CLI Git refers to the command-line interface for Git, allowing users to manage version control tasks efficiently through textual commands rather than graphical user interfaces.
git commit -m "Your commit message here"
What is Git?
Git is a powerful, widely-used version control system that allows developers to track changes in their code, collaborate with others, and manage project history effectively. As a distributed system, it provides every developer with a full copy of the repository, enabling offline work and robust collaboration.
Understanding Git is crucial in today's software development landscape. It allows teams to work simultaneously on the same project without stepping on each other's toes, thereby fostering a more efficient and organized workflow.
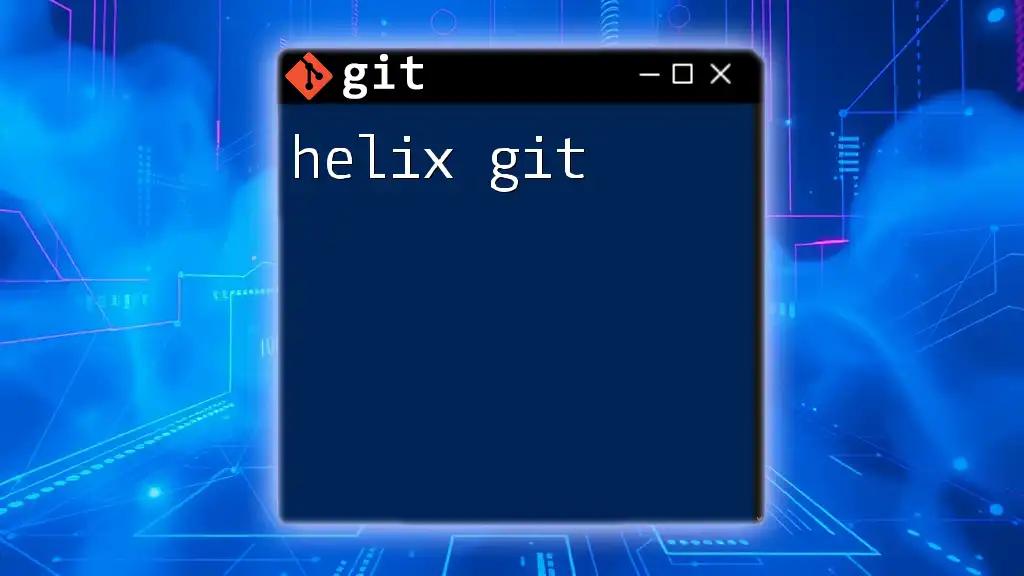
What is CLI?
The Command-Line Interface (CLI) is a text-based interface used to interact with computer systems and applications. Unlike Graphical User Interfaces (GUIs), where users click buttons and icons, the CLI allows users to execute commands through text input. This can make the interaction faster and more precise, especially for experienced users.
Advantages of using CLI for Git
- Speed: Using commands can be much faster than navigating through graphical interfaces.
- Efficiency: Advanced users can perform complex tasks quickly without needing to mouse click.
- Scriptable: CLI commands can easily be incorporated into scripts for automation, making it easier to manage repetitive tasks.
- Control: Fine-tuned control over operations can be achieved using CLI with greater flexibility.
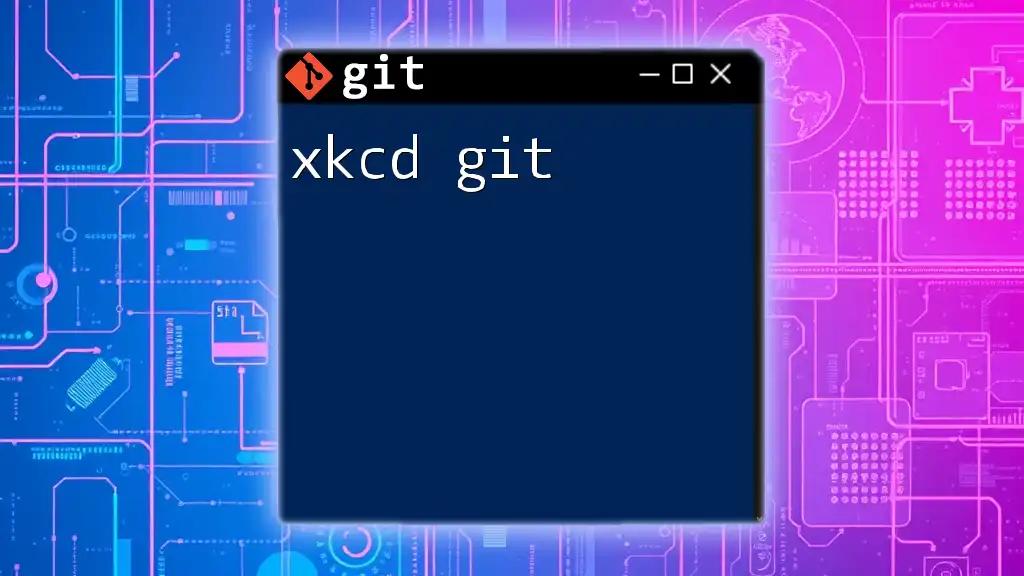
Setting Up Git on Your Machine
Installing Git
To start using CLI Git, you first need to install Git on your machine. Depending on your operating system, the process may vary slightly:
- Windows: Download and run the Git installer from the official Git website. Follow the prompts and choose the default options for most users.
- macOS: You can install Git using Homebrew by running:
brew install git
- Linux: Use your package manager. For example, on Ubuntu, you can run:
sudo apt-get install git
Configuring Git
Once Git is installed, it's important to configure your settings so that your commits are accurately attributed. You can do this with the following commands:
- Setting up your user name:
git config --global user.name "Your Name"
- Setting up your email:
git config --global user.email "you@example.com"
After running these commands, you can verify your configurations by executing:
git config --list
This will display all your Git settings, confirming that everything is set correctly.
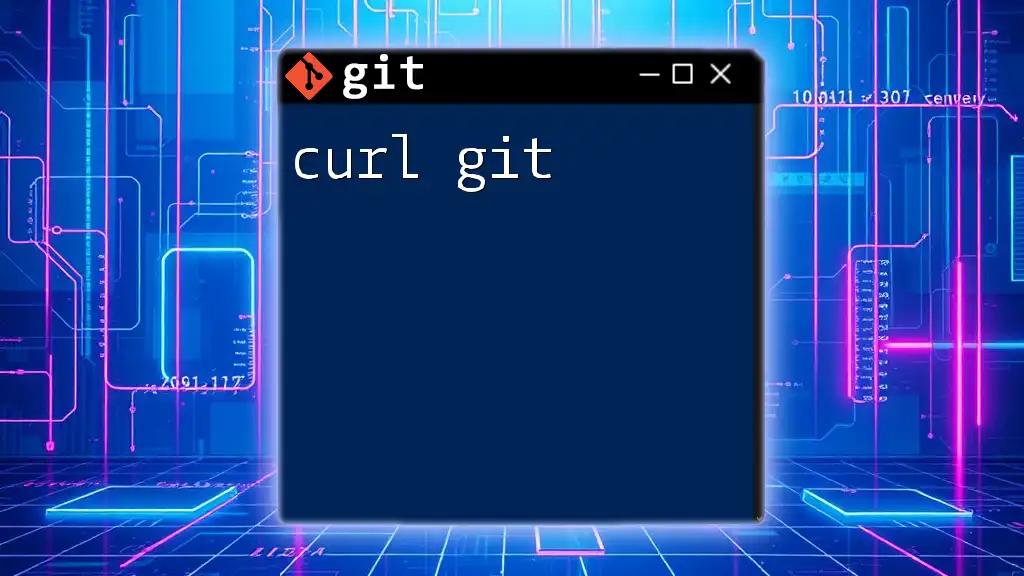
Essential Git Commands in CLI
Creating a New Repository
To start a new project with Git, you need to create a repository. Here’s how to do that:
-
Create a directory for your project:
mkdir my-project cd my-project
-
Initialize a Git repository:
git init
This command will set up a new `.git` directory in your project folder, where all the necessary files for version control will be stored.
Cloning an Existing Repository
If you want to contribute to an existing project, you can clone a remote repository to your local machine. The command would look like this:
git clone https://github.com/user/repo.git
This command creates a complete local copy of the repository, including all its history.
Checking the Status
To see the current state of your working directory and staging area, use:
git status
This command provides a summary of files that are staged for commit, files that are modified, and untracked files. This overview helps you understand what needs to be addressed before making a commit.
Staging Changes
Before committing, you must stage your changes. This is done using the `git add` command:
-
Adding a specific file:
git add filename.txt
-
Adding all changes:
git add .
Committing Changes
Once you've staged your changes, you can commit them to the repository. Use:
git commit -m "Your commit message here"
Writing meaningful commit messages is a crucial practice as it provides context for why changes were made.
Viewing Commit History
To review the history of your project, you can use:
git log
This command displays a list of commits in the current branch, showing commit IDs, authors, and messages. You can enrich this output with various options, such as `--oneline` for a compact view or `--graph` to visualize branching.
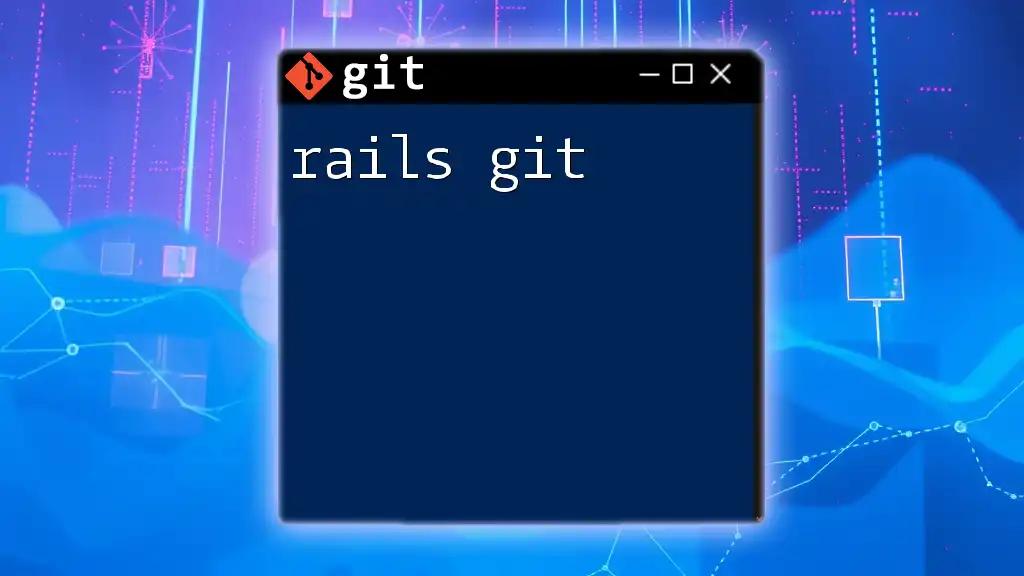
Branching and Merging
Understanding Branches
A branch in Git represents an independent line of development. Branching is valuable as it allows developers to create features, fix bugs, or experiment without affecting the main codebase.
Creating and Switching Branches
To create a new branch, use:
git branch feature-branch
Then, switch to that branch with:
git checkout feature-branch
As of recent updates, you can also create and switch branches in one step using:
git switch -b feature-branch
Merging Branches
Once you've made changes in your new branch and wish to incorporate them into another, you can merge. First, switch to the branch you want to merge into (e.g., `main`) and then run:
git merge feature-branch
Sometimes, you may encounter merge conflicts when two branches have competing changes. Git will highlight these conflicts, and you’ll need to manually resolve them before completing the merge.
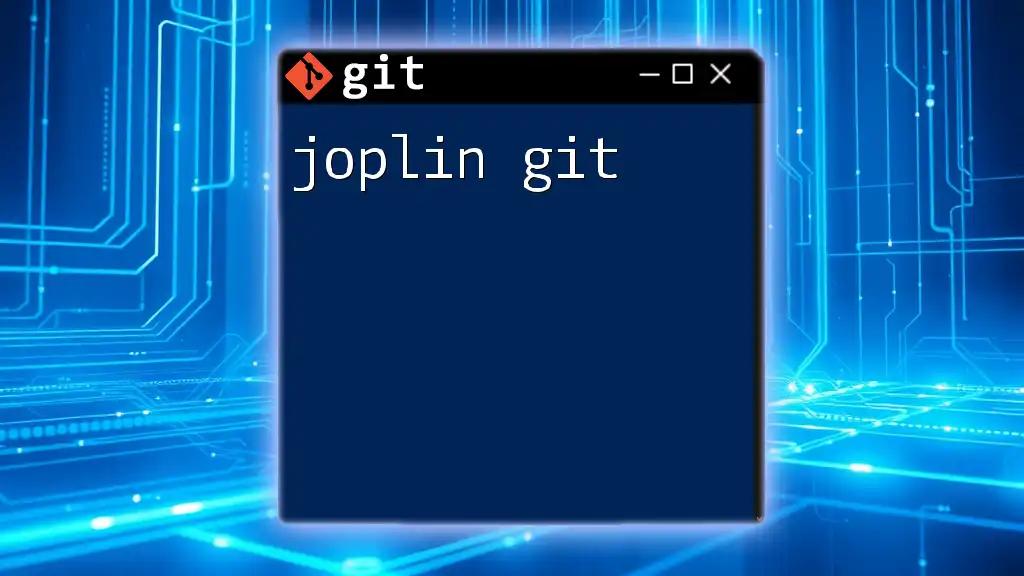
Collaborating with Remote Repositories
Adding a Remote Repository
To link your local repository with a remote one, use:
git remote add origin https://github.com/user/repo.git
This command establishes a connection with the remote repository, enabling you to push and pull changes.
Pushing Changes to Remote
To upload your changes to the remote repository, execute:
git push origin master
This command updates the remote repository with your local changes, making them available to others.
Pulling Changes from Remote
To fetch and integrate changes from the remote to your local repository, use:
git pull origin master
This command combines fetching and merging, making it an efficient way to keep your local repository in sync with remote developments.
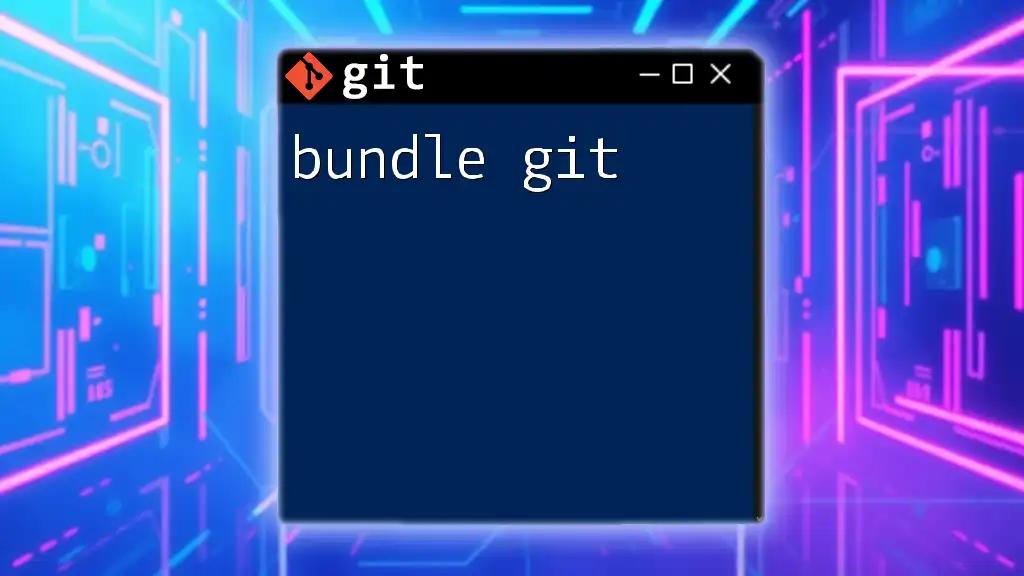
Advanced Git Commands
Rebasing
Rebasing is a powerful feature that rewrites the commit history. Instead of merging branches, rebasing moves your entire branch to begin on the tip of another branch. To rebase, you would run:
git rebase master
This can help avoid unnecessary merge commits, resulting in a cleaner project history.
Stashing Changes
If you want to save your changes temporarily without committing, you can stash them:
git stash
This command saves your local modifications. When you want to reapply your stashed changes, use:
git stash apply
Using Tags
Tags are a way to mark specific points in the repository’s history as significant, such as releases. You can create a tag with:
git tag v1.0
To share tags with remote repositories, use:
git push origin --tags
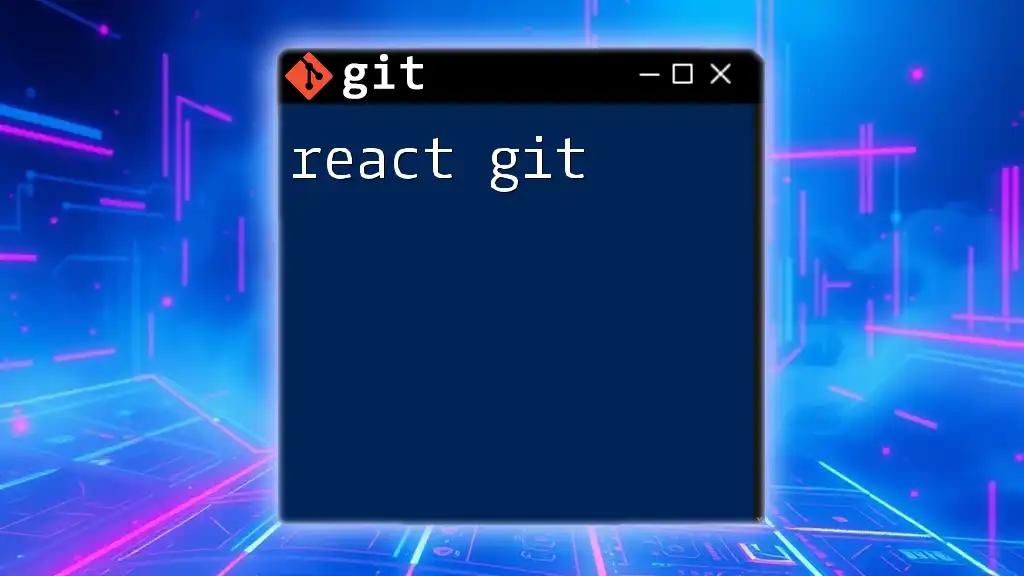
Best Practices for Using Git in CLI
Commit Often with Meaningful Messages
Make it a habit to commit frequently and use clear, descriptive messages. This practice not only keeps your project organized but also makes it easier for collaborators to understand the changes you’ve made.
Keep Your Branches Organized
Use a consistent naming convention for your branches to keep them understandable. For example, using prefixes like `feature/`, `bugfix/`, or `hotfix/` can provide immediate context about the branch's purpose.
Use .gitignore Files
Creating a `.gitignore` file in your repository allows you to specify files and directories that Git should ignore. This is essential for excluding files such as build directories or sensitive information, which should not be tracked. An example contents of `.gitignore` might include:
node_modules/
*.log
.env
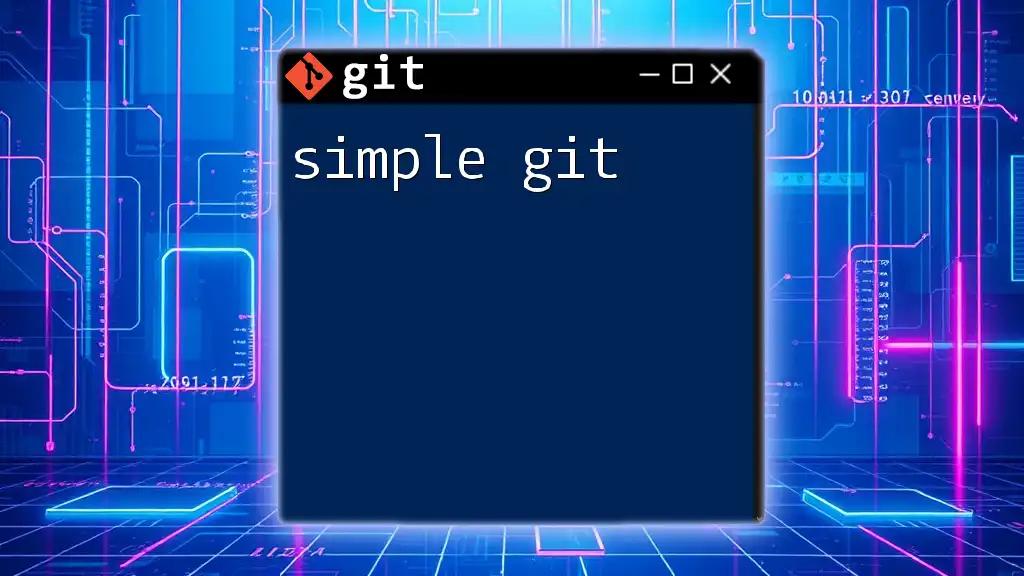
Conclusion
By mastering CLI Git, you arm yourself with a powerful toolset for effective version control, collaboration, and project management. The commands outlined in this guide are fundamental to navigating and utilizing Git efficiently.
Dive deeper into your Git journey, explore advanced features, and practice the commands to fully harness the capabilities of CLI Git. Whether you are a newcomer or looking to refine your skills, the command line is your gateway to powerful version control practices.
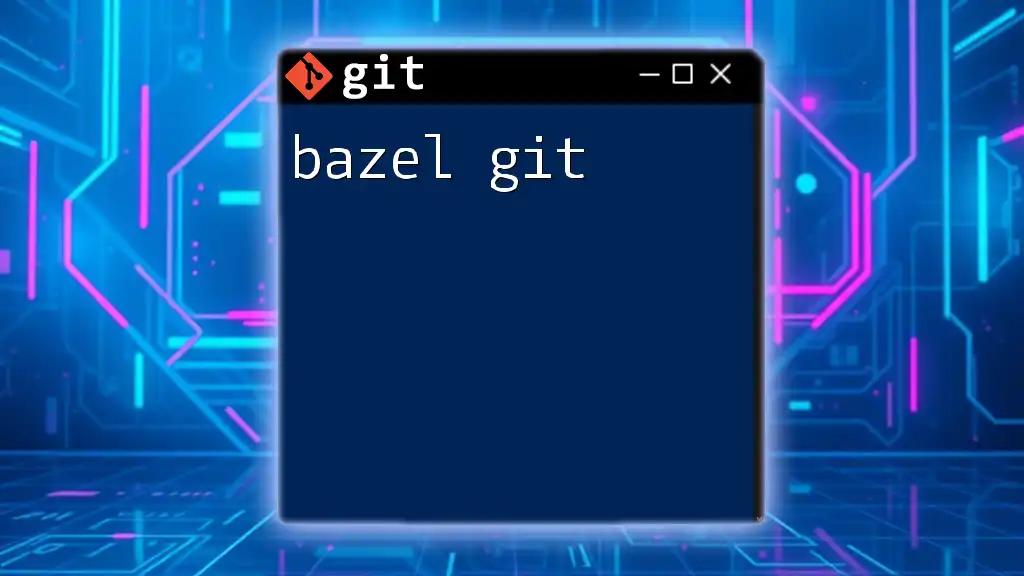
Call to Action
Stay informed and improve your Git skills by subscribing for more tutorials. Feel free to leave comments or questions; your journey towards mastering CLI Git starts now!
Additional Resources
For further learning, refer to the official [Git documentation](https://git-scm.com/doc) and consider exploring popular Git courses that cover both fundamental and advanced topics.