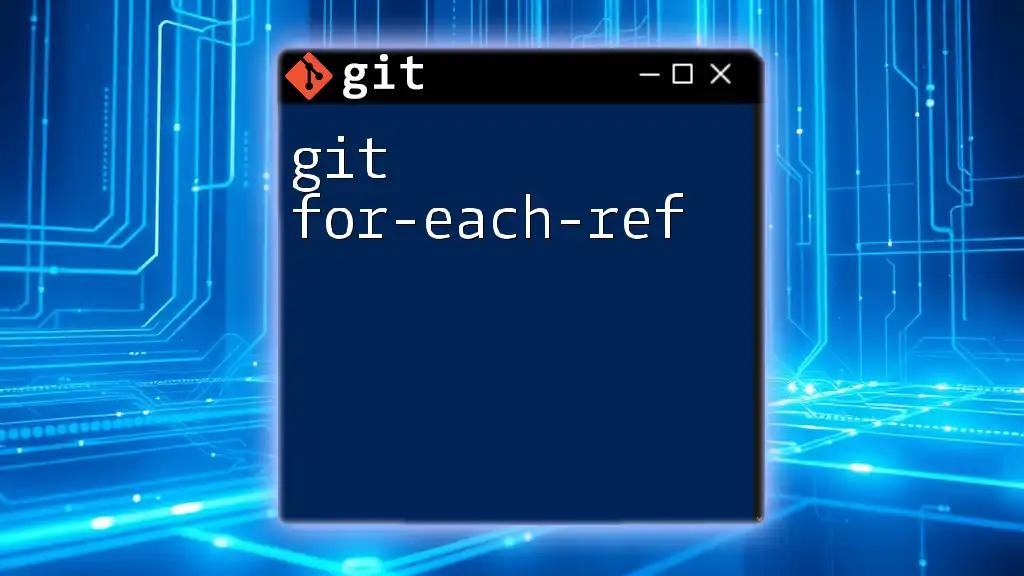
Additional Resources
For further learning, consider exploring Git's official documentation, various GUI clients, and educational platforms that offer hands-on exercises to improve your command of Git folders and the version control process.